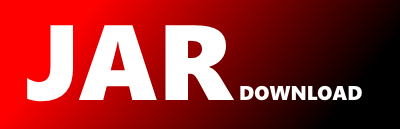
org.purejava.appindicator._GThreadFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libappindicator-gtk3-java-full Show documentation
Show all versions of libappindicator-gtk3-java-full Show documentation
Java bindings for libappindicator-gtk3 in 100% pure Java
// Generated by jextract
package org.purejava.appindicator;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.SegmentAllocator;
import java.lang.invoke.VarHandle;
/**
* {@snippet :
* struct _GThreadFunctions {
* union _GMutex* (*mutex_new)();
* void (*mutex_lock)(union _GMutex*);
* int (*mutex_trylock)(union _GMutex*);
* void (*mutex_unlock)(union _GMutex*);
* void (*mutex_free)(union _GMutex*);
* struct _GCond* (*cond_new)();
* void (*cond_signal)(struct _GCond*);
* void (*cond_broadcast)(struct _GCond*);
* void (*cond_wait)(struct _GCond*,union _GMutex*);
* int (*cond_timed_wait)(struct _GCond*,union _GMutex*,struct _GTimeVal*);
* void (*cond_free)(struct _GCond*);
* struct _GPrivate* (*private_new)(void (*)(void*));
* void* (*private_get)(struct _GPrivate*);
* void (*private_set)(struct _GPrivate*,void*);
* void (*thread_create)(void* (*)(void*),void*,unsigned long,int,int,enum GThreadPriority,void*,struct _GError**);
* void (*thread_yield)();
* void (*thread_join)(void*);
* void (*thread_exit)();
* void (*thread_set_priority)(void*,enum GThreadPriority);
* void (*thread_self)(void*);
* int (*thread_equal)(void*,void*);
* };
* }
*/
public class _GThreadFunctions {
public static MemoryLayout $LAYOUT() {
return constants$503.const$0;
}
/**
* {@snippet :
* union _GMutex* (*mutex_new)();
* }
*/
public interface mutex_new {
java.lang.foreign.MemorySegment apply();
static MemorySegment allocate(mutex_new fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$503.const$1, fi, constants$35.const$2, scope);
}
static mutex_new ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
return (java.lang.foreign.MemorySegment)constants$503.const$2.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle mutex_new$VH() {
return constants$503.const$3;
}
/**
* Getter for field:
* {@snippet :
* union _GMutex* (*mutex_new)();
* }
*/
public static MemorySegment mutex_new$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$503.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* union _GMutex* (*mutex_new)();
* }
*/
public static void mutex_new$set(MemorySegment seg, MemorySegment x) {
constants$503.const$3.set(seg, x);
}
public static MemorySegment mutex_new$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$503.const$3.get(seg.asSlice(index*sizeof()));
}
public static void mutex_new$set(MemorySegment seg, long index, MemorySegment x) {
constants$503.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static mutex_new mutex_new(MemorySegment segment, Arena scope) {
return mutex_new.ofAddress(mutex_new$get(segment), scope);
}
/**
* {@snippet :
* void (*mutex_lock)(union _GMutex*);
* }
*/
public interface mutex_lock {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(mutex_lock fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$503.const$4, fi, constants$13.const$1, scope);
}
static mutex_lock ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle mutex_lock$VH() {
return constants$503.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*mutex_lock)(union _GMutex*);
* }
*/
public static MemorySegment mutex_lock$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$503.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*mutex_lock)(union _GMutex*);
* }
*/
public static void mutex_lock$set(MemorySegment seg, MemorySegment x) {
constants$503.const$5.set(seg, x);
}
public static MemorySegment mutex_lock$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$503.const$5.get(seg.asSlice(index*sizeof()));
}
public static void mutex_lock$set(MemorySegment seg, long index, MemorySegment x) {
constants$503.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static mutex_lock mutex_lock(MemorySegment segment, Arena scope) {
return mutex_lock.ofAddress(mutex_lock$get(segment), scope);
}
/**
* {@snippet :
* int (*mutex_trylock)(union _GMutex*);
* }
*/
public interface mutex_trylock {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(mutex_trylock fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$504.const$0, fi, constants$10.const$5, scope);
}
static mutex_trylock ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle mutex_trylock$VH() {
return constants$504.const$1;
}
/**
* Getter for field:
* {@snippet :
* int (*mutex_trylock)(union _GMutex*);
* }
*/
public static MemorySegment mutex_trylock$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$504.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*mutex_trylock)(union _GMutex*);
* }
*/
public static void mutex_trylock$set(MemorySegment seg, MemorySegment x) {
constants$504.const$1.set(seg, x);
}
public static MemorySegment mutex_trylock$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$504.const$1.get(seg.asSlice(index*sizeof()));
}
public static void mutex_trylock$set(MemorySegment seg, long index, MemorySegment x) {
constants$504.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static mutex_trylock mutex_trylock(MemorySegment segment, Arena scope) {
return mutex_trylock.ofAddress(mutex_trylock$get(segment), scope);
}
/**
* {@snippet :
* void (*mutex_unlock)(union _GMutex*);
* }
*/
public interface mutex_unlock {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(mutex_unlock fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$504.const$2, fi, constants$13.const$1, scope);
}
static mutex_unlock ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle mutex_unlock$VH() {
return constants$504.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*mutex_unlock)(union _GMutex*);
* }
*/
public static MemorySegment mutex_unlock$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$504.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*mutex_unlock)(union _GMutex*);
* }
*/
public static void mutex_unlock$set(MemorySegment seg, MemorySegment x) {
constants$504.const$3.set(seg, x);
}
public static MemorySegment mutex_unlock$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$504.const$3.get(seg.asSlice(index*sizeof()));
}
public static void mutex_unlock$set(MemorySegment seg, long index, MemorySegment x) {
constants$504.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static mutex_unlock mutex_unlock(MemorySegment segment, Arena scope) {
return mutex_unlock.ofAddress(mutex_unlock$get(segment), scope);
}
/**
* {@snippet :
* void (*mutex_free)(union _GMutex*);
* }
*/
public interface mutex_free {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(mutex_free fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$504.const$4, fi, constants$13.const$1, scope);
}
static mutex_free ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle mutex_free$VH() {
return constants$504.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*mutex_free)(union _GMutex*);
* }
*/
public static MemorySegment mutex_free$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$504.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*mutex_free)(union _GMutex*);
* }
*/
public static void mutex_free$set(MemorySegment seg, MemorySegment x) {
constants$504.const$5.set(seg, x);
}
public static MemorySegment mutex_free$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$504.const$5.get(seg.asSlice(index*sizeof()));
}
public static void mutex_free$set(MemorySegment seg, long index, MemorySegment x) {
constants$504.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static mutex_free mutex_free(MemorySegment segment, Arena scope) {
return mutex_free.ofAddress(mutex_free$get(segment), scope);
}
/**
* {@snippet :
* struct _GCond* (*cond_new)();
* }
*/
public interface cond_new {
java.lang.foreign.MemorySegment apply();
static MemorySegment allocate(cond_new fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$505.const$0, fi, constants$35.const$2, scope);
}
static cond_new ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
return (java.lang.foreign.MemorySegment)constants$503.const$2.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle cond_new$VH() {
return constants$505.const$1;
}
/**
* Getter for field:
* {@snippet :
* struct _GCond* (*cond_new)();
* }
*/
public static MemorySegment cond_new$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$505.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GCond* (*cond_new)();
* }
*/
public static void cond_new$set(MemorySegment seg, MemorySegment x) {
constants$505.const$1.set(seg, x);
}
public static MemorySegment cond_new$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$505.const$1.get(seg.asSlice(index*sizeof()));
}
public static void cond_new$set(MemorySegment seg, long index, MemorySegment x) {
constants$505.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static cond_new cond_new(MemorySegment segment, Arena scope) {
return cond_new.ofAddress(cond_new$get(segment), scope);
}
/**
* {@snippet :
* void (*cond_signal)(struct _GCond*);
* }
*/
public interface cond_signal {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(cond_signal fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$505.const$2, fi, constants$13.const$1, scope);
}
static cond_signal ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle cond_signal$VH() {
return constants$505.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*cond_signal)(struct _GCond*);
* }
*/
public static MemorySegment cond_signal$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$505.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*cond_signal)(struct _GCond*);
* }
*/
public static void cond_signal$set(MemorySegment seg, MemorySegment x) {
constants$505.const$3.set(seg, x);
}
public static MemorySegment cond_signal$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$505.const$3.get(seg.asSlice(index*sizeof()));
}
public static void cond_signal$set(MemorySegment seg, long index, MemorySegment x) {
constants$505.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static cond_signal cond_signal(MemorySegment segment, Arena scope) {
return cond_signal.ofAddress(cond_signal$get(segment), scope);
}
/**
* {@snippet :
* void (*cond_broadcast)(struct _GCond*);
* }
*/
public interface cond_broadcast {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(cond_broadcast fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$505.const$4, fi, constants$13.const$1, scope);
}
static cond_broadcast ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle cond_broadcast$VH() {
return constants$505.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*cond_broadcast)(struct _GCond*);
* }
*/
public static MemorySegment cond_broadcast$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$505.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*cond_broadcast)(struct _GCond*);
* }
*/
public static void cond_broadcast$set(MemorySegment seg, MemorySegment x) {
constants$505.const$5.set(seg, x);
}
public static MemorySegment cond_broadcast$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$505.const$5.get(seg.asSlice(index*sizeof()));
}
public static void cond_broadcast$set(MemorySegment seg, long index, MemorySegment x) {
constants$505.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static cond_broadcast cond_broadcast(MemorySegment segment, Arena scope) {
return cond_broadcast.ofAddress(cond_broadcast$get(segment), scope);
}
/**
* {@snippet :
* void (*cond_wait)(struct _GCond*,union _GMutex*);
* }
*/
public interface cond_wait {
void apply(java.lang.foreign.MemorySegment tag, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(cond_wait fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$506.const$0, fi, constants$13.const$4, scope);
}
static cond_wait ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _tag, java.lang.foreign.MemorySegment _data) -> {
try {
constants$14.const$0.invokeExact(symbol, _tag, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle cond_wait$VH() {
return constants$506.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*cond_wait)(struct _GCond*,union _GMutex*);
* }
*/
public static MemorySegment cond_wait$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$506.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*cond_wait)(struct _GCond*,union _GMutex*);
* }
*/
public static void cond_wait$set(MemorySegment seg, MemorySegment x) {
constants$506.const$1.set(seg, x);
}
public static MemorySegment cond_wait$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$506.const$1.get(seg.asSlice(index*sizeof()));
}
public static void cond_wait$set(MemorySegment seg, long index, MemorySegment x) {
constants$506.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static cond_wait cond_wait(MemorySegment segment, Arena scope) {
return cond_wait.ofAddress(cond_wait$get(segment), scope);
}
/**
* {@snippet :
* int (*cond_timed_wait)(struct _GCond*,union _GMutex*,struct _GTimeVal*);
* }
*/
public interface cond_timed_wait {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(cond_timed_wait fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$506.const$2, fi, constants$12.const$2, scope);
}
static cond_timed_wait ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle cond_timed_wait$VH() {
return constants$506.const$3;
}
/**
* Getter for field:
* {@snippet :
* int (*cond_timed_wait)(struct _GCond*,union _GMutex*,struct _GTimeVal*);
* }
*/
public static MemorySegment cond_timed_wait$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$506.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*cond_timed_wait)(struct _GCond*,union _GMutex*,struct _GTimeVal*);
* }
*/
public static void cond_timed_wait$set(MemorySegment seg, MemorySegment x) {
constants$506.const$3.set(seg, x);
}
public static MemorySegment cond_timed_wait$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$506.const$3.get(seg.asSlice(index*sizeof()));
}
public static void cond_timed_wait$set(MemorySegment seg, long index, MemorySegment x) {
constants$506.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static cond_timed_wait cond_timed_wait(MemorySegment segment, Arena scope) {
return cond_timed_wait.ofAddress(cond_timed_wait$get(segment), scope);
}
/**
* {@snippet :
* void (*cond_free)(struct _GCond*);
* }
*/
public interface cond_free {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(cond_free fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$506.const$4, fi, constants$13.const$1, scope);
}
static cond_free ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle cond_free$VH() {
return constants$506.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*cond_free)(struct _GCond*);
* }
*/
public static MemorySegment cond_free$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$506.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*cond_free)(struct _GCond*);
* }
*/
public static void cond_free$set(MemorySegment seg, MemorySegment x) {
constants$506.const$5.set(seg, x);
}
public static MemorySegment cond_free$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$506.const$5.get(seg.asSlice(index*sizeof()));
}
public static void cond_free$set(MemorySegment seg, long index, MemorySegment x) {
constants$506.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static cond_free cond_free(MemorySegment segment, Arena scope) {
return cond_free.ofAddress(cond_free$get(segment), scope);
}
/**
* {@snippet :
* struct _GPrivate* (*private_new)(void (*)(void*));
* }
*/
public interface private_new {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(private_new fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$507.const$0, fi, constants$5.const$2, scope);
}
static private_new ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle private_new$VH() {
return constants$507.const$1;
}
/**
* Getter for field:
* {@snippet :
* struct _GPrivate* (*private_new)(void (*)(void*));
* }
*/
public static MemorySegment private_new$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$507.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* struct _GPrivate* (*private_new)(void (*)(void*));
* }
*/
public static void private_new$set(MemorySegment seg, MemorySegment x) {
constants$507.const$1.set(seg, x);
}
public static MemorySegment private_new$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$507.const$1.get(seg.asSlice(index*sizeof()));
}
public static void private_new$set(MemorySegment seg, long index, MemorySegment x) {
constants$507.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static private_new private_new(MemorySegment segment, Arena scope) {
return private_new.ofAddress(private_new$get(segment), scope);
}
/**
* {@snippet :
* void* (*private_get)(struct _GPrivate*);
* }
*/
public interface private_get {
java.lang.foreign.MemorySegment apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(private_get fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$507.const$2, fi, constants$5.const$2, scope);
}
static private_get ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (java.lang.foreign.MemorySegment)constants$99.const$0.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle private_get$VH() {
return constants$507.const$3;
}
/**
* Getter for field:
* {@snippet :
* void* (*private_get)(struct _GPrivate*);
* }
*/
public static MemorySegment private_get$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$507.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void* (*private_get)(struct _GPrivate*);
* }
*/
public static void private_get$set(MemorySegment seg, MemorySegment x) {
constants$507.const$3.set(seg, x);
}
public static MemorySegment private_get$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$507.const$3.get(seg.asSlice(index*sizeof()));
}
public static void private_get$set(MemorySegment seg, long index, MemorySegment x) {
constants$507.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static private_get private_get(MemorySegment segment, Arena scope) {
return private_get.ofAddress(private_get$get(segment), scope);
}
/**
* {@snippet :
* void (*private_set)(struct _GPrivate*,void*);
* }
*/
public interface private_set {
void apply(java.lang.foreign.MemorySegment tag, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(private_set fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$507.const$4, fi, constants$13.const$4, scope);
}
static private_set ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _tag, java.lang.foreign.MemorySegment _data) -> {
try {
constants$14.const$0.invokeExact(symbol, _tag, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle private_set$VH() {
return constants$507.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*private_set)(struct _GPrivate*,void*);
* }
*/
public static MemorySegment private_set$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$507.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*private_set)(struct _GPrivate*,void*);
* }
*/
public static void private_set$set(MemorySegment seg, MemorySegment x) {
constants$507.const$5.set(seg, x);
}
public static MemorySegment private_set$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$507.const$5.get(seg.asSlice(index*sizeof()));
}
public static void private_set$set(MemorySegment seg, long index, MemorySegment x) {
constants$507.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static private_set private_set(MemorySegment segment, Arena scope) {
return private_set.ofAddress(private_set$get(segment), scope);
}
/**
* {@snippet :
* void (*thread_create)(void* (*)(void*),void*,unsigned long,int,int,enum GThreadPriority,void*,struct _GError**);
* }
*/
public interface thread_create {
void apply(java.lang.foreign.MemorySegment _x0, java.lang.foreign.MemorySegment _x1, long _x2, int _x3, int _x4, int _x5, java.lang.foreign.MemorySegment _x6, java.lang.foreign.MemorySegment _x7);
static MemorySegment allocate(thread_create fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$508.const$1, fi, constants$508.const$0, scope);
}
static thread_create ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, java.lang.foreign.MemorySegment __x1, long __x2, int __x3, int __x4, int __x5, java.lang.foreign.MemorySegment __x6, java.lang.foreign.MemorySegment __x7) -> {
try {
constants$508.const$2.invokeExact(symbol, __x0, __x1, __x2, __x3, __x4, __x5, __x6, __x7);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_create$VH() {
return constants$508.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*thread_create)(void* (*)(void*),void*,unsigned long,int,int,enum GThreadPriority,void*,struct _GError**);
* }
*/
public static MemorySegment thread_create$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$508.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*thread_create)(void* (*)(void*),void*,unsigned long,int,int,enum GThreadPriority,void*,struct _GError**);
* }
*/
public static void thread_create$set(MemorySegment seg, MemorySegment x) {
constants$508.const$3.set(seg, x);
}
public static MemorySegment thread_create$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$508.const$3.get(seg.asSlice(index*sizeof()));
}
public static void thread_create$set(MemorySegment seg, long index, MemorySegment x) {
constants$508.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static thread_create thread_create(MemorySegment segment, Arena scope) {
return thread_create.ofAddress(thread_create$get(segment), scope);
}
/**
* {@snippet :
* void (*thread_yield)();
* }
*/
public interface thread_yield {
void apply();
static MemorySegment allocate(thread_yield fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$508.const$4, fi, constants$7.const$5, scope);
}
static thread_yield ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_yield$VH() {
return constants$508.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*thread_yield)();
* }
*/
public static MemorySegment thread_yield$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$508.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*thread_yield)();
* }
*/
public static void thread_yield$set(MemorySegment seg, MemorySegment x) {
constants$508.const$5.set(seg, x);
}
public static MemorySegment thread_yield$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$508.const$5.get(seg.asSlice(index*sizeof()));
}
public static void thread_yield$set(MemorySegment seg, long index, MemorySegment x) {
constants$508.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static thread_yield thread_yield(MemorySegment segment, Arena scope) {
return thread_yield.ofAddress(thread_yield$get(segment), scope);
}
/**
* {@snippet :
* void (*thread_join)(void*);
* }
*/
public interface thread_join {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(thread_join fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$509.const$0, fi, constants$13.const$1, scope);
}
static thread_join ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_join$VH() {
return constants$509.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*thread_join)(void*);
* }
*/
public static MemorySegment thread_join$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$509.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*thread_join)(void*);
* }
*/
public static void thread_join$set(MemorySegment seg, MemorySegment x) {
constants$509.const$1.set(seg, x);
}
public static MemorySegment thread_join$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$509.const$1.get(seg.asSlice(index*sizeof()));
}
public static void thread_join$set(MemorySegment seg, long index, MemorySegment x) {
constants$509.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static thread_join thread_join(MemorySegment segment, Arena scope) {
return thread_join.ofAddress(thread_join$get(segment), scope);
}
/**
* {@snippet :
* void (*thread_exit)();
* }
*/
public interface thread_exit {
void apply();
static MemorySegment allocate(thread_exit fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$509.const$2, fi, constants$7.const$5, scope);
}
static thread_exit ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_exit$VH() {
return constants$509.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*thread_exit)();
* }
*/
public static MemorySegment thread_exit$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$509.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*thread_exit)();
* }
*/
public static void thread_exit$set(MemorySegment seg, MemorySegment x) {
constants$509.const$3.set(seg, x);
}
public static MemorySegment thread_exit$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$509.const$3.get(seg.asSlice(index*sizeof()));
}
public static void thread_exit$set(MemorySegment seg, long index, MemorySegment x) {
constants$509.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static thread_exit thread_exit(MemorySegment segment, Arena scope) {
return thread_exit.ofAddress(thread_exit$get(segment), scope);
}
/**
* {@snippet :
* void (*thread_set_priority)(void*,enum GThreadPriority);
* }
*/
public interface thread_set_priority {
void apply(java.lang.foreign.MemorySegment _x0, int _x1);
static MemorySegment allocate(thread_set_priority fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$509.const$4, fi, constants$40.const$2, scope);
}
static thread_set_priority ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1) -> {
try {
constants$509.const$5.invokeExact(symbol, __x0, __x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_set_priority$VH() {
return constants$510.const$0;
}
/**
* Getter for field:
* {@snippet :
* void (*thread_set_priority)(void*,enum GThreadPriority);
* }
*/
public static MemorySegment thread_set_priority$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$510.const$0.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*thread_set_priority)(void*,enum GThreadPriority);
* }
*/
public static void thread_set_priority$set(MemorySegment seg, MemorySegment x) {
constants$510.const$0.set(seg, x);
}
public static MemorySegment thread_set_priority$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$510.const$0.get(seg.asSlice(index*sizeof()));
}
public static void thread_set_priority$set(MemorySegment seg, long index, MemorySegment x) {
constants$510.const$0.set(seg.asSlice(index*sizeof()), x);
}
public static thread_set_priority thread_set_priority(MemorySegment segment, Arena scope) {
return thread_set_priority.ofAddress(thread_set_priority$get(segment), scope);
}
/**
* {@snippet :
* void (*thread_self)(void*);
* }
*/
public interface thread_self {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(thread_self fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$510.const$1, fi, constants$13.const$1, scope);
}
static thread_self ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_self$VH() {
return constants$510.const$2;
}
/**
* Getter for field:
* {@snippet :
* void (*thread_self)(void*);
* }
*/
public static MemorySegment thread_self$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$510.const$2.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*thread_self)(void*);
* }
*/
public static void thread_self$set(MemorySegment seg, MemorySegment x) {
constants$510.const$2.set(seg, x);
}
public static MemorySegment thread_self$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$510.const$2.get(seg.asSlice(index*sizeof()));
}
public static void thread_self$set(MemorySegment seg, long index, MemorySegment x) {
constants$510.const$2.set(seg.asSlice(index*sizeof()), x);
}
public static thread_self thread_self(MemorySegment segment, Arena scope) {
return thread_self.ofAddress(thread_self$get(segment), scope);
}
/**
* {@snippet :
* int (*thread_equal)(void*,void*);
* }
*/
public interface thread_equal {
int apply(java.lang.foreign.MemorySegment filter_info, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(thread_equal fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$510.const$3, fi, constants$9.const$0, scope);
}
static thread_equal ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _filter_info, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$1.invokeExact(symbol, _filter_info, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle thread_equal$VH() {
return constants$510.const$4;
}
/**
* Getter for field:
* {@snippet :
* int (*thread_equal)(void*,void*);
* }
*/
public static MemorySegment thread_equal$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$510.const$4.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*thread_equal)(void*,void*);
* }
*/
public static void thread_equal$set(MemorySegment seg, MemorySegment x) {
constants$510.const$4.set(seg, x);
}
public static MemorySegment thread_equal$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$510.const$4.get(seg.asSlice(index*sizeof()));
}
public static void thread_equal$set(MemorySegment seg, long index, MemorySegment x) {
constants$510.const$4.set(seg.asSlice(index*sizeof()), x);
}
public static thread_equal thread_equal(MemorySegment segment, Arena scope) {
return thread_equal.ofAddress(thread_equal$get(segment), scope);
}
public static long sizeof() { return $LAYOUT().byteSize(); }
public static MemorySegment allocate(SegmentAllocator allocator) { return allocator.allocate($LAYOUT()); }
public static MemorySegment allocateArray(long len, SegmentAllocator allocator) {
return allocator.allocate(MemoryLayout.sequenceLayout(len, $LAYOUT()));
}
public static MemorySegment ofAddress(MemorySegment addr, Arena scope) { return RuntimeHelper.asArray(addr, $LAYOUT(), 1, scope); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy