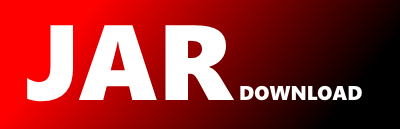
org.purejava.appindicator._GtkIMContextClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libappindicator-gtk3-java-full Show documentation
Show all versions of libappindicator-gtk3-java-full Show documentation
Java bindings for libappindicator-gtk3 in 100% pure Java
// Generated by jextract
package org.purejava.appindicator;
import java.lang.foreign.Arena;
import java.lang.foreign.MemoryLayout;
import java.lang.foreign.MemorySegment;
import java.lang.foreign.SegmentAllocator;
import java.lang.invoke.VarHandle;
/**
* {@snippet :
* struct _GtkIMContextClass {
* struct _GObjectClass parent_class;
* void (*preedit_start)(struct _GtkIMContext*);
* void (*preedit_end)(struct _GtkIMContext*);
* void (*preedit_changed)(struct _GtkIMContext*);
* void (*commit)(struct _GtkIMContext*,char*);
* int (*retrieve_surrounding)(struct _GtkIMContext*);
* int (*delete_surrounding)(struct _GtkIMContext*,int,int);
* void (*set_client_window)(struct _GtkIMContext*,struct _GdkWindow*);
* void (*get_preedit_string)(struct _GtkIMContext*,char**,struct _PangoAttrList**,int*);
* int (*filter_keypress)(struct _GtkIMContext*,struct _GdkEventKey*);
* void (*focus_in)(struct _GtkIMContext*);
* void (*focus_out)(struct _GtkIMContext*);
* void (*reset)(struct _GtkIMContext*);
* void (*set_cursor_location)(struct _GtkIMContext*,struct _cairo_rectangle_int*);
* void (*set_use_preedit)(struct _GtkIMContext*,int);
* void (*set_surrounding)(struct _GtkIMContext*,char*,int,int);
* int (*get_surrounding)(struct _GtkIMContext*,char**,int*);
* void (*_gtk_reserved1)();
* void (*_gtk_reserved2)();
* void (*_gtk_reserved3)();
* void (*_gtk_reserved4)();
* void (*_gtk_reserved5)();
* void (*_gtk_reserved6)();
* };
* }
*/
public class _GtkIMContextClass {
public static MemoryLayout $LAYOUT() {
return constants$2402.const$5;
}
public static MemorySegment parent_class$slice(MemorySegment seg) {
return seg.asSlice(0, 136);
}
/**
* {@snippet :
* void (*preedit_start)(struct _GtkIMContext*);
* }
*/
public interface preedit_start {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(preedit_start fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2403.const$0, fi, constants$13.const$1, scope);
}
static preedit_start ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle preedit_start$VH() {
return constants$2403.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*preedit_start)(struct _GtkIMContext*);
* }
*/
public static MemorySegment preedit_start$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2403.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*preedit_start)(struct _GtkIMContext*);
* }
*/
public static void preedit_start$set(MemorySegment seg, MemorySegment x) {
constants$2403.const$1.set(seg, x);
}
public static MemorySegment preedit_start$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2403.const$1.get(seg.asSlice(index*sizeof()));
}
public static void preedit_start$set(MemorySegment seg, long index, MemorySegment x) {
constants$2403.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static preedit_start preedit_start(MemorySegment segment, Arena scope) {
return preedit_start.ofAddress(preedit_start$get(segment), scope);
}
/**
* {@snippet :
* void (*preedit_end)(struct _GtkIMContext*);
* }
*/
public interface preedit_end {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(preedit_end fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2403.const$2, fi, constants$13.const$1, scope);
}
static preedit_end ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle preedit_end$VH() {
return constants$2403.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*preedit_end)(struct _GtkIMContext*);
* }
*/
public static MemorySegment preedit_end$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2403.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*preedit_end)(struct _GtkIMContext*);
* }
*/
public static void preedit_end$set(MemorySegment seg, MemorySegment x) {
constants$2403.const$3.set(seg, x);
}
public static MemorySegment preedit_end$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2403.const$3.get(seg.asSlice(index*sizeof()));
}
public static void preedit_end$set(MemorySegment seg, long index, MemorySegment x) {
constants$2403.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static preedit_end preedit_end(MemorySegment segment, Arena scope) {
return preedit_end.ofAddress(preedit_end$get(segment), scope);
}
/**
* {@snippet :
* void (*preedit_changed)(struct _GtkIMContext*);
* }
*/
public interface preedit_changed {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(preedit_changed fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2403.const$4, fi, constants$13.const$1, scope);
}
static preedit_changed ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle preedit_changed$VH() {
return constants$2403.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*preedit_changed)(struct _GtkIMContext*);
* }
*/
public static MemorySegment preedit_changed$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2403.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*preedit_changed)(struct _GtkIMContext*);
* }
*/
public static void preedit_changed$set(MemorySegment seg, MemorySegment x) {
constants$2403.const$5.set(seg, x);
}
public static MemorySegment preedit_changed$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2403.const$5.get(seg.asSlice(index*sizeof()));
}
public static void preedit_changed$set(MemorySegment seg, long index, MemorySegment x) {
constants$2403.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static preedit_changed preedit_changed(MemorySegment segment, Arena scope) {
return preedit_changed.ofAddress(preedit_changed$get(segment), scope);
}
/**
* {@snippet :
* void (*commit)(struct _GtkIMContext*,char*);
* }
*/
public interface commit {
void apply(java.lang.foreign.MemorySegment tag, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(commit fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2404.const$0, fi, constants$13.const$4, scope);
}
static commit ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _tag, java.lang.foreign.MemorySegment _data) -> {
try {
constants$14.const$0.invokeExact(symbol, _tag, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle commit$VH() {
return constants$2404.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*commit)(struct _GtkIMContext*,char*);
* }
*/
public static MemorySegment commit$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2404.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*commit)(struct _GtkIMContext*,char*);
* }
*/
public static void commit$set(MemorySegment seg, MemorySegment x) {
constants$2404.const$1.set(seg, x);
}
public static MemorySegment commit$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2404.const$1.get(seg.asSlice(index*sizeof()));
}
public static void commit$set(MemorySegment seg, long index, MemorySegment x) {
constants$2404.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static commit commit(MemorySegment segment, Arena scope) {
return commit.ofAddress(commit$get(segment), scope);
}
/**
* {@snippet :
* int (*retrieve_surrounding)(struct _GtkIMContext*);
* }
*/
public interface retrieve_surrounding {
int apply(java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(retrieve_surrounding fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2404.const$2, fi, constants$10.const$5, scope);
}
static retrieve_surrounding ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$14.const$2.invokeExact(symbol, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle retrieve_surrounding$VH() {
return constants$2404.const$3;
}
/**
* Getter for field:
* {@snippet :
* int (*retrieve_surrounding)(struct _GtkIMContext*);
* }
*/
public static MemorySegment retrieve_surrounding$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2404.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*retrieve_surrounding)(struct _GtkIMContext*);
* }
*/
public static void retrieve_surrounding$set(MemorySegment seg, MemorySegment x) {
constants$2404.const$3.set(seg, x);
}
public static MemorySegment retrieve_surrounding$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2404.const$3.get(seg.asSlice(index*sizeof()));
}
public static void retrieve_surrounding$set(MemorySegment seg, long index, MemorySegment x) {
constants$2404.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static retrieve_surrounding retrieve_surrounding(MemorySegment segment, Arena scope) {
return retrieve_surrounding.ofAddress(retrieve_surrounding$get(segment), scope);
}
/**
* {@snippet :
* int (*delete_surrounding)(struct _GtkIMContext*,int,int);
* }
*/
public interface delete_surrounding {
int apply(java.lang.foreign.MemorySegment _x0, int _x1, int _x2);
static MemorySegment allocate(delete_surrounding fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2404.const$4, fi, constants$49.const$0, scope);
}
static delete_surrounding ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, int __x1, int __x2) -> {
try {
return (int)constants$247.const$2.invokeExact(symbol, __x0, __x1, __x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle delete_surrounding$VH() {
return constants$2404.const$5;
}
/**
* Getter for field:
* {@snippet :
* int (*delete_surrounding)(struct _GtkIMContext*,int,int);
* }
*/
public static MemorySegment delete_surrounding$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2404.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*delete_surrounding)(struct _GtkIMContext*,int,int);
* }
*/
public static void delete_surrounding$set(MemorySegment seg, MemorySegment x) {
constants$2404.const$5.set(seg, x);
}
public static MemorySegment delete_surrounding$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2404.const$5.get(seg.asSlice(index*sizeof()));
}
public static void delete_surrounding$set(MemorySegment seg, long index, MemorySegment x) {
constants$2404.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static delete_surrounding delete_surrounding(MemorySegment segment, Arena scope) {
return delete_surrounding.ofAddress(delete_surrounding$get(segment), scope);
}
/**
* {@snippet :
* void (*set_client_window)(struct _GtkIMContext*,struct _GdkWindow*);
* }
*/
public interface set_client_window {
void apply(java.lang.foreign.MemorySegment tag, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(set_client_window fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2405.const$0, fi, constants$13.const$4, scope);
}
static set_client_window ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _tag, java.lang.foreign.MemorySegment _data) -> {
try {
constants$14.const$0.invokeExact(symbol, _tag, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle set_client_window$VH() {
return constants$2405.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*set_client_window)(struct _GtkIMContext*,struct _GdkWindow*);
* }
*/
public static MemorySegment set_client_window$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2405.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*set_client_window)(struct _GtkIMContext*,struct _GdkWindow*);
* }
*/
public static void set_client_window$set(MemorySegment seg, MemorySegment x) {
constants$2405.const$1.set(seg, x);
}
public static MemorySegment set_client_window$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2405.const$1.get(seg.asSlice(index*sizeof()));
}
public static void set_client_window$set(MemorySegment seg, long index, MemorySegment x) {
constants$2405.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static set_client_window set_client_window(MemorySegment segment, Arena scope) {
return set_client_window.ofAddress(set_client_window$get(segment), scope);
}
/**
* {@snippet :
* void (*get_preedit_string)(struct _GtkIMContext*,char**,struct _PangoAttrList**,int*);
* }
*/
public interface get_preedit_string {
void apply(java.lang.foreign.MemorySegment model, java.lang.foreign.MemorySegment path, java.lang.foreign.MemorySegment iter, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(get_preedit_string fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2405.const$2, fi, constants$42.const$1, scope);
}
static get_preedit_string ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _model, java.lang.foreign.MemorySegment _path, java.lang.foreign.MemorySegment _iter, java.lang.foreign.MemorySegment _data) -> {
try {
constants$259.const$4.invokeExact(symbol, _model, _path, _iter, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_preedit_string$VH() {
return constants$2405.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*get_preedit_string)(struct _GtkIMContext*,char**,struct _PangoAttrList**,int*);
* }
*/
public static MemorySegment get_preedit_string$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2405.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*get_preedit_string)(struct _GtkIMContext*,char**,struct _PangoAttrList**,int*);
* }
*/
public static void get_preedit_string$set(MemorySegment seg, MemorySegment x) {
constants$2405.const$3.set(seg, x);
}
public static MemorySegment get_preedit_string$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2405.const$3.get(seg.asSlice(index*sizeof()));
}
public static void get_preedit_string$set(MemorySegment seg, long index, MemorySegment x) {
constants$2405.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static get_preedit_string get_preedit_string(MemorySegment segment, Arena scope) {
return get_preedit_string.ofAddress(get_preedit_string$get(segment), scope);
}
/**
* {@snippet :
* int (*filter_keypress)(struct _GtkIMContext*,struct _GdkEventKey*);
* }
*/
public interface filter_keypress {
int apply(java.lang.foreign.MemorySegment filter_info, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(filter_keypress fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2405.const$4, fi, constants$9.const$0, scope);
}
static filter_keypress ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _filter_info, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$1.invokeExact(symbol, _filter_info, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle filter_keypress$VH() {
return constants$2405.const$5;
}
/**
* Getter for field:
* {@snippet :
* int (*filter_keypress)(struct _GtkIMContext*,struct _GdkEventKey*);
* }
*/
public static MemorySegment filter_keypress$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2405.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*filter_keypress)(struct _GtkIMContext*,struct _GdkEventKey*);
* }
*/
public static void filter_keypress$set(MemorySegment seg, MemorySegment x) {
constants$2405.const$5.set(seg, x);
}
public static MemorySegment filter_keypress$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2405.const$5.get(seg.asSlice(index*sizeof()));
}
public static void filter_keypress$set(MemorySegment seg, long index, MemorySegment x) {
constants$2405.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static filter_keypress filter_keypress(MemorySegment segment, Arena scope) {
return filter_keypress.ofAddress(filter_keypress$get(segment), scope);
}
/**
* {@snippet :
* void (*focus_in)(struct _GtkIMContext*);
* }
*/
public interface focus_in {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(focus_in fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2406.const$0, fi, constants$13.const$1, scope);
}
static focus_in ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle focus_in$VH() {
return constants$2406.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*focus_in)(struct _GtkIMContext*);
* }
*/
public static MemorySegment focus_in$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2406.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*focus_in)(struct _GtkIMContext*);
* }
*/
public static void focus_in$set(MemorySegment seg, MemorySegment x) {
constants$2406.const$1.set(seg, x);
}
public static MemorySegment focus_in$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2406.const$1.get(seg.asSlice(index*sizeof()));
}
public static void focus_in$set(MemorySegment seg, long index, MemorySegment x) {
constants$2406.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static focus_in focus_in(MemorySegment segment, Arena scope) {
return focus_in.ofAddress(focus_in$get(segment), scope);
}
/**
* {@snippet :
* void (*focus_out)(struct _GtkIMContext*);
* }
*/
public interface focus_out {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(focus_out fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2406.const$2, fi, constants$13.const$1, scope);
}
static focus_out ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle focus_out$VH() {
return constants$2406.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*focus_out)(struct _GtkIMContext*);
* }
*/
public static MemorySegment focus_out$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2406.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*focus_out)(struct _GtkIMContext*);
* }
*/
public static void focus_out$set(MemorySegment seg, MemorySegment x) {
constants$2406.const$3.set(seg, x);
}
public static MemorySegment focus_out$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2406.const$3.get(seg.asSlice(index*sizeof()));
}
public static void focus_out$set(MemorySegment seg, long index, MemorySegment x) {
constants$2406.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static focus_out focus_out(MemorySegment segment, Arena scope) {
return focus_out.ofAddress(focus_out$get(segment), scope);
}
/**
* {@snippet :
* void (*reset)(struct _GtkIMContext*);
* }
*/
public interface reset {
void apply(java.lang.foreign.MemorySegment display);
static MemorySegment allocate(reset fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2406.const$4, fi, constants$13.const$1, scope);
}
static reset ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _display) -> {
try {
constants$13.const$3.invokeExact(symbol, _display);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle reset$VH() {
return constants$2406.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*reset)(struct _GtkIMContext*);
* }
*/
public static MemorySegment reset$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2406.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*reset)(struct _GtkIMContext*);
* }
*/
public static void reset$set(MemorySegment seg, MemorySegment x) {
constants$2406.const$5.set(seg, x);
}
public static MemorySegment reset$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2406.const$5.get(seg.asSlice(index*sizeof()));
}
public static void reset$set(MemorySegment seg, long index, MemorySegment x) {
constants$2406.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static reset reset(MemorySegment segment, Arena scope) {
return reset.ofAddress(reset$get(segment), scope);
}
/**
* {@snippet :
* void (*set_cursor_location)(struct _GtkIMContext*,struct _cairo_rectangle_int*);
* }
*/
public interface set_cursor_location {
void apply(java.lang.foreign.MemorySegment tag, java.lang.foreign.MemorySegment data);
static MemorySegment allocate(set_cursor_location fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2407.const$0, fi, constants$13.const$4, scope);
}
static set_cursor_location ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _tag, java.lang.foreign.MemorySegment _data) -> {
try {
constants$14.const$0.invokeExact(symbol, _tag, _data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle set_cursor_location$VH() {
return constants$2407.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*set_cursor_location)(struct _GtkIMContext*,struct _cairo_rectangle_int*);
* }
*/
public static MemorySegment set_cursor_location$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2407.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*set_cursor_location)(struct _GtkIMContext*,struct _cairo_rectangle_int*);
* }
*/
public static void set_cursor_location$set(MemorySegment seg, MemorySegment x) {
constants$2407.const$1.set(seg, x);
}
public static MemorySegment set_cursor_location$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2407.const$1.get(seg.asSlice(index*sizeof()));
}
public static void set_cursor_location$set(MemorySegment seg, long index, MemorySegment x) {
constants$2407.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static set_cursor_location set_cursor_location(MemorySegment segment, Arena scope) {
return set_cursor_location.ofAddress(set_cursor_location$get(segment), scope);
}
/**
* {@snippet :
* void (*set_use_preedit)(struct _GtkIMContext*,int);
* }
*/
public interface set_use_preedit {
void apply(java.lang.foreign.MemorySegment colors, int n_colors);
static MemorySegment allocate(set_use_preedit fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2407.const$2, fi, constants$40.const$2, scope);
}
static set_use_preedit ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _colors, int _n_colors) -> {
try {
constants$509.const$5.invokeExact(symbol, _colors, _n_colors);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle set_use_preedit$VH() {
return constants$2407.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*set_use_preedit)(struct _GtkIMContext*,int);
* }
*/
public static MemorySegment set_use_preedit$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2407.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*set_use_preedit)(struct _GtkIMContext*,int);
* }
*/
public static void set_use_preedit$set(MemorySegment seg, MemorySegment x) {
constants$2407.const$3.set(seg, x);
}
public static MemorySegment set_use_preedit$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2407.const$3.get(seg.asSlice(index*sizeof()));
}
public static void set_use_preedit$set(MemorySegment seg, long index, MemorySegment x) {
constants$2407.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static set_use_preedit set_use_preedit(MemorySegment segment, Arena scope) {
return set_use_preedit.ofAddress(set_use_preedit$get(segment), scope);
}
/**
* {@snippet :
* void (*set_surrounding)(struct _GtkIMContext*,char*,int,int);
* }
*/
public interface set_surrounding {
void apply(java.lang.foreign.MemorySegment _x0, java.lang.foreign.MemorySegment _x1, int _x2, int _x3);
static MemorySegment allocate(set_surrounding fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2407.const$4, fi, constants$1043.const$2, scope);
}
static set_surrounding ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment __x0, java.lang.foreign.MemorySegment __x1, int __x2, int __x3) -> {
try {
constants$1644.const$0.invokeExact(symbol, __x0, __x1, __x2, __x3);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle set_surrounding$VH() {
return constants$2407.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*set_surrounding)(struct _GtkIMContext*,char*,int,int);
* }
*/
public static MemorySegment set_surrounding$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2407.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*set_surrounding)(struct _GtkIMContext*,char*,int,int);
* }
*/
public static void set_surrounding$set(MemorySegment seg, MemorySegment x) {
constants$2407.const$5.set(seg, x);
}
public static MemorySegment set_surrounding$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2407.const$5.get(seg.asSlice(index*sizeof()));
}
public static void set_surrounding$set(MemorySegment seg, long index, MemorySegment x) {
constants$2407.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static set_surrounding set_surrounding(MemorySegment segment, Arena scope) {
return set_surrounding.ofAddress(set_surrounding$get(segment), scope);
}
/**
* {@snippet :
* int (*get_surrounding)(struct _GtkIMContext*,char**,int*);
* }
*/
public interface get_surrounding {
int apply(java.lang.foreign.MemorySegment a, java.lang.foreign.MemorySegment b, java.lang.foreign.MemorySegment user_data);
static MemorySegment allocate(get_surrounding fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2408.const$0, fi, constants$12.const$2, scope);
}
static get_surrounding ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return (java.lang.foreign.MemorySegment _a, java.lang.foreign.MemorySegment _b, java.lang.foreign.MemorySegment _user_data) -> {
try {
return (int)constants$12.const$4.invokeExact(symbol, _a, _b, _user_data);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle get_surrounding$VH() {
return constants$2408.const$1;
}
/**
* Getter for field:
* {@snippet :
* int (*get_surrounding)(struct _GtkIMContext*,char**,int*);
* }
*/
public static MemorySegment get_surrounding$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2408.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* int (*get_surrounding)(struct _GtkIMContext*,char**,int*);
* }
*/
public static void get_surrounding$set(MemorySegment seg, MemorySegment x) {
constants$2408.const$1.set(seg, x);
}
public static MemorySegment get_surrounding$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2408.const$1.get(seg.asSlice(index*sizeof()));
}
public static void get_surrounding$set(MemorySegment seg, long index, MemorySegment x) {
constants$2408.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static get_surrounding get_surrounding(MemorySegment segment, Arena scope) {
return get_surrounding.ofAddress(get_surrounding$get(segment), scope);
}
/**
* {@snippet :
* void (*_gtk_reserved1)();
* }
*/
public interface _gtk_reserved1 {
void apply();
static MemorySegment allocate(_gtk_reserved1 fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2408.const$2, fi, constants$7.const$5, scope);
}
static _gtk_reserved1 ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle _gtk_reserved1$VH() {
return constants$2408.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*_gtk_reserved1)();
* }
*/
public static MemorySegment _gtk_reserved1$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2408.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*_gtk_reserved1)();
* }
*/
public static void _gtk_reserved1$set(MemorySegment seg, MemorySegment x) {
constants$2408.const$3.set(seg, x);
}
public static MemorySegment _gtk_reserved1$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2408.const$3.get(seg.asSlice(index*sizeof()));
}
public static void _gtk_reserved1$set(MemorySegment seg, long index, MemorySegment x) {
constants$2408.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static _gtk_reserved1 _gtk_reserved1(MemorySegment segment, Arena scope) {
return _gtk_reserved1.ofAddress(_gtk_reserved1$get(segment), scope);
}
/**
* {@snippet :
* void (*_gtk_reserved2)();
* }
*/
public interface _gtk_reserved2 {
void apply();
static MemorySegment allocate(_gtk_reserved2 fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2408.const$4, fi, constants$7.const$5, scope);
}
static _gtk_reserved2 ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle _gtk_reserved2$VH() {
return constants$2408.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*_gtk_reserved2)();
* }
*/
public static MemorySegment _gtk_reserved2$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2408.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*_gtk_reserved2)();
* }
*/
public static void _gtk_reserved2$set(MemorySegment seg, MemorySegment x) {
constants$2408.const$5.set(seg, x);
}
public static MemorySegment _gtk_reserved2$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2408.const$5.get(seg.asSlice(index*sizeof()));
}
public static void _gtk_reserved2$set(MemorySegment seg, long index, MemorySegment x) {
constants$2408.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static _gtk_reserved2 _gtk_reserved2(MemorySegment segment, Arena scope) {
return _gtk_reserved2.ofAddress(_gtk_reserved2$get(segment), scope);
}
/**
* {@snippet :
* void (*_gtk_reserved3)();
* }
*/
public interface _gtk_reserved3 {
void apply();
static MemorySegment allocate(_gtk_reserved3 fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2409.const$0, fi, constants$7.const$5, scope);
}
static _gtk_reserved3 ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle _gtk_reserved3$VH() {
return constants$2409.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*_gtk_reserved3)();
* }
*/
public static MemorySegment _gtk_reserved3$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2409.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*_gtk_reserved3)();
* }
*/
public static void _gtk_reserved3$set(MemorySegment seg, MemorySegment x) {
constants$2409.const$1.set(seg, x);
}
public static MemorySegment _gtk_reserved3$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2409.const$1.get(seg.asSlice(index*sizeof()));
}
public static void _gtk_reserved3$set(MemorySegment seg, long index, MemorySegment x) {
constants$2409.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static _gtk_reserved3 _gtk_reserved3(MemorySegment segment, Arena scope) {
return _gtk_reserved3.ofAddress(_gtk_reserved3$get(segment), scope);
}
/**
* {@snippet :
* void (*_gtk_reserved4)();
* }
*/
public interface _gtk_reserved4 {
void apply();
static MemorySegment allocate(_gtk_reserved4 fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2409.const$2, fi, constants$7.const$5, scope);
}
static _gtk_reserved4 ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle _gtk_reserved4$VH() {
return constants$2409.const$3;
}
/**
* Getter for field:
* {@snippet :
* void (*_gtk_reserved4)();
* }
*/
public static MemorySegment _gtk_reserved4$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2409.const$3.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*_gtk_reserved4)();
* }
*/
public static void _gtk_reserved4$set(MemorySegment seg, MemorySegment x) {
constants$2409.const$3.set(seg, x);
}
public static MemorySegment _gtk_reserved4$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2409.const$3.get(seg.asSlice(index*sizeof()));
}
public static void _gtk_reserved4$set(MemorySegment seg, long index, MemorySegment x) {
constants$2409.const$3.set(seg.asSlice(index*sizeof()), x);
}
public static _gtk_reserved4 _gtk_reserved4(MemorySegment segment, Arena scope) {
return _gtk_reserved4.ofAddress(_gtk_reserved4$get(segment), scope);
}
/**
* {@snippet :
* void (*_gtk_reserved5)();
* }
*/
public interface _gtk_reserved5 {
void apply();
static MemorySegment allocate(_gtk_reserved5 fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2409.const$4, fi, constants$7.const$5, scope);
}
static _gtk_reserved5 ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle _gtk_reserved5$VH() {
return constants$2409.const$5;
}
/**
* Getter for field:
* {@snippet :
* void (*_gtk_reserved5)();
* }
*/
public static MemorySegment _gtk_reserved5$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2409.const$5.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*_gtk_reserved5)();
* }
*/
public static void _gtk_reserved5$set(MemorySegment seg, MemorySegment x) {
constants$2409.const$5.set(seg, x);
}
public static MemorySegment _gtk_reserved5$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2409.const$5.get(seg.asSlice(index*sizeof()));
}
public static void _gtk_reserved5$set(MemorySegment seg, long index, MemorySegment x) {
constants$2409.const$5.set(seg.asSlice(index*sizeof()), x);
}
public static _gtk_reserved5 _gtk_reserved5(MemorySegment segment, Arena scope) {
return _gtk_reserved5.ofAddress(_gtk_reserved5$get(segment), scope);
}
/**
* {@snippet :
* void (*_gtk_reserved6)();
* }
*/
public interface _gtk_reserved6 {
void apply();
static MemorySegment allocate(_gtk_reserved6 fi, Arena scope) {
return RuntimeHelper.upcallStub(constants$2410.const$0, fi, constants$7.const$5, scope);
}
static _gtk_reserved6 ofAddress(MemorySegment addr, Arena arena) {
MemorySegment symbol = addr.reinterpret(arena, null);
return () -> {
try {
constants$64.const$1.invokeExact(symbol);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
};
}
}
public static VarHandle _gtk_reserved6$VH() {
return constants$2410.const$1;
}
/**
* Getter for field:
* {@snippet :
* void (*_gtk_reserved6)();
* }
*/
public static MemorySegment _gtk_reserved6$get(MemorySegment seg) {
return (java.lang.foreign.MemorySegment)constants$2410.const$1.get(seg);
}
/**
* Setter for field:
* {@snippet :
* void (*_gtk_reserved6)();
* }
*/
public static void _gtk_reserved6$set(MemorySegment seg, MemorySegment x) {
constants$2410.const$1.set(seg, x);
}
public static MemorySegment _gtk_reserved6$get(MemorySegment seg, long index) {
return (java.lang.foreign.MemorySegment)constants$2410.const$1.get(seg.asSlice(index*sizeof()));
}
public static void _gtk_reserved6$set(MemorySegment seg, long index, MemorySegment x) {
constants$2410.const$1.set(seg.asSlice(index*sizeof()), x);
}
public static _gtk_reserved6 _gtk_reserved6(MemorySegment segment, Arena scope) {
return _gtk_reserved6.ofAddress(_gtk_reserved6$get(segment), scope);
}
public static long sizeof() { return $LAYOUT().byteSize(); }
public static MemorySegment allocate(SegmentAllocator allocator) { return allocator.allocate($LAYOUT()); }
public static MemorySegment allocateArray(long len, SegmentAllocator allocator) {
return allocator.allocate(MemoryLayout.sequenceLayout(len, $LAYOUT()));
}
public static MemorySegment ofAddress(MemorySegment addr, Arena scope) { return RuntimeHelper.asArray(addr, $LAYOUT(), 1, scope); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy