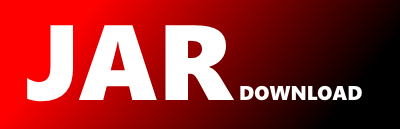
org.purejava.appindicator._AtkTableIface Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libappindicator-gtk3-java-full Show documentation
Show all versions of libappindicator-gtk3-java-full Show documentation
Java bindings for libappindicator-gtk3 in 100% pure Java
The newest version!
// Generated by jextract
package org.purejava.appindicator;
import java.lang.invoke.*;
import java.lang.foreign.*;
import java.nio.ByteOrder;
import java.util.*;
import java.util.function.*;
import java.util.stream.*;
import static java.lang.foreign.ValueLayout.*;
import static java.lang.foreign.MemoryLayout.PathElement.*;
/**
* {@snippet lang=c :
* struct _AtkTableIface {
* GTypeInterface parent;
* AtkObject *(*ref_at)(AtkTable *, gint, gint);
* gint (*get_index_at)(AtkTable *, gint, gint);
* gint (*get_column_at_index)(AtkTable *, gint);
* gint (*get_row_at_index)(AtkTable *, gint);
* gint (*get_n_columns)(AtkTable *);
* gint (*get_n_rows)(AtkTable *);
* gint (*get_column_extent_at)(AtkTable *, gint, gint);
* gint (*get_row_extent_at)(AtkTable *, gint, gint);
* AtkObject *(*get_caption)(AtkTable *);
* const gchar *(*get_column_description)(AtkTable *, gint);
* AtkObject *(*get_column_header)(AtkTable *, gint);
* const gchar *(*get_row_description)(AtkTable *, gint);
* AtkObject *(*get_row_header)(AtkTable *, gint);
* AtkObject *(*get_summary)(AtkTable *);
* void (*set_caption)(AtkTable *, AtkObject *);
* void (*set_column_description)(AtkTable *, gint, const gchar *);
* void (*set_column_header)(AtkTable *, gint, AtkObject *);
* void (*set_row_description)(AtkTable *, gint, const gchar *);
* void (*set_row_header)(AtkTable *, gint, AtkObject *);
* void (*set_summary)(AtkTable *, AtkObject *);
* gint (*get_selected_columns)(AtkTable *, gint **);
* gint (*get_selected_rows)(AtkTable *, gint **);
* gboolean (*is_column_selected)(AtkTable *, gint);
* gboolean (*is_row_selected)(AtkTable *, gint);
* gboolean (*is_selected)(AtkTable *, gint, gint);
* gboolean (*add_row_selection)(AtkTable *, gint);
* gboolean (*remove_row_selection)(AtkTable *, gint);
* gboolean (*add_column_selection)(AtkTable *, gint);
* gboolean (*remove_column_selection)(AtkTable *, gint);
* void (*row_inserted)(AtkTable *, gint, gint);
* void (*column_inserted)(AtkTable *, gint, gint);
* void (*row_deleted)(AtkTable *, gint, gint);
* void (*column_deleted)(AtkTable *, gint, gint);
* void (*row_reordered)(AtkTable *);
* void (*column_reordered)(AtkTable *);
* void (*model_changed)(AtkTable *);
* }
* }
*/
public class _AtkTableIface {
_AtkTableIface() {
// Should not be called directly
}
private static final GroupLayout $LAYOUT = MemoryLayout.structLayout(
_GTypeInterface.layout().withName("parent"),
app_indicator_h.C_POINTER.withName("ref_at"),
app_indicator_h.C_POINTER.withName("get_index_at"),
app_indicator_h.C_POINTER.withName("get_column_at_index"),
app_indicator_h.C_POINTER.withName("get_row_at_index"),
app_indicator_h.C_POINTER.withName("get_n_columns"),
app_indicator_h.C_POINTER.withName("get_n_rows"),
app_indicator_h.C_POINTER.withName("get_column_extent_at"),
app_indicator_h.C_POINTER.withName("get_row_extent_at"),
app_indicator_h.C_POINTER.withName("get_caption"),
app_indicator_h.C_POINTER.withName("get_column_description"),
app_indicator_h.C_POINTER.withName("get_column_header"),
app_indicator_h.C_POINTER.withName("get_row_description"),
app_indicator_h.C_POINTER.withName("get_row_header"),
app_indicator_h.C_POINTER.withName("get_summary"),
app_indicator_h.C_POINTER.withName("set_caption"),
app_indicator_h.C_POINTER.withName("set_column_description"),
app_indicator_h.C_POINTER.withName("set_column_header"),
app_indicator_h.C_POINTER.withName("set_row_description"),
app_indicator_h.C_POINTER.withName("set_row_header"),
app_indicator_h.C_POINTER.withName("set_summary"),
app_indicator_h.C_POINTER.withName("get_selected_columns"),
app_indicator_h.C_POINTER.withName("get_selected_rows"),
app_indicator_h.C_POINTER.withName("is_column_selected"),
app_indicator_h.C_POINTER.withName("is_row_selected"),
app_indicator_h.C_POINTER.withName("is_selected"),
app_indicator_h.C_POINTER.withName("add_row_selection"),
app_indicator_h.C_POINTER.withName("remove_row_selection"),
app_indicator_h.C_POINTER.withName("add_column_selection"),
app_indicator_h.C_POINTER.withName("remove_column_selection"),
app_indicator_h.C_POINTER.withName("row_inserted"),
app_indicator_h.C_POINTER.withName("column_inserted"),
app_indicator_h.C_POINTER.withName("row_deleted"),
app_indicator_h.C_POINTER.withName("column_deleted"),
app_indicator_h.C_POINTER.withName("row_reordered"),
app_indicator_h.C_POINTER.withName("column_reordered"),
app_indicator_h.C_POINTER.withName("model_changed")
).withName("_AtkTableIface");
/**
* The layout of this struct
*/
public static final GroupLayout layout() {
return $LAYOUT;
}
private static final GroupLayout parent$LAYOUT = (GroupLayout)$LAYOUT.select(groupElement("parent"));
/**
* Layout for field:
* {@snippet lang=c :
* GTypeInterface parent
* }
*/
public static final GroupLayout parent$layout() {
return parent$LAYOUT;
}
private static final long parent$OFFSET = 0;
/**
* Offset for field:
* {@snippet lang=c :
* GTypeInterface parent
* }
*/
public static final long parent$offset() {
return parent$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* GTypeInterface parent
* }
*/
public static MemorySegment parent(MemorySegment struct) {
return struct.asSlice(parent$OFFSET, parent$LAYOUT.byteSize());
}
/**
* Setter for field:
* {@snippet lang=c :
* GTypeInterface parent
* }
*/
public static void parent(MemorySegment struct, MemorySegment fieldValue) {
MemorySegment.copy(fieldValue, 0L, struct, parent$OFFSET, parent$LAYOUT.byteSize());
}
/**
* {@snippet lang=c :
* AtkObject *(*ref_at)(AtkTable *, gint, gint)
* }
*/
public class ref_at {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(ref_at.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(ref_at.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout ref_at$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("ref_at"));
/**
* Layout for field:
* {@snippet lang=c :
* AtkObject *(*ref_at)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout ref_at$layout() {
return ref_at$LAYOUT;
}
private static final long ref_at$OFFSET = 16;
/**
* Offset for field:
* {@snippet lang=c :
* AtkObject *(*ref_at)(AtkTable *, gint, gint)
* }
*/
public static final long ref_at$offset() {
return ref_at$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* AtkObject *(*ref_at)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment ref_at(MemorySegment struct) {
return struct.get(ref_at$LAYOUT, ref_at$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* AtkObject *(*ref_at)(AtkTable *, gint, gint)
* }
*/
public static void ref_at(MemorySegment struct, MemorySegment fieldValue) {
struct.set(ref_at$LAYOUT, ref_at$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_index_at)(AtkTable *, gint, gint)
* }
*/
public class get_index_at {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_index_at.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_index_at.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_index_at$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_index_at"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_index_at)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout get_index_at$layout() {
return get_index_at$LAYOUT;
}
private static final long get_index_at$OFFSET = 24;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_index_at)(AtkTable *, gint, gint)
* }
*/
public static final long get_index_at$offset() {
return get_index_at$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_index_at)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment get_index_at(MemorySegment struct) {
return struct.get(get_index_at$LAYOUT, get_index_at$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_index_at)(AtkTable *, gint, gint)
* }
*/
public static void get_index_at(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_index_at$LAYOUT, get_index_at$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_column_at_index)(AtkTable *, gint)
* }
*/
public class get_column_at_index {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_column_at_index.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_column_at_index.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_column_at_index$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_column_at_index"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_column_at_index)(AtkTable *, gint)
* }
*/
public static final AddressLayout get_column_at_index$layout() {
return get_column_at_index$LAYOUT;
}
private static final long get_column_at_index$OFFSET = 32;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_column_at_index)(AtkTable *, gint)
* }
*/
public static final long get_column_at_index$offset() {
return get_column_at_index$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_column_at_index)(AtkTable *, gint)
* }
*/
public static MemorySegment get_column_at_index(MemorySegment struct) {
return struct.get(get_column_at_index$LAYOUT, get_column_at_index$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_column_at_index)(AtkTable *, gint)
* }
*/
public static void get_column_at_index(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_column_at_index$LAYOUT, get_column_at_index$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_row_at_index)(AtkTable *, gint)
* }
*/
public class get_row_at_index {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_row_at_index.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_row_at_index.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_row_at_index$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_row_at_index"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_row_at_index)(AtkTable *, gint)
* }
*/
public static final AddressLayout get_row_at_index$layout() {
return get_row_at_index$LAYOUT;
}
private static final long get_row_at_index$OFFSET = 40;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_row_at_index)(AtkTable *, gint)
* }
*/
public static final long get_row_at_index$offset() {
return get_row_at_index$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_row_at_index)(AtkTable *, gint)
* }
*/
public static MemorySegment get_row_at_index(MemorySegment struct) {
return struct.get(get_row_at_index$LAYOUT, get_row_at_index$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_row_at_index)(AtkTable *, gint)
* }
*/
public static void get_row_at_index(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_row_at_index$LAYOUT, get_row_at_index$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_n_columns)(AtkTable *)
* }
*/
public class get_n_columns {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_n_columns.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_n_columns.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_n_columns$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_n_columns"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_n_columns)(AtkTable *)
* }
*/
public static final AddressLayout get_n_columns$layout() {
return get_n_columns$LAYOUT;
}
private static final long get_n_columns$OFFSET = 48;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_n_columns)(AtkTable *)
* }
*/
public static final long get_n_columns$offset() {
return get_n_columns$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_n_columns)(AtkTable *)
* }
*/
public static MemorySegment get_n_columns(MemorySegment struct) {
return struct.get(get_n_columns$LAYOUT, get_n_columns$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_n_columns)(AtkTable *)
* }
*/
public static void get_n_columns(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_n_columns$LAYOUT, get_n_columns$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_n_rows)(AtkTable *)
* }
*/
public class get_n_rows {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_n_rows.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_n_rows.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_n_rows$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_n_rows"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_n_rows)(AtkTable *)
* }
*/
public static final AddressLayout get_n_rows$layout() {
return get_n_rows$LAYOUT;
}
private static final long get_n_rows$OFFSET = 56;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_n_rows)(AtkTable *)
* }
*/
public static final long get_n_rows$offset() {
return get_n_rows$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_n_rows)(AtkTable *)
* }
*/
public static MemorySegment get_n_rows(MemorySegment struct) {
return struct.get(get_n_rows$LAYOUT, get_n_rows$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_n_rows)(AtkTable *)
* }
*/
public static void get_n_rows(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_n_rows$LAYOUT, get_n_rows$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_column_extent_at)(AtkTable *, gint, gint)
* }
*/
public class get_column_extent_at {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_column_extent_at.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_column_extent_at.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_column_extent_at$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_column_extent_at"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_column_extent_at)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout get_column_extent_at$layout() {
return get_column_extent_at$LAYOUT;
}
private static final long get_column_extent_at$OFFSET = 64;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_column_extent_at)(AtkTable *, gint, gint)
* }
*/
public static final long get_column_extent_at$offset() {
return get_column_extent_at$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_column_extent_at)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment get_column_extent_at(MemorySegment struct) {
return struct.get(get_column_extent_at$LAYOUT, get_column_extent_at$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_column_extent_at)(AtkTable *, gint, gint)
* }
*/
public static void get_column_extent_at(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_column_extent_at$LAYOUT, get_column_extent_at$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_row_extent_at)(AtkTable *, gint, gint)
* }
*/
public class get_row_extent_at {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_row_extent_at.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_row_extent_at.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_row_extent_at$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_row_extent_at"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_row_extent_at)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout get_row_extent_at$layout() {
return get_row_extent_at$LAYOUT;
}
private static final long get_row_extent_at$OFFSET = 72;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_row_extent_at)(AtkTable *, gint, gint)
* }
*/
public static final long get_row_extent_at$offset() {
return get_row_extent_at$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_row_extent_at)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment get_row_extent_at(MemorySegment struct) {
return struct.get(get_row_extent_at$LAYOUT, get_row_extent_at$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_row_extent_at)(AtkTable *, gint, gint)
* }
*/
public static void get_row_extent_at(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_row_extent_at$LAYOUT, get_row_extent_at$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* AtkObject *(*get_caption)(AtkTable *)
* }
*/
public class get_caption {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_caption.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_caption.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_caption$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_caption"));
/**
* Layout for field:
* {@snippet lang=c :
* AtkObject *(*get_caption)(AtkTable *)
* }
*/
public static final AddressLayout get_caption$layout() {
return get_caption$LAYOUT;
}
private static final long get_caption$OFFSET = 80;
/**
* Offset for field:
* {@snippet lang=c :
* AtkObject *(*get_caption)(AtkTable *)
* }
*/
public static final long get_caption$offset() {
return get_caption$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* AtkObject *(*get_caption)(AtkTable *)
* }
*/
public static MemorySegment get_caption(MemorySegment struct) {
return struct.get(get_caption$LAYOUT, get_caption$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* AtkObject *(*get_caption)(AtkTable *)
* }
*/
public static void get_caption(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_caption$LAYOUT, get_caption$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* const gchar *(*get_column_description)(AtkTable *, gint)
* }
*/
public class get_column_description {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_column_description.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_column_description.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_column_description$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_column_description"));
/**
* Layout for field:
* {@snippet lang=c :
* const gchar *(*get_column_description)(AtkTable *, gint)
* }
*/
public static final AddressLayout get_column_description$layout() {
return get_column_description$LAYOUT;
}
private static final long get_column_description$OFFSET = 88;
/**
* Offset for field:
* {@snippet lang=c :
* const gchar *(*get_column_description)(AtkTable *, gint)
* }
*/
public static final long get_column_description$offset() {
return get_column_description$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* const gchar *(*get_column_description)(AtkTable *, gint)
* }
*/
public static MemorySegment get_column_description(MemorySegment struct) {
return struct.get(get_column_description$LAYOUT, get_column_description$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* const gchar *(*get_column_description)(AtkTable *, gint)
* }
*/
public static void get_column_description(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_column_description$LAYOUT, get_column_description$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* AtkObject *(*get_column_header)(AtkTable *, gint)
* }
*/
public class get_column_header {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_column_header.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_column_header.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_column_header$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_column_header"));
/**
* Layout for field:
* {@snippet lang=c :
* AtkObject *(*get_column_header)(AtkTable *, gint)
* }
*/
public static final AddressLayout get_column_header$layout() {
return get_column_header$LAYOUT;
}
private static final long get_column_header$OFFSET = 96;
/**
* Offset for field:
* {@snippet lang=c :
* AtkObject *(*get_column_header)(AtkTable *, gint)
* }
*/
public static final long get_column_header$offset() {
return get_column_header$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* AtkObject *(*get_column_header)(AtkTable *, gint)
* }
*/
public static MemorySegment get_column_header(MemorySegment struct) {
return struct.get(get_column_header$LAYOUT, get_column_header$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* AtkObject *(*get_column_header)(AtkTable *, gint)
* }
*/
public static void get_column_header(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_column_header$LAYOUT, get_column_header$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* const gchar *(*get_row_description)(AtkTable *, gint)
* }
*/
public class get_row_description {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_row_description.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_row_description.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_row_description$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_row_description"));
/**
* Layout for field:
* {@snippet lang=c :
* const gchar *(*get_row_description)(AtkTable *, gint)
* }
*/
public static final AddressLayout get_row_description$layout() {
return get_row_description$LAYOUT;
}
private static final long get_row_description$OFFSET = 104;
/**
* Offset for field:
* {@snippet lang=c :
* const gchar *(*get_row_description)(AtkTable *, gint)
* }
*/
public static final long get_row_description$offset() {
return get_row_description$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* const gchar *(*get_row_description)(AtkTable *, gint)
* }
*/
public static MemorySegment get_row_description(MemorySegment struct) {
return struct.get(get_row_description$LAYOUT, get_row_description$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* const gchar *(*get_row_description)(AtkTable *, gint)
* }
*/
public static void get_row_description(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_row_description$LAYOUT, get_row_description$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* AtkObject *(*get_row_header)(AtkTable *, gint)
* }
*/
public class get_row_header {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_row_header.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_row_header.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_row_header$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_row_header"));
/**
* Layout for field:
* {@snippet lang=c :
* AtkObject *(*get_row_header)(AtkTable *, gint)
* }
*/
public static final AddressLayout get_row_header$layout() {
return get_row_header$LAYOUT;
}
private static final long get_row_header$OFFSET = 112;
/**
* Offset for field:
* {@snippet lang=c :
* AtkObject *(*get_row_header)(AtkTable *, gint)
* }
*/
public static final long get_row_header$offset() {
return get_row_header$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* AtkObject *(*get_row_header)(AtkTable *, gint)
* }
*/
public static MemorySegment get_row_header(MemorySegment struct) {
return struct.get(get_row_header$LAYOUT, get_row_header$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* AtkObject *(*get_row_header)(AtkTable *, gint)
* }
*/
public static void get_row_header(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_row_header$LAYOUT, get_row_header$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* AtkObject *(*get_summary)(AtkTable *)
* }
*/
public class get_summary {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
MemorySegment apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_summary.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_summary.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static MemorySegment invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
return (MemorySegment) DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_summary$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_summary"));
/**
* Layout for field:
* {@snippet lang=c :
* AtkObject *(*get_summary)(AtkTable *)
* }
*/
public static final AddressLayout get_summary$layout() {
return get_summary$LAYOUT;
}
private static final long get_summary$OFFSET = 120;
/**
* Offset for field:
* {@snippet lang=c :
* AtkObject *(*get_summary)(AtkTable *)
* }
*/
public static final long get_summary$offset() {
return get_summary$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* AtkObject *(*get_summary)(AtkTable *)
* }
*/
public static MemorySegment get_summary(MemorySegment struct) {
return struct.get(get_summary$LAYOUT, get_summary$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* AtkObject *(*get_summary)(AtkTable *)
* }
*/
public static void get_summary(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_summary$LAYOUT, get_summary$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*set_caption)(AtkTable *, AtkObject *)
* }
*/
public class set_caption {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, MemorySegment _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(set_caption.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(set_caption.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, MemorySegment _x1) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout set_caption$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("set_caption"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*set_caption)(AtkTable *, AtkObject *)
* }
*/
public static final AddressLayout set_caption$layout() {
return set_caption$LAYOUT;
}
private static final long set_caption$OFFSET = 128;
/**
* Offset for field:
* {@snippet lang=c :
* void (*set_caption)(AtkTable *, AtkObject *)
* }
*/
public static final long set_caption$offset() {
return set_caption$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*set_caption)(AtkTable *, AtkObject *)
* }
*/
public static MemorySegment set_caption(MemorySegment struct) {
return struct.get(set_caption$LAYOUT, set_caption$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*set_caption)(AtkTable *, AtkObject *)
* }
*/
public static void set_caption(MemorySegment struct, MemorySegment fieldValue) {
struct.set(set_caption$LAYOUT, set_caption$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*set_column_description)(AtkTable *, gint, const gchar *)
* }
*/
public class set_column_description {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, MemorySegment _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(set_column_description.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(set_column_description.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, MemorySegment _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout set_column_description$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("set_column_description"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*set_column_description)(AtkTable *, gint, const gchar *)
* }
*/
public static final AddressLayout set_column_description$layout() {
return set_column_description$LAYOUT;
}
private static final long set_column_description$OFFSET = 136;
/**
* Offset for field:
* {@snippet lang=c :
* void (*set_column_description)(AtkTable *, gint, const gchar *)
* }
*/
public static final long set_column_description$offset() {
return set_column_description$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*set_column_description)(AtkTable *, gint, const gchar *)
* }
*/
public static MemorySegment set_column_description(MemorySegment struct) {
return struct.get(set_column_description$LAYOUT, set_column_description$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*set_column_description)(AtkTable *, gint, const gchar *)
* }
*/
public static void set_column_description(MemorySegment struct, MemorySegment fieldValue) {
struct.set(set_column_description$LAYOUT, set_column_description$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*set_column_header)(AtkTable *, gint, AtkObject *)
* }
*/
public class set_column_header {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, MemorySegment _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(set_column_header.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(set_column_header.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, MemorySegment _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout set_column_header$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("set_column_header"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*set_column_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static final AddressLayout set_column_header$layout() {
return set_column_header$LAYOUT;
}
private static final long set_column_header$OFFSET = 144;
/**
* Offset for field:
* {@snippet lang=c :
* void (*set_column_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static final long set_column_header$offset() {
return set_column_header$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*set_column_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static MemorySegment set_column_header(MemorySegment struct) {
return struct.get(set_column_header$LAYOUT, set_column_header$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*set_column_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static void set_column_header(MemorySegment struct, MemorySegment fieldValue) {
struct.set(set_column_header$LAYOUT, set_column_header$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*set_row_description)(AtkTable *, gint, const gchar *)
* }
*/
public class set_row_description {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, MemorySegment _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(set_row_description.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(set_row_description.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, MemorySegment _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout set_row_description$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("set_row_description"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*set_row_description)(AtkTable *, gint, const gchar *)
* }
*/
public static final AddressLayout set_row_description$layout() {
return set_row_description$LAYOUT;
}
private static final long set_row_description$OFFSET = 152;
/**
* Offset for field:
* {@snippet lang=c :
* void (*set_row_description)(AtkTable *, gint, const gchar *)
* }
*/
public static final long set_row_description$offset() {
return set_row_description$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*set_row_description)(AtkTable *, gint, const gchar *)
* }
*/
public static MemorySegment set_row_description(MemorySegment struct) {
return struct.get(set_row_description$LAYOUT, set_row_description$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*set_row_description)(AtkTable *, gint, const gchar *)
* }
*/
public static void set_row_description(MemorySegment struct, MemorySegment fieldValue) {
struct.set(set_row_description$LAYOUT, set_row_description$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*set_row_header)(AtkTable *, gint, AtkObject *)
* }
*/
public class set_row_header {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, MemorySegment _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(set_row_header.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(set_row_header.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, MemorySegment _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout set_row_header$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("set_row_header"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*set_row_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static final AddressLayout set_row_header$layout() {
return set_row_header$LAYOUT;
}
private static final long set_row_header$OFFSET = 160;
/**
* Offset for field:
* {@snippet lang=c :
* void (*set_row_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static final long set_row_header$offset() {
return set_row_header$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*set_row_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static MemorySegment set_row_header(MemorySegment struct) {
return struct.get(set_row_header$LAYOUT, set_row_header$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*set_row_header)(AtkTable *, gint, AtkObject *)
* }
*/
public static void set_row_header(MemorySegment struct, MemorySegment fieldValue) {
struct.set(set_row_header$LAYOUT, set_row_header$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*set_summary)(AtkTable *, AtkObject *)
* }
*/
public class set_summary {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, MemorySegment _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(set_summary.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(set_summary.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, MemorySegment _x1) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout set_summary$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("set_summary"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*set_summary)(AtkTable *, AtkObject *)
* }
*/
public static final AddressLayout set_summary$layout() {
return set_summary$LAYOUT;
}
private static final long set_summary$OFFSET = 168;
/**
* Offset for field:
* {@snippet lang=c :
* void (*set_summary)(AtkTable *, AtkObject *)
* }
*/
public static final long set_summary$offset() {
return set_summary$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*set_summary)(AtkTable *, AtkObject *)
* }
*/
public static MemorySegment set_summary(MemorySegment struct) {
return struct.get(set_summary$LAYOUT, set_summary$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*set_summary)(AtkTable *, AtkObject *)
* }
*/
public static void set_summary(MemorySegment struct, MemorySegment fieldValue) {
struct.set(set_summary$LAYOUT, set_summary$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_selected_columns)(AtkTable *, gint **)
* }
*/
public class get_selected_columns {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, MemorySegment _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_selected_columns.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_selected_columns.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, MemorySegment _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_selected_columns$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_selected_columns"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_selected_columns)(AtkTable *, gint **)
* }
*/
public static final AddressLayout get_selected_columns$layout() {
return get_selected_columns$LAYOUT;
}
private static final long get_selected_columns$OFFSET = 176;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_selected_columns)(AtkTable *, gint **)
* }
*/
public static final long get_selected_columns$offset() {
return get_selected_columns$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_selected_columns)(AtkTable *, gint **)
* }
*/
public static MemorySegment get_selected_columns(MemorySegment struct) {
return struct.get(get_selected_columns$LAYOUT, get_selected_columns$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_selected_columns)(AtkTable *, gint **)
* }
*/
public static void get_selected_columns(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_selected_columns$LAYOUT, get_selected_columns$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gint (*get_selected_rows)(AtkTable *, gint **)
* }
*/
public class get_selected_rows {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, MemorySegment _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(get_selected_rows.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(get_selected_rows.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, MemorySegment _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout get_selected_rows$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("get_selected_rows"));
/**
* Layout for field:
* {@snippet lang=c :
* gint (*get_selected_rows)(AtkTable *, gint **)
* }
*/
public static final AddressLayout get_selected_rows$layout() {
return get_selected_rows$LAYOUT;
}
private static final long get_selected_rows$OFFSET = 184;
/**
* Offset for field:
* {@snippet lang=c :
* gint (*get_selected_rows)(AtkTable *, gint **)
* }
*/
public static final long get_selected_rows$offset() {
return get_selected_rows$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gint (*get_selected_rows)(AtkTable *, gint **)
* }
*/
public static MemorySegment get_selected_rows(MemorySegment struct) {
return struct.get(get_selected_rows$LAYOUT, get_selected_rows$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gint (*get_selected_rows)(AtkTable *, gint **)
* }
*/
public static void get_selected_rows(MemorySegment struct, MemorySegment fieldValue) {
struct.set(get_selected_rows$LAYOUT, get_selected_rows$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*is_column_selected)(AtkTable *, gint)
* }
*/
public class is_column_selected {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(is_column_selected.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(is_column_selected.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout is_column_selected$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("is_column_selected"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*is_column_selected)(AtkTable *, gint)
* }
*/
public static final AddressLayout is_column_selected$layout() {
return is_column_selected$LAYOUT;
}
private static final long is_column_selected$OFFSET = 192;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*is_column_selected)(AtkTable *, gint)
* }
*/
public static final long is_column_selected$offset() {
return is_column_selected$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*is_column_selected)(AtkTable *, gint)
* }
*/
public static MemorySegment is_column_selected(MemorySegment struct) {
return struct.get(is_column_selected$LAYOUT, is_column_selected$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*is_column_selected)(AtkTable *, gint)
* }
*/
public static void is_column_selected(MemorySegment struct, MemorySegment fieldValue) {
struct.set(is_column_selected$LAYOUT, is_column_selected$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*is_row_selected)(AtkTable *, gint)
* }
*/
public class is_row_selected {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(is_row_selected.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(is_row_selected.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout is_row_selected$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("is_row_selected"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*is_row_selected)(AtkTable *, gint)
* }
*/
public static final AddressLayout is_row_selected$layout() {
return is_row_selected$LAYOUT;
}
private static final long is_row_selected$OFFSET = 200;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*is_row_selected)(AtkTable *, gint)
* }
*/
public static final long is_row_selected$offset() {
return is_row_selected$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*is_row_selected)(AtkTable *, gint)
* }
*/
public static MemorySegment is_row_selected(MemorySegment struct) {
return struct.get(is_row_selected$LAYOUT, is_row_selected$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*is_row_selected)(AtkTable *, gint)
* }
*/
public static void is_row_selected(MemorySegment struct, MemorySegment fieldValue) {
struct.set(is_row_selected$LAYOUT, is_row_selected$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*is_selected)(AtkTable *, gint, gint)
* }
*/
public class is_selected {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(is_selected.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(is_selected.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout is_selected$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("is_selected"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*is_selected)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout is_selected$layout() {
return is_selected$LAYOUT;
}
private static final long is_selected$OFFSET = 208;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*is_selected)(AtkTable *, gint, gint)
* }
*/
public static final long is_selected$offset() {
return is_selected$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*is_selected)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment is_selected(MemorySegment struct) {
return struct.get(is_selected$LAYOUT, is_selected$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*is_selected)(AtkTable *, gint, gint)
* }
*/
public static void is_selected(MemorySegment struct, MemorySegment fieldValue) {
struct.set(is_selected$LAYOUT, is_selected$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*add_row_selection)(AtkTable *, gint)
* }
*/
public class add_row_selection {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(add_row_selection.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(add_row_selection.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout add_row_selection$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("add_row_selection"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*add_row_selection)(AtkTable *, gint)
* }
*/
public static final AddressLayout add_row_selection$layout() {
return add_row_selection$LAYOUT;
}
private static final long add_row_selection$OFFSET = 216;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*add_row_selection)(AtkTable *, gint)
* }
*/
public static final long add_row_selection$offset() {
return add_row_selection$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*add_row_selection)(AtkTable *, gint)
* }
*/
public static MemorySegment add_row_selection(MemorySegment struct) {
return struct.get(add_row_selection$LAYOUT, add_row_selection$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*add_row_selection)(AtkTable *, gint)
* }
*/
public static void add_row_selection(MemorySegment struct, MemorySegment fieldValue) {
struct.set(add_row_selection$LAYOUT, add_row_selection$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*remove_row_selection)(AtkTable *, gint)
* }
*/
public class remove_row_selection {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(remove_row_selection.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(remove_row_selection.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout remove_row_selection$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("remove_row_selection"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*remove_row_selection)(AtkTable *, gint)
* }
*/
public static final AddressLayout remove_row_selection$layout() {
return remove_row_selection$LAYOUT;
}
private static final long remove_row_selection$OFFSET = 224;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*remove_row_selection)(AtkTable *, gint)
* }
*/
public static final long remove_row_selection$offset() {
return remove_row_selection$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*remove_row_selection)(AtkTable *, gint)
* }
*/
public static MemorySegment remove_row_selection(MemorySegment struct) {
return struct.get(remove_row_selection$LAYOUT, remove_row_selection$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*remove_row_selection)(AtkTable *, gint)
* }
*/
public static void remove_row_selection(MemorySegment struct, MemorySegment fieldValue) {
struct.set(remove_row_selection$LAYOUT, remove_row_selection$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*add_column_selection)(AtkTable *, gint)
* }
*/
public class add_column_selection {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(add_column_selection.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(add_column_selection.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout add_column_selection$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("add_column_selection"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*add_column_selection)(AtkTable *, gint)
* }
*/
public static final AddressLayout add_column_selection$layout() {
return add_column_selection$LAYOUT;
}
private static final long add_column_selection$OFFSET = 232;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*add_column_selection)(AtkTable *, gint)
* }
*/
public static final long add_column_selection$offset() {
return add_column_selection$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*add_column_selection)(AtkTable *, gint)
* }
*/
public static MemorySegment add_column_selection(MemorySegment struct) {
return struct.get(add_column_selection$LAYOUT, add_column_selection$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*add_column_selection)(AtkTable *, gint)
* }
*/
public static void add_column_selection(MemorySegment struct, MemorySegment fieldValue) {
struct.set(add_column_selection$LAYOUT, add_column_selection$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* gboolean (*remove_column_selection)(AtkTable *, gint)
* }
*/
public class remove_column_selection {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
int apply(MemorySegment _x0, int _x1);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.of(
app_indicator_h.C_INT,
app_indicator_h.C_POINTER,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(remove_column_selection.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(remove_column_selection.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static int invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1) {
try {
return (int) DOWN$MH.invokeExact(funcPtr, _x0, _x1);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout remove_column_selection$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("remove_column_selection"));
/**
* Layout for field:
* {@snippet lang=c :
* gboolean (*remove_column_selection)(AtkTable *, gint)
* }
*/
public static final AddressLayout remove_column_selection$layout() {
return remove_column_selection$LAYOUT;
}
private static final long remove_column_selection$OFFSET = 240;
/**
* Offset for field:
* {@snippet lang=c :
* gboolean (*remove_column_selection)(AtkTable *, gint)
* }
*/
public static final long remove_column_selection$offset() {
return remove_column_selection$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* gboolean (*remove_column_selection)(AtkTable *, gint)
* }
*/
public static MemorySegment remove_column_selection(MemorySegment struct) {
return struct.get(remove_column_selection$LAYOUT, remove_column_selection$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* gboolean (*remove_column_selection)(AtkTable *, gint)
* }
*/
public static void remove_column_selection(MemorySegment struct, MemorySegment fieldValue) {
struct.set(remove_column_selection$LAYOUT, remove_column_selection$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*row_inserted)(AtkTable *, gint, gint)
* }
*/
public class row_inserted {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(row_inserted.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(row_inserted.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout row_inserted$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("row_inserted"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*row_inserted)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout row_inserted$layout() {
return row_inserted$LAYOUT;
}
private static final long row_inserted$OFFSET = 248;
/**
* Offset for field:
* {@snippet lang=c :
* void (*row_inserted)(AtkTable *, gint, gint)
* }
*/
public static final long row_inserted$offset() {
return row_inserted$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*row_inserted)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment row_inserted(MemorySegment struct) {
return struct.get(row_inserted$LAYOUT, row_inserted$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*row_inserted)(AtkTable *, gint, gint)
* }
*/
public static void row_inserted(MemorySegment struct, MemorySegment fieldValue) {
struct.set(row_inserted$LAYOUT, row_inserted$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*column_inserted)(AtkTable *, gint, gint)
* }
*/
public class column_inserted {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(column_inserted.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(column_inserted.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout column_inserted$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("column_inserted"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*column_inserted)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout column_inserted$layout() {
return column_inserted$LAYOUT;
}
private static final long column_inserted$OFFSET = 256;
/**
* Offset for field:
* {@snippet lang=c :
* void (*column_inserted)(AtkTable *, gint, gint)
* }
*/
public static final long column_inserted$offset() {
return column_inserted$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*column_inserted)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment column_inserted(MemorySegment struct) {
return struct.get(column_inserted$LAYOUT, column_inserted$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*column_inserted)(AtkTable *, gint, gint)
* }
*/
public static void column_inserted(MemorySegment struct, MemorySegment fieldValue) {
struct.set(column_inserted$LAYOUT, column_inserted$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*row_deleted)(AtkTable *, gint, gint)
* }
*/
public class row_deleted {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(row_deleted.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(row_deleted.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout row_deleted$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("row_deleted"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*row_deleted)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout row_deleted$layout() {
return row_deleted$LAYOUT;
}
private static final long row_deleted$OFFSET = 264;
/**
* Offset for field:
* {@snippet lang=c :
* void (*row_deleted)(AtkTable *, gint, gint)
* }
*/
public static final long row_deleted$offset() {
return row_deleted$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*row_deleted)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment row_deleted(MemorySegment struct) {
return struct.get(row_deleted$LAYOUT, row_deleted$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*row_deleted)(AtkTable *, gint, gint)
* }
*/
public static void row_deleted(MemorySegment struct, MemorySegment fieldValue) {
struct.set(row_deleted$LAYOUT, row_deleted$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*column_deleted)(AtkTable *, gint, gint)
* }
*/
public class column_deleted {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0, int _x1, int _x2);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER,
app_indicator_h.C_INT,
app_indicator_h.C_INT
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(column_deleted.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(column_deleted.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0, int _x1, int _x2) {
try {
DOWN$MH.invokeExact(funcPtr, _x0, _x1, _x2);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout column_deleted$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("column_deleted"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*column_deleted)(AtkTable *, gint, gint)
* }
*/
public static final AddressLayout column_deleted$layout() {
return column_deleted$LAYOUT;
}
private static final long column_deleted$OFFSET = 272;
/**
* Offset for field:
* {@snippet lang=c :
* void (*column_deleted)(AtkTable *, gint, gint)
* }
*/
public static final long column_deleted$offset() {
return column_deleted$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*column_deleted)(AtkTable *, gint, gint)
* }
*/
public static MemorySegment column_deleted(MemorySegment struct) {
return struct.get(column_deleted$LAYOUT, column_deleted$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*column_deleted)(AtkTable *, gint, gint)
* }
*/
public static void column_deleted(MemorySegment struct, MemorySegment fieldValue) {
struct.set(column_deleted$LAYOUT, column_deleted$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*row_reordered)(AtkTable *)
* }
*/
public class row_reordered {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(row_reordered.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(row_reordered.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout row_reordered$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("row_reordered"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*row_reordered)(AtkTable *)
* }
*/
public static final AddressLayout row_reordered$layout() {
return row_reordered$LAYOUT;
}
private static final long row_reordered$OFFSET = 280;
/**
* Offset for field:
* {@snippet lang=c :
* void (*row_reordered)(AtkTable *)
* }
*/
public static final long row_reordered$offset() {
return row_reordered$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*row_reordered)(AtkTable *)
* }
*/
public static MemorySegment row_reordered(MemorySegment struct) {
return struct.get(row_reordered$LAYOUT, row_reordered$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*row_reordered)(AtkTable *)
* }
*/
public static void row_reordered(MemorySegment struct, MemorySegment fieldValue) {
struct.set(row_reordered$LAYOUT, row_reordered$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*column_reordered)(AtkTable *)
* }
*/
public class column_reordered {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(column_reordered.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(column_reordered.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout column_reordered$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("column_reordered"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*column_reordered)(AtkTable *)
* }
*/
public static final AddressLayout column_reordered$layout() {
return column_reordered$LAYOUT;
}
private static final long column_reordered$OFFSET = 288;
/**
* Offset for field:
* {@snippet lang=c :
* void (*column_reordered)(AtkTable *)
* }
*/
public static final long column_reordered$offset() {
return column_reordered$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*column_reordered)(AtkTable *)
* }
*/
public static MemorySegment column_reordered(MemorySegment struct) {
return struct.get(column_reordered$LAYOUT, column_reordered$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*column_reordered)(AtkTable *)
* }
*/
public static void column_reordered(MemorySegment struct, MemorySegment fieldValue) {
struct.set(column_reordered$LAYOUT, column_reordered$OFFSET, fieldValue);
}
/**
* {@snippet lang=c :
* void (*model_changed)(AtkTable *)
* }
*/
public class model_changed {
/**
* The function pointer signature, expressed as a functional interface
*/
public interface Function {
void apply(MemorySegment _x0);
}
private static final FunctionDescriptor $DESC = FunctionDescriptor.ofVoid(
app_indicator_h.C_POINTER
);
/**
* The descriptor of this function pointer
*/
public static FunctionDescriptor descriptor() {
return $DESC;
}
private static final MethodHandle UP$MH = app_indicator_h.upcallHandle(model_changed.Function.class, "apply", $DESC);
/**
* Allocates a new upcall stub, whose implementation is defined by {@code fi}.
* The lifetime of the returned segment is managed by {@code arena}
*/
public static MemorySegment allocate(model_changed.Function fi, Arena arena) {
return Linker.nativeLinker().upcallStub(UP$MH.bindTo(fi), $DESC, arena);
}
private static final MethodHandle DOWN$MH = Linker.nativeLinker().downcallHandle($DESC);
/**
* Invoke the upcall stub {@code funcPtr}, with given parameters
*/
public static void invoke(MemorySegment funcPtr,MemorySegment _x0) {
try {
DOWN$MH.invokeExact(funcPtr, _x0);
} catch (Throwable ex$) {
throw new AssertionError("should not reach here", ex$);
}
}
}
private static final AddressLayout model_changed$LAYOUT = (AddressLayout)$LAYOUT.select(groupElement("model_changed"));
/**
* Layout for field:
* {@snippet lang=c :
* void (*model_changed)(AtkTable *)
* }
*/
public static final AddressLayout model_changed$layout() {
return model_changed$LAYOUT;
}
private static final long model_changed$OFFSET = 296;
/**
* Offset for field:
* {@snippet lang=c :
* void (*model_changed)(AtkTable *)
* }
*/
public static final long model_changed$offset() {
return model_changed$OFFSET;
}
/**
* Getter for field:
* {@snippet lang=c :
* void (*model_changed)(AtkTable *)
* }
*/
public static MemorySegment model_changed(MemorySegment struct) {
return struct.get(model_changed$LAYOUT, model_changed$OFFSET);
}
/**
* Setter for field:
* {@snippet lang=c :
* void (*model_changed)(AtkTable *)
* }
*/
public static void model_changed(MemorySegment struct, MemorySegment fieldValue) {
struct.set(model_changed$LAYOUT, model_changed$OFFSET, fieldValue);
}
/**
* Obtains a slice of {@code arrayParam} which selects the array element at {@code index}.
* The returned segment has address {@code arrayParam.address() + index * layout().byteSize()}
*/
public static MemorySegment asSlice(MemorySegment array, long index) {
return array.asSlice(layout().byteSize() * index);
}
/**
* The size (in bytes) of this struct
*/
public static long sizeof() { return layout().byteSize(); }
/**
* Allocate a segment of size {@code layout().byteSize()} using {@code allocator}
*/
public static MemorySegment allocate(SegmentAllocator allocator) {
return allocator.allocate(layout());
}
/**
* Allocate an array of size {@code elementCount} using {@code allocator}.
* The returned segment has size {@code elementCount * layout().byteSize()}.
*/
public static MemorySegment allocateArray(long elementCount, SegmentAllocator allocator) {
return allocator.allocate(MemoryLayout.sequenceLayout(elementCount, layout()));
}
/**
* Reinterprets {@code addr} using target {@code arena} and {@code cleanupAction) (if any).
* The returned segment has size {@code layout().byteSize()}
*/
public static MemorySegment reinterpret(MemorySegment addr, Arena arena, Consumer cleanup) {
return reinterpret(addr, 1, arena, cleanup);
}
/**
* Reinterprets {@code addr} using target {@code arena} and {@code cleanupAction) (if any).
* The returned segment has size {@code elementCount * layout().byteSize()}
*/
public static MemorySegment reinterpret(MemorySegment addr, long elementCount, Arena arena, Consumer cleanup) {
return addr.reinterpret(layout().byteSize() * elementCount, arena, cleanup);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy