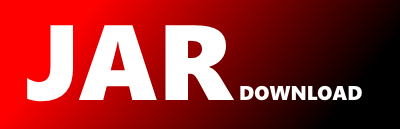
org.python.parser.PythonGrammarTreeConstants Maven / Gradle / Ivy
Go to download
Jython is an implementation of the high-level, dynamic, object-oriented
language Python written in 100% Pure Java, and seamlessly integrated with
the Java platform. It thus allows you to run Python on any Java platform.
/* Generated By:JJTree: Do not edit this line. /Users/fwier/src/jython/org/python/parser/PythonGrammarTreeConstants.java */
package org.python.parser;
public interface PythonGrammarTreeConstants
{
public int JJTSINGLE_INPUT = 0;
public int JJTFILE_INPUT = 1;
public int JJTEVAL_INPUT = 2;
public int JJTFUNCDEF = 3;
public int JJTVOID = 4;
public int JJTEXTRAARGLIST = 5;
public int JJTEXTRAKEYWORDLIST = 6;
public int JJTDEFAULTARG = 7;
public int JJTTUPLE = 8;
public int JJTAUG_PLUS = 9;
public int JJTAUG_MINUS = 10;
public int JJTAUG_MULTIPLY = 11;
public int JJTAUG_DIVIDE = 12;
public int JJTAUG_FLOORDIVIDE = 13;
public int JJTAUG_MODULO = 14;
public int JJTAUG_AND = 15;
public int JJTAUG_OR = 16;
public int JJTAUG_XOR = 17;
public int JJTAUG_LSHIFT = 18;
public int JJTAUG_RSHIFT = 19;
public int JJTAUG_POWER = 20;
public int JJTEXPR_STMT = 21;
public int JJTPRINTEXT_STMT = 22;
public int JJTPRINT_STMT = 23;
public int JJTDEL_STMT = 24;
public int JJTPASS_STMT = 25;
public int JJTBREAK_STMT = 26;
public int JJTCONTINUE_STMT = 27;
public int JJTRETURN_STMT = 28;
public int JJTYIELD_STMT = 29;
public int JJTRAISE_STMT = 30;
public int JJTIMPORT = 31;
public int JJTIMPORTFROM = 32;
public int JJTDOTTED_AS_NAME = 33;
public int JJTDOTTED_NAME = 34;
public int JJTIMPORT_AS_NAME = 35;
public int JJTGLOBAL_STMT = 36;
public int JJTEXEC_STMT = 37;
public int JJTASSERT_STMT = 38;
public int JJTIF_STMT = 39;
public int JJTWHILE_STMT = 40;
public int JJTFOR_STMT = 41;
public int JJTTRY_STMT = 42;
public int JJTTRYFINALLY_STMT = 43;
public int JJTEXCEPT_CLAUSE = 44;
public int JJTSUITE = 45;
public int JJTOR_BOOLEAN = 46;
public int JJTAND_BOOLEAN = 47;
public int JJTNOT_1OP = 48;
public int JJTCOMPARISION = 49;
public int JJTLESS_CMP = 50;
public int JJTGREATER_CMP = 51;
public int JJTEQUAL_CMP = 52;
public int JJTGREATER_EQUAL_CMP = 53;
public int JJTLESS_EQUAL_CMP = 54;
public int JJTNOTEQUAL_CMP = 55;
public int JJTIN_CMP = 56;
public int JJTNOT_IN_CMP = 57;
public int JJTIS_NOT_CMP = 58;
public int JJTIS_CMP = 59;
public int JJTOR_2OP = 60;
public int JJTXOR_2OP = 61;
public int JJTAND_2OP = 62;
public int JJTLSHIFT_2OP = 63;
public int JJTRSHIFT_2OP = 64;
public int JJTADD_2OP = 65;
public int JJTSUB_2OP = 66;
public int JJTMUL_2OP = 67;
public int JJTDIV_2OP = 68;
public int JJTFLOORDIV_2OP = 69;
public int JJTMOD_2OP = 70;
public int JJTPOS_1OP = 71;
public int JJTNEG_1OP = 72;
public int JJTINVERT_1OP = 73;
public int JJTPOW_2OP = 74;
public int JJTCALL_OP = 75;
public int JJTINDEX_OP = 76;
public int JJTDOT_OP = 77;
public int JJTLIST = 78;
public int JJTDICTIONARY = 79;
public int JJTSTR_1OP = 80;
public int JJTSTRJOIN = 81;
public int JJTLAMBDEF = 82;
public int JJTSUBSCRIPTLIST = 83;
public int JJTELLIPSES = 84;
public int JJTSLICE = 85;
public int JJTCOLON = 86;
public int JJTCOMMA = 87;
public int JJTLIST_FOR = 88;
public int JJTCLASSDEF = 89;
public int JJTEXTRAARGVALUELIST = 90;
public int JJTEXTRAKEYWORDVALUELIST = 91;
public int JJTKEYWORD = 92;
public int JJTNUM = 93;
public int JJTCOMPLEX = 94;
public int JJTNAME = 95;
public int JJTSTRING = 96;
public int JJTUNICODE = 97;
public String[] jjtNodeName = {
"single_input",
"file_input",
"eval_input",
"funcdef",
"void",
"ExtraArgList",
"ExtraKeywordList",
"defaultarg",
"tuple",
"aug_plus",
"aug_minus",
"aug_multiply",
"aug_divide",
"aug_floordivide",
"aug_modulo",
"aug_and",
"aug_or",
"aug_xor",
"aug_lshift",
"aug_rshift",
"aug_power",
"expr_stmt",
"printext_stmt",
"print_stmt",
"del_stmt",
"pass_stmt",
"break_stmt",
"continue_stmt",
"return_stmt",
"yield_stmt",
"raise_stmt",
"Import",
"ImportFrom",
"dotted_as_name",
"dotted_name",
"import_as_name",
"global_stmt",
"exec_stmt",
"assert_stmt",
"if_stmt",
"while_stmt",
"for_stmt",
"try_stmt",
"tryfinally_stmt",
"except_clause",
"suite",
"or_boolean",
"and_boolean",
"not_1op",
"comparision",
"less_cmp",
"greater_cmp",
"equal_cmp",
"greater_equal_cmp",
"less_equal_cmp",
"notequal_cmp",
"in_cmp",
"not_in_cmp",
"is_not_cmp",
"is_cmp",
"or_2op",
"xor_2op",
"and_2op",
"lshift_2op",
"rshift_2op",
"add_2op",
"sub_2op",
"mul_2op",
"div_2op",
"floordiv_2op",
"mod_2op",
"pos_1op",
"neg_1op",
"invert_1op",
"pow_2op",
"Call_Op",
"Index_Op",
"Dot_Op",
"list",
"dictionary",
"str_1op",
"strjoin",
"lambdef",
"subscriptlist",
"Ellipses",
"Slice",
"Colon",
"Comma",
"list_for",
"classdef",
"ExtraArgValueList",
"ExtraKeywordValueList",
"Keyword",
"Num",
"Complex",
"Name",
"String",
"Unicode",
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy