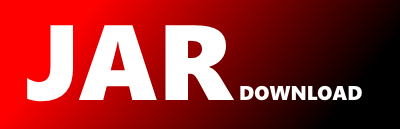
jdk.internal.util.xml.PropertiesDefaultHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qbicc-rt-java.base Show documentation
Show all versions of qbicc-rt-java.base Show documentation
The Qbicc builder for the java.base JDK module
/*
* Copyright (c) 2012, 2020, Oracle and/or its affiliates. All rights reserved.
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS FILE HEADER.
*
* This code is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License version 2 only, as
* published by the Free Software Foundation. Oracle designates this
* particular file as subject to the "Classpath" exception as provided
* by Oracle in the LICENSE file that accompanied this code.
*
* This code is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
* version 2 for more details (a copy is included in the LICENSE file that
* accompanied this code).
*
* You should have received a copy of the GNU General Public License version
* 2 along with this work; if not, write to the Free Software Foundation,
* Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* Please contact Oracle, 500 Oracle Parkway, Redwood Shores, CA 94065 USA
* or visit www.oracle.com if you need additional information or have any
* questions.
*/
package jdk.internal.util.xml;
import java.io.*;
import java.nio.charset.Charset;
import java.util.InvalidPropertiesFormatException;
import java.util.Map.Entry;
import java.util.Properties;
import jdk.internal.org.xml.sax.Attributes;
import jdk.internal.org.xml.sax.InputSource;
import jdk.internal.org.xml.sax.SAXException;
import jdk.internal.org.xml.sax.SAXParseException;
import jdk.internal.org.xml.sax.helpers.DefaultHandler;
import jdk.internal.util.xml.impl.SAXParserImpl;
import jdk.internal.util.xml.impl.XMLStreamWriterImpl;
/**
* A class used to aid in Properties load and save in XML. This class is
* re-implemented using a subset of SAX
*
* @author Joe Wang
* @since 1.8
*/
public class PropertiesDefaultHandler extends DefaultHandler {
// Elements specified in the properties.dtd
private static final String ELEMENT_ROOT = "properties";
private static final String ELEMENT_COMMENT = "comment";
private static final String ELEMENT_ENTRY = "entry";
private static final String ATTR_KEY = "key";
// The required DTD URI for exported properties
private static final String PROPS_DTD_DECL =
"";
private static final String PROPS_DTD_URI =
"http://java.sun.com/dtd/properties.dtd";
private static final String PROPS_DTD =
""
+ ""
+ ""
+ ""
+ ""
+ ""
+ "";
/**
* Version number for the format of exported properties files.
*/
private static final String EXTERNAL_XML_VERSION = "1.0";
private Properties properties;
public void load(Properties props, InputStream in)
throws IOException, InvalidPropertiesFormatException, UnsupportedEncodingException
{
this.properties = props;
try {
SAXParser parser = new SAXParserImpl();
parser.parse(in, this);
} catch (SAXException saxe) {
throw new InvalidPropertiesFormatException(saxe);
}
/**
* String xmlVersion = propertiesElement.getAttribute("version"); if
* (xmlVersion.compareTo(EXTERNAL_XML_VERSION) > 0) throw new
* InvalidPropertiesFormatException( "Exported Properties file format
* version " + xmlVersion + " is not supported. This java installation
* can read" + " versions " + EXTERNAL_XML_VERSION + " or older. You" +
* " may need to install a newer version of JDK.");
*/
}
public void store(Properties props, OutputStream os, String comment, Charset charset)
throws IOException
{
try {
XMLStreamWriter writer = new XMLStreamWriterImpl(os, charset);
writer.writeStartDocument();
writer.writeDTD(PROPS_DTD_DECL);
writer.writeStartElement(ELEMENT_ROOT);
if (comment != null && !comment.isEmpty()) {
writer.writeStartElement(ELEMENT_COMMENT);
writer.writeCharacters(comment);
writer.writeEndElement();
}
synchronized(props) {
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy