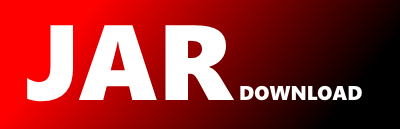
org.qbicc.graph.literal.CompoundLiteral Maven / Gradle / Ivy
package org.qbicc.graph.literal;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import org.qbicc.graph.Value;
import org.qbicc.type.CompoundType;
/**
*
*/
public final class CompoundLiteral extends Literal {
private final CompoundType type;
private final Map values;
private final List valuesAsList;
private final int hashCode;
CompoundLiteral(final CompoundType type, final Map values) {
this.type = type;
this.values = values;
hashCode = Objects.hash(type, values);
valuesAsList = new ArrayList<>(values.size());
for (CompoundType.Member member : type.getMembers()) {
Literal literal = values.get(member);
if (literal != null) {
valuesAsList.add(literal);
}
}
}
@Override
public int getValueDependencyCount() {
return valuesAsList.size();
}
@Override
public Value getValueDependency(int index) throws IndexOutOfBoundsException {
return valuesAsList.get(index);
}
public CompoundType getType() {
return type;
}
public Map getValues() {
return values;
}
public R accept(final LiteralVisitor visitor, final T param) {
return visitor.visit(param, this);
}
public Value extractMember(LiteralFactory lf, CompoundType.Member member) {
return values.get(member);
}
public boolean isZero() {
return false;
}
public boolean equals(final Literal other) {
return other instanceof CompoundLiteral && equals((CompoundLiteral) other);
}
public boolean equals(final CompoundLiteral other) {
return this == other || other != null && hashCode == other.hashCode && type.equals(other.type) && values.equals(other.values);
}
public int hashCode() {
return hashCode;
}
public StringBuilder toString(StringBuilder builder) {
builder.append('{');
Iterator> iterator = values.entrySet().iterator();
if (iterator.hasNext()) {
Map.Entry entry = iterator.next();
builder.append(entry.getKey().getName());
builder.append('=');
builder.append(entry.getValue());
while (iterator.hasNext()) {
builder.append(',');
entry = iterator.next();
builder.append(entry.getKey().getName());
builder.append('=');
builder.append(entry.getValue());
}
}
builder.append('}');
return builder;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy