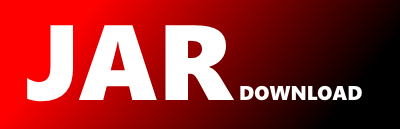
org.qbicc.plugin.vio.VIO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qbicc-plugin-vio Show documentation
Show all versions of qbicc-plugin-vio Show documentation
Support for virtual I/O on the host
package org.qbicc.plugin.vio;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.ByteBuffer;
import java.util.List;
import org.qbicc.context.AttachmentKey;
import org.qbicc.context.ClassContext;
import org.qbicc.context.CompilationContext;
import org.qbicc.interpreter.Thrown;
import org.qbicc.interpreter.Vm;
import org.qbicc.interpreter.VmArray;
import org.qbicc.interpreter.VmObject;
import org.qbicc.interpreter.VmThread;
import org.qbicc.interpreter.VmThrowableClass;
import org.qbicc.machine.vio.VIOSystem;
import org.qbicc.type.definition.LoadedTypeDefinition;
/**
* Virtual I/O.
*/
public final class VIO {
private static final AttachmentKey KEY = new AttachmentKey<>();
private final CompilationContext ctxt;
private final VIOSystem system;
private final VmThrowableClass ioException;
private VIO(CompilationContext ctxt) {
this.ctxt = ctxt;
// todo: configurable max fd
system = new VIOSystem(256);
ioException = (VmThrowableClass) ctxt.getBootstrapClassContext().findDefinedType("java/io/IOException").load().getVmClass();
try {
// todo: configurable build-time process input source
system.openInputStream(0, InputStream::nullInputStream);
// todo: capture output and error streams
system.openOutputStream(1, OutputStream::nullOutputStream);
system.openOutputStream(2, OutputStream::nullOutputStream);
} catch (IOException e) {
// should be impossible
throw new RuntimeException(e);
}
}
public static VIO get(CompilationContext ctxt) {
VIO instance = ctxt.getAttachment(KEY);
if (instance == null) {
instance = new VIO(ctxt);
VIO appearing = ctxt.putAttachmentIfAbsent(KEY, instance);
if (appearing != null) {
instance = appearing;
} else {
instance.registerInvokables();
}
}
return instance;
}
public VIOSystem getVIOSystem() {
return system;
}
private void registerInvokables() {
Vm vm = ctxt.getVm();
ClassContext classContext = ctxt.getBootstrapClassContext();
// HostIO (VIO aspects; VFS methods can be found in org.qbicc.plugin.vfs.VFS)
LoadedTypeDefinition hostIoDef = classContext.findDefinedType("org/qbicc/runtime/host/HostIO").load();
vm.registerInvokable(hostIoDef.requireSingleMethod("close"), this::doHostClose);
vm.registerInvokable(hostIoDef.requireSingleMethod("pipe"), this::doHostPipe);
vm.registerInvokable(hostIoDef.requireSingleMethod("socketpair"), this::doHostSocketPair);
vm.registerInvokable(hostIoDef.requireSingleMethod("dup"), this::doHostDup);
vm.registerInvokable(hostIoDef.requireSingleMethod("dup2"), this::doHostDup2);
vm.registerInvokable(hostIoDef.requireSingleMethod("read"), this::doHostRead);
vm.registerInvokable(hostIoDef.requireSingleMethod("pread"), this::doHostPRead);
vm.registerInvokable(hostIoDef.requireSingleMethod("readSingle"), this::doHostReadSingle);
vm.registerInvokable(hostIoDef.requireSingleMethod("available"), this::doHostAvailable);
vm.registerInvokable(hostIoDef.requireSingleMethod("write"), this::doHostWrite);
vm.registerInvokable(hostIoDef.requireSingleMethod("writeSingle"), this::doHostWriteSingle);
vm.registerInvokable(hostIoDef.requireSingleMethod("getFileSize"), this::doHostGetFileSize);
vm.registerInvokable(hostIoDef.requireSingleMethod("seekAbsolute"), this::doHostSeekAbsolute);
vm.registerInvokable(hostIoDef.requireSingleMethod("seekRelative"), this::doHostSeekRelative);
}
private Object doHostClose(final VmThread vmThread, final VmObject ignored, final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy