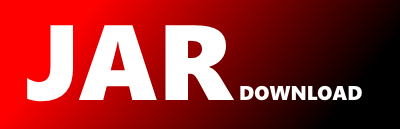
org.qbicc.runtime.stackwalk.MethodData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qbicc-runtime-main Show documentation
Show all versions of qbicc-runtime-main Show documentation
Qbicc run time main entry point
package org.qbicc.runtime.stackwalk;
import org.qbicc.runtime.AutoQueued;
import org.qbicc.runtime.CNative;
import org.qbicc.runtime.main.CompilerIntrinsics;
import static org.qbicc.runtime.CNative.*;
public final class MethodData {
public static native String getFileName(int minfoIndex);
public static native String getMethodName(int minfoIndex);
public static native String getMethodDesc(int minfoIndex);
public static native type_id getTypeId(int minfoIndex);
public static native int getModifiers(int minfoIndex);
public static String getClassName(int minfoIndex) {
Class> clazz = getClass(minfoIndex);
return clazz == null ? "" : clazz.getName();
}
public static Class> getClass(int minfoIndex) {
type_id typeId = getTypeId(minfoIndex);
Class> clazz = typeId.isZero() ? null : CompilerIntrinsics.getClassFromTypeId(typeId, word(0));
return clazz;
}
public static boolean hasAllModifiersOf(int minfoIndex, int mask) {
int modifiers = getModifiers(minfoIndex);
return (modifiers & mask) == mask;
}
public static boolean hasNoModifiersOf(int minfoIndex, int mask) {
int modifiers = getModifiers(minfoIndex);
return (modifiers & mask) == 0;
}
public static native int getMethodInfoIndex(int scIndex);
public static native int getLineNumber(int scIndex);
public static native int getBytecodeIndex(int scIndex);
public static native int getInlinedAtIndex(int scIndex);
public static native int getSourceCodeInfoIndex(int index);
public static native long getInstructionAddress(int index);
public static native int getInstructionListSize();
static int findInstructionIndex(long ip) {
// do a binary search in instruction table
int upper = MethodData.getInstructionListSize();
int lower = 0;
while (upper >= lower) {
int mid = ( upper + lower ) >>> 1;
long addr = MethodData.getInstructionAddress(mid);
if (ip == addr) {
return mid;
} else if (ip > addr) {
lower = mid+1;
} else {
upper = mid-1;
}
}
return -1;
}
// Debug method to validate linker order was as expected.
static void validateMethodData() {
int upper = MethodData.getInstructionListSize();
long prev = 0;
printString("Starting validation");
printString(Integer.toString(upper));
for (int i=0; i clazz = getClass(mi);
Module module = clazz.getModule();
String modName = module == null ? null : module.getName();
String modVer = /* todo: access directly via module */ null;
ClassLoader classLoader = clazz.getClassLoader();
String classLoaderName = classLoader == null ? null : classLoader.getName();
steArray[i] = new StackTraceElement(classLoaderName, modName, modVer, clazz.getName(), getMethodName(mi), getFileName(mi), getLineNumber(sc));
((StackTraceElementAccess)(Object)steArray[i]).declaringClassObject = clazz;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy