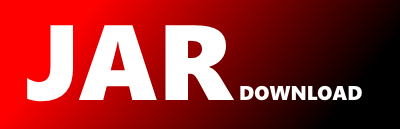
querqy.rewrite.commonrules.PropertiesBuilder Maven / Gradle / Ivy
package querqy.rewrite.commonrules;
import static querqy.rewrite.commonrules.PropertiesBuilder.JsonObjState.IN_OBJECT;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.jayway.jsonpath.Configuration;
import com.jayway.jsonpath.Option;
import com.jayway.jsonpath.spi.json.JacksonJsonProvider;
import com.jayway.jsonpath.spi.mapper.JacksonMappingProvider;
import querqy.rewrite.commonrules.RuleParseException;
import querqy.rewrite.commonrules.ValidationError;
import querqy.rewrite.commonrules.model.InstructionsProperties;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
public class PropertiesBuilder {
enum JsonObjState {BEFORE, IN_OBJECT, AFTER_OBJECT}
private JsonObjState jsonObjState = JsonObjState.BEFORE;
private java.util.Map primitiveProperties;
private StringBuilder jsonObjectString;
private final ObjectMapper objectMapper;
private final Configuration jsonPathConfiguration;
public PropertiesBuilder() {
objectMapper = new ObjectMapper();
objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
objectMapper.configure(JsonParser.Feature.ALLOW_YAML_COMMENTS, true);
objectMapper.configure(JsonParser.Feature.ALLOW_SINGLE_QUOTES, true);
objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_CONTROL_CHARS, true);
objectMapper.configure(JsonParser.Feature.ALLOW_NUMERIC_LEADING_ZEROS, true);
objectMapper.configure(JsonParser.Feature.STRICT_DUPLICATE_DETECTION, true);
jsonPathConfiguration = Configuration.builder()
.jsonProvider(new JacksonJsonProvider())
.mappingProvider(new JacksonMappingProvider()).build();
jsonPathConfiguration.addOptions(Option.ALWAYS_RETURN_LIST);
primitiveProperties = new HashMap<>();
jsonObjectString = new StringBuilder();
}
/**
* Consumes the next line and returns an optional validation error.
*
* @param line The next line from the input
* @return An optional validation error.
* @throws RuleParseException if a JSON property cannot be parsed.
*/
public Optional nextLine(final String line) throws RuleParseException {
String str = line.trim();
if (str.length() > 0) {
if (jsonObjState != IN_OBJECT) {
// left trim only (as this might be the start of an object)
str = ltrim(line);
if (!str.startsWith("@")) {
return Optional.of(new ValidationError("@ expected at beginning of line: " + line));
}
str = ltrim(str.substring(1));
if (str.length() == 0) {
return Optional.of(new ValidationError("property expected after @"));
}
if (str.charAt(0) == '{') {
// start of object
if (jsonObjState == JsonObjState.AFTER_OBJECT) {
return Optional.of(new ValidationError("Only one property object can be defined"));
}
final String rStr = rtrim(str);
if (rStr.endsWith("}@") && !rStr.endsWith("\\}@")) {
// the entire JSON object is on a single line
jsonObjectString.append(rStr.substring(0, rStr.length() - 1));
mergeObjectWithPrimitiveProperties();
} else {
jsonObjState = IN_OBJECT;
jsonObjectString.append(str);
}
} else {
// primitive property
try {
final Map map = stringToProperty(str);
for (final Map.Entry entry : map.entrySet()) {
addProperty(entry.getKey(), entry.getValue(), primitiveProperties);
}
} catch (final IOException | RuleParseException e) {
return Optional.of(new ValidationError("Line could not be read as a property: " +
e.getMessage() + ", " + line));
}
}
} else {
str = rtrim(line);
if (str.endsWith("\\}@") || !str.endsWith("}@")) {
jsonObjectString.append('\n').append(line);
} else {
jsonObjectString.append('\n').append(str.substring(0, str.length() - 1));
mergeObjectWithPrimitiveProperties();
}
}
}
return Optional.empty();
}
public void reset() {
jsonObjState = JsonObjState.BEFORE;
primitiveProperties = new HashMap<>();
jsonObjectString = new StringBuilder();
}
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy