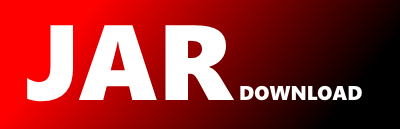
querqy.rewrite.commonrules.model.Input Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of querqy-core Show documentation
Show all versions of querqy-core Show documentation
Querqy library for query rewriting: Querqy Core
/**
*
*/
package querqy.rewrite.commonrules.model;
import java.util.LinkedList;
import java.util.List;
import querqy.ComparableCharSequence;
import querqy.CompoundCharSequence;
/**
* An input object representing the left-hand side of a rewriting rule
*
* @author rene
*
*/
public class Input {
final List inputTerms;
final boolean requiresLeftBoundary;
final boolean requiresRightBoundary;
private final String matchExpression;
/**
* Same as {@link #Input(List, boolean, boolean, String)} with both boundaries not required (set to false)
* @param inputTerms The sequence of terms to match
* @param matchExpression A string that represents the input match condition (i.e. the left-hand side in rules.txt)
*/
public Input(final List inputTerms, final String matchExpression) {
this(inputTerms, false, false, matchExpression);
}
/**
*
* @param inputTerms The sequence of terms to match
* @param requiresLeftBoundary true iff the first input term must be the first term in the query
* @param requiresRightBoundary true iff the last input term must be the last term in the query
* @param matchExpression A string that represents the input match condition (i.e. the left hand-side in rules.txt)
*/
public Input(final List inputTerms, final boolean requiresLeftBoundary, final boolean requiresRightBoundary,
final String matchExpression) {
if (matchExpression == null) {
throw new IllegalArgumentException("matchExpression must not be null");
}
this.inputTerms = inputTerms;
this.requiresLeftBoundary = requiresLeftBoundary;
this.requiresRightBoundary = requiresRightBoundary;
this.matchExpression = matchExpression;
}
public boolean isEmpty() {
return inputTerms == null || inputTerms.isEmpty();
}
public List getInputSequences(boolean lowerCaseValues) {
if (inputTerms.size() == 1) {
return inputTerms.get(0).getCharSequences(lowerCaseValues);
}
LinkedList> slots = new LinkedList<>();
for (Term inputTerm : inputTerms) {
slots.add(inputTerm.getCharSequences(lowerCaseValues));
}
List seqs = new LinkedList<>();
collectTails(new LinkedList<>(), slots, seqs);
return seqs;
}
void collectTails(List prefix, List> tailSlots,
List result) {
if (tailSlots.size() == 1) {
for (ComparableCharSequence sequence : tailSlots.get(0)) {
List combined = new LinkedList<>(prefix);
combined.add(sequence);
result.add(new CompoundCharSequence(" ", combined));
}
} else {
List> newTail = tailSlots.subList(1, tailSlots.size());
for (ComparableCharSequence sequence : tailSlots.get(0)) {
List newPrefix = new LinkedList<>(prefix);
newPrefix.add(sequence);
collectTails(newPrefix, newTail, result);
}
}
}
public List getInputTerms() {
return inputTerms;
}
@Override
public String toString() {
return "Input [inputTerms=" + inputTerms + "]";
}
public boolean requiresLeftBoundary() {
return requiresLeftBoundary;
}
public boolean requiresRightBoundary() {
return requiresRightBoundary;
}
public String getMatchExpression() {
return matchExpression;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy