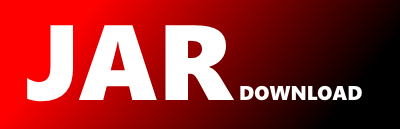
io.questdb.griffin.engine.functions.CursorFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of questdb Show documentation
Show all versions of questdb Show documentation
QuestDB is high performance SQL time series database
/*******************************************************************************
* ___ _ ____ ____
* / _ \ _ _ ___ ___| |_| _ \| __ )
* | | | | | | |/ _ \/ __| __| | | | _ \
* | |_| | |_| | __/\__ \ |_| |_| | |_) |
* \__\_\\__,_|\___||___/\__|____/|____/
*
* Copyright (c) 2014-2019 Appsicle
* Copyright (c) 2019-2024 QuestDB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
******************************************************************************/
package io.questdb.griffin.engine.functions;
import io.questdb.cairo.ColumnType;
import io.questdb.cairo.sql.Record;
import io.questdb.cairo.sql.RecordCursorFactory;
import io.questdb.cairo.sql.ScalarFunction;
import io.questdb.griffin.PlanSink;
import io.questdb.std.BinarySequence;
import io.questdb.std.Long256;
import io.questdb.std.str.CharSink;
import io.questdb.std.str.Utf8Sequence;
public class CursorFunction implements ScalarFunction {
private final RecordCursorFactory factory;
public CursorFunction(RecordCursorFactory factory) {
this.factory = factory;
}
@Override
public void close() {
factory.close();
}
@Override
public final BinarySequence getBin(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public long getBinLen(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final boolean getBool(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final byte getByte(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public char getChar(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final long getDate(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final double getDouble(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final float getFloat(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public byte getGeoByte(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public int getGeoInt(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public long getGeoLong(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public short getGeoShort(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final int getIPv4(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final int getInt(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final long getLong(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public long getLong128Hi(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public long getLong128Lo(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public void getLong256(Record rec, CharSink> sink) {
throw new UnsupportedOperationException();
}
@Override
public Long256 getLong256A(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public Long256 getLong256B(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public RecordCursorFactory getRecordCursorFactory() {
return factory;
}
@Override
public final short getShort(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final CharSequence getStrA(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final CharSequence getStrB(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final int getStrLen(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final CharSequence getSymbol(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public CharSequence getSymbolB(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final long getTimestamp(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final int getType() {
return ColumnType.CURSOR;
}
@Override
public Utf8Sequence getVarcharA(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public Utf8Sequence getVarcharB(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public final int getVarcharSize(Record rec) {
throw new UnsupportedOperationException();
}
@Override
public void toPlan(PlanSink sink) {
sink.val("cursor ").child(factory);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy