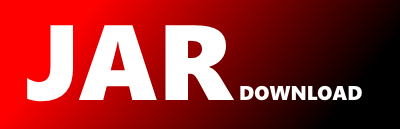
org.radarcns.connector.upload.altoida.AltoidaTestScreenElement Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.radarcns.connector.upload.altoida;
import org.apache.avro.generic.GenericArray;
import org.apache.avro.specific.SpecificData;
import org.apache.avro.util.Utf8;
import org.apache.avro.message.BinaryMessageEncoder;
import org.apache.avro.message.BinaryMessageDecoder;
import org.apache.avro.message.SchemaStore;
/** Schema for the Altoida test screen element. */
@org.apache.avro.specific.AvroGenerated
public class AltoidaTestScreenElement extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = -4992727433469625853L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"AltoidaTestScreenElement\",\"namespace\":\"org.radarcns.connector.upload.altoida\",\"doc\":\"Schema for the Altoida test screen element.\",\"fields\":[{\"name\":\"time\",\"type\":\"double\",\"doc\":\"Timestamp in UTC (s) when the assessment is started by the subject.\"},{\"name\":\"assessmentName\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Assessment name.\"},{\"name\":\"id\",\"type\":[\"null\",\"int\"],\"doc\":\"Circle id.\",\"default\":null},{\"name\":\"name\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Element name.\",\"default\":null},{\"name\":\"width\",\"type\":[\"null\",\"double\"],\"doc\":\"Element width in cm.\",\"default\":null},{\"name\":\"height\",\"type\":[\"null\",\"double\"],\"doc\":\"Element height in cm.\",\"default\":null},{\"name\":\"radius\",\"type\":[\"null\",\"double\"],\"doc\":\"Circle radius.\",\"default\":null},{\"name\":\"xCenterOffset\",\"type\":[\"null\",\"double\"],\"doc\":\"Circle location x center offset in cm.\",\"default\":null},{\"name\":\"yCenterOffset\",\"type\":[\"null\",\"double\"],\"doc\":\"Circle location y center offset in cm.\",\"default\":null},{\"name\":\"colorAlpha\",\"type\":[\"null\",\"float\"],\"doc\":\"Circle alpha value in RGBA color space.\",\"default\":null},{\"name\":\"colorRed\",\"type\":[\"null\",\"float\"],\"doc\":\"Circle red value in RGBA color space.\",\"default\":null},{\"name\":\"colorGreen\",\"type\":[\"null\",\"float\"],\"doc\":\"Circle green value in RGBA color space.\",\"default\":null},{\"name\":\"colorBlue\",\"type\":[\"null\",\"float\"],\"doc\":\"Circle blue value in RGBA color space.\",\"default\":null}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private static final SpecificData MODEL$ = new SpecificData();
private static final BinaryMessageEncoder ENCODER =
new BinaryMessageEncoder<>(MODEL$, SCHEMA$);
private static final BinaryMessageDecoder DECODER =
new BinaryMessageDecoder<>(MODEL$, SCHEMA$);
/**
* Return the BinaryMessageEncoder instance used by this class.
* @return the message encoder used by this class
*/
public static BinaryMessageEncoder getEncoder() {
return ENCODER;
}
/**
* Return the BinaryMessageDecoder instance used by this class.
* @return the message decoder used by this class
*/
public static BinaryMessageDecoder getDecoder() {
return DECODER;
}
/**
* Create a new BinaryMessageDecoder instance for this class that uses the specified {@link SchemaStore}.
* @param resolver a {@link SchemaStore} used to find schemas by fingerprint
* @return a BinaryMessageDecoder instance for this class backed by the given SchemaStore
*/
public static BinaryMessageDecoder createDecoder(SchemaStore resolver) {
return new BinaryMessageDecoder<>(MODEL$, SCHEMA$, resolver);
}
/**
* Serializes this AltoidaTestScreenElement to a ByteBuffer.
* @return a buffer holding the serialized data for this instance
* @throws java.io.IOException if this instance could not be serialized
*/
public java.nio.ByteBuffer toByteBuffer() throws java.io.IOException {
return ENCODER.encode(this);
}
/**
* Deserializes a AltoidaTestScreenElement from a ByteBuffer.
* @param b a byte buffer holding serialized data for an instance of this class
* @return a AltoidaTestScreenElement instance decoded from the given buffer
* @throws java.io.IOException if the given bytes could not be deserialized into an instance of this class
*/
public static AltoidaTestScreenElement fromByteBuffer(
java.nio.ByteBuffer b) throws java.io.IOException {
return DECODER.decode(b);
}
/** Timestamp in UTC (s) when the assessment is started by the subject. */
private double time;
/** Assessment name. */
private java.lang.String assessmentName;
/** Circle id. */
private java.lang.Integer id;
/** Element name. */
private java.lang.String name;
/** Element width in cm. */
private java.lang.Double width;
/** Element height in cm. */
private java.lang.Double height;
/** Circle radius. */
private java.lang.Double radius;
/** Circle location x center offset in cm. */
private java.lang.Double xCenterOffset;
/** Circle location y center offset in cm. */
private java.lang.Double yCenterOffset;
/** Circle alpha value in RGBA color space. */
private java.lang.Float colorAlpha;
/** Circle red value in RGBA color space. */
private java.lang.Float colorRed;
/** Circle green value in RGBA color space. */
private java.lang.Float colorGreen;
/** Circle blue value in RGBA color space. */
private java.lang.Float colorBlue;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public AltoidaTestScreenElement() {}
/**
* All-args constructor.
* @param time Timestamp in UTC (s) when the assessment is started by the subject.
* @param assessmentName Assessment name.
* @param id Circle id.
* @param name Element name.
* @param width Element width in cm.
* @param height Element height in cm.
* @param radius Circle radius.
* @param xCenterOffset Circle location x center offset in cm.
* @param yCenterOffset Circle location y center offset in cm.
* @param colorAlpha Circle alpha value in RGBA color space.
* @param colorRed Circle red value in RGBA color space.
* @param colorGreen Circle green value in RGBA color space.
* @param colorBlue Circle blue value in RGBA color space.
*/
public AltoidaTestScreenElement(java.lang.Double time, java.lang.String assessmentName, java.lang.Integer id, java.lang.String name, java.lang.Double width, java.lang.Double height, java.lang.Double radius, java.lang.Double xCenterOffset, java.lang.Double yCenterOffset, java.lang.Float colorAlpha, java.lang.Float colorRed, java.lang.Float colorGreen, java.lang.Float colorBlue) {
this.time = time;
this.assessmentName = assessmentName;
this.id = id;
this.name = name;
this.width = width;
this.height = height;
this.radius = radius;
this.xCenterOffset = xCenterOffset;
this.yCenterOffset = yCenterOffset;
this.colorAlpha = colorAlpha;
this.colorRed = colorRed;
this.colorGreen = colorGreen;
this.colorBlue = colorBlue;
}
@Override
public org.apache.avro.specific.SpecificData getSpecificData() { return MODEL$; }
@Override
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
@Override
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return time;
case 1: return assessmentName;
case 2: return id;
case 3: return name;
case 4: return width;
case 5: return height;
case 6: return radius;
case 7: return xCenterOffset;
case 8: return yCenterOffset;
case 9: return colorAlpha;
case 10: return colorRed;
case 11: return colorGreen;
case 12: return colorBlue;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
// Used by DatumReader. Applications should not call.
@Override
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: time = (java.lang.Double)value$; break;
case 1: assessmentName = value$ != null ? value$.toString() : null; break;
case 2: id = (java.lang.Integer)value$; break;
case 3: name = value$ != null ? value$.toString() : null; break;
case 4: width = (java.lang.Double)value$; break;
case 5: height = (java.lang.Double)value$; break;
case 6: radius = (java.lang.Double)value$; break;
case 7: xCenterOffset = (java.lang.Double)value$; break;
case 8: yCenterOffset = (java.lang.Double)value$; break;
case 9: colorAlpha = (java.lang.Float)value$; break;
case 10: colorRed = (java.lang.Float)value$; break;
case 11: colorGreen = (java.lang.Float)value$; break;
case 12: colorBlue = (java.lang.Float)value$; break;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
/**
* Gets the value of the 'time' field.
* @return Timestamp in UTC (s) when the assessment is started by the subject.
*/
public double getTime() {
return time;
}
/**
* Sets the value of the 'time' field.
* Timestamp in UTC (s) when the assessment is started by the subject.
* @param value the value to set.
*/
public void setTime(double value) {
this.time = value;
}
/**
* Gets the value of the 'assessmentName' field.
* @return Assessment name.
*/
public java.lang.String getAssessmentName() {
return assessmentName;
}
/**
* Sets the value of the 'assessmentName' field.
* Assessment name.
* @param value the value to set.
*/
public void setAssessmentName(java.lang.String value) {
this.assessmentName = value;
}
/**
* Gets the value of the 'id' field.
* @return Circle id.
*/
public java.lang.Integer getId() {
return id;
}
/**
* Sets the value of the 'id' field.
* Circle id.
* @param value the value to set.
*/
public void setId(java.lang.Integer value) {
this.id = value;
}
/**
* Gets the value of the 'name' field.
* @return Element name.
*/
public java.lang.String getName() {
return name;
}
/**
* Sets the value of the 'name' field.
* Element name.
* @param value the value to set.
*/
public void setName(java.lang.String value) {
this.name = value;
}
/**
* Gets the value of the 'width' field.
* @return Element width in cm.
*/
public java.lang.Double getWidth() {
return width;
}
/**
* Sets the value of the 'width' field.
* Element width in cm.
* @param value the value to set.
*/
public void setWidth(java.lang.Double value) {
this.width = value;
}
/**
* Gets the value of the 'height' field.
* @return Element height in cm.
*/
public java.lang.Double getHeight() {
return height;
}
/**
* Sets the value of the 'height' field.
* Element height in cm.
* @param value the value to set.
*/
public void setHeight(java.lang.Double value) {
this.height = value;
}
/**
* Gets the value of the 'radius' field.
* @return Circle radius.
*/
public java.lang.Double getRadius() {
return radius;
}
/**
* Sets the value of the 'radius' field.
* Circle radius.
* @param value the value to set.
*/
public void setRadius(java.lang.Double value) {
this.radius = value;
}
/**
* Gets the value of the 'xCenterOffset' field.
* @return Circle location x center offset in cm.
*/
public java.lang.Double getXCenterOffset() {
return xCenterOffset;
}
/**
* Sets the value of the 'xCenterOffset' field.
* Circle location x center offset in cm.
* @param value the value to set.
*/
public void setXCenterOffset(java.lang.Double value) {
this.xCenterOffset = value;
}
/**
* Gets the value of the 'yCenterOffset' field.
* @return Circle location y center offset in cm.
*/
public java.lang.Double getYCenterOffset() {
return yCenterOffset;
}
/**
* Sets the value of the 'yCenterOffset' field.
* Circle location y center offset in cm.
* @param value the value to set.
*/
public void setYCenterOffset(java.lang.Double value) {
this.yCenterOffset = value;
}
/**
* Gets the value of the 'colorAlpha' field.
* @return Circle alpha value in RGBA color space.
*/
public java.lang.Float getColorAlpha() {
return colorAlpha;
}
/**
* Sets the value of the 'colorAlpha' field.
* Circle alpha value in RGBA color space.
* @param value the value to set.
*/
public void setColorAlpha(java.lang.Float value) {
this.colorAlpha = value;
}
/**
* Gets the value of the 'colorRed' field.
* @return Circle red value in RGBA color space.
*/
public java.lang.Float getColorRed() {
return colorRed;
}
/**
* Sets the value of the 'colorRed' field.
* Circle red value in RGBA color space.
* @param value the value to set.
*/
public void setColorRed(java.lang.Float value) {
this.colorRed = value;
}
/**
* Gets the value of the 'colorGreen' field.
* @return Circle green value in RGBA color space.
*/
public java.lang.Float getColorGreen() {
return colorGreen;
}
/**
* Sets the value of the 'colorGreen' field.
* Circle green value in RGBA color space.
* @param value the value to set.
*/
public void setColorGreen(java.lang.Float value) {
this.colorGreen = value;
}
/**
* Gets the value of the 'colorBlue' field.
* @return Circle blue value in RGBA color space.
*/
public java.lang.Float getColorBlue() {
return colorBlue;
}
/**
* Sets the value of the 'colorBlue' field.
* Circle blue value in RGBA color space.
* @param value the value to set.
*/
public void setColorBlue(java.lang.Float value) {
this.colorBlue = value;
}
/**
* Creates a new AltoidaTestScreenElement RecordBuilder.
* @return A new AltoidaTestScreenElement RecordBuilder
*/
public static org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder newBuilder() {
return new org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder();
}
/**
* Creates a new AltoidaTestScreenElement RecordBuilder by copying an existing Builder.
* @param other The existing builder to copy.
* @return A new AltoidaTestScreenElement RecordBuilder
*/
public static org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder newBuilder(org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder other) {
if (other == null) {
return new org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder();
} else {
return new org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder(other);
}
}
/**
* Creates a new AltoidaTestScreenElement RecordBuilder by copying an existing AltoidaTestScreenElement instance.
* @param other The existing instance to copy.
* @return A new AltoidaTestScreenElement RecordBuilder
*/
public static org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder newBuilder(org.radarcns.connector.upload.altoida.AltoidaTestScreenElement other) {
if (other == null) {
return new org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder();
} else {
return new org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder(other);
}
}
/**
* RecordBuilder for AltoidaTestScreenElement instances.
*/
@org.apache.avro.specific.AvroGenerated
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
/** Timestamp in UTC (s) when the assessment is started by the subject. */
private double time;
/** Assessment name. */
private java.lang.String assessmentName;
/** Circle id. */
private java.lang.Integer id;
/** Element name. */
private java.lang.String name;
/** Element width in cm. */
private java.lang.Double width;
/** Element height in cm. */
private java.lang.Double height;
/** Circle radius. */
private java.lang.Double radius;
/** Circle location x center offset in cm. */
private java.lang.Double xCenterOffset;
/** Circle location y center offset in cm. */
private java.lang.Double yCenterOffset;
/** Circle alpha value in RGBA color space. */
private java.lang.Float colorAlpha;
/** Circle red value in RGBA color space. */
private java.lang.Float colorRed;
/** Circle green value in RGBA color space. */
private java.lang.Float colorGreen;
/** Circle blue value in RGBA color space. */
private java.lang.Float colorBlue;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$, MODEL$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder other) {
super(other);
if (isValidValue(fields()[0], other.time)) {
this.time = data().deepCopy(fields()[0].schema(), other.time);
fieldSetFlags()[0] = other.fieldSetFlags()[0];
}
if (isValidValue(fields()[1], other.assessmentName)) {
this.assessmentName = data().deepCopy(fields()[1].schema(), other.assessmentName);
fieldSetFlags()[1] = other.fieldSetFlags()[1];
}
if (isValidValue(fields()[2], other.id)) {
this.id = data().deepCopy(fields()[2].schema(), other.id);
fieldSetFlags()[2] = other.fieldSetFlags()[2];
}
if (isValidValue(fields()[3], other.name)) {
this.name = data().deepCopy(fields()[3].schema(), other.name);
fieldSetFlags()[3] = other.fieldSetFlags()[3];
}
if (isValidValue(fields()[4], other.width)) {
this.width = data().deepCopy(fields()[4].schema(), other.width);
fieldSetFlags()[4] = other.fieldSetFlags()[4];
}
if (isValidValue(fields()[5], other.height)) {
this.height = data().deepCopy(fields()[5].schema(), other.height);
fieldSetFlags()[5] = other.fieldSetFlags()[5];
}
if (isValidValue(fields()[6], other.radius)) {
this.radius = data().deepCopy(fields()[6].schema(), other.radius);
fieldSetFlags()[6] = other.fieldSetFlags()[6];
}
if (isValidValue(fields()[7], other.xCenterOffset)) {
this.xCenterOffset = data().deepCopy(fields()[7].schema(), other.xCenterOffset);
fieldSetFlags()[7] = other.fieldSetFlags()[7];
}
if (isValidValue(fields()[8], other.yCenterOffset)) {
this.yCenterOffset = data().deepCopy(fields()[8].schema(), other.yCenterOffset);
fieldSetFlags()[8] = other.fieldSetFlags()[8];
}
if (isValidValue(fields()[9], other.colorAlpha)) {
this.colorAlpha = data().deepCopy(fields()[9].schema(), other.colorAlpha);
fieldSetFlags()[9] = other.fieldSetFlags()[9];
}
if (isValidValue(fields()[10], other.colorRed)) {
this.colorRed = data().deepCopy(fields()[10].schema(), other.colorRed);
fieldSetFlags()[10] = other.fieldSetFlags()[10];
}
if (isValidValue(fields()[11], other.colorGreen)) {
this.colorGreen = data().deepCopy(fields()[11].schema(), other.colorGreen);
fieldSetFlags()[11] = other.fieldSetFlags()[11];
}
if (isValidValue(fields()[12], other.colorBlue)) {
this.colorBlue = data().deepCopy(fields()[12].schema(), other.colorBlue);
fieldSetFlags()[12] = other.fieldSetFlags()[12];
}
}
/**
* Creates a Builder by copying an existing AltoidaTestScreenElement instance
* @param other The existing instance to copy.
*/
private Builder(org.radarcns.connector.upload.altoida.AltoidaTestScreenElement other) {
super(SCHEMA$, MODEL$);
if (isValidValue(fields()[0], other.time)) {
this.time = data().deepCopy(fields()[0].schema(), other.time);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.assessmentName)) {
this.assessmentName = data().deepCopy(fields()[1].schema(), other.assessmentName);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.id)) {
this.id = data().deepCopy(fields()[2].schema(), other.id);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.name)) {
this.name = data().deepCopy(fields()[3].schema(), other.name);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.width)) {
this.width = data().deepCopy(fields()[4].schema(), other.width);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.height)) {
this.height = data().deepCopy(fields()[5].schema(), other.height);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.radius)) {
this.radius = data().deepCopy(fields()[6].schema(), other.radius);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.xCenterOffset)) {
this.xCenterOffset = data().deepCopy(fields()[7].schema(), other.xCenterOffset);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.yCenterOffset)) {
this.yCenterOffset = data().deepCopy(fields()[8].schema(), other.yCenterOffset);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.colorAlpha)) {
this.colorAlpha = data().deepCopy(fields()[9].schema(), other.colorAlpha);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.colorRed)) {
this.colorRed = data().deepCopy(fields()[10].schema(), other.colorRed);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.colorGreen)) {
this.colorGreen = data().deepCopy(fields()[11].schema(), other.colorGreen);
fieldSetFlags()[11] = true;
}
if (isValidValue(fields()[12], other.colorBlue)) {
this.colorBlue = data().deepCopy(fields()[12].schema(), other.colorBlue);
fieldSetFlags()[12] = true;
}
}
/**
* Gets the value of the 'time' field.
* Timestamp in UTC (s) when the assessment is started by the subject.
* @return The value.
*/
public double getTime() {
return time;
}
/**
* Sets the value of the 'time' field.
* Timestamp in UTC (s) when the assessment is started by the subject.
* @param value The value of 'time'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setTime(double value) {
validate(fields()[0], value);
this.time = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'time' field has been set.
* Timestamp in UTC (s) when the assessment is started by the subject.
* @return True if the 'time' field has been set, false otherwise.
*/
public boolean hasTime() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'time' field.
* Timestamp in UTC (s) when the assessment is started by the subject.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearTime() {
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'assessmentName' field.
* Assessment name.
* @return The value.
*/
public java.lang.String getAssessmentName() {
return assessmentName;
}
/**
* Sets the value of the 'assessmentName' field.
* Assessment name.
* @param value The value of 'assessmentName'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setAssessmentName(java.lang.String value) {
validate(fields()[1], value);
this.assessmentName = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'assessmentName' field has been set.
* Assessment name.
* @return True if the 'assessmentName' field has been set, false otherwise.
*/
public boolean hasAssessmentName() {
return fieldSetFlags()[1];
}
/**
* Clears the value of the 'assessmentName' field.
* Assessment name.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearAssessmentName() {
assessmentName = null;
fieldSetFlags()[1] = false;
return this;
}
/**
* Gets the value of the 'id' field.
* Circle id.
* @return The value.
*/
public java.lang.Integer getId() {
return id;
}
/**
* Sets the value of the 'id' field.
* Circle id.
* @param value The value of 'id'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setId(java.lang.Integer value) {
validate(fields()[2], value);
this.id = value;
fieldSetFlags()[2] = true;
return this;
}
/**
* Checks whether the 'id' field has been set.
* Circle id.
* @return True if the 'id' field has been set, false otherwise.
*/
public boolean hasId() {
return fieldSetFlags()[2];
}
/**
* Clears the value of the 'id' field.
* Circle id.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearId() {
id = null;
fieldSetFlags()[2] = false;
return this;
}
/**
* Gets the value of the 'name' field.
* Element name.
* @return The value.
*/
public java.lang.String getName() {
return name;
}
/**
* Sets the value of the 'name' field.
* Element name.
* @param value The value of 'name'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setName(java.lang.String value) {
validate(fields()[3], value);
this.name = value;
fieldSetFlags()[3] = true;
return this;
}
/**
* Checks whether the 'name' field has been set.
* Element name.
* @return True if the 'name' field has been set, false otherwise.
*/
public boolean hasName() {
return fieldSetFlags()[3];
}
/**
* Clears the value of the 'name' field.
* Element name.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearName() {
name = null;
fieldSetFlags()[3] = false;
return this;
}
/**
* Gets the value of the 'width' field.
* Element width in cm.
* @return The value.
*/
public java.lang.Double getWidth() {
return width;
}
/**
* Sets the value of the 'width' field.
* Element width in cm.
* @param value The value of 'width'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setWidth(java.lang.Double value) {
validate(fields()[4], value);
this.width = value;
fieldSetFlags()[4] = true;
return this;
}
/**
* Checks whether the 'width' field has been set.
* Element width in cm.
* @return True if the 'width' field has been set, false otherwise.
*/
public boolean hasWidth() {
return fieldSetFlags()[4];
}
/**
* Clears the value of the 'width' field.
* Element width in cm.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearWidth() {
width = null;
fieldSetFlags()[4] = false;
return this;
}
/**
* Gets the value of the 'height' field.
* Element height in cm.
* @return The value.
*/
public java.lang.Double getHeight() {
return height;
}
/**
* Sets the value of the 'height' field.
* Element height in cm.
* @param value The value of 'height'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setHeight(java.lang.Double value) {
validate(fields()[5], value);
this.height = value;
fieldSetFlags()[5] = true;
return this;
}
/**
* Checks whether the 'height' field has been set.
* Element height in cm.
* @return True if the 'height' field has been set, false otherwise.
*/
public boolean hasHeight() {
return fieldSetFlags()[5];
}
/**
* Clears the value of the 'height' field.
* Element height in cm.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearHeight() {
height = null;
fieldSetFlags()[5] = false;
return this;
}
/**
* Gets the value of the 'radius' field.
* Circle radius.
* @return The value.
*/
public java.lang.Double getRadius() {
return radius;
}
/**
* Sets the value of the 'radius' field.
* Circle radius.
* @param value The value of 'radius'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setRadius(java.lang.Double value) {
validate(fields()[6], value);
this.radius = value;
fieldSetFlags()[6] = true;
return this;
}
/**
* Checks whether the 'radius' field has been set.
* Circle radius.
* @return True if the 'radius' field has been set, false otherwise.
*/
public boolean hasRadius() {
return fieldSetFlags()[6];
}
/**
* Clears the value of the 'radius' field.
* Circle radius.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearRadius() {
radius = null;
fieldSetFlags()[6] = false;
return this;
}
/**
* Gets the value of the 'xCenterOffset' field.
* Circle location x center offset in cm.
* @return The value.
*/
public java.lang.Double getXCenterOffset() {
return xCenterOffset;
}
/**
* Sets the value of the 'xCenterOffset' field.
* Circle location x center offset in cm.
* @param value The value of 'xCenterOffset'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setXCenterOffset(java.lang.Double value) {
validate(fields()[7], value);
this.xCenterOffset = value;
fieldSetFlags()[7] = true;
return this;
}
/**
* Checks whether the 'xCenterOffset' field has been set.
* Circle location x center offset in cm.
* @return True if the 'xCenterOffset' field has been set, false otherwise.
*/
public boolean hasXCenterOffset() {
return fieldSetFlags()[7];
}
/**
* Clears the value of the 'xCenterOffset' field.
* Circle location x center offset in cm.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearXCenterOffset() {
xCenterOffset = null;
fieldSetFlags()[7] = false;
return this;
}
/**
* Gets the value of the 'yCenterOffset' field.
* Circle location y center offset in cm.
* @return The value.
*/
public java.lang.Double getYCenterOffset() {
return yCenterOffset;
}
/**
* Sets the value of the 'yCenterOffset' field.
* Circle location y center offset in cm.
* @param value The value of 'yCenterOffset'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setYCenterOffset(java.lang.Double value) {
validate(fields()[8], value);
this.yCenterOffset = value;
fieldSetFlags()[8] = true;
return this;
}
/**
* Checks whether the 'yCenterOffset' field has been set.
* Circle location y center offset in cm.
* @return True if the 'yCenterOffset' field has been set, false otherwise.
*/
public boolean hasYCenterOffset() {
return fieldSetFlags()[8];
}
/**
* Clears the value of the 'yCenterOffset' field.
* Circle location y center offset in cm.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearYCenterOffset() {
yCenterOffset = null;
fieldSetFlags()[8] = false;
return this;
}
/**
* Gets the value of the 'colorAlpha' field.
* Circle alpha value in RGBA color space.
* @return The value.
*/
public java.lang.Float getColorAlpha() {
return colorAlpha;
}
/**
* Sets the value of the 'colorAlpha' field.
* Circle alpha value in RGBA color space.
* @param value The value of 'colorAlpha'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setColorAlpha(java.lang.Float value) {
validate(fields()[9], value);
this.colorAlpha = value;
fieldSetFlags()[9] = true;
return this;
}
/**
* Checks whether the 'colorAlpha' field has been set.
* Circle alpha value in RGBA color space.
* @return True if the 'colorAlpha' field has been set, false otherwise.
*/
public boolean hasColorAlpha() {
return fieldSetFlags()[9];
}
/**
* Clears the value of the 'colorAlpha' field.
* Circle alpha value in RGBA color space.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearColorAlpha() {
colorAlpha = null;
fieldSetFlags()[9] = false;
return this;
}
/**
* Gets the value of the 'colorRed' field.
* Circle red value in RGBA color space.
* @return The value.
*/
public java.lang.Float getColorRed() {
return colorRed;
}
/**
* Sets the value of the 'colorRed' field.
* Circle red value in RGBA color space.
* @param value The value of 'colorRed'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setColorRed(java.lang.Float value) {
validate(fields()[10], value);
this.colorRed = value;
fieldSetFlags()[10] = true;
return this;
}
/**
* Checks whether the 'colorRed' field has been set.
* Circle red value in RGBA color space.
* @return True if the 'colorRed' field has been set, false otherwise.
*/
public boolean hasColorRed() {
return fieldSetFlags()[10];
}
/**
* Clears the value of the 'colorRed' field.
* Circle red value in RGBA color space.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearColorRed() {
colorRed = null;
fieldSetFlags()[10] = false;
return this;
}
/**
* Gets the value of the 'colorGreen' field.
* Circle green value in RGBA color space.
* @return The value.
*/
public java.lang.Float getColorGreen() {
return colorGreen;
}
/**
* Sets the value of the 'colorGreen' field.
* Circle green value in RGBA color space.
* @param value The value of 'colorGreen'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setColorGreen(java.lang.Float value) {
validate(fields()[11], value);
this.colorGreen = value;
fieldSetFlags()[11] = true;
return this;
}
/**
* Checks whether the 'colorGreen' field has been set.
* Circle green value in RGBA color space.
* @return True if the 'colorGreen' field has been set, false otherwise.
*/
public boolean hasColorGreen() {
return fieldSetFlags()[11];
}
/**
* Clears the value of the 'colorGreen' field.
* Circle green value in RGBA color space.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearColorGreen() {
colorGreen = null;
fieldSetFlags()[11] = false;
return this;
}
/**
* Gets the value of the 'colorBlue' field.
* Circle blue value in RGBA color space.
* @return The value.
*/
public java.lang.Float getColorBlue() {
return colorBlue;
}
/**
* Sets the value of the 'colorBlue' field.
* Circle blue value in RGBA color space.
* @param value The value of 'colorBlue'.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder setColorBlue(java.lang.Float value) {
validate(fields()[12], value);
this.colorBlue = value;
fieldSetFlags()[12] = true;
return this;
}
/**
* Checks whether the 'colorBlue' field has been set.
* Circle blue value in RGBA color space.
* @return True if the 'colorBlue' field has been set, false otherwise.
*/
public boolean hasColorBlue() {
return fieldSetFlags()[12];
}
/**
* Clears the value of the 'colorBlue' field.
* Circle blue value in RGBA color space.
* @return This builder.
*/
public org.radarcns.connector.upload.altoida.AltoidaTestScreenElement.Builder clearColorBlue() {
colorBlue = null;
fieldSetFlags()[12] = false;
return this;
}
@Override
@SuppressWarnings("unchecked")
public AltoidaTestScreenElement build() {
try {
AltoidaTestScreenElement record = new AltoidaTestScreenElement();
record.time = fieldSetFlags()[0] ? this.time : (java.lang.Double) defaultValue(fields()[0]);
record.assessmentName = fieldSetFlags()[1] ? this.assessmentName : (java.lang.String) defaultValue(fields()[1]);
record.id = fieldSetFlags()[2] ? this.id : (java.lang.Integer) defaultValue(fields()[2]);
record.name = fieldSetFlags()[3] ? this.name : (java.lang.String) defaultValue(fields()[3]);
record.width = fieldSetFlags()[4] ? this.width : (java.lang.Double) defaultValue(fields()[4]);
record.height = fieldSetFlags()[5] ? this.height : (java.lang.Double) defaultValue(fields()[5]);
record.radius = fieldSetFlags()[6] ? this.radius : (java.lang.Double) defaultValue(fields()[6]);
record.xCenterOffset = fieldSetFlags()[7] ? this.xCenterOffset : (java.lang.Double) defaultValue(fields()[7]);
record.yCenterOffset = fieldSetFlags()[8] ? this.yCenterOffset : (java.lang.Double) defaultValue(fields()[8]);
record.colorAlpha = fieldSetFlags()[9] ? this.colorAlpha : (java.lang.Float) defaultValue(fields()[9]);
record.colorRed = fieldSetFlags()[10] ? this.colorRed : (java.lang.Float) defaultValue(fields()[10]);
record.colorGreen = fieldSetFlags()[11] ? this.colorGreen : (java.lang.Float) defaultValue(fields()[11]);
record.colorBlue = fieldSetFlags()[12] ? this.colorBlue : (java.lang.Float) defaultValue(fields()[12]);
return record;
} catch (org.apache.avro.AvroMissingFieldException e) {
throw e;
} catch (java.lang.Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumWriter
WRITER$ = (org.apache.avro.io.DatumWriter)MODEL$.createDatumWriter(SCHEMA$);
@Override public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumReader
READER$ = (org.apache.avro.io.DatumReader)MODEL$.createDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
@Override protected boolean hasCustomCoders() { return true; }
@Override public void customEncode(org.apache.avro.io.Encoder out)
throws java.io.IOException
{
out.writeDouble(this.time);
out.writeString(this.assessmentName);
if (this.id == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeInt(this.id);
}
if (this.name == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeString(this.name);
}
if (this.width == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeDouble(this.width);
}
if (this.height == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeDouble(this.height);
}
if (this.radius == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeDouble(this.radius);
}
if (this.xCenterOffset == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeDouble(this.xCenterOffset);
}
if (this.yCenterOffset == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeDouble(this.yCenterOffset);
}
if (this.colorAlpha == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.colorAlpha);
}
if (this.colorRed == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.colorRed);
}
if (this.colorGreen == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.colorGreen);
}
if (this.colorBlue == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.colorBlue);
}
}
@Override public void customDecode(org.apache.avro.io.ResolvingDecoder in)
throws java.io.IOException
{
org.apache.avro.Schema.Field[] fieldOrder = in.readFieldOrderIfDiff();
if (fieldOrder == null) {
this.time = in.readDouble();
this.assessmentName = in.readString();
if (in.readIndex() != 1) {
in.readNull();
this.id = null;
} else {
this.id = in.readInt();
}
if (in.readIndex() != 1) {
in.readNull();
this.name = null;
} else {
this.name = in.readString();
}
if (in.readIndex() != 1) {
in.readNull();
this.width = null;
} else {
this.width = in.readDouble();
}
if (in.readIndex() != 1) {
in.readNull();
this.height = null;
} else {
this.height = in.readDouble();
}
if (in.readIndex() != 1) {
in.readNull();
this.radius = null;
} else {
this.radius = in.readDouble();
}
if (in.readIndex() != 1) {
in.readNull();
this.xCenterOffset = null;
} else {
this.xCenterOffset = in.readDouble();
}
if (in.readIndex() != 1) {
in.readNull();
this.yCenterOffset = null;
} else {
this.yCenterOffset = in.readDouble();
}
if (in.readIndex() != 1) {
in.readNull();
this.colorAlpha = null;
} else {
this.colorAlpha = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.colorRed = null;
} else {
this.colorRed = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.colorGreen = null;
} else {
this.colorGreen = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.colorBlue = null;
} else {
this.colorBlue = in.readFloat();
}
} else {
for (int i = 0; i < 13; i++) {
switch (fieldOrder[i].pos()) {
case 0:
this.time = in.readDouble();
break;
case 1:
this.assessmentName = in.readString();
break;
case 2:
if (in.readIndex() != 1) {
in.readNull();
this.id = null;
} else {
this.id = in.readInt();
}
break;
case 3:
if (in.readIndex() != 1) {
in.readNull();
this.name = null;
} else {
this.name = in.readString();
}
break;
case 4:
if (in.readIndex() != 1) {
in.readNull();
this.width = null;
} else {
this.width = in.readDouble();
}
break;
case 5:
if (in.readIndex() != 1) {
in.readNull();
this.height = null;
} else {
this.height = in.readDouble();
}
break;
case 6:
if (in.readIndex() != 1) {
in.readNull();
this.radius = null;
} else {
this.radius = in.readDouble();
}
break;
case 7:
if (in.readIndex() != 1) {
in.readNull();
this.xCenterOffset = null;
} else {
this.xCenterOffset = in.readDouble();
}
break;
case 8:
if (in.readIndex() != 1) {
in.readNull();
this.yCenterOffset = null;
} else {
this.yCenterOffset = in.readDouble();
}
break;
case 9:
if (in.readIndex() != 1) {
in.readNull();
this.colorAlpha = null;
} else {
this.colorAlpha = in.readFloat();
}
break;
case 10:
if (in.readIndex() != 1) {
in.readNull();
this.colorRed = null;
} else {
this.colorRed = in.readFloat();
}
break;
case 11:
if (in.readIndex() != 1) {
in.readNull();
this.colorGreen = null;
} else {
this.colorGreen = in.readFloat();
}
break;
case 12:
if (in.readIndex() != 1) {
in.readNull();
this.colorBlue = null;
} else {
this.colorBlue = in.readFloat();
}
break;
default:
throw new java.io.IOException("Corrupt ResolvingDecoder.");
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy