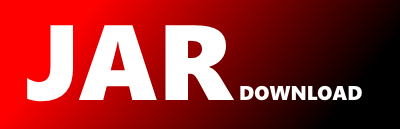
org.radarcns.passive.carl.FibaroDevice Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.radarcns.passive.carl;
import org.apache.avro.generic.GenericArray;
import org.apache.avro.specific.SpecificData;
import org.apache.avro.util.Utf8;
import org.apache.avro.message.BinaryMessageEncoder;
import org.apache.avro.message.BinaryMessageDecoder;
import org.apache.avro.message.SchemaStore;
/** A Fibaro Device, capturing multiple variables for a specific Fibaro device. More information can be found https://www.fibaro.com/. */
@org.apache.avro.specific.AvroGenerated
public class FibaroDevice extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = 8083304347629627536L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"FibaroDevice\",\"namespace\":\"org.radarcns.passive.carl\",\"doc\":\"A Fibaro Device, capturing multiple variables for a specific Fibaro device. More information can be found https://www.fibaro.com/.\",\"fields\":[{\"name\":\"time\",\"type\":\"double\",\"doc\":\"Time since the Unix Epoch (seconds), represents the time that an Event was registered.\"},{\"name\":\"timeReceived\",\"type\":\"double\",\"doc\":\"Time since the Unix Epoch (seconds), represents the time this record was received.\"},{\"name\":\"id\",\"type\":\"int\",\"doc\":\"A unique ID for a Fibaro Device. As registered in Carl Cloud.\"},{\"name\":\"hclId\",\"type\":\"int\",\"doc\":\"Unique ID for a Fibaro Device. As registered in Home Center Lite's API.\"},{\"name\":\"deviceType\",\"type\":{\"type\":\"enum\",\"name\":\"DeviceType\",\"doc\":\"PRESENSE_SENSOR: Devices that act as motion sensor, temperature sensor, seismic sensor ,light sensor, accelerometer,DOOR_SENSOR: Devices that register if the door/windows was opened as well as heat changes, FLODD_SENSOR: Devices that registeres flood inside the house, PANIC_BUTTON: Device that when pressed alerts the system, WALLPLUG: Device that measures the electric consumption of a device connected to it.\",\"symbols\":[\"PRESENSE_SENSOR\",\"DOOR_SENSOR\",\"FLOOD_SENSOR\",\"PANIC_BUTTON\",\"WALLPLUG\"]},\"doc\":\"Type of the device (Presense, Door, Flood, Panic, Wall Plug).\"},{\"name\":\"serial\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"A serial number for a Fibaro Device.\"},{\"name\":\"make\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The name of a Device's Manufacturer.\",\"default\":null},{\"name\":\"model\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"The model name of the Fibaro Device.\"},{\"name\":\"battery\",\"type\":\"double\",\"doc\":\"The level of Device's Battery.\"},{\"name\":\"lastSyncTime\",\"type\":\"double\",\"doc\":\"Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.\"},{\"name\":\"mac\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The MAC Addresss of the Fibaro Device.\",\"default\":null},{\"name\":\"userId\",\"type\":\"int\",\"doc\":\"Unique ID for the user who registered the Fibaro Device.\"},{\"name\":\"name\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"Name of the Fibaro Device.\",\"default\":null},{\"name\":\"roomId\",\"type\":\"int\",\"doc\":\"Unique ID of the Room that the Fibaro Device is registered to.\"},{\"name\":\"enabled\",\"type\":\"boolean\",\"doc\":\"True if the Fibaro Device is enabled, False if it is not.\"},{\"name\":\"parentId\",\"type\":\"int\",\"doc\":\"Unique ID of the Fibaro Device where this Fibaro Instance belongs to.\"},{\"name\":\"categories\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"Show the categories in which the Device belongs to.\"},{\"name\":\"configured\",\"type\":\"boolean\",\"doc\":\"True if the device is configured.\"},{\"name\":\"dead\",\"type\":\"boolean\",\"doc\":\"True if the device is not found on the network.\"},{\"name\":\"deadReason\",\"type\":[\"null\",{\"type\":\"string\",\"avro.java.string\":\"String\"}],\"doc\":\"The reason that 'dead' field was set as True.\",\"default\":null},{\"name\":\"created\",\"type\":\"double\",\"doc\":\"Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.\"},{\"name\":\"modified\",\"type\":\"double\",\"doc\":\"Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private static final SpecificData MODEL$ = new SpecificData();
private static final BinaryMessageEncoder ENCODER =
new BinaryMessageEncoder<>(MODEL$, SCHEMA$);
private static final BinaryMessageDecoder DECODER =
new BinaryMessageDecoder<>(MODEL$, SCHEMA$);
/**
* Return the BinaryMessageEncoder instance used by this class.
* @return the message encoder used by this class
*/
public static BinaryMessageEncoder getEncoder() {
return ENCODER;
}
/**
* Return the BinaryMessageDecoder instance used by this class.
* @return the message decoder used by this class
*/
public static BinaryMessageDecoder getDecoder() {
return DECODER;
}
/**
* Create a new BinaryMessageDecoder instance for this class that uses the specified {@link SchemaStore}.
* @param resolver a {@link SchemaStore} used to find schemas by fingerprint
* @return a BinaryMessageDecoder instance for this class backed by the given SchemaStore
*/
public static BinaryMessageDecoder createDecoder(SchemaStore resolver) {
return new BinaryMessageDecoder<>(MODEL$, SCHEMA$, resolver);
}
/**
* Serializes this FibaroDevice to a ByteBuffer.
* @return a buffer holding the serialized data for this instance
* @throws java.io.IOException if this instance could not be serialized
*/
public java.nio.ByteBuffer toByteBuffer() throws java.io.IOException {
return ENCODER.encode(this);
}
/**
* Deserializes a FibaroDevice from a ByteBuffer.
* @param b a byte buffer holding serialized data for an instance of this class
* @return a FibaroDevice instance decoded from the given buffer
* @throws java.io.IOException if the given bytes could not be deserialized into an instance of this class
*/
public static FibaroDevice fromByteBuffer(
java.nio.ByteBuffer b) throws java.io.IOException {
return DECODER.decode(b);
}
/** Time since the Unix Epoch (seconds), represents the time that an Event was registered. */
private double time;
/** Time since the Unix Epoch (seconds), represents the time this record was received. */
private double timeReceived;
/** A unique ID for a Fibaro Device. As registered in Carl Cloud. */
private int id;
/** Unique ID for a Fibaro Device. As registered in Home Center Lite's API. */
private int hclId;
/** Type of the device (Presense, Door, Flood, Panic, Wall Plug). */
private org.radarcns.passive.carl.DeviceType deviceType;
/** A serial number for a Fibaro Device. */
private java.lang.String serial;
/** The name of a Device's Manufacturer. */
private java.lang.String make;
/** The model name of the Fibaro Device. */
private java.lang.String model;
/** The level of Device's Battery. */
private double battery;
/** Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud. */
private double lastSyncTime;
/** The MAC Addresss of the Fibaro Device. */
private java.lang.String mac;
/** Unique ID for the user who registered the Fibaro Device. */
private int userId;
/** Name of the Fibaro Device. */
private java.lang.String name;
/** Unique ID of the Room that the Fibaro Device is registered to. */
private int roomId;
/** True if the Fibaro Device is enabled, False if it is not. */
private boolean enabled;
/** Unique ID of the Fibaro Device where this Fibaro Instance belongs to. */
private int parentId;
/** Show the categories in which the Device belongs to. */
private java.lang.String categories;
/** True if the device is configured. */
private boolean configured;
/** True if the device is not found on the network. */
private boolean dead;
/** The reason that 'dead' field was set as True. */
private java.lang.String deadReason;
/** Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync. */
private double created;
/** Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification. */
private double modified;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public FibaroDevice() {}
/**
* All-args constructor.
* @param time Time since the Unix Epoch (seconds), represents the time that an Event was registered.
* @param timeReceived Time since the Unix Epoch (seconds), represents the time this record was received.
* @param id A unique ID for a Fibaro Device. As registered in Carl Cloud.
* @param hclId Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
* @param deviceType Type of the device (Presense, Door, Flood, Panic, Wall Plug).
* @param serial A serial number for a Fibaro Device.
* @param make The name of a Device's Manufacturer.
* @param model The model name of the Fibaro Device.
* @param battery The level of Device's Battery.
* @param lastSyncTime Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
* @param mac The MAC Addresss of the Fibaro Device.
* @param userId Unique ID for the user who registered the Fibaro Device.
* @param name Name of the Fibaro Device.
* @param roomId Unique ID of the Room that the Fibaro Device is registered to.
* @param enabled True if the Fibaro Device is enabled, False if it is not.
* @param parentId Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
* @param categories Show the categories in which the Device belongs to.
* @param configured True if the device is configured.
* @param dead True if the device is not found on the network.
* @param deadReason The reason that 'dead' field was set as True.
* @param created Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
* @param modified Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
*/
public FibaroDevice(java.lang.Double time, java.lang.Double timeReceived, java.lang.Integer id, java.lang.Integer hclId, org.radarcns.passive.carl.DeviceType deviceType, java.lang.String serial, java.lang.String make, java.lang.String model, java.lang.Double battery, java.lang.Double lastSyncTime, java.lang.String mac, java.lang.Integer userId, java.lang.String name, java.lang.Integer roomId, java.lang.Boolean enabled, java.lang.Integer parentId, java.lang.String categories, java.lang.Boolean configured, java.lang.Boolean dead, java.lang.String deadReason, java.lang.Double created, java.lang.Double modified) {
this.time = time;
this.timeReceived = timeReceived;
this.id = id;
this.hclId = hclId;
this.deviceType = deviceType;
this.serial = serial;
this.make = make;
this.model = model;
this.battery = battery;
this.lastSyncTime = lastSyncTime;
this.mac = mac;
this.userId = userId;
this.name = name;
this.roomId = roomId;
this.enabled = enabled;
this.parentId = parentId;
this.categories = categories;
this.configured = configured;
this.dead = dead;
this.deadReason = deadReason;
this.created = created;
this.modified = modified;
}
@Override
public org.apache.avro.specific.SpecificData getSpecificData() { return MODEL$; }
@Override
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
@Override
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return time;
case 1: return timeReceived;
case 2: return id;
case 3: return hclId;
case 4: return deviceType;
case 5: return serial;
case 6: return make;
case 7: return model;
case 8: return battery;
case 9: return lastSyncTime;
case 10: return mac;
case 11: return userId;
case 12: return name;
case 13: return roomId;
case 14: return enabled;
case 15: return parentId;
case 16: return categories;
case 17: return configured;
case 18: return dead;
case 19: return deadReason;
case 20: return created;
case 21: return modified;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
// Used by DatumReader. Applications should not call.
@Override
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: time = (java.lang.Double)value$; break;
case 1: timeReceived = (java.lang.Double)value$; break;
case 2: id = (java.lang.Integer)value$; break;
case 3: hclId = (java.lang.Integer)value$; break;
case 4: deviceType = (org.radarcns.passive.carl.DeviceType)value$; break;
case 5: serial = value$ != null ? value$.toString() : null; break;
case 6: make = value$ != null ? value$.toString() : null; break;
case 7: model = value$ != null ? value$.toString() : null; break;
case 8: battery = (java.lang.Double)value$; break;
case 9: lastSyncTime = (java.lang.Double)value$; break;
case 10: mac = value$ != null ? value$.toString() : null; break;
case 11: userId = (java.lang.Integer)value$; break;
case 12: name = value$ != null ? value$.toString() : null; break;
case 13: roomId = (java.lang.Integer)value$; break;
case 14: enabled = (java.lang.Boolean)value$; break;
case 15: parentId = (java.lang.Integer)value$; break;
case 16: categories = value$ != null ? value$.toString() : null; break;
case 17: configured = (java.lang.Boolean)value$; break;
case 18: dead = (java.lang.Boolean)value$; break;
case 19: deadReason = value$ != null ? value$.toString() : null; break;
case 20: created = (java.lang.Double)value$; break;
case 21: modified = (java.lang.Double)value$; break;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
/**
* Gets the value of the 'time' field.
* @return Time since the Unix Epoch (seconds), represents the time that an Event was registered.
*/
public double getTime() {
return time;
}
/**
* Sets the value of the 'time' field.
* Time since the Unix Epoch (seconds), represents the time that an Event was registered.
* @param value the value to set.
*/
public void setTime(double value) {
this.time = value;
}
/**
* Gets the value of the 'timeReceived' field.
* @return Time since the Unix Epoch (seconds), represents the time this record was received.
*/
public double getTimeReceived() {
return timeReceived;
}
/**
* Sets the value of the 'timeReceived' field.
* Time since the Unix Epoch (seconds), represents the time this record was received.
* @param value the value to set.
*/
public void setTimeReceived(double value) {
this.timeReceived = value;
}
/**
* Gets the value of the 'id' field.
* @return A unique ID for a Fibaro Device. As registered in Carl Cloud.
*/
public int getId() {
return id;
}
/**
* Sets the value of the 'id' field.
* A unique ID for a Fibaro Device. As registered in Carl Cloud.
* @param value the value to set.
*/
public void setId(int value) {
this.id = value;
}
/**
* Gets the value of the 'hclId' field.
* @return Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
*/
public int getHclId() {
return hclId;
}
/**
* Sets the value of the 'hclId' field.
* Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
* @param value the value to set.
*/
public void setHclId(int value) {
this.hclId = value;
}
/**
* Gets the value of the 'deviceType' field.
* @return Type of the device (Presense, Door, Flood, Panic, Wall Plug).
*/
public org.radarcns.passive.carl.DeviceType getDeviceType() {
return deviceType;
}
/**
* Sets the value of the 'deviceType' field.
* Type of the device (Presense, Door, Flood, Panic, Wall Plug).
* @param value the value to set.
*/
public void setDeviceType(org.radarcns.passive.carl.DeviceType value) {
this.deviceType = value;
}
/**
* Gets the value of the 'serial' field.
* @return A serial number for a Fibaro Device.
*/
public java.lang.String getSerial() {
return serial;
}
/**
* Sets the value of the 'serial' field.
* A serial number for a Fibaro Device.
* @param value the value to set.
*/
public void setSerial(java.lang.String value) {
this.serial = value;
}
/**
* Gets the value of the 'make' field.
* @return The name of a Device's Manufacturer.
*/
public java.lang.String getMake() {
return make;
}
/**
* Sets the value of the 'make' field.
* The name of a Device's Manufacturer.
* @param value the value to set.
*/
public void setMake(java.lang.String value) {
this.make = value;
}
/**
* Gets the value of the 'model' field.
* @return The model name of the Fibaro Device.
*/
public java.lang.String getModel() {
return model;
}
/**
* Sets the value of the 'model' field.
* The model name of the Fibaro Device.
* @param value the value to set.
*/
public void setModel(java.lang.String value) {
this.model = value;
}
/**
* Gets the value of the 'battery' field.
* @return The level of Device's Battery.
*/
public double getBattery() {
return battery;
}
/**
* Sets the value of the 'battery' field.
* The level of Device's Battery.
* @param value the value to set.
*/
public void setBattery(double value) {
this.battery = value;
}
/**
* Gets the value of the 'lastSyncTime' field.
* @return Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
*/
public double getLastSyncTime() {
return lastSyncTime;
}
/**
* Sets the value of the 'lastSyncTime' field.
* Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
* @param value the value to set.
*/
public void setLastSyncTime(double value) {
this.lastSyncTime = value;
}
/**
* Gets the value of the 'mac' field.
* @return The MAC Addresss of the Fibaro Device.
*/
public java.lang.String getMac() {
return mac;
}
/**
* Sets the value of the 'mac' field.
* The MAC Addresss of the Fibaro Device.
* @param value the value to set.
*/
public void setMac(java.lang.String value) {
this.mac = value;
}
/**
* Gets the value of the 'userId' field.
* @return Unique ID for the user who registered the Fibaro Device.
*/
public int getUserId() {
return userId;
}
/**
* Sets the value of the 'userId' field.
* Unique ID for the user who registered the Fibaro Device.
* @param value the value to set.
*/
public void setUserId(int value) {
this.userId = value;
}
/**
* Gets the value of the 'name' field.
* @return Name of the Fibaro Device.
*/
public java.lang.String getName() {
return name;
}
/**
* Sets the value of the 'name' field.
* Name of the Fibaro Device.
* @param value the value to set.
*/
public void setName(java.lang.String value) {
this.name = value;
}
/**
* Gets the value of the 'roomId' field.
* @return Unique ID of the Room that the Fibaro Device is registered to.
*/
public int getRoomId() {
return roomId;
}
/**
* Sets the value of the 'roomId' field.
* Unique ID of the Room that the Fibaro Device is registered to.
* @param value the value to set.
*/
public void setRoomId(int value) {
this.roomId = value;
}
/**
* Gets the value of the 'enabled' field.
* @return True if the Fibaro Device is enabled, False if it is not.
*/
public boolean getEnabled() {
return enabled;
}
/**
* Sets the value of the 'enabled' field.
* True if the Fibaro Device is enabled, False if it is not.
* @param value the value to set.
*/
public void setEnabled(boolean value) {
this.enabled = value;
}
/**
* Gets the value of the 'parentId' field.
* @return Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
*/
public int getParentId() {
return parentId;
}
/**
* Sets the value of the 'parentId' field.
* Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
* @param value the value to set.
*/
public void setParentId(int value) {
this.parentId = value;
}
/**
* Gets the value of the 'categories' field.
* @return Show the categories in which the Device belongs to.
*/
public java.lang.String getCategories() {
return categories;
}
/**
* Sets the value of the 'categories' field.
* Show the categories in which the Device belongs to.
* @param value the value to set.
*/
public void setCategories(java.lang.String value) {
this.categories = value;
}
/**
* Gets the value of the 'configured' field.
* @return True if the device is configured.
*/
public boolean getConfigured() {
return configured;
}
/**
* Sets the value of the 'configured' field.
* True if the device is configured.
* @param value the value to set.
*/
public void setConfigured(boolean value) {
this.configured = value;
}
/**
* Gets the value of the 'dead' field.
* @return True if the device is not found on the network.
*/
public boolean getDead() {
return dead;
}
/**
* Sets the value of the 'dead' field.
* True if the device is not found on the network.
* @param value the value to set.
*/
public void setDead(boolean value) {
this.dead = value;
}
/**
* Gets the value of the 'deadReason' field.
* @return The reason that 'dead' field was set as True.
*/
public java.lang.String getDeadReason() {
return deadReason;
}
/**
* Sets the value of the 'deadReason' field.
* The reason that 'dead' field was set as True.
* @param value the value to set.
*/
public void setDeadReason(java.lang.String value) {
this.deadReason = value;
}
/**
* Gets the value of the 'created' field.
* @return Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
*/
public double getCreated() {
return created;
}
/**
* Sets the value of the 'created' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
* @param value the value to set.
*/
public void setCreated(double value) {
this.created = value;
}
/**
* Gets the value of the 'modified' field.
* @return Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
*/
public double getModified() {
return modified;
}
/**
* Sets the value of the 'modified' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
* @param value the value to set.
*/
public void setModified(double value) {
this.modified = value;
}
/**
* Creates a new FibaroDevice RecordBuilder.
* @return A new FibaroDevice RecordBuilder
*/
public static org.radarcns.passive.carl.FibaroDevice.Builder newBuilder() {
return new org.radarcns.passive.carl.FibaroDevice.Builder();
}
/**
* Creates a new FibaroDevice RecordBuilder by copying an existing Builder.
* @param other The existing builder to copy.
* @return A new FibaroDevice RecordBuilder
*/
public static org.radarcns.passive.carl.FibaroDevice.Builder newBuilder(org.radarcns.passive.carl.FibaroDevice.Builder other) {
if (other == null) {
return new org.radarcns.passive.carl.FibaroDevice.Builder();
} else {
return new org.radarcns.passive.carl.FibaroDevice.Builder(other);
}
}
/**
* Creates a new FibaroDevice RecordBuilder by copying an existing FibaroDevice instance.
* @param other The existing instance to copy.
* @return A new FibaroDevice RecordBuilder
*/
public static org.radarcns.passive.carl.FibaroDevice.Builder newBuilder(org.radarcns.passive.carl.FibaroDevice other) {
if (other == null) {
return new org.radarcns.passive.carl.FibaroDevice.Builder();
} else {
return new org.radarcns.passive.carl.FibaroDevice.Builder(other);
}
}
/**
* RecordBuilder for FibaroDevice instances.
*/
@org.apache.avro.specific.AvroGenerated
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
/** Time since the Unix Epoch (seconds), represents the time that an Event was registered. */
private double time;
/** Time since the Unix Epoch (seconds), represents the time this record was received. */
private double timeReceived;
/** A unique ID for a Fibaro Device. As registered in Carl Cloud. */
private int id;
/** Unique ID for a Fibaro Device. As registered in Home Center Lite's API. */
private int hclId;
/** Type of the device (Presense, Door, Flood, Panic, Wall Plug). */
private org.radarcns.passive.carl.DeviceType deviceType;
/** A serial number for a Fibaro Device. */
private java.lang.String serial;
/** The name of a Device's Manufacturer. */
private java.lang.String make;
/** The model name of the Fibaro Device. */
private java.lang.String model;
/** The level of Device's Battery. */
private double battery;
/** Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud. */
private double lastSyncTime;
/** The MAC Addresss of the Fibaro Device. */
private java.lang.String mac;
/** Unique ID for the user who registered the Fibaro Device. */
private int userId;
/** Name of the Fibaro Device. */
private java.lang.String name;
/** Unique ID of the Room that the Fibaro Device is registered to. */
private int roomId;
/** True if the Fibaro Device is enabled, False if it is not. */
private boolean enabled;
/** Unique ID of the Fibaro Device where this Fibaro Instance belongs to. */
private int parentId;
/** Show the categories in which the Device belongs to. */
private java.lang.String categories;
/** True if the device is configured. */
private boolean configured;
/** True if the device is not found on the network. */
private boolean dead;
/** The reason that 'dead' field was set as True. */
private java.lang.String deadReason;
/** Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync. */
private double created;
/** Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification. */
private double modified;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$, MODEL$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(org.radarcns.passive.carl.FibaroDevice.Builder other) {
super(other);
if (isValidValue(fields()[0], other.time)) {
this.time = data().deepCopy(fields()[0].schema(), other.time);
fieldSetFlags()[0] = other.fieldSetFlags()[0];
}
if (isValidValue(fields()[1], other.timeReceived)) {
this.timeReceived = data().deepCopy(fields()[1].schema(), other.timeReceived);
fieldSetFlags()[1] = other.fieldSetFlags()[1];
}
if (isValidValue(fields()[2], other.id)) {
this.id = data().deepCopy(fields()[2].schema(), other.id);
fieldSetFlags()[2] = other.fieldSetFlags()[2];
}
if (isValidValue(fields()[3], other.hclId)) {
this.hclId = data().deepCopy(fields()[3].schema(), other.hclId);
fieldSetFlags()[3] = other.fieldSetFlags()[3];
}
if (isValidValue(fields()[4], other.deviceType)) {
this.deviceType = data().deepCopy(fields()[4].schema(), other.deviceType);
fieldSetFlags()[4] = other.fieldSetFlags()[4];
}
if (isValidValue(fields()[5], other.serial)) {
this.serial = data().deepCopy(fields()[5].schema(), other.serial);
fieldSetFlags()[5] = other.fieldSetFlags()[5];
}
if (isValidValue(fields()[6], other.make)) {
this.make = data().deepCopy(fields()[6].schema(), other.make);
fieldSetFlags()[6] = other.fieldSetFlags()[6];
}
if (isValidValue(fields()[7], other.model)) {
this.model = data().deepCopy(fields()[7].schema(), other.model);
fieldSetFlags()[7] = other.fieldSetFlags()[7];
}
if (isValidValue(fields()[8], other.battery)) {
this.battery = data().deepCopy(fields()[8].schema(), other.battery);
fieldSetFlags()[8] = other.fieldSetFlags()[8];
}
if (isValidValue(fields()[9], other.lastSyncTime)) {
this.lastSyncTime = data().deepCopy(fields()[9].schema(), other.lastSyncTime);
fieldSetFlags()[9] = other.fieldSetFlags()[9];
}
if (isValidValue(fields()[10], other.mac)) {
this.mac = data().deepCopy(fields()[10].schema(), other.mac);
fieldSetFlags()[10] = other.fieldSetFlags()[10];
}
if (isValidValue(fields()[11], other.userId)) {
this.userId = data().deepCopy(fields()[11].schema(), other.userId);
fieldSetFlags()[11] = other.fieldSetFlags()[11];
}
if (isValidValue(fields()[12], other.name)) {
this.name = data().deepCopy(fields()[12].schema(), other.name);
fieldSetFlags()[12] = other.fieldSetFlags()[12];
}
if (isValidValue(fields()[13], other.roomId)) {
this.roomId = data().deepCopy(fields()[13].schema(), other.roomId);
fieldSetFlags()[13] = other.fieldSetFlags()[13];
}
if (isValidValue(fields()[14], other.enabled)) {
this.enabled = data().deepCopy(fields()[14].schema(), other.enabled);
fieldSetFlags()[14] = other.fieldSetFlags()[14];
}
if (isValidValue(fields()[15], other.parentId)) {
this.parentId = data().deepCopy(fields()[15].schema(), other.parentId);
fieldSetFlags()[15] = other.fieldSetFlags()[15];
}
if (isValidValue(fields()[16], other.categories)) {
this.categories = data().deepCopy(fields()[16].schema(), other.categories);
fieldSetFlags()[16] = other.fieldSetFlags()[16];
}
if (isValidValue(fields()[17], other.configured)) {
this.configured = data().deepCopy(fields()[17].schema(), other.configured);
fieldSetFlags()[17] = other.fieldSetFlags()[17];
}
if (isValidValue(fields()[18], other.dead)) {
this.dead = data().deepCopy(fields()[18].schema(), other.dead);
fieldSetFlags()[18] = other.fieldSetFlags()[18];
}
if (isValidValue(fields()[19], other.deadReason)) {
this.deadReason = data().deepCopy(fields()[19].schema(), other.deadReason);
fieldSetFlags()[19] = other.fieldSetFlags()[19];
}
if (isValidValue(fields()[20], other.created)) {
this.created = data().deepCopy(fields()[20].schema(), other.created);
fieldSetFlags()[20] = other.fieldSetFlags()[20];
}
if (isValidValue(fields()[21], other.modified)) {
this.modified = data().deepCopy(fields()[21].schema(), other.modified);
fieldSetFlags()[21] = other.fieldSetFlags()[21];
}
}
/**
* Creates a Builder by copying an existing FibaroDevice instance
* @param other The existing instance to copy.
*/
private Builder(org.radarcns.passive.carl.FibaroDevice other) {
super(SCHEMA$, MODEL$);
if (isValidValue(fields()[0], other.time)) {
this.time = data().deepCopy(fields()[0].schema(), other.time);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.timeReceived)) {
this.timeReceived = data().deepCopy(fields()[1].schema(), other.timeReceived);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.id)) {
this.id = data().deepCopy(fields()[2].schema(), other.id);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.hclId)) {
this.hclId = data().deepCopy(fields()[3].schema(), other.hclId);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.deviceType)) {
this.deviceType = data().deepCopy(fields()[4].schema(), other.deviceType);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.serial)) {
this.serial = data().deepCopy(fields()[5].schema(), other.serial);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.make)) {
this.make = data().deepCopy(fields()[6].schema(), other.make);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.model)) {
this.model = data().deepCopy(fields()[7].schema(), other.model);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.battery)) {
this.battery = data().deepCopy(fields()[8].schema(), other.battery);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.lastSyncTime)) {
this.lastSyncTime = data().deepCopy(fields()[9].schema(), other.lastSyncTime);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.mac)) {
this.mac = data().deepCopy(fields()[10].schema(), other.mac);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.userId)) {
this.userId = data().deepCopy(fields()[11].schema(), other.userId);
fieldSetFlags()[11] = true;
}
if (isValidValue(fields()[12], other.name)) {
this.name = data().deepCopy(fields()[12].schema(), other.name);
fieldSetFlags()[12] = true;
}
if (isValidValue(fields()[13], other.roomId)) {
this.roomId = data().deepCopy(fields()[13].schema(), other.roomId);
fieldSetFlags()[13] = true;
}
if (isValidValue(fields()[14], other.enabled)) {
this.enabled = data().deepCopy(fields()[14].schema(), other.enabled);
fieldSetFlags()[14] = true;
}
if (isValidValue(fields()[15], other.parentId)) {
this.parentId = data().deepCopy(fields()[15].schema(), other.parentId);
fieldSetFlags()[15] = true;
}
if (isValidValue(fields()[16], other.categories)) {
this.categories = data().deepCopy(fields()[16].schema(), other.categories);
fieldSetFlags()[16] = true;
}
if (isValidValue(fields()[17], other.configured)) {
this.configured = data().deepCopy(fields()[17].schema(), other.configured);
fieldSetFlags()[17] = true;
}
if (isValidValue(fields()[18], other.dead)) {
this.dead = data().deepCopy(fields()[18].schema(), other.dead);
fieldSetFlags()[18] = true;
}
if (isValidValue(fields()[19], other.deadReason)) {
this.deadReason = data().deepCopy(fields()[19].schema(), other.deadReason);
fieldSetFlags()[19] = true;
}
if (isValidValue(fields()[20], other.created)) {
this.created = data().deepCopy(fields()[20].schema(), other.created);
fieldSetFlags()[20] = true;
}
if (isValidValue(fields()[21], other.modified)) {
this.modified = data().deepCopy(fields()[21].schema(), other.modified);
fieldSetFlags()[21] = true;
}
}
/**
* Gets the value of the 'time' field.
* Time since the Unix Epoch (seconds), represents the time that an Event was registered.
* @return The value.
*/
public double getTime() {
return time;
}
/**
* Sets the value of the 'time' field.
* Time since the Unix Epoch (seconds), represents the time that an Event was registered.
* @param value The value of 'time'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setTime(double value) {
validate(fields()[0], value);
this.time = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'time' field has been set.
* Time since the Unix Epoch (seconds), represents the time that an Event was registered.
* @return True if the 'time' field has been set, false otherwise.
*/
public boolean hasTime() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'time' field.
* Time since the Unix Epoch (seconds), represents the time that an Event was registered.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearTime() {
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'timeReceived' field.
* Time since the Unix Epoch (seconds), represents the time this record was received.
* @return The value.
*/
public double getTimeReceived() {
return timeReceived;
}
/**
* Sets the value of the 'timeReceived' field.
* Time since the Unix Epoch (seconds), represents the time this record was received.
* @param value The value of 'timeReceived'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setTimeReceived(double value) {
validate(fields()[1], value);
this.timeReceived = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'timeReceived' field has been set.
* Time since the Unix Epoch (seconds), represents the time this record was received.
* @return True if the 'timeReceived' field has been set, false otherwise.
*/
public boolean hasTimeReceived() {
return fieldSetFlags()[1];
}
/**
* Clears the value of the 'timeReceived' field.
* Time since the Unix Epoch (seconds), represents the time this record was received.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearTimeReceived() {
fieldSetFlags()[1] = false;
return this;
}
/**
* Gets the value of the 'id' field.
* A unique ID for a Fibaro Device. As registered in Carl Cloud.
* @return The value.
*/
public int getId() {
return id;
}
/**
* Sets the value of the 'id' field.
* A unique ID for a Fibaro Device. As registered in Carl Cloud.
* @param value The value of 'id'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setId(int value) {
validate(fields()[2], value);
this.id = value;
fieldSetFlags()[2] = true;
return this;
}
/**
* Checks whether the 'id' field has been set.
* A unique ID for a Fibaro Device. As registered in Carl Cloud.
* @return True if the 'id' field has been set, false otherwise.
*/
public boolean hasId() {
return fieldSetFlags()[2];
}
/**
* Clears the value of the 'id' field.
* A unique ID for a Fibaro Device. As registered in Carl Cloud.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearId() {
fieldSetFlags()[2] = false;
return this;
}
/**
* Gets the value of the 'hclId' field.
* Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
* @return The value.
*/
public int getHclId() {
return hclId;
}
/**
* Sets the value of the 'hclId' field.
* Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
* @param value The value of 'hclId'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setHclId(int value) {
validate(fields()[3], value);
this.hclId = value;
fieldSetFlags()[3] = true;
return this;
}
/**
* Checks whether the 'hclId' field has been set.
* Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
* @return True if the 'hclId' field has been set, false otherwise.
*/
public boolean hasHclId() {
return fieldSetFlags()[3];
}
/**
* Clears the value of the 'hclId' field.
* Unique ID for a Fibaro Device. As registered in Home Center Lite's API.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearHclId() {
fieldSetFlags()[3] = false;
return this;
}
/**
* Gets the value of the 'deviceType' field.
* Type of the device (Presense, Door, Flood, Panic, Wall Plug).
* @return The value.
*/
public org.radarcns.passive.carl.DeviceType getDeviceType() {
return deviceType;
}
/**
* Sets the value of the 'deviceType' field.
* Type of the device (Presense, Door, Flood, Panic, Wall Plug).
* @param value The value of 'deviceType'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setDeviceType(org.radarcns.passive.carl.DeviceType value) {
validate(fields()[4], value);
this.deviceType = value;
fieldSetFlags()[4] = true;
return this;
}
/**
* Checks whether the 'deviceType' field has been set.
* Type of the device (Presense, Door, Flood, Panic, Wall Plug).
* @return True if the 'deviceType' field has been set, false otherwise.
*/
public boolean hasDeviceType() {
return fieldSetFlags()[4];
}
/**
* Clears the value of the 'deviceType' field.
* Type of the device (Presense, Door, Flood, Panic, Wall Plug).
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearDeviceType() {
deviceType = null;
fieldSetFlags()[4] = false;
return this;
}
/**
* Gets the value of the 'serial' field.
* A serial number for a Fibaro Device.
* @return The value.
*/
public java.lang.String getSerial() {
return serial;
}
/**
* Sets the value of the 'serial' field.
* A serial number for a Fibaro Device.
* @param value The value of 'serial'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setSerial(java.lang.String value) {
validate(fields()[5], value);
this.serial = value;
fieldSetFlags()[5] = true;
return this;
}
/**
* Checks whether the 'serial' field has been set.
* A serial number for a Fibaro Device.
* @return True if the 'serial' field has been set, false otherwise.
*/
public boolean hasSerial() {
return fieldSetFlags()[5];
}
/**
* Clears the value of the 'serial' field.
* A serial number for a Fibaro Device.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearSerial() {
serial = null;
fieldSetFlags()[5] = false;
return this;
}
/**
* Gets the value of the 'make' field.
* The name of a Device's Manufacturer.
* @return The value.
*/
public java.lang.String getMake() {
return make;
}
/**
* Sets the value of the 'make' field.
* The name of a Device's Manufacturer.
* @param value The value of 'make'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setMake(java.lang.String value) {
validate(fields()[6], value);
this.make = value;
fieldSetFlags()[6] = true;
return this;
}
/**
* Checks whether the 'make' field has been set.
* The name of a Device's Manufacturer.
* @return True if the 'make' field has been set, false otherwise.
*/
public boolean hasMake() {
return fieldSetFlags()[6];
}
/**
* Clears the value of the 'make' field.
* The name of a Device's Manufacturer.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearMake() {
make = null;
fieldSetFlags()[6] = false;
return this;
}
/**
* Gets the value of the 'model' field.
* The model name of the Fibaro Device.
* @return The value.
*/
public java.lang.String getModel() {
return model;
}
/**
* Sets the value of the 'model' field.
* The model name of the Fibaro Device.
* @param value The value of 'model'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setModel(java.lang.String value) {
validate(fields()[7], value);
this.model = value;
fieldSetFlags()[7] = true;
return this;
}
/**
* Checks whether the 'model' field has been set.
* The model name of the Fibaro Device.
* @return True if the 'model' field has been set, false otherwise.
*/
public boolean hasModel() {
return fieldSetFlags()[7];
}
/**
* Clears the value of the 'model' field.
* The model name of the Fibaro Device.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearModel() {
model = null;
fieldSetFlags()[7] = false;
return this;
}
/**
* Gets the value of the 'battery' field.
* The level of Device's Battery.
* @return The value.
*/
public double getBattery() {
return battery;
}
/**
* Sets the value of the 'battery' field.
* The level of Device's Battery.
* @param value The value of 'battery'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setBattery(double value) {
validate(fields()[8], value);
this.battery = value;
fieldSetFlags()[8] = true;
return this;
}
/**
* Checks whether the 'battery' field has been set.
* The level of Device's Battery.
* @return True if the 'battery' field has been set, false otherwise.
*/
public boolean hasBattery() {
return fieldSetFlags()[8];
}
/**
* Clears the value of the 'battery' field.
* The level of Device's Battery.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearBattery() {
fieldSetFlags()[8] = false;
return this;
}
/**
* Gets the value of the 'lastSyncTime' field.
* Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
* @return The value.
*/
public double getLastSyncTime() {
return lastSyncTime;
}
/**
* Sets the value of the 'lastSyncTime' field.
* Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
* @param value The value of 'lastSyncTime'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setLastSyncTime(double value) {
validate(fields()[9], value);
this.lastSyncTime = value;
fieldSetFlags()[9] = true;
return this;
}
/**
* Checks whether the 'lastSyncTime' field has been set.
* Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
* @return True if the 'lastSyncTime' field has been set, false otherwise.
*/
public boolean hasLastSyncTime() {
return fieldSetFlags()[9];
}
/**
* Clears the value of the 'lastSyncTime' field.
* Time since the Unix Epoch (seconds), represent the time of the Fibaro Device's last sync with CARL Cloud.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearLastSyncTime() {
fieldSetFlags()[9] = false;
return this;
}
/**
* Gets the value of the 'mac' field.
* The MAC Addresss of the Fibaro Device.
* @return The value.
*/
public java.lang.String getMac() {
return mac;
}
/**
* Sets the value of the 'mac' field.
* The MAC Addresss of the Fibaro Device.
* @param value The value of 'mac'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setMac(java.lang.String value) {
validate(fields()[10], value);
this.mac = value;
fieldSetFlags()[10] = true;
return this;
}
/**
* Checks whether the 'mac' field has been set.
* The MAC Addresss of the Fibaro Device.
* @return True if the 'mac' field has been set, false otherwise.
*/
public boolean hasMac() {
return fieldSetFlags()[10];
}
/**
* Clears the value of the 'mac' field.
* The MAC Addresss of the Fibaro Device.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearMac() {
mac = null;
fieldSetFlags()[10] = false;
return this;
}
/**
* Gets the value of the 'userId' field.
* Unique ID for the user who registered the Fibaro Device.
* @return The value.
*/
public int getUserId() {
return userId;
}
/**
* Sets the value of the 'userId' field.
* Unique ID for the user who registered the Fibaro Device.
* @param value The value of 'userId'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setUserId(int value) {
validate(fields()[11], value);
this.userId = value;
fieldSetFlags()[11] = true;
return this;
}
/**
* Checks whether the 'userId' field has been set.
* Unique ID for the user who registered the Fibaro Device.
* @return True if the 'userId' field has been set, false otherwise.
*/
public boolean hasUserId() {
return fieldSetFlags()[11];
}
/**
* Clears the value of the 'userId' field.
* Unique ID for the user who registered the Fibaro Device.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearUserId() {
fieldSetFlags()[11] = false;
return this;
}
/**
* Gets the value of the 'name' field.
* Name of the Fibaro Device.
* @return The value.
*/
public java.lang.String getName() {
return name;
}
/**
* Sets the value of the 'name' field.
* Name of the Fibaro Device.
* @param value The value of 'name'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setName(java.lang.String value) {
validate(fields()[12], value);
this.name = value;
fieldSetFlags()[12] = true;
return this;
}
/**
* Checks whether the 'name' field has been set.
* Name of the Fibaro Device.
* @return True if the 'name' field has been set, false otherwise.
*/
public boolean hasName() {
return fieldSetFlags()[12];
}
/**
* Clears the value of the 'name' field.
* Name of the Fibaro Device.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearName() {
name = null;
fieldSetFlags()[12] = false;
return this;
}
/**
* Gets the value of the 'roomId' field.
* Unique ID of the Room that the Fibaro Device is registered to.
* @return The value.
*/
public int getRoomId() {
return roomId;
}
/**
* Sets the value of the 'roomId' field.
* Unique ID of the Room that the Fibaro Device is registered to.
* @param value The value of 'roomId'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setRoomId(int value) {
validate(fields()[13], value);
this.roomId = value;
fieldSetFlags()[13] = true;
return this;
}
/**
* Checks whether the 'roomId' field has been set.
* Unique ID of the Room that the Fibaro Device is registered to.
* @return True if the 'roomId' field has been set, false otherwise.
*/
public boolean hasRoomId() {
return fieldSetFlags()[13];
}
/**
* Clears the value of the 'roomId' field.
* Unique ID of the Room that the Fibaro Device is registered to.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearRoomId() {
fieldSetFlags()[13] = false;
return this;
}
/**
* Gets the value of the 'enabled' field.
* True if the Fibaro Device is enabled, False if it is not.
* @return The value.
*/
public boolean getEnabled() {
return enabled;
}
/**
* Sets the value of the 'enabled' field.
* True if the Fibaro Device is enabled, False if it is not.
* @param value The value of 'enabled'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setEnabled(boolean value) {
validate(fields()[14], value);
this.enabled = value;
fieldSetFlags()[14] = true;
return this;
}
/**
* Checks whether the 'enabled' field has been set.
* True if the Fibaro Device is enabled, False if it is not.
* @return True if the 'enabled' field has been set, false otherwise.
*/
public boolean hasEnabled() {
return fieldSetFlags()[14];
}
/**
* Clears the value of the 'enabled' field.
* True if the Fibaro Device is enabled, False if it is not.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearEnabled() {
fieldSetFlags()[14] = false;
return this;
}
/**
* Gets the value of the 'parentId' field.
* Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
* @return The value.
*/
public int getParentId() {
return parentId;
}
/**
* Sets the value of the 'parentId' field.
* Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
* @param value The value of 'parentId'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setParentId(int value) {
validate(fields()[15], value);
this.parentId = value;
fieldSetFlags()[15] = true;
return this;
}
/**
* Checks whether the 'parentId' field has been set.
* Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
* @return True if the 'parentId' field has been set, false otherwise.
*/
public boolean hasParentId() {
return fieldSetFlags()[15];
}
/**
* Clears the value of the 'parentId' field.
* Unique ID of the Fibaro Device where this Fibaro Instance belongs to.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearParentId() {
fieldSetFlags()[15] = false;
return this;
}
/**
* Gets the value of the 'categories' field.
* Show the categories in which the Device belongs to.
* @return The value.
*/
public java.lang.String getCategories() {
return categories;
}
/**
* Sets the value of the 'categories' field.
* Show the categories in which the Device belongs to.
* @param value The value of 'categories'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setCategories(java.lang.String value) {
validate(fields()[16], value);
this.categories = value;
fieldSetFlags()[16] = true;
return this;
}
/**
* Checks whether the 'categories' field has been set.
* Show the categories in which the Device belongs to.
* @return True if the 'categories' field has been set, false otherwise.
*/
public boolean hasCategories() {
return fieldSetFlags()[16];
}
/**
* Clears the value of the 'categories' field.
* Show the categories in which the Device belongs to.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearCategories() {
categories = null;
fieldSetFlags()[16] = false;
return this;
}
/**
* Gets the value of the 'configured' field.
* True if the device is configured.
* @return The value.
*/
public boolean getConfigured() {
return configured;
}
/**
* Sets the value of the 'configured' field.
* True if the device is configured.
* @param value The value of 'configured'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setConfigured(boolean value) {
validate(fields()[17], value);
this.configured = value;
fieldSetFlags()[17] = true;
return this;
}
/**
* Checks whether the 'configured' field has been set.
* True if the device is configured.
* @return True if the 'configured' field has been set, false otherwise.
*/
public boolean hasConfigured() {
return fieldSetFlags()[17];
}
/**
* Clears the value of the 'configured' field.
* True if the device is configured.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearConfigured() {
fieldSetFlags()[17] = false;
return this;
}
/**
* Gets the value of the 'dead' field.
* True if the device is not found on the network.
* @return The value.
*/
public boolean getDead() {
return dead;
}
/**
* Sets the value of the 'dead' field.
* True if the device is not found on the network.
* @param value The value of 'dead'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setDead(boolean value) {
validate(fields()[18], value);
this.dead = value;
fieldSetFlags()[18] = true;
return this;
}
/**
* Checks whether the 'dead' field has been set.
* True if the device is not found on the network.
* @return True if the 'dead' field has been set, false otherwise.
*/
public boolean hasDead() {
return fieldSetFlags()[18];
}
/**
* Clears the value of the 'dead' field.
* True if the device is not found on the network.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearDead() {
fieldSetFlags()[18] = false;
return this;
}
/**
* Gets the value of the 'deadReason' field.
* The reason that 'dead' field was set as True.
* @return The value.
*/
public java.lang.String getDeadReason() {
return deadReason;
}
/**
* Sets the value of the 'deadReason' field.
* The reason that 'dead' field was set as True.
* @param value The value of 'deadReason'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setDeadReason(java.lang.String value) {
validate(fields()[19], value);
this.deadReason = value;
fieldSetFlags()[19] = true;
return this;
}
/**
* Checks whether the 'deadReason' field has been set.
* The reason that 'dead' field was set as True.
* @return True if the 'deadReason' field has been set, false otherwise.
*/
public boolean hasDeadReason() {
return fieldSetFlags()[19];
}
/**
* Clears the value of the 'deadReason' field.
* The reason that 'dead' field was set as True.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearDeadReason() {
deadReason = null;
fieldSetFlags()[19] = false;
return this;
}
/**
* Gets the value of the 'created' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
* @return The value.
*/
public double getCreated() {
return created;
}
/**
* Sets the value of the 'created' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
* @param value The value of 'created'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setCreated(double value) {
validate(fields()[20], value);
this.created = value;
fieldSetFlags()[20] = true;
return this;
}
/**
* Checks whether the 'created' field has been set.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
* @return True if the 'created' field has been set, false otherwise.
*/
public boolean hasCreated() {
return fieldSetFlags()[20];
}
/**
* Clears the value of the 'created' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's first sync.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearCreated() {
fieldSetFlags()[20] = false;
return this;
}
/**
* Gets the value of the 'modified' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
* @return The value.
*/
public double getModified() {
return modified;
}
/**
* Sets the value of the 'modified' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
* @param value The value of 'modified'.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder setModified(double value) {
validate(fields()[21], value);
this.modified = value;
fieldSetFlags()[21] = true;
return this;
}
/**
* Checks whether the 'modified' field has been set.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
* @return True if the 'modified' field has been set, false otherwise.
*/
public boolean hasModified() {
return fieldSetFlags()[21];
}
/**
* Clears the value of the 'modified' field.
* Time since the Unix Epoch (seconds), represents the time of the Fibaro Device's last modification.
* @return This builder.
*/
public org.radarcns.passive.carl.FibaroDevice.Builder clearModified() {
fieldSetFlags()[21] = false;
return this;
}
@Override
@SuppressWarnings("unchecked")
public FibaroDevice build() {
try {
FibaroDevice record = new FibaroDevice();
record.time = fieldSetFlags()[0] ? this.time : (java.lang.Double) defaultValue(fields()[0]);
record.timeReceived = fieldSetFlags()[1] ? this.timeReceived : (java.lang.Double) defaultValue(fields()[1]);
record.id = fieldSetFlags()[2] ? this.id : (java.lang.Integer) defaultValue(fields()[2]);
record.hclId = fieldSetFlags()[3] ? this.hclId : (java.lang.Integer) defaultValue(fields()[3]);
record.deviceType = fieldSetFlags()[4] ? this.deviceType : (org.radarcns.passive.carl.DeviceType) defaultValue(fields()[4]);
record.serial = fieldSetFlags()[5] ? this.serial : (java.lang.String) defaultValue(fields()[5]);
record.make = fieldSetFlags()[6] ? this.make : (java.lang.String) defaultValue(fields()[6]);
record.model = fieldSetFlags()[7] ? this.model : (java.lang.String) defaultValue(fields()[7]);
record.battery = fieldSetFlags()[8] ? this.battery : (java.lang.Double) defaultValue(fields()[8]);
record.lastSyncTime = fieldSetFlags()[9] ? this.lastSyncTime : (java.lang.Double) defaultValue(fields()[9]);
record.mac = fieldSetFlags()[10] ? this.mac : (java.lang.String) defaultValue(fields()[10]);
record.userId = fieldSetFlags()[11] ? this.userId : (java.lang.Integer) defaultValue(fields()[11]);
record.name = fieldSetFlags()[12] ? this.name : (java.lang.String) defaultValue(fields()[12]);
record.roomId = fieldSetFlags()[13] ? this.roomId : (java.lang.Integer) defaultValue(fields()[13]);
record.enabled = fieldSetFlags()[14] ? this.enabled : (java.lang.Boolean) defaultValue(fields()[14]);
record.parentId = fieldSetFlags()[15] ? this.parentId : (java.lang.Integer) defaultValue(fields()[15]);
record.categories = fieldSetFlags()[16] ? this.categories : (java.lang.String) defaultValue(fields()[16]);
record.configured = fieldSetFlags()[17] ? this.configured : (java.lang.Boolean) defaultValue(fields()[17]);
record.dead = fieldSetFlags()[18] ? this.dead : (java.lang.Boolean) defaultValue(fields()[18]);
record.deadReason = fieldSetFlags()[19] ? this.deadReason : (java.lang.String) defaultValue(fields()[19]);
record.created = fieldSetFlags()[20] ? this.created : (java.lang.Double) defaultValue(fields()[20]);
record.modified = fieldSetFlags()[21] ? this.modified : (java.lang.Double) defaultValue(fields()[21]);
return record;
} catch (org.apache.avro.AvroMissingFieldException e) {
throw e;
} catch (java.lang.Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumWriter
WRITER$ = (org.apache.avro.io.DatumWriter)MODEL$.createDatumWriter(SCHEMA$);
@Override public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumReader
READER$ = (org.apache.avro.io.DatumReader)MODEL$.createDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
@Override protected boolean hasCustomCoders() { return true; }
@Override public void customEncode(org.apache.avro.io.Encoder out)
throws java.io.IOException
{
out.writeDouble(this.time);
out.writeDouble(this.timeReceived);
out.writeInt(this.id);
out.writeInt(this.hclId);
out.writeEnum(this.deviceType.ordinal());
out.writeString(this.serial);
if (this.make == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeString(this.make);
}
out.writeString(this.model);
out.writeDouble(this.battery);
out.writeDouble(this.lastSyncTime);
if (this.mac == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeString(this.mac);
}
out.writeInt(this.userId);
if (this.name == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeString(this.name);
}
out.writeInt(this.roomId);
out.writeBoolean(this.enabled);
out.writeInt(this.parentId);
out.writeString(this.categories);
out.writeBoolean(this.configured);
out.writeBoolean(this.dead);
if (this.deadReason == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeString(this.deadReason);
}
out.writeDouble(this.created);
out.writeDouble(this.modified);
}
@Override public void customDecode(org.apache.avro.io.ResolvingDecoder in)
throws java.io.IOException
{
org.apache.avro.Schema.Field[] fieldOrder = in.readFieldOrderIfDiff();
if (fieldOrder == null) {
this.time = in.readDouble();
this.timeReceived = in.readDouble();
this.id = in.readInt();
this.hclId = in.readInt();
this.deviceType = org.radarcns.passive.carl.DeviceType.values()[in.readEnum()];
this.serial = in.readString();
if (in.readIndex() != 1) {
in.readNull();
this.make = null;
} else {
this.make = in.readString();
}
this.model = in.readString();
this.battery = in.readDouble();
this.lastSyncTime = in.readDouble();
if (in.readIndex() != 1) {
in.readNull();
this.mac = null;
} else {
this.mac = in.readString();
}
this.userId = in.readInt();
if (in.readIndex() != 1) {
in.readNull();
this.name = null;
} else {
this.name = in.readString();
}
this.roomId = in.readInt();
this.enabled = in.readBoolean();
this.parentId = in.readInt();
this.categories = in.readString();
this.configured = in.readBoolean();
this.dead = in.readBoolean();
if (in.readIndex() != 1) {
in.readNull();
this.deadReason = null;
} else {
this.deadReason = in.readString();
}
this.created = in.readDouble();
this.modified = in.readDouble();
} else {
for (int i = 0; i < 22; i++) {
switch (fieldOrder[i].pos()) {
case 0:
this.time = in.readDouble();
break;
case 1:
this.timeReceived = in.readDouble();
break;
case 2:
this.id = in.readInt();
break;
case 3:
this.hclId = in.readInt();
break;
case 4:
this.deviceType = org.radarcns.passive.carl.DeviceType.values()[in.readEnum()];
break;
case 5:
this.serial = in.readString();
break;
case 6:
if (in.readIndex() != 1) {
in.readNull();
this.make = null;
} else {
this.make = in.readString();
}
break;
case 7:
this.model = in.readString();
break;
case 8:
this.battery = in.readDouble();
break;
case 9:
this.lastSyncTime = in.readDouble();
break;
case 10:
if (in.readIndex() != 1) {
in.readNull();
this.mac = null;
} else {
this.mac = in.readString();
}
break;
case 11:
this.userId = in.readInt();
break;
case 12:
if (in.readIndex() != 1) {
in.readNull();
this.name = null;
} else {
this.name = in.readString();
}
break;
case 13:
this.roomId = in.readInt();
break;
case 14:
this.enabled = in.readBoolean();
break;
case 15:
this.parentId = in.readInt();
break;
case 16:
this.categories = in.readString();
break;
case 17:
this.configured = in.readBoolean();
break;
case 18:
this.dead = in.readBoolean();
break;
case 19:
if (in.readIndex() != 1) {
in.readNull();
this.deadReason = null;
} else {
this.deadReason = in.readString();
}
break;
case 20:
this.created = in.readDouble();
break;
case 21:
this.modified = in.readDouble();
break;
default:
throw new java.io.IOException("Corrupt ResolvingDecoder.");
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy