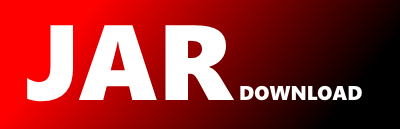
org.radarcns.passive.weather.LocalWeather Maven / Gradle / Ivy
The newest version!
/**
* Autogenerated by Avro
*
* DO NOT EDIT DIRECTLY
*/
package org.radarcns.passive.weather;
import org.apache.avro.generic.GenericArray;
import org.apache.avro.specific.SpecificData;
import org.apache.avro.util.Utf8;
import org.apache.avro.message.BinaryMessageEncoder;
import org.apache.avro.message.BinaryMessageDecoder;
import org.apache.avro.message.SchemaStore;
/** Current weather information at the location of the user. */
@org.apache.avro.specific.AvroGenerated
public class LocalWeather extends org.apache.avro.specific.SpecificRecordBase implements org.apache.avro.specific.SpecificRecord {
private static final long serialVersionUID = -5220806470102923603L;
public static final org.apache.avro.Schema SCHEMA$ = new org.apache.avro.Schema.Parser().parse("{\"type\":\"record\",\"name\":\"LocalWeather\",\"namespace\":\"org.radarcns.passive.weather\",\"doc\":\"Current weather information at the location of the user.\",\"fields\":[{\"name\":\"time\",\"type\":\"double\",\"doc\":\"Device timestamp in UTC (s).\"},{\"name\":\"timeReceived\",\"type\":\"double\",\"doc\":\"Device receiver timestamp in UTC (s).\"},{\"name\":\"sunrise\",\"type\":[\"null\",\"int\"],\"doc\":\"Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.\",\"default\":null},{\"name\":\"sunset\",\"type\":[\"null\",\"int\"],\"doc\":\"Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.\",\"default\":null},{\"name\":\"temperature\",\"type\":[\"null\",\"float\"],\"doc\":\"Current ambient temperature (C).\",\"default\":null},{\"name\":\"pressure\",\"type\":[\"null\",\"float\"],\"doc\":\"Current atmospheric pressure on sea level (hPa).\",\"default\":null},{\"name\":\"humidity\",\"type\":[\"null\",\"float\"],\"doc\":\"Current humidity (%).\",\"default\":null},{\"name\":\"cloudiness\",\"type\":[\"null\",\"float\"],\"doc\":\"Current cloudiness (%).\",\"default\":null},{\"name\":\"precipitation\",\"type\":[\"null\",\"float\"],\"doc\":\"Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).\",\"default\":null},{\"name\":\"precipitationPeriod\",\"type\":[\"null\",\"int\"],\"doc\":\"Period over which the precipitation was determined (h).\",\"default\":null},{\"name\":\"condition\",\"type\":{\"type\":\"enum\",\"name\":\"WeatherCondition\",\"doc\":\"Types of weather condition.\",\"symbols\":[\"CLEAR\",\"CLOUDY\",\"DRIZZLE\",\"RAINY\",\"SNOWY\",\"ICY\",\"FOGGY\",\"STORM\",\"THUNDER\",\"OTHER\",\"UNKNOWN\"]},\"doc\":\"Current weather condition.\",\"default\":\"UNKNOWN\"},{\"name\":\"source\",\"type\":{\"type\":\"string\",\"avro.java.string\":\"String\"},\"doc\":\"From which source/API the weather data was retrieved.\"},{\"name\":\"locationSource\",\"type\":{\"type\":\"enum\",\"name\":\"LocationType\",\"doc\":\"How the location was determined.\",\"symbols\":[\"GPS\",\"NETWORK\",\"CITY_NAME\",\"OTHER\",\"UNKNOWN\"]},\"doc\":\"The source of the location used to get the weather details.\",\"default\":\"UNKNOWN\"}]}");
public static org.apache.avro.Schema getClassSchema() { return SCHEMA$; }
private static final SpecificData MODEL$ = new SpecificData();
private static final BinaryMessageEncoder ENCODER =
new BinaryMessageEncoder<>(MODEL$, SCHEMA$);
private static final BinaryMessageDecoder DECODER =
new BinaryMessageDecoder<>(MODEL$, SCHEMA$);
/**
* Return the BinaryMessageEncoder instance used by this class.
* @return the message encoder used by this class
*/
public static BinaryMessageEncoder getEncoder() {
return ENCODER;
}
/**
* Return the BinaryMessageDecoder instance used by this class.
* @return the message decoder used by this class
*/
public static BinaryMessageDecoder getDecoder() {
return DECODER;
}
/**
* Create a new BinaryMessageDecoder instance for this class that uses the specified {@link SchemaStore}.
* @param resolver a {@link SchemaStore} used to find schemas by fingerprint
* @return a BinaryMessageDecoder instance for this class backed by the given SchemaStore
*/
public static BinaryMessageDecoder createDecoder(SchemaStore resolver) {
return new BinaryMessageDecoder<>(MODEL$, SCHEMA$, resolver);
}
/**
* Serializes this LocalWeather to a ByteBuffer.
* @return a buffer holding the serialized data for this instance
* @throws java.io.IOException if this instance could not be serialized
*/
public java.nio.ByteBuffer toByteBuffer() throws java.io.IOException {
return ENCODER.encode(this);
}
/**
* Deserializes a LocalWeather from a ByteBuffer.
* @param b a byte buffer holding serialized data for an instance of this class
* @return a LocalWeather instance decoded from the given buffer
* @throws java.io.IOException if the given bytes could not be deserialized into an instance of this class
*/
public static LocalWeather fromByteBuffer(
java.nio.ByteBuffer b) throws java.io.IOException {
return DECODER.decode(b);
}
/** Device timestamp in UTC (s). */
private double time;
/** Device receiver timestamp in UTC (s). */
private double timeReceived;
/** Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight. */
private java.lang.Integer sunrise;
/** Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight. */
private java.lang.Integer sunset;
/** Current ambient temperature (C). */
private java.lang.Float temperature;
/** Current atmospheric pressure on sea level (hPa). */
private java.lang.Float pressure;
/** Current humidity (%). */
private java.lang.Float humidity;
/** Current cloudiness (%). */
private java.lang.Float cloudiness;
/** Rain or snow volume over last hours reported in 'percipitationPeriod' (mm). */
private java.lang.Float precipitation;
/** Period over which the precipitation was determined (h). */
private java.lang.Integer precipitationPeriod;
/** Current weather condition. */
private org.radarcns.passive.weather.WeatherCondition condition;
/** From which source/API the weather data was retrieved. */
private java.lang.String source;
/** The source of the location used to get the weather details. */
private org.radarcns.passive.weather.LocationType locationSource;
/**
* Default constructor. Note that this does not initialize fields
* to their default values from the schema. If that is desired then
* one should use newBuilder()
.
*/
public LocalWeather() {}
/**
* All-args constructor.
* @param time Device timestamp in UTC (s).
* @param timeReceived Device receiver timestamp in UTC (s).
* @param sunrise Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
* @param sunset Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
* @param temperature Current ambient temperature (C).
* @param pressure Current atmospheric pressure on sea level (hPa).
* @param humidity Current humidity (%).
* @param cloudiness Current cloudiness (%).
* @param precipitation Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
* @param precipitationPeriod Period over which the precipitation was determined (h).
* @param condition Current weather condition.
* @param source From which source/API the weather data was retrieved.
* @param locationSource The source of the location used to get the weather details.
*/
public LocalWeather(java.lang.Double time, java.lang.Double timeReceived, java.lang.Integer sunrise, java.lang.Integer sunset, java.lang.Float temperature, java.lang.Float pressure, java.lang.Float humidity, java.lang.Float cloudiness, java.lang.Float precipitation, java.lang.Integer precipitationPeriod, org.radarcns.passive.weather.WeatherCondition condition, java.lang.String source, org.radarcns.passive.weather.LocationType locationSource) {
this.time = time;
this.timeReceived = timeReceived;
this.sunrise = sunrise;
this.sunset = sunset;
this.temperature = temperature;
this.pressure = pressure;
this.humidity = humidity;
this.cloudiness = cloudiness;
this.precipitation = precipitation;
this.precipitationPeriod = precipitationPeriod;
this.condition = condition;
this.source = source;
this.locationSource = locationSource;
}
@Override
public org.apache.avro.specific.SpecificData getSpecificData() { return MODEL$; }
@Override
public org.apache.avro.Schema getSchema() { return SCHEMA$; }
// Used by DatumWriter. Applications should not call.
@Override
public java.lang.Object get(int field$) {
switch (field$) {
case 0: return time;
case 1: return timeReceived;
case 2: return sunrise;
case 3: return sunset;
case 4: return temperature;
case 5: return pressure;
case 6: return humidity;
case 7: return cloudiness;
case 8: return precipitation;
case 9: return precipitationPeriod;
case 10: return condition;
case 11: return source;
case 12: return locationSource;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
// Used by DatumReader. Applications should not call.
@Override
@SuppressWarnings(value="unchecked")
public void put(int field$, java.lang.Object value$) {
switch (field$) {
case 0: time = (java.lang.Double)value$; break;
case 1: timeReceived = (java.lang.Double)value$; break;
case 2: sunrise = (java.lang.Integer)value$; break;
case 3: sunset = (java.lang.Integer)value$; break;
case 4: temperature = (java.lang.Float)value$; break;
case 5: pressure = (java.lang.Float)value$; break;
case 6: humidity = (java.lang.Float)value$; break;
case 7: cloudiness = (java.lang.Float)value$; break;
case 8: precipitation = (java.lang.Float)value$; break;
case 9: precipitationPeriod = (java.lang.Integer)value$; break;
case 10: condition = (org.radarcns.passive.weather.WeatherCondition)value$; break;
case 11: source = value$ != null ? value$.toString() : null; break;
case 12: locationSource = (org.radarcns.passive.weather.LocationType)value$; break;
default: throw new IndexOutOfBoundsException("Invalid index: " + field$);
}
}
/**
* Gets the value of the 'time' field.
* @return Device timestamp in UTC (s).
*/
public double getTime() {
return time;
}
/**
* Sets the value of the 'time' field.
* Device timestamp in UTC (s).
* @param value the value to set.
*/
public void setTime(double value) {
this.time = value;
}
/**
* Gets the value of the 'timeReceived' field.
* @return Device receiver timestamp in UTC (s).
*/
public double getTimeReceived() {
return timeReceived;
}
/**
* Sets the value of the 'timeReceived' field.
* Device receiver timestamp in UTC (s).
* @param value the value to set.
*/
public void setTimeReceived(double value) {
this.timeReceived = value;
}
/**
* Gets the value of the 'sunrise' field.
* @return Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
*/
public java.lang.Integer getSunrise() {
return sunrise;
}
/**
* Sets the value of the 'sunrise' field.
* Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
* @param value the value to set.
*/
public void setSunrise(java.lang.Integer value) {
this.sunrise = value;
}
/**
* Gets the value of the 'sunset' field.
* @return Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
*/
public java.lang.Integer getSunset() {
return sunset;
}
/**
* Sets the value of the 'sunset' field.
* Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
* @param value the value to set.
*/
public void setSunset(java.lang.Integer value) {
this.sunset = value;
}
/**
* Gets the value of the 'temperature' field.
* @return Current ambient temperature (C).
*/
public java.lang.Float getTemperature() {
return temperature;
}
/**
* Sets the value of the 'temperature' field.
* Current ambient temperature (C).
* @param value the value to set.
*/
public void setTemperature(java.lang.Float value) {
this.temperature = value;
}
/**
* Gets the value of the 'pressure' field.
* @return Current atmospheric pressure on sea level (hPa).
*/
public java.lang.Float getPressure() {
return pressure;
}
/**
* Sets the value of the 'pressure' field.
* Current atmospheric pressure on sea level (hPa).
* @param value the value to set.
*/
public void setPressure(java.lang.Float value) {
this.pressure = value;
}
/**
* Gets the value of the 'humidity' field.
* @return Current humidity (%).
*/
public java.lang.Float getHumidity() {
return humidity;
}
/**
* Sets the value of the 'humidity' field.
* Current humidity (%).
* @param value the value to set.
*/
public void setHumidity(java.lang.Float value) {
this.humidity = value;
}
/**
* Gets the value of the 'cloudiness' field.
* @return Current cloudiness (%).
*/
public java.lang.Float getCloudiness() {
return cloudiness;
}
/**
* Sets the value of the 'cloudiness' field.
* Current cloudiness (%).
* @param value the value to set.
*/
public void setCloudiness(java.lang.Float value) {
this.cloudiness = value;
}
/**
* Gets the value of the 'precipitation' field.
* @return Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
*/
public java.lang.Float getPrecipitation() {
return precipitation;
}
/**
* Sets the value of the 'precipitation' field.
* Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
* @param value the value to set.
*/
public void setPrecipitation(java.lang.Float value) {
this.precipitation = value;
}
/**
* Gets the value of the 'precipitationPeriod' field.
* @return Period over which the precipitation was determined (h).
*/
public java.lang.Integer getPrecipitationPeriod() {
return precipitationPeriod;
}
/**
* Sets the value of the 'precipitationPeriod' field.
* Period over which the precipitation was determined (h).
* @param value the value to set.
*/
public void setPrecipitationPeriod(java.lang.Integer value) {
this.precipitationPeriod = value;
}
/**
* Gets the value of the 'condition' field.
* @return Current weather condition.
*/
public org.radarcns.passive.weather.WeatherCondition getCondition() {
return condition;
}
/**
* Sets the value of the 'condition' field.
* Current weather condition.
* @param value the value to set.
*/
public void setCondition(org.radarcns.passive.weather.WeatherCondition value) {
this.condition = value;
}
/**
* Gets the value of the 'source' field.
* @return From which source/API the weather data was retrieved.
*/
public java.lang.String getSource() {
return source;
}
/**
* Sets the value of the 'source' field.
* From which source/API the weather data was retrieved.
* @param value the value to set.
*/
public void setSource(java.lang.String value) {
this.source = value;
}
/**
* Gets the value of the 'locationSource' field.
* @return The source of the location used to get the weather details.
*/
public org.radarcns.passive.weather.LocationType getLocationSource() {
return locationSource;
}
/**
* Sets the value of the 'locationSource' field.
* The source of the location used to get the weather details.
* @param value the value to set.
*/
public void setLocationSource(org.radarcns.passive.weather.LocationType value) {
this.locationSource = value;
}
/**
* Creates a new LocalWeather RecordBuilder.
* @return A new LocalWeather RecordBuilder
*/
public static org.radarcns.passive.weather.LocalWeather.Builder newBuilder() {
return new org.radarcns.passive.weather.LocalWeather.Builder();
}
/**
* Creates a new LocalWeather RecordBuilder by copying an existing Builder.
* @param other The existing builder to copy.
* @return A new LocalWeather RecordBuilder
*/
public static org.radarcns.passive.weather.LocalWeather.Builder newBuilder(org.radarcns.passive.weather.LocalWeather.Builder other) {
if (other == null) {
return new org.radarcns.passive.weather.LocalWeather.Builder();
} else {
return new org.radarcns.passive.weather.LocalWeather.Builder(other);
}
}
/**
* Creates a new LocalWeather RecordBuilder by copying an existing LocalWeather instance.
* @param other The existing instance to copy.
* @return A new LocalWeather RecordBuilder
*/
public static org.radarcns.passive.weather.LocalWeather.Builder newBuilder(org.radarcns.passive.weather.LocalWeather other) {
if (other == null) {
return new org.radarcns.passive.weather.LocalWeather.Builder();
} else {
return new org.radarcns.passive.weather.LocalWeather.Builder(other);
}
}
/**
* RecordBuilder for LocalWeather instances.
*/
@org.apache.avro.specific.AvroGenerated
public static class Builder extends org.apache.avro.specific.SpecificRecordBuilderBase
implements org.apache.avro.data.RecordBuilder {
/** Device timestamp in UTC (s). */
private double time;
/** Device receiver timestamp in UTC (s). */
private double timeReceived;
/** Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight. */
private java.lang.Integer sunrise;
/** Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight. */
private java.lang.Integer sunset;
/** Current ambient temperature (C). */
private java.lang.Float temperature;
/** Current atmospheric pressure on sea level (hPa). */
private java.lang.Float pressure;
/** Current humidity (%). */
private java.lang.Float humidity;
/** Current cloudiness (%). */
private java.lang.Float cloudiness;
/** Rain or snow volume over last hours reported in 'percipitationPeriod' (mm). */
private java.lang.Float precipitation;
/** Period over which the precipitation was determined (h). */
private java.lang.Integer precipitationPeriod;
/** Current weather condition. */
private org.radarcns.passive.weather.WeatherCondition condition;
/** From which source/API the weather data was retrieved. */
private java.lang.String source;
/** The source of the location used to get the weather details. */
private org.radarcns.passive.weather.LocationType locationSource;
/** Creates a new Builder */
private Builder() {
super(SCHEMA$, MODEL$);
}
/**
* Creates a Builder by copying an existing Builder.
* @param other The existing Builder to copy.
*/
private Builder(org.radarcns.passive.weather.LocalWeather.Builder other) {
super(other);
if (isValidValue(fields()[0], other.time)) {
this.time = data().deepCopy(fields()[0].schema(), other.time);
fieldSetFlags()[0] = other.fieldSetFlags()[0];
}
if (isValidValue(fields()[1], other.timeReceived)) {
this.timeReceived = data().deepCopy(fields()[1].schema(), other.timeReceived);
fieldSetFlags()[1] = other.fieldSetFlags()[1];
}
if (isValidValue(fields()[2], other.sunrise)) {
this.sunrise = data().deepCopy(fields()[2].schema(), other.sunrise);
fieldSetFlags()[2] = other.fieldSetFlags()[2];
}
if (isValidValue(fields()[3], other.sunset)) {
this.sunset = data().deepCopy(fields()[3].schema(), other.sunset);
fieldSetFlags()[3] = other.fieldSetFlags()[3];
}
if (isValidValue(fields()[4], other.temperature)) {
this.temperature = data().deepCopy(fields()[4].schema(), other.temperature);
fieldSetFlags()[4] = other.fieldSetFlags()[4];
}
if (isValidValue(fields()[5], other.pressure)) {
this.pressure = data().deepCopy(fields()[5].schema(), other.pressure);
fieldSetFlags()[5] = other.fieldSetFlags()[5];
}
if (isValidValue(fields()[6], other.humidity)) {
this.humidity = data().deepCopy(fields()[6].schema(), other.humidity);
fieldSetFlags()[6] = other.fieldSetFlags()[6];
}
if (isValidValue(fields()[7], other.cloudiness)) {
this.cloudiness = data().deepCopy(fields()[7].schema(), other.cloudiness);
fieldSetFlags()[7] = other.fieldSetFlags()[7];
}
if (isValidValue(fields()[8], other.precipitation)) {
this.precipitation = data().deepCopy(fields()[8].schema(), other.precipitation);
fieldSetFlags()[8] = other.fieldSetFlags()[8];
}
if (isValidValue(fields()[9], other.precipitationPeriod)) {
this.precipitationPeriod = data().deepCopy(fields()[9].schema(), other.precipitationPeriod);
fieldSetFlags()[9] = other.fieldSetFlags()[9];
}
if (isValidValue(fields()[10], other.condition)) {
this.condition = data().deepCopy(fields()[10].schema(), other.condition);
fieldSetFlags()[10] = other.fieldSetFlags()[10];
}
if (isValidValue(fields()[11], other.source)) {
this.source = data().deepCopy(fields()[11].schema(), other.source);
fieldSetFlags()[11] = other.fieldSetFlags()[11];
}
if (isValidValue(fields()[12], other.locationSource)) {
this.locationSource = data().deepCopy(fields()[12].schema(), other.locationSource);
fieldSetFlags()[12] = other.fieldSetFlags()[12];
}
}
/**
* Creates a Builder by copying an existing LocalWeather instance
* @param other The existing instance to copy.
*/
private Builder(org.radarcns.passive.weather.LocalWeather other) {
super(SCHEMA$, MODEL$);
if (isValidValue(fields()[0], other.time)) {
this.time = data().deepCopy(fields()[0].schema(), other.time);
fieldSetFlags()[0] = true;
}
if (isValidValue(fields()[1], other.timeReceived)) {
this.timeReceived = data().deepCopy(fields()[1].schema(), other.timeReceived);
fieldSetFlags()[1] = true;
}
if (isValidValue(fields()[2], other.sunrise)) {
this.sunrise = data().deepCopy(fields()[2].schema(), other.sunrise);
fieldSetFlags()[2] = true;
}
if (isValidValue(fields()[3], other.sunset)) {
this.sunset = data().deepCopy(fields()[3].schema(), other.sunset);
fieldSetFlags()[3] = true;
}
if (isValidValue(fields()[4], other.temperature)) {
this.temperature = data().deepCopy(fields()[4].schema(), other.temperature);
fieldSetFlags()[4] = true;
}
if (isValidValue(fields()[5], other.pressure)) {
this.pressure = data().deepCopy(fields()[5].schema(), other.pressure);
fieldSetFlags()[5] = true;
}
if (isValidValue(fields()[6], other.humidity)) {
this.humidity = data().deepCopy(fields()[6].schema(), other.humidity);
fieldSetFlags()[6] = true;
}
if (isValidValue(fields()[7], other.cloudiness)) {
this.cloudiness = data().deepCopy(fields()[7].schema(), other.cloudiness);
fieldSetFlags()[7] = true;
}
if (isValidValue(fields()[8], other.precipitation)) {
this.precipitation = data().deepCopy(fields()[8].schema(), other.precipitation);
fieldSetFlags()[8] = true;
}
if (isValidValue(fields()[9], other.precipitationPeriod)) {
this.precipitationPeriod = data().deepCopy(fields()[9].schema(), other.precipitationPeriod);
fieldSetFlags()[9] = true;
}
if (isValidValue(fields()[10], other.condition)) {
this.condition = data().deepCopy(fields()[10].schema(), other.condition);
fieldSetFlags()[10] = true;
}
if (isValidValue(fields()[11], other.source)) {
this.source = data().deepCopy(fields()[11].schema(), other.source);
fieldSetFlags()[11] = true;
}
if (isValidValue(fields()[12], other.locationSource)) {
this.locationSource = data().deepCopy(fields()[12].schema(), other.locationSource);
fieldSetFlags()[12] = true;
}
}
/**
* Gets the value of the 'time' field.
* Device timestamp in UTC (s).
* @return The value.
*/
public double getTime() {
return time;
}
/**
* Sets the value of the 'time' field.
* Device timestamp in UTC (s).
* @param value The value of 'time'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setTime(double value) {
validate(fields()[0], value);
this.time = value;
fieldSetFlags()[0] = true;
return this;
}
/**
* Checks whether the 'time' field has been set.
* Device timestamp in UTC (s).
* @return True if the 'time' field has been set, false otherwise.
*/
public boolean hasTime() {
return fieldSetFlags()[0];
}
/**
* Clears the value of the 'time' field.
* Device timestamp in UTC (s).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearTime() {
fieldSetFlags()[0] = false;
return this;
}
/**
* Gets the value of the 'timeReceived' field.
* Device receiver timestamp in UTC (s).
* @return The value.
*/
public double getTimeReceived() {
return timeReceived;
}
/**
* Sets the value of the 'timeReceived' field.
* Device receiver timestamp in UTC (s).
* @param value The value of 'timeReceived'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setTimeReceived(double value) {
validate(fields()[1], value);
this.timeReceived = value;
fieldSetFlags()[1] = true;
return this;
}
/**
* Checks whether the 'timeReceived' field has been set.
* Device receiver timestamp in UTC (s).
* @return True if the 'timeReceived' field has been set, false otherwise.
*/
public boolean hasTimeReceived() {
return fieldSetFlags()[1];
}
/**
* Clears the value of the 'timeReceived' field.
* Device receiver timestamp in UTC (s).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearTimeReceived() {
fieldSetFlags()[1] = false;
return this;
}
/**
* Gets the value of the 'sunrise' field.
* Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
* @return The value.
*/
public java.lang.Integer getSunrise() {
return sunrise;
}
/**
* Sets the value of the 'sunrise' field.
* Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
* @param value The value of 'sunrise'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setSunrise(java.lang.Integer value) {
validate(fields()[2], value);
this.sunrise = value;
fieldSetFlags()[2] = true;
return this;
}
/**
* Checks whether the 'sunrise' field has been set.
* Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
* @return True if the 'sunrise' field has been set, false otherwise.
*/
public boolean hasSunrise() {
return fieldSetFlags()[2];
}
/**
* Clears the value of the 'sunrise' field.
* Sunrise time of day in minutes after midnight. The difference with sunset is the minutes of daylight.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearSunrise() {
sunrise = null;
fieldSetFlags()[2] = false;
return this;
}
/**
* Gets the value of the 'sunset' field.
* Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
* @return The value.
*/
public java.lang.Integer getSunset() {
return sunset;
}
/**
* Sets the value of the 'sunset' field.
* Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
* @param value The value of 'sunset'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setSunset(java.lang.Integer value) {
validate(fields()[3], value);
this.sunset = value;
fieldSetFlags()[3] = true;
return this;
}
/**
* Checks whether the 'sunset' field has been set.
* Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
* @return True if the 'sunset' field has been set, false otherwise.
*/
public boolean hasSunset() {
return fieldSetFlags()[3];
}
/**
* Clears the value of the 'sunset' field.
* Sunset time of day in minutes after midnight. The difference with sunrise is the minutes of daylight.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearSunset() {
sunset = null;
fieldSetFlags()[3] = false;
return this;
}
/**
* Gets the value of the 'temperature' field.
* Current ambient temperature (C).
* @return The value.
*/
public java.lang.Float getTemperature() {
return temperature;
}
/**
* Sets the value of the 'temperature' field.
* Current ambient temperature (C).
* @param value The value of 'temperature'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setTemperature(java.lang.Float value) {
validate(fields()[4], value);
this.temperature = value;
fieldSetFlags()[4] = true;
return this;
}
/**
* Checks whether the 'temperature' field has been set.
* Current ambient temperature (C).
* @return True if the 'temperature' field has been set, false otherwise.
*/
public boolean hasTemperature() {
return fieldSetFlags()[4];
}
/**
* Clears the value of the 'temperature' field.
* Current ambient temperature (C).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearTemperature() {
temperature = null;
fieldSetFlags()[4] = false;
return this;
}
/**
* Gets the value of the 'pressure' field.
* Current atmospheric pressure on sea level (hPa).
* @return The value.
*/
public java.lang.Float getPressure() {
return pressure;
}
/**
* Sets the value of the 'pressure' field.
* Current atmospheric pressure on sea level (hPa).
* @param value The value of 'pressure'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setPressure(java.lang.Float value) {
validate(fields()[5], value);
this.pressure = value;
fieldSetFlags()[5] = true;
return this;
}
/**
* Checks whether the 'pressure' field has been set.
* Current atmospheric pressure on sea level (hPa).
* @return True if the 'pressure' field has been set, false otherwise.
*/
public boolean hasPressure() {
return fieldSetFlags()[5];
}
/**
* Clears the value of the 'pressure' field.
* Current atmospheric pressure on sea level (hPa).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearPressure() {
pressure = null;
fieldSetFlags()[5] = false;
return this;
}
/**
* Gets the value of the 'humidity' field.
* Current humidity (%).
* @return The value.
*/
public java.lang.Float getHumidity() {
return humidity;
}
/**
* Sets the value of the 'humidity' field.
* Current humidity (%).
* @param value The value of 'humidity'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setHumidity(java.lang.Float value) {
validate(fields()[6], value);
this.humidity = value;
fieldSetFlags()[6] = true;
return this;
}
/**
* Checks whether the 'humidity' field has been set.
* Current humidity (%).
* @return True if the 'humidity' field has been set, false otherwise.
*/
public boolean hasHumidity() {
return fieldSetFlags()[6];
}
/**
* Clears the value of the 'humidity' field.
* Current humidity (%).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearHumidity() {
humidity = null;
fieldSetFlags()[6] = false;
return this;
}
/**
* Gets the value of the 'cloudiness' field.
* Current cloudiness (%).
* @return The value.
*/
public java.lang.Float getCloudiness() {
return cloudiness;
}
/**
* Sets the value of the 'cloudiness' field.
* Current cloudiness (%).
* @param value The value of 'cloudiness'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setCloudiness(java.lang.Float value) {
validate(fields()[7], value);
this.cloudiness = value;
fieldSetFlags()[7] = true;
return this;
}
/**
* Checks whether the 'cloudiness' field has been set.
* Current cloudiness (%).
* @return True if the 'cloudiness' field has been set, false otherwise.
*/
public boolean hasCloudiness() {
return fieldSetFlags()[7];
}
/**
* Clears the value of the 'cloudiness' field.
* Current cloudiness (%).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearCloudiness() {
cloudiness = null;
fieldSetFlags()[7] = false;
return this;
}
/**
* Gets the value of the 'precipitation' field.
* Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
* @return The value.
*/
public java.lang.Float getPrecipitation() {
return precipitation;
}
/**
* Sets the value of the 'precipitation' field.
* Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
* @param value The value of 'precipitation'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setPrecipitation(java.lang.Float value) {
validate(fields()[8], value);
this.precipitation = value;
fieldSetFlags()[8] = true;
return this;
}
/**
* Checks whether the 'precipitation' field has been set.
* Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
* @return True if the 'precipitation' field has been set, false otherwise.
*/
public boolean hasPrecipitation() {
return fieldSetFlags()[8];
}
/**
* Clears the value of the 'precipitation' field.
* Rain or snow volume over last hours reported in 'percipitationPeriod' (mm).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearPrecipitation() {
precipitation = null;
fieldSetFlags()[8] = false;
return this;
}
/**
* Gets the value of the 'precipitationPeriod' field.
* Period over which the precipitation was determined (h).
* @return The value.
*/
public java.lang.Integer getPrecipitationPeriod() {
return precipitationPeriod;
}
/**
* Sets the value of the 'precipitationPeriod' field.
* Period over which the precipitation was determined (h).
* @param value The value of 'precipitationPeriod'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setPrecipitationPeriod(java.lang.Integer value) {
validate(fields()[9], value);
this.precipitationPeriod = value;
fieldSetFlags()[9] = true;
return this;
}
/**
* Checks whether the 'precipitationPeriod' field has been set.
* Period over which the precipitation was determined (h).
* @return True if the 'precipitationPeriod' field has been set, false otherwise.
*/
public boolean hasPrecipitationPeriod() {
return fieldSetFlags()[9];
}
/**
* Clears the value of the 'precipitationPeriod' field.
* Period over which the precipitation was determined (h).
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearPrecipitationPeriod() {
precipitationPeriod = null;
fieldSetFlags()[9] = false;
return this;
}
/**
* Gets the value of the 'condition' field.
* Current weather condition.
* @return The value.
*/
public org.radarcns.passive.weather.WeatherCondition getCondition() {
return condition;
}
/**
* Sets the value of the 'condition' field.
* Current weather condition.
* @param value The value of 'condition'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setCondition(org.radarcns.passive.weather.WeatherCondition value) {
validate(fields()[10], value);
this.condition = value;
fieldSetFlags()[10] = true;
return this;
}
/**
* Checks whether the 'condition' field has been set.
* Current weather condition.
* @return True if the 'condition' field has been set, false otherwise.
*/
public boolean hasCondition() {
return fieldSetFlags()[10];
}
/**
* Clears the value of the 'condition' field.
* Current weather condition.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearCondition() {
condition = null;
fieldSetFlags()[10] = false;
return this;
}
/**
* Gets the value of the 'source' field.
* From which source/API the weather data was retrieved.
* @return The value.
*/
public java.lang.String getSource() {
return source;
}
/**
* Sets the value of the 'source' field.
* From which source/API the weather data was retrieved.
* @param value The value of 'source'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setSource(java.lang.String value) {
validate(fields()[11], value);
this.source = value;
fieldSetFlags()[11] = true;
return this;
}
/**
* Checks whether the 'source' field has been set.
* From which source/API the weather data was retrieved.
* @return True if the 'source' field has been set, false otherwise.
*/
public boolean hasSource() {
return fieldSetFlags()[11];
}
/**
* Clears the value of the 'source' field.
* From which source/API the weather data was retrieved.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearSource() {
source = null;
fieldSetFlags()[11] = false;
return this;
}
/**
* Gets the value of the 'locationSource' field.
* The source of the location used to get the weather details.
* @return The value.
*/
public org.radarcns.passive.weather.LocationType getLocationSource() {
return locationSource;
}
/**
* Sets the value of the 'locationSource' field.
* The source of the location used to get the weather details.
* @param value The value of 'locationSource'.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder setLocationSource(org.radarcns.passive.weather.LocationType value) {
validate(fields()[12], value);
this.locationSource = value;
fieldSetFlags()[12] = true;
return this;
}
/**
* Checks whether the 'locationSource' field has been set.
* The source of the location used to get the weather details.
* @return True if the 'locationSource' field has been set, false otherwise.
*/
public boolean hasLocationSource() {
return fieldSetFlags()[12];
}
/**
* Clears the value of the 'locationSource' field.
* The source of the location used to get the weather details.
* @return This builder.
*/
public org.radarcns.passive.weather.LocalWeather.Builder clearLocationSource() {
locationSource = null;
fieldSetFlags()[12] = false;
return this;
}
@Override
@SuppressWarnings("unchecked")
public LocalWeather build() {
try {
LocalWeather record = new LocalWeather();
record.time = fieldSetFlags()[0] ? this.time : (java.lang.Double) defaultValue(fields()[0]);
record.timeReceived = fieldSetFlags()[1] ? this.timeReceived : (java.lang.Double) defaultValue(fields()[1]);
record.sunrise = fieldSetFlags()[2] ? this.sunrise : (java.lang.Integer) defaultValue(fields()[2]);
record.sunset = fieldSetFlags()[3] ? this.sunset : (java.lang.Integer) defaultValue(fields()[3]);
record.temperature = fieldSetFlags()[4] ? this.temperature : (java.lang.Float) defaultValue(fields()[4]);
record.pressure = fieldSetFlags()[5] ? this.pressure : (java.lang.Float) defaultValue(fields()[5]);
record.humidity = fieldSetFlags()[6] ? this.humidity : (java.lang.Float) defaultValue(fields()[6]);
record.cloudiness = fieldSetFlags()[7] ? this.cloudiness : (java.lang.Float) defaultValue(fields()[7]);
record.precipitation = fieldSetFlags()[8] ? this.precipitation : (java.lang.Float) defaultValue(fields()[8]);
record.precipitationPeriod = fieldSetFlags()[9] ? this.precipitationPeriod : (java.lang.Integer) defaultValue(fields()[9]);
record.condition = fieldSetFlags()[10] ? this.condition : (org.radarcns.passive.weather.WeatherCondition) defaultValue(fields()[10]);
record.source = fieldSetFlags()[11] ? this.source : (java.lang.String) defaultValue(fields()[11]);
record.locationSource = fieldSetFlags()[12] ? this.locationSource : (org.radarcns.passive.weather.LocationType) defaultValue(fields()[12]);
return record;
} catch (org.apache.avro.AvroMissingFieldException e) {
throw e;
} catch (java.lang.Exception e) {
throw new org.apache.avro.AvroRuntimeException(e);
}
}
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumWriter
WRITER$ = (org.apache.avro.io.DatumWriter)MODEL$.createDatumWriter(SCHEMA$);
@Override public void writeExternal(java.io.ObjectOutput out)
throws java.io.IOException {
WRITER$.write(this, SpecificData.getEncoder(out));
}
@SuppressWarnings("unchecked")
private static final org.apache.avro.io.DatumReader
READER$ = (org.apache.avro.io.DatumReader)MODEL$.createDatumReader(SCHEMA$);
@Override public void readExternal(java.io.ObjectInput in)
throws java.io.IOException {
READER$.read(this, SpecificData.getDecoder(in));
}
@Override protected boolean hasCustomCoders() { return true; }
@Override public void customEncode(org.apache.avro.io.Encoder out)
throws java.io.IOException
{
out.writeDouble(this.time);
out.writeDouble(this.timeReceived);
if (this.sunrise == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeInt(this.sunrise);
}
if (this.sunset == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeInt(this.sunset);
}
if (this.temperature == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.temperature);
}
if (this.pressure == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.pressure);
}
if (this.humidity == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.humidity);
}
if (this.cloudiness == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.cloudiness);
}
if (this.precipitation == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeFloat(this.precipitation);
}
if (this.precipitationPeriod == null) {
out.writeIndex(0);
out.writeNull();
} else {
out.writeIndex(1);
out.writeInt(this.precipitationPeriod);
}
out.writeEnum(this.condition.ordinal());
out.writeString(this.source);
out.writeEnum(this.locationSource.ordinal());
}
@Override public void customDecode(org.apache.avro.io.ResolvingDecoder in)
throws java.io.IOException
{
org.apache.avro.Schema.Field[] fieldOrder = in.readFieldOrderIfDiff();
if (fieldOrder == null) {
this.time = in.readDouble();
this.timeReceived = in.readDouble();
if (in.readIndex() != 1) {
in.readNull();
this.sunrise = null;
} else {
this.sunrise = in.readInt();
}
if (in.readIndex() != 1) {
in.readNull();
this.sunset = null;
} else {
this.sunset = in.readInt();
}
if (in.readIndex() != 1) {
in.readNull();
this.temperature = null;
} else {
this.temperature = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.pressure = null;
} else {
this.pressure = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.humidity = null;
} else {
this.humidity = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.cloudiness = null;
} else {
this.cloudiness = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.precipitation = null;
} else {
this.precipitation = in.readFloat();
}
if (in.readIndex() != 1) {
in.readNull();
this.precipitationPeriod = null;
} else {
this.precipitationPeriod = in.readInt();
}
this.condition = org.radarcns.passive.weather.WeatherCondition.values()[in.readEnum()];
this.source = in.readString();
this.locationSource = org.radarcns.passive.weather.LocationType.values()[in.readEnum()];
} else {
for (int i = 0; i < 13; i++) {
switch (fieldOrder[i].pos()) {
case 0:
this.time = in.readDouble();
break;
case 1:
this.timeReceived = in.readDouble();
break;
case 2:
if (in.readIndex() != 1) {
in.readNull();
this.sunrise = null;
} else {
this.sunrise = in.readInt();
}
break;
case 3:
if (in.readIndex() != 1) {
in.readNull();
this.sunset = null;
} else {
this.sunset = in.readInt();
}
break;
case 4:
if (in.readIndex() != 1) {
in.readNull();
this.temperature = null;
} else {
this.temperature = in.readFloat();
}
break;
case 5:
if (in.readIndex() != 1) {
in.readNull();
this.pressure = null;
} else {
this.pressure = in.readFloat();
}
break;
case 6:
if (in.readIndex() != 1) {
in.readNull();
this.humidity = null;
} else {
this.humidity = in.readFloat();
}
break;
case 7:
if (in.readIndex() != 1) {
in.readNull();
this.cloudiness = null;
} else {
this.cloudiness = in.readFloat();
}
break;
case 8:
if (in.readIndex() != 1) {
in.readNull();
this.precipitation = null;
} else {
this.precipitation = in.readFloat();
}
break;
case 9:
if (in.readIndex() != 1) {
in.readNull();
this.precipitationPeriod = null;
} else {
this.precipitationPeriod = in.readInt();
}
break;
case 10:
this.condition = org.radarcns.passive.weather.WeatherCondition.values()[in.readEnum()];
break;
case 11:
this.source = in.readString();
break;
case 12:
this.locationSource = org.radarcns.passive.weather.LocationType.values()[in.readEnum()];
break;
default:
throw new java.io.IOException("Corrupt ResolvingDecoder.");
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy