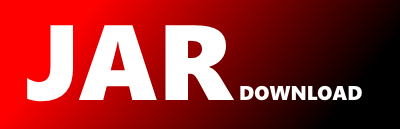
org.randombits.confluence.intercom.thing.ThingFinder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of confluence-intercom Show documentation
Show all versions of confluence-intercom Show documentation
This is a support library for Confluence plugins, providing some
commonly-used classes and libraries.
The newest version!
package org.randombits.confluence.intercom.thing;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import org.apache.log4j.Logger;
import org.randombits.confluence.intercom.ConnectionBundle;
import org.randombits.confluence.intercom.LocalIntercom4;
import org.randombits.confluence.intercom.LocalIntercomListener;
/**
* This class provides support for the common case of plugins wishing to publish
* services to other plugins, or subscribe to specific services provided by
* other plugins.
*
* @author David Peterson
* @param
* The value type.
* @param
* The provider connection type.
*/
public abstract class ThingFinder> implements LocalIntercomListener {
private static final Logger LOG = Logger.getLogger( ThingFinder.class );
private Class connectionType;
private Set mThings;
private Set uThings;
private Class thingType;
private List> listeners;
public ThingFinder( Class thingType, Class connectionType ) {
if ( ThingConnection.class.equals( connectionType ) )
throw new IllegalArgumentException(
"Connection types must be a subclass of ThingConnection, not ThingConnection itself." );
mThings = new java.util.HashSet();
uThings = Collections.unmodifiableSet( mThings );
this.connectionType = connectionType;
this.thingType = thingType;
LocalIntercom4 intercom = LocalIntercom4.getInstance();
synchronized ( intercom ) {
addThings( intercom.findConnections( connectionType ) );
intercom.addLocalIntercomListener( this );
}
}
private void addThings( Collection connections ) {
if ( connections != null ) {
for ( ThingConnection p : connections ) {
addThing( p );
}
} else {
warnAboutNullThings();
}
}
private void warnAboutNullThings() {
LOG.warn( "Null connections passed into ThingFinder." );
}
private void addThings( C[] connections ) {
if ( connections != null ) {
for ( ThingConnection p : connections ) {
addThing( p );
}
} else {
warnAboutNullThings();
}
}
private void addThing( ThingConnection p ) {
T value = p.getThing();
if ( mThings.add( value ) ) {
if ( listeners != null ) {
for ( ThingListener listener : listeners )
listener.thingProvided( value );
}
}
}
/**
* Returns an unmodifiable set of the values found.
*
* @return
*/
public Set getThings() {
return uThings;
}
public Class getThingType() {
return thingType;
}
public synchronized void addedConnectionBundle( ConnectionBundle bundle ) {
addThings( bundle.getConnections( connectionType ) );
}
public synchronized void removedConnectionBundle( ConnectionBundle bundle ) {
removeThings( bundle.getConnections( connectionType ) );
}
private void removeThings( C[] connections ) {
if ( connections != null ) {
for ( ThingConnection p : connections ) {
T value = p.getThing();
if ( mThings.remove( value ) ) {
if ( listeners != null ) {
for ( ThingListener listener : listeners )
listener.thingRemoved( value );
}
}
}
} else {
warnAboutNullThings();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy