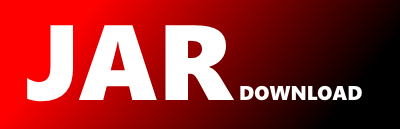
org.rapidoid.collection.Coll Maven / Gradle / Ivy
/*-
* #%L
* rapidoid-commons
* %%
* Copyright (C) 2014 - 2018 Nikolche Mihajlovski and contributors
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package org.rapidoid.collection;
import org.rapidoid.RapidoidThing;
import org.rapidoid.annotation.Authors;
import org.rapidoid.annotation.Since;
import org.rapidoid.cls.Cls;
import org.rapidoid.commons.Err;
import org.rapidoid.data.JSON;
import org.rapidoid.datamodel.Results;
import org.rapidoid.lambda.Mapper;
import org.rapidoid.u.U;
import java.lang.reflect.Constructor;
import java.util.*;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicBoolean;
@Authors("Nikolche Mihajlovski")
@Since("5.1.0")
public class Coll extends RapidoidThing {
@SuppressWarnings("unchecked")
@SafeVarargs
public static Set synchronizedSet(T... values) {
return Collections.synchronizedSet(U.set(values));
}
@SuppressWarnings("unchecked")
@SafeVarargs
public static List synchronizedList(T... values) {
return Collections.synchronizedList(U.list(values));
}
public static Set concurrentSet() {
return Collections.newSetFromMap(new ConcurrentHashMap());
}
public static boolean isMap(Object obj) {
return obj instanceof Map, ?>;
}
public static boolean isList(Object obj) {
return obj instanceof List>;
}
public static boolean isSet(Object obj) {
return obj instanceof Set>;
}
public static boolean isCollection(Object obj) {
return obj instanceof Collection>;
}
public static void assign(Collection destination, Collection extends T> source) {
Err.argMust(destination != null, "destination cannot be null!");
destination.clear();
if (source != null) {
destination.addAll(source);
}
}
public static void assign(Collection super T> destination, T[] source) {
Err.argMust(destination != null, "destination cannot be null!");
destination.clear();
if (source != null) {
Collections.addAll(destination, source);
}
}
public static void assign(Map destination, Map extends K, ? extends V> source) {
Err.argMust(destination != null, "destination cannot be null!");
destination.clear();
if (source != null) {
destination.putAll(source);
}
}
public static V get(Map map, K key) {
V value = map.get(key);
U.notNull(value, "map[%s]", key);
return value;
}
public static V get(Map map, K key, V defaultValue) {
V value = map.get(key);
return value != null ? value : defaultValue;
}
public static ConcurrentMap concurrentMap() {
return new ConcurrentHashMap();
}
public static ConcurrentMap concurrentMap(Map extends K, ? extends V> src, boolean ignoreNullValues) {
ConcurrentMap map = concurrentMap();
for (Map.Entry extends K, ? extends V> e : src.entrySet()) {
if (!ignoreNullValues || e.getValue() != null) {
map.put(e.getKey(), e.getValue());
}
}
return map;
}
public static ConcurrentMap concurrentMap(K key, V value) {
ConcurrentMap map = concurrentMap();
map.put(key, value);
return map;
}
public static ConcurrentMap concurrentMap(K key1, V value1, K key2, V value2) {
ConcurrentMap map = concurrentMap(key1, value1);
map.put(key2, value2);
return map;
}
public static ConcurrentMap concurrentMap(K key1, V value1, K key2, V value2, K key3, V value3) {
ConcurrentMap map = concurrentMap(key1, value1, key2, value2);
map.put(key3, value3);
return map;
}
public static ConcurrentMap concurrentMap(K key1, V value1, K key2, V value2, K key3, V value3,
K key4, V value4) {
ConcurrentMap map = concurrentMap(key1, value1, key2, value2, key3, value3);
map.put(key4, value4);
return map;
}
public static ConcurrentMap concurrentMap(K key1, V value1, K key2, V value2, K key3, V value3,
K key4, V value4, K key5, V value5) {
ConcurrentMap map = concurrentMap(key1, value1, key2, value2, key3, value3, key4, value4);
map.put(key5, value5);
return map;
}
@SuppressWarnings("unchecked")
public static ConcurrentMap concurrentMap(Object... keysAndValues) {
U.must(keysAndValues.length % 2 == 0, "Incorrect number of arguments (expected key-value pairs)!");
ConcurrentMap map = concurrentMap();
for (int i = 0; i < keysAndValues.length / 2; i++) {
map.put((K) keysAndValues[i * 2], (V) keysAndValues[i * 2 + 1]);
}
return map;
}
public static Map orderedMap() {
return new LinkedHashMap();
}
public static Map orderedMap(Map extends K, ? extends V> src, boolean ignoreNullValues) {
Map map = orderedMap();
for (Map.Entry extends K, ? extends V> e : src.entrySet()) {
if (!ignoreNullValues || e.getValue() != null) {
map.put(e.getKey(), e.getValue());
}
}
return map;
}
public static Map orderedMap(K key, V value) {
Map map = orderedMap();
map.put(key, value);
return map;
}
public static Map orderedMap(K key1, V value1, K key2, V value2) {
Map map = orderedMap(key1, value1);
map.put(key2, value2);
return map;
}
public static Map orderedMap(K key1, V value1, K key2, V value2, K key3, V value3) {
Map map = orderedMap(key1, value1, key2, value2);
map.put(key3, value3);
return map;
}
public static Map orderedMap(K key1, V value1, K key2, V value2, K key3, V value3, K key4, V value4) {
Map map = orderedMap(key1, value1, key2, value2, key3, value3);
map.put(key4, value4);
return map;
}
public static Map orderedMap(K key1, V value1, K key2, V value2, K key3, V value3, K key4, V value4,
K key5, V value5) {
Map map = orderedMap(key1, value1, key2, value2, key3, value3, key4, value4);
map.put(key5, value5);
return map;
}
@SuppressWarnings("unchecked")
public static Map orderedMap(Object... keysAndValues) {
U.must(keysAndValues.length % 2 == 0, "Incorrect number of arguments (expected key-value pairs)!");
Map map = orderedMap();
for (int i = 0; i < keysAndValues.length / 2; i++) {
map.put((K) keysAndValues[i * 2], (V) keysAndValues[i * 2 + 1]);
}
return map;
}
public static Map synchronizedMap() {
return Collections.synchronizedMap(U.map());
}
public static Queue queue() {
return new ConcurrentLinkedQueue();
}
public static BlockingQueue queue(int maxSize) {
Err.argMust(maxSize > 0, "Maximum queue size must be > 0!");
return new ArrayBlockingQueue(maxSize);
}
public static Map autoExpandingMap(final Class keyClass, final Class valueClass) {
try {
// search for the key-based constructor
final Constructor constructor = valueClass.getConstructor(keyClass);
return autoExpandingMap(new Mapper() {
@SuppressWarnings("unchecked")
@Override
public V map(K key) throws Exception {
try {
return (V) constructor.newInstance(key);
} catch (Exception e) {
throw U.rte(e);
}
}
});
} catch (NoSuchMethodException e) {
// otherwise, use the default constructor
Constructor constructor;
try {
constructor = valueClass.getConstructor();
} catch (NoSuchMethodException e2) {
throw U.rte("Couldn't find a matching constructor for the auto-expanding map!");
}
final Constructor defConstructor = constructor;
return autoExpandingMap(new Mapper() {
@SuppressWarnings("unchecked")
@Override
public V map(K key) throws Exception {
try {
return (V) defConstructor.newInstance();
} catch (Exception e) {
throw U.rte(e);
}
}
});
}
}
@SuppressWarnings("serial")
public static Map autoExpandingMap(Mapper valueFactory) {
return new AutoExpandingMap(valueFactory);
}
public static Map> mapOfMaps() {
return autoExpandingMap(new Mapper>() {
@Override
public Map map(K1 src) throws Exception {
return synchronizedMap();
}
});
}
public static Map>> mapOfMapOfMaps() {
return autoExpandingMap(new Mapper>>() {
@Override
public Map> map(K1 src) throws Exception {
return mapOfMaps();
}
});
}
public static Map> mapOfLists() {
return autoExpandingMap(new Mapper>() {
@SuppressWarnings("unchecked")
@Override
public List map(K src) throws Exception {
return synchronizedList();
}
});
}
public static Map>> mapOfMapOfLists() {
return autoExpandingMap(new Mapper>>() {
@Override
public Map> map(K1 src) throws Exception {
return mapOfLists();
}
});
}
public static Map> mapOfSets() {
return autoExpandingMap(new Mapper>() {
@SuppressWarnings("unchecked")
@Override
public Set map(K src) throws Exception {
return synchronizedSet();
}
});
}
public static Map>> mapOfMapOfSets() {
return autoExpandingMap(new Mapper>>() {
@Override
public Map> map(K1 src) throws Exception {
return mapOfSets();
}
});
}
@SuppressWarnings("unchecked")
public static List range(Iterable items, int from, int to) {
U.must(from <= to, "'from' (%s) must be <= 'to' (%s)!", from, to);
if (from == to) {
return Collections.emptyList();
}
if (items instanceof Results) {
Results results = (Results) items;
return results.page(from, to - from);
}
List> list = (items instanceof List>) ? (List>) items : U.list(items);
from = Math.min(from, list.size());
to = Math.min(to, list.size());
return U.cast(list.subList(from, to));
}
public static Integer getSizeOrNull(Iterable> items) {
return (items instanceof Collection>) ? ((Collection>) items).size() : null;
}
public static ChangeTrackingMap trackChanges(Map map, AtomicBoolean dirtyFlag) {
return new ChangeTrackingMap(map, dirtyFlag);
}
public static Map toBeanMap(Map data, Class type) {
Map map = U.map();
for (Map.Entry e : data.entrySet()) {
T bean;
Object value = e.getValue();
if (value instanceof Map) {
bean = JSON.MAPPER.convertValue(value, type);
} else if (value instanceof String) {
bean = Cls.newInstance(type, value);
} else {
throw U.rte("Unsupported configuration type: %s", Cls.of(value));
}
map.put(e.getKey(), bean);
}
return Collections.unmodifiableMap(map);
}
public static Map deepCopyOf(Map map) {
return JSON.parseMap(JSON.stringify(map)); // FIXME proper implementation
}
public static Set copyOf(Set src) {
Set copy;
synchronized (src) {
copy = U.set(src);
}
return copy;
}
public static List copyOf(List src) {
List copy;
synchronized (src) {
copy = U.list(src);
}
return copy;
}
public static Map syncCopy(Map env) {
return Collections.synchronizedMap(U.map(env));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy