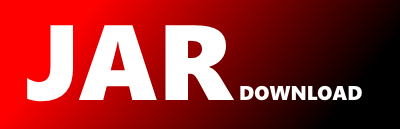
org.rapidoid.http.Req Maven / Gradle / Ivy
package org.rapidoid.http;
/*
* #%L
* rapidoid-http-api
* %%
* Copyright (C) 2014 - 2015 Nikolche Mihajlovski and contributors
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import java.io.Serializable;
import java.util.Map;
import org.rapidoid.annotation.Authors;
import org.rapidoid.annotation.P;
import org.rapidoid.annotation.Since;
@Authors("Nikolche Mihajlovski")
@Since("4.2.0")
public interface Req {
/* REQUEST METHODS: */
String verb();
String uri();
String path();
String subpath();
String query();
String protocol();
String body();
String host();
Map params();
String param(@P("name") String name);
String param(@P("name") String name, @P("defaultValue") String defaultValue);
Map headers();
String header(@P("name") String name);
String header(@P("name") String name, @P("defaultValue") String defaultValue);
Map cookies();
String cookie(@P("name") String name);
String cookie(@P("name") String name, @P("defaultValue") String defaultValue);
Map posted();
T posted(@P("name") String name);
T posted(@P("name") String name, @P("defaultValue") T defaultValue);
Map files();
byte[] file(@P("name") String name);
byte[] file(@P("name") String name, @P("defaultValue") byte[] defaultValue);
/**
* Data includes params + posted.
*/
Map data();
/**
* Data includes params + posted.
*/
T data(@P("name") String name);
/**
* Data includes params + posted.
*/
T data(@P("name") String name, @P("defaultValue") T defaultValue);
String home();
String[] pathSegments();
String pathSegment(@P("segmentIndex") int segmentIndex);
String realIpAddress();
boolean isGetReq();
boolean isPostReq();
boolean isDevMode();
long requestId();
/* STATE: */
String sessionId();
/* SESSION SCOPE: */
Map session();
T session(@P("name") String name);
T session(@P("name") String name, @P("defaultValue") T defaultValue);
T sessionGetOrCreate(@P("name") String name, @P("valueClass") Class valueClass,
@P("constructorArgs") Object... constructorArgs);
/* COOKIEPACK SCOPE: */
Map cookiepack();
T cookiepack(@P("name") String name);
T cookiepack(@P("name") String name, @P("defaultValue") T defaultValue);
T cookiepackGetOrCreate(@P("name") String name, @P("valueClass") Class valueClass,
@P("constructorArgs") Object... constructorArgs);
/* LOCAL SCOPE: */
Map locals();
T local(@P("key") String key);
T local(@P("key") String key, @P("defaultValue") T defaultValue);
T localGetOrCreate(@P("name") String name, @P("valueClass") Class valueClass,
@P("constructorArgs") Object... constructorArgs);
/* TMP SCOPE: */
Map tmps();
T tmp(@P("key") String key);
T tmp(@P("key") String key, @P("defaultValue") T defaultValue);
T tmpGetOrCreate(@P("name") String name, @P("valueClass") Class valueClass,
@P("constructorArgs") Object... constructorArgs);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy