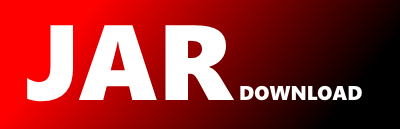
org.rapidoid.http.Resp Maven / Gradle / Ivy
package org.rapidoid.http;
import org.rapidoid.annotation.Since;
import org.rapidoid.net.AsyncLogic;
import org.rapidoid.web.Screen;
import java.io.File;
import java.io.OutputStream;
import java.io.Serializable;
import java.nio.ByteBuffer;
import java.util.Map;
/*
* #%L
* rapidoid-http-fast
* %%
* Copyright (C) 2014 - 2017 Nikolche Mihajlovski and contributors
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
/**
* HTTP Response API.
*/
@Since("5.0.x")
public interface Resp {
/**
* Sets the content to be serialized into a body when the HTTP response is rendered.
*/
Resp result(Object content);
/**
* Gets the content to be serialized into a body when the HTTP response is rendered.
*/
Object result();
/**
* Sets the HTTP response body from a byte[]
data that is written as a HTTP response body when rendered.
*/
Resp body(byte[] body);
/**
* Sets the HTTP response body from a ByteBuffer
data that is written as a HTTP response body when rendered.
*/
Resp body(ByteBuffer body);
/**
* Gets the HTTP response body data (of type byte[] or ByteBuffer) that is written as a HTTP response body when rendered.
*/
Object body();
/**
* Sets the raw HTTP response (headers and body) from a byte[]
data that is written as a HTTP response when rendered.
*/
Resp raw(byte[] raw);
/**
* Sets the raw HTTP response (headers and body) from a ByteBuffer
data that is written as a HTTP response when rendered.
*/
Resp raw(ByteBuffer raw);
/**
* Gets the raw HTTP response (headers and body) data (of type byte[] or ByteBuffer) that is written as a HTTP response when rendered.
*/
Object raw();
/**
* Sets the status code (e.g. 200, 404, 500) of the HTTP response.
*/
Resp code(int code);
/**
* Gets the status code (e.g. 200, 404, 500) of the HTTP response.
*/
int code();
/**
* Sets the Content-Type
header to be rendered in the HTTP response.
*/
Resp contentType(MediaType contentType);
/**
* Gets the Content-Type
header to be rendered in the HTTP response.
*/
MediaType contentType();
/**
* Sets the redirect URI of the HTTP response.
* Setting this will cause a HTTP 30x redirect response.
*/
Resp redirect(String redirectURI);
/**
* Gets the redirect URI of the HTTP response.
*/
String redirect();
/**
* Sets the filename when serving a file in the HTTP response.
*/
Resp filename(String filename);
/**
* Gets the filename when serving a file in the HTTP response.
*/
String filename();
/**
* Sets a custom name of the view (V from MVC) of the HTTP response.
* This also sets mvc to true
.
* The default view name equals the request path without the "/" prefix, except for the "/" path, where the view name is "index".
* E.g. "/abc" -> "abc", "/" -> "index", "/my/books" -> "my/books".
*/
Resp view(String viewName);
/**
* Gets the (default or customized) name of the view (V from MVC) of the HTTP response.
* The default view name equals the request path without the "/" prefix, except for the "/" path, where the view name is "index".
* E.g. "/abc" -> "abc", "/" -> "index", "/my/books" -> "my/books".
*/
String view();
/**
* Disables the view rendering for the target MVC route. The page decorator remains enabled.
*/
Resp noView();
/**
* Sets the file to be served when the HTTP response is rendered.
*/
Resp file(File file);
/**
* Gets the file to be served when the HTTP response is rendered.
*/
File file();
/**
* Provides read/write access to the headers of the HTTP response.
*/
Map headers();
/**
* Sets a header of the HTTP response.
*/
Resp header(String name, String value);
/**
* Provides read/write access to the cookies of the HTTP response.
*/
Map cookies();
/**
* Sets a cookie of the HTTP response.
*/
Resp cookie(String name, String value, String... extras);
/**
* Provides read/write access to the server-side session attributes of the HTTP request/response.
*/
Map session();
/**
* Sets a session attribute of the HTTP response.
*/
Resp session(String name, Serializable value);
/**
* Provides read/write access to the token attributes of the HTTP request/response.
*/
Map token();
/**
* Sets a token attribute of the HTTP response.
*/
Resp token(String name, Serializable value);
/**
* Provides read/write access to the model (M from MVC) that will be rendered by the view renderer.
*/
Map model();
/**
* Sets an attribute of the model (M from MVC) that will be rendered by the view renderer.
*/
Resp model(String name, Object value);
/**
* Informs the HTTP server that the asynchronous handling has finished and the response is complete.
* Alias to request().done()
.
*/
Resp done();
/**
* Sets the Content-Type: text/plain; charset=utf-8
header and the content of the HTTP response.
* Alias to contentType(MediaType.PLAIN_TEXT_UTF_8).body(content)
.
*/
Resp plain(Object content);
/**
* Sets the Content-Type: text/html; charset=utf-8
header and the content of the HTTP response.
* Alias to contentType(MediaType.HTML_UTF_8).body(content)
.
*/
Resp html(Object content);
/**
* Sets the Content-Type: application/json
header and the content of the HTTP response.
* Alias to contentType(MediaType.JSON).body(content)
.
*/
Resp json(Object content);
/**
* Sets the Content-Type: application/octet-stream
header and the content of the HTTP response.
* Alias to contentType(MediaType.BINARY).body(content)
.
*/
Resp binary(Object content);
/**
* Checks whether the response model and view will be rendered in a MVC fashion.
* A typical renderer would use Resp#view
to get the view name, and Resp#model
to get the model.
* A custom view renderer can be configured/implemented via the On.custom().viewResolver(...)
method.
*/
boolean mvc();
/**
* Sets whether the response model and view will be rendered in a MVC fashion.
* A typical renderer would use Resp#view
to get the view name, and Resp#model
to get the model.
* A custom view renderer can be configured/implemented via the On.custom().viewResolver(...)
method.
*/
Resp mvc(boolean mvc);
/**
* First renders the response headers, then returns an OutputStream representing
* the response body. The response body will be constructed by writing to the OutputStream.
*/
OutputStream out();
/**
* Gets the reference to the request object.
*/
Req request();
/**
* Initiates a user login process with the specified username and password.
* After a successful login, the username will be persisted in the token.
* Returns information whether the login was successful
*/
boolean login(String username, String password);
/**
* Initiates a user logout process, clearing the login information (username) from the token.
*/
void logout();
/**
* Provides access to the screen model for custom (MVC) page rendering.
*/
Screen screen();
/**
* Resumes the asynchronous request handling.
*/
void resume(AsyncLogic asyncLogic);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy