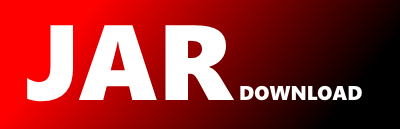
org.rapidoid.http.Req Maven / Gradle / Ivy
The newest version!
/*-
* #%L
* rapidoid-http-fast
* %%
* Copyright (C) 2014 - 2018 Nikolche Mihajlovski and contributors
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package org.rapidoid.http;
import org.rapidoid.annotation.Authors;
import org.rapidoid.annotation.Since;
import org.rapidoid.http.customize.Customization;
import org.rapidoid.io.Upload;
import java.io.OutputStream;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
/**
* HTTP Request API.
*/
@Authors("Nikolche Mihajlovski")
@Since("5.0.2")
public interface Req {
/* HTTP REQUEST DATA */
/**
* Gets the verb of the HTTP request.
*/
String verb();
/**
* Gets the uri of the HTTP request.
*/
String uri();
/**
* Gets the path of the HTTP request.
*/
String path();
/**
* Gets the query of the HTTP request.
*/
String query();
/**
* Gets the raw body data of the HTTP request.
*/
byte[] body();
/**
* Gets the value of the Host header of the HTTP request.
*/
String host();
/**
* Gets the name of the application zone handling the request.
* The default zone name is main
for the On
API, and admin
for the Admin
API.
*/
String zone();
/**
* Gets the context path of the application zone handling the request.
* The default context path is /
for the On
API, and /_
for the Admin
API.
*/
String contextPath();
/**
* Gets the IP address of the HTTP client directly sending the request.
* This can be the address of a real user, or a HTTP proxy (if the user uses such), or a reverse proxy (if the application/server uses such).
*/
String clientIpAddress();
/**
* A best-effort attempt to infer the real IP address of the end user/client/client proxy.
* If a reverse proxy is detected with high confidence (or configured), its headers will be used to get the real IP address of the user.
* Otherwise, the value of Req#clientIpAddress()
is returned.
*/
String realIpAddress();
/**
* Gets the HTTP connection ID, which is unique per HTTP server instance.
*/
long connectionId();
/**
* Gets the HTTP request ID, which is unique per HTTP server instance.
*/
long requestId();
/* URL PARAMETERS: */
/**
* Gets the URL parameters of the HTTP request.
*/
Map params();
/**
* Returns the value of the specified mandatory URL parameter from the HTTP request, or throws a runtime
* exception if it is not found.
*/
String param(String name);
/**
* Returns the value of the specified optional URL parameter from the HTTP request, or the specified default
* value, if not found.
*/
String param(String name, String defaultValue);
/**
* Returns a new instance of the specified bean type, with properties initialized from the URL parameters of the HTTP request.
*/
T param(Class beanType);
/* POSTED PARAMETERS IN THE REQUEST BODY: */
/**
* Gets the posted parameters of the HTTP request body.
*/
Map posted();
/**
* Returns the value of the specified posted parameter from the HTTP request body, or throws a runtime
* exception if it is not found.
*/
T posted(String name);
/**
* Returns the value of the specified posted parameter from the HTTP request body, or the specified default
* value, if it is not found.
*/
T posted(String name, T defaultValue);
/**
* Returns a new instance of the specified bean type, with properties initialized from the posted parameters of the HTTP request.
*/
T posted(Class beanType);
/* UPLOADED FILES IN THE REQUEST BODY: */
/**
* Gets the uploaded files from the HTTP request body.
*/
Map> files();
/**
* Returns the uploaded files with the specified form parameter name (not filename) from the HTTP request body,
* or throws a runtime exception if not found.
*/
List files(String name);
/**
* Returns exactly one posted file with the specified form parameter name (not filename) from the HTTP request body,
* or throws a runtime exception if not found.
*/
Upload file(String name);
/* REQUEST DATA PARAMETERS (URL PARAMETERS + POSTED PARAMETERS + UPLOADED FILES): */
/**
* Gets the data parameters (URL parameters + posted parameters + uploaded files) of the HTTP request.
*/
Map data();
/**
* Returns the value of the specified data parameter from the HTTP request, or throws a runtime exception if
* it is not found.
*/
T data(String name);
/**
* Returns the value of the specified data parameter from the HTTP request, or the specified default value,
* if it is not found.
*/
T data(String name, T defaultValue);
/**
* Returns a new instance of the specified bean type, with properties initialized from the data parameters of the HTTP request.
*/
T data(Class beanType);
/* EXTRA ATTRIBUTES ATTACHED TO THE REQUEST: */
/**
* Gets the extra attributes of the HTTP request.
*/
Map attrs();
/**
* Returns the value of an extra attribute from the HTTP request, or throws a runtime exception if it is not
* found.
*/
T attr(String name);
/**
* Returns the value of the specified extra attribute from the HTTP request, or the specified default value,
* if it is not found.
*/
T attr(String name, T defaultValue);
/* SERVER-SIDE SESSION: */
/**
* Returns the ID of the session (the value of the "JSESSIONID" cookie). If a session doesn't exist, a new
* session is created.
*/
String sessionId();
/**
* Does the HTTP request have a server-side session attached?
*/
boolean hasSession();
/**
* Provides read/write access to the server-side session attributes of the HTTP request/response.
*/
Map session();
/**
* Returns the value of the specified server-side session attribute from the HTTP request/response, or throws a
* runtime exception if it is not found.
*/
T session(String name);
/**
* Returns the value of the specified server-side session attribute from the HTTP request/response, or the specified
* default value, if it is not found.
*/
T session(String name, T defaultValue);
/* TOKEN DATA: */
/**
* Does the HTTP request have a token attached?
*/
boolean hasToken();
/**
* Provides read/write access to the token attributes of the HTTP request/response.
*/
Map token();
/**
* Returns the value of the specified token attribute from the HTTP request/response, or throws a
* runtime exception if it is not found.
*/
T token(String name);
/**
* Returns the value of the specified token attribute from the HTTP request/response, or the
* specified default value, if it is not found.
*/
T token(String name, T defaultValue);
/* REQUEST HEADERS: */
/**
* Gets the headers of the HTTP request.
*/
Map headers();
/**
* Returns the value of the specified header from the HTTP request, or throws a runtime exception if it is
* not found.
*/
String header(String name);
/**
* Returns the value of the specified header from the HTTP request, or the specified default value, if it is
* not found.
*/
String header(String name, String defaultValue);
/* REQUEST COOKIES: */
/**
* Gets the cookies of the HTTP request.
*/
Map cookies();
/**
* Returns the value of the specified cookie from the HTTP request, or throws a runtime exception if it is
* not found.
*/
String cookie(String name);
/**
* Returns the value of the specified cookie from the HTTP request, or the specified default value, if it is
* not found.
*/
String cookie(String name, String defaultValue);
/* RESPONSE: */
/**
* Gets the reference to the response object.
*/
Resp response();
/* ASYNCHRONOUS REQUEST HANDLING: */
/**
* Informs the HTTP server that the request will be handled asynchronously (typically on another thread). When the
* response is complete, the Req#done()
or Resp#done()
method must be called, to
* inform the server.
*/
Req async();
/**
* Is/was the request being handled in asynchronous mode?
*/
boolean isAsync();
/**
* Informs the HTTP server that the asynchronous handling has finished and the response is complete.
*/
Req done();
/**
* Has the request handling and response construction finished?
*/
boolean isDone();
/* WEB APPLICATION SETUP: */
/**
* Provides access to the HTTP routes of the web application setup.
*/
HttpRoutes routes();
/**
* Provides access to the matching HTTP route (if any) of the web application setup.
* In case a generic handler handles the request, or no matching route was found, null
is returned.
*/
Route route();
/**
* Provides access to the customization of the web application setup.
*/
Customization custom();
/**
* Reverts the previous processing of the request, usually with intention to process the same request again.
*/
void revert();
/**
* First renders the response headers, then returns an OutputStream representing
* the response body. The response body will be constructed by writing to the OutputStream.
*/
OutputStream out();
/**
* Gets the Content-Type
header of the HTTP response if it has been assigned,
* or the default value as configured in the HTTP route.
*/
MediaType contentType();
/**
* Returns the request handle, which is used when resuming the request handling in asynchronous way.
* See Resp#resume
.
*/
long handle();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy