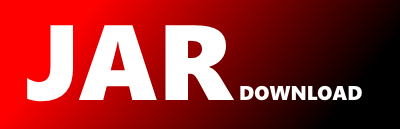
org.rapidpm.frp.reactive.CompletableFutureQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rapidpm-functional-reactive Show documentation
Show all versions of rapidpm-functional-reactive Show documentation
Functional Reactive with Core Java
/**
* Copyright © 2017 Sven Ruppert ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.rapidpm.frp.reactive;
import java.util.concurrent.CompletableFuture;
import java.util.function.Function;
import static java.util.concurrent.CompletableFuture.supplyAsync;
/**
* CompletableFutureQueue class.
*
* @author svenruppert
* @version $Id: $Id
*/
public class CompletableFutureQueue {
private Function> resultFunction;
private CompletableFutureQueue(Function> resultFunction) {
this.resultFunction = resultFunction;
}
/**
* define.
*
* @param transformation a {@link java.util.function.Function} object.
* @param a T object.
* @param a R object.
* @return a {@link org.rapidpm.frp.reactive.CompletableFutureQueue} object.
*/
public static CompletableFutureQueue define(Function transformation) {
return new CompletableFutureQueue<>(t -> CompletableFuture.completedFuture(transformation.apply(t)));
}
/**
* thenCombineAsync.
*
* @param nextTransformation a {@link java.util.function.Function} object.
* @param a N object.
* @return a {@link org.rapidpm.frp.reactive.CompletableFutureQueue} object.
*/
public CompletableFutureQueue thenCombineAsync(Function nextTransformation) {
final Function> f = this.resultFunction
.andThen(before -> before.thenComposeAsync(v -> supplyAsync(() -> nextTransformation.apply(v))));
return new CompletableFutureQueue<>(f);
}
//TODO : how to combine a list of CF ?
public CompletableFutureQueue thenCombineAsyncFromArray(Function[] nextTransformations) {
CompletableFutureQueue cfq = this;
for (Function nextTransformation : nextTransformations) {
cfq = cfq.thenCombineAsync(nextTransformation);
}
return cfq;
}
// public CompletableFutureQueue thenCombineAsync(Collection> nextTransformations) {
//
// nextTransformations
// .forEach(nextTransformation -> {
// this.resultFunction = this.resultFunction
// .andThen(before -> before.thenComposeAsync(v -> supplyAsync(() -> nextTransformation.apply(v))));
// });
//
//
// return new CompletableFutureQueue<>(this.resultFunction);
// }
//
//
//
//
// public CompletableFutureQueue thenCombineAsync(Function firstTransformation, Function... nextTransformations) {
// final Function> f = this.resultFunction
// .andThen(before -> before.thenComposeAsync(v -> supplyAsync(() -> firstTransformation.apply(v))));
//
// if (nextTransformations != null) {
// Arrays
// .stream(nextTransformations)
// .map(nf -> this.resultFunction.andThen(before -> before.thenComposeAsync(v -> supplyAsync(() -> nf.apply(v)))))
// .forEach(nF -> { /** don something **/ });
//
// }
// return new CompletableFutureQueue<>(f);
// }
/**
* resultFunction.
*
* @return a {@link java.util.function.Function} object.
*/
public Function> resultFunction() {
return this.resultFunction;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy