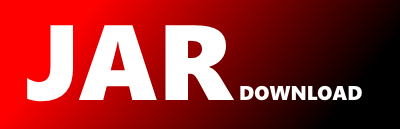
org.rcsb.strucmotif.update.Partition Maven / Gradle / Ivy
package org.rcsb.strucmotif.update;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* Partitions a collection into (roughly) equal-sized chunks. Will shuffle the original collection.
*
* code from: https://e.printstacktrace.blog/divide-a-list-to-lists-of-n-size-in-Java-8/
* @param type of the original collection
*/
public class Partition extends AbstractList> {
private final List list;
private final int chunkSize;
public Partition(Collection list, int chunkSize) {
this.list = new ArrayList<>(list);
// shuffle to prevent troublemakers such as ribosome and virus capsids occurring in the same chunk
Collections.shuffle(this.list);
this.chunkSize = chunkSize;
}
@Override
public List get(int index) {
int start = index * chunkSize;
int end = Math.min(start + chunkSize, list.size());
if (start > end) {
throw new IndexOutOfBoundsException("Index " + index + " is out of the list range <0," + (size() - 1) + ">");
}
return new ArrayList<>(list.subList(start, end));
}
@Override
public int size() {
return (int) Math.ceil((double) list.size() / (double) chunkSize);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy