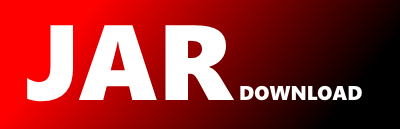
org.reactfx.BiEventStream Maven / Gradle / Ivy
package org.reactfx;
import static org.reactfx.util.Tuples.*;
import java.util.Optional;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
import java.util.function.Consumer;
import javafx.application.Platform;
import javafx.concurrent.Task;
import org.reactfx.util.Either;
import org.reactfx.util.Tuple2;
import org.reactfx.util.Tuple3;
public interface BiEventStream extends EventStream> {
Subscription subscribe(BiConsumer super A, ? super B> subscriber);
@Override
default Subscription subscribe(Consumer super Tuple2> subscriber) {
return subscribe((a, b) -> subscriber.accept(t(a, b)));
}
default Subscription watch(
BiConsumer super A, ? super B> subscriber,
Consumer super Throwable> monitor) {
return monitor(monitor).and(subscribe(subscriber));
}
default Subscription feedTo2(BiEventSink super A, ? super B> sink) {
return subscribe((a, b) -> sink.push(a, b));
}
default BiEventStream hook(
BiConsumer super A, ? super B> sideEffect) {
return new SideEffectBiStream<>(this, sideEffect);
}
default BiEventStream filter(
BiPredicate super A, ? super B> predicate) {
return new FilterBiStream<>(this, predicate);
}
@Override
default BiEventStream distinct() {
return new DistinctBiStream<>(this);
}
default EventStream map(
BiFunction super A, ? super B, ? extends U> f) {
return new MappedBiStream<>(this, f);
}
default BiEventStream mapToBi(BiFunction super A, ? super B, Tuple2> f) {
return new MappedBiToBiStream<>(this, f);
}
default TriEventStream mapToTri(BiFunction super A, ? super B, Tuple3> f) {
return new MappedBiToTriStream<>(this, f);
}
/**
* @deprecated See deprecation comment at {@link EitherEventStream}.
*/
@Deprecated
default EitherEventStream split(
BiFunction super A, ? super B, Either> f) {
return new MappedToEitherBiStream<>(this, f);
}
default CompletionStageStream mapToCompletionStage(
BiFunction super A, ? super B, CompletionStage> f) {
return new MappedToCompletionStageBiStream<>(this, f);
}
default TaskStream mapToTask(
BiFunction super A, ? super B, Task> f) {
return new MappedToTaskBiStream<>(this, f);
}
default EventStream filterMap(
BiPredicate super A, ? super B> predicate,
BiFunction super A, ? super B, ? extends U> f) {
return new FilterMapBiStream<>(this, predicate, f);
}
default EventStream filterMap(BiFunction super A, ? super B, Optional> f) {
return filterMap(t -> f.apply(t._1, t._2));
}
default EventStream flatMap(BiFunction super A, ? super B, ? extends EventStream> f) {
return flatMap(t -> f.apply(t._1, t._2));
}
/**
* @deprecated Since 1.2.1. Renamed to {@link #filterMap(BiFunction)}.
*/
@Deprecated
default EventStream flatMapOpt(BiFunction super A, ? super B, Optional> f) {
return flatMapOpt(t -> f.apply(t._1, t._2));
}
@Override
default BiEventStream emitOn(EventStream> impulse) {
return new EmitOnBiStream<>(this, impulse);
}
@Override
default BiEventStream emitOnEach(EventStream> impulse) {
return new EmitOnEachBiStream<>(this, impulse);
}
default TriEventStream emitAllOnEach(EventStream impulse) {
return new EmitAll3OnEachStream<>(this, impulse);
}
@Override
default BiEventStream repeatOn(EventStream> impulse) {
return new RepeatOnBiStream<>(this, impulse);
}
@Deprecated
@Override
default InterceptableBiEventStream interceptable() {
if(this instanceof InterceptableBiEventStream) {
return (InterceptableBiEventStream) this;
} else {
return new InterceptableBiEventStreamImpl(this);
}
}
@Override
default BiEventStream threadBridge(
Executor sourceThreadExecutor,
Executor targetThreadExecutor) {
return new BiThreadBridge(this, sourceThreadExecutor, targetThreadExecutor);
}
@Override
default BiEventStream threadBridgeFromFx(Executor targetThreadExecutor) {
return threadBridge(Platform::runLater, targetThreadExecutor);
}
@Override
default BiEventStream threadBridgeToFx(Executor sourceThreadExecutor) {
return threadBridge(sourceThreadExecutor, Platform::runLater);
}
@Override
default BiEventStream guardedBy(Guardian... guardians) {
return new GuardedBiStream<>(this, guardians);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy