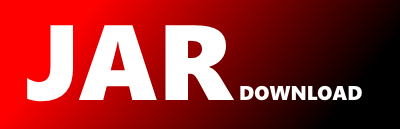
org.reactfx.DistinctStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reactfx Show documentation
Show all versions of reactfx Show documentation
Reactive event streams for JavaFX
package org.reactfx;
import java.util.Objects;
import org.reactfx.util.Either;
class DistinctStream extends LazilyBoundStream {
static final Object NONE = new Object();
private final EventStream input;
private Object previous = NONE;
public DistinctStream(EventStream input) {
this.input = input;
}
@Override
protected Subscription subscribeToInputs() {
return subscribeTo(input, value -> {
Object prevToCompare = previous;
previous = value;
if (!Objects.equals(value, prevToCompare)) {
emit(value);
}
});
}
}
class DistinctBiStream extends LazilyBoundBiStream {
private final BiEventStream input;
private Object previousA = DistinctStream.NONE;
private Object previousB = DistinctStream.NONE;
DistinctBiStream(BiEventStream input) {
this.input = input;
}
@Override
protected Subscription subscribeToInputs() {
return subscribeToBi(input, (a, b) -> {
Object aPrevToCompare = previousA;
previousA = a;
Object bPrevToCompare = previousB;
previousB = b;
if (Objects.equals(a, aPrevToCompare) && Objects.equals(b, bPrevToCompare)) {
return;
}
emit(a, b);
});
}
}
class DistinctTriStream extends LazilyBoundTriStream {
private final TriEventStream input;
private Object previousA = DistinctStream.NONE;
private Object previousB = DistinctStream.NONE;
private Object previousC = DistinctStream.NONE;
DistinctTriStream(TriEventStream input) {
this.input = input;
}
@Override
protected Subscription subscribeToInputs() {
return subscribeToTri(input, (a, b, c) -> {
Object aPrevToCompare = previousA;
previousA = a;
Object bPrevToCompare = previousB;
previousB = b;
Object cPrevToCompare = previousC;
previousC = c;
if (Objects.equals(a, aPrevToCompare) &&
Objects.equals(b, bPrevToCompare) &&
Objects.equals(c, cPrevToCompare)) {
return;
}
emit(a, b, c);
});
}
}
@Deprecated
class DistinctEitherStream
extends DistinctStream>
implements EitherEventStream {
public DistinctEitherStream(EventStream> input) {
super(input);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy