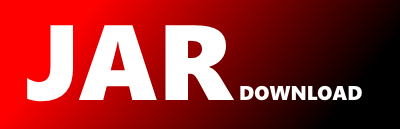
org.reactfx.LatestNStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of reactfx Show documentation
Show all versions of reactfx Show documentation
Reactive event streams for JavaFX
package org.reactfx;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import org.reactfx.util.Lists;
public class LatestNStream extends EventStreamBase> {
private final EventStream source;
private final int n;
private List first = null;
private List second = null;
private List concatView = null;
public LatestNStream(EventStream source, int n) {
if(n <= 0) {
throw new IllegalArgumentException("n must be positive. Was " + n);
}
this.source = source;
this.n = n;
}
@Override
protected Subscription observeInputs() {
first = Collections.emptyList();
second = new ArrayList<>(n);
concatView = Lists.concatView(first, second);
return source.subscribe(this::onEvent);
}
private void onEvent(T event) {
if(second.size() == n) {
first = second;
second = new ArrayList<>(n);
concatView = Lists.concatView(first, second);
}
second.add(event);
int total = concatView.size();
emit(concatView.subList(Math.max(0, total - n), total));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy