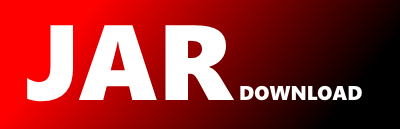
org.reactfx.collection.MappedList Maven / Gradle / Ivy
package org.reactfx.collection;
import java.util.List;
import java.util.function.Function;
import javafx.beans.value.ObservableValue;
import javafx.collections.ObservableList;
import org.reactfx.Subscription;
import org.reactfx.util.Lists;
import org.reactfx.value.Val;
class MappedList extends LiveListBase
implements ReadOnlyLiveListImpl {
private final ObservableList extends E> source;
private final Function super E, ? extends F> mapper;
public MappedList(
ObservableList extends E> source,
Function super E, ? extends F> mapper) {
this.source = source;
this.mapper = mapper;
}
@Override
public F get(int index) {
return mapper.apply(source.get(index));
}
@Override
public int size() {
return source.size();
}
@Override
protected Subscription observeInputs() {
return LiveList.observeQuasiChanges(source, this::sourceChanged);
}
private void sourceChanged(QuasiListChange extends E> change) {
notifyObservers(mappedChangeView(change, mapper));
}
static QuasiListChange mappedChangeView(
QuasiListChange extends E> change,
Function super E, ? extends F> mapper) {
return new QuasiListChange() {
@Override
public List extends QuasiListModification extends F>> getModifications() {
List extends QuasiListModification extends E>> mods = change.getModifications();
return Lists., QuasiListModification>mappedView(mods, mod -> new QuasiListModification() {
@Override
public int getFrom() {
return mod.getFrom();
}
@Override
public int getAddedSize() {
return mod.getAddedSize();
}
@Override
public List extends F> getRemoved() {
return Lists.mappedView(mod.getRemoved(), mapper);
}
});
}
};
}
}
class DynamicallyMappedList extends LiveListBase
implements ReadOnlyLiveListImpl {
private final ObservableList extends E> source;
private final Val extends Function super E, ? extends F>> mapper;
public DynamicallyMappedList(
ObservableList extends E> source,
ObservableValue extends Function super E, ? extends F>> mapper) {
this.source = source;
this.mapper = Val.wrap(mapper);
}
@Override
public F get(int index) {
return mapper.getValue().apply(source.get(index));
}
@Override
public int size() {
return source.size();
}
@Override
protected Subscription observeInputs() {
return Subscription.multi(
LiveList.observeQuasiChanges(source, this::sourceChanged),
mapper.observeInvalidations(this::mapperInvalidated));
}
private void sourceChanged(QuasiListChange extends E> change) {
notifyObservers(MappedList.mappedChangeView(change, mapper.getValue()));
}
private void mapperInvalidated(Function super E, ? extends F> oldMapper) {
fireContentReplacement(Lists.mappedView(source, oldMapper));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy