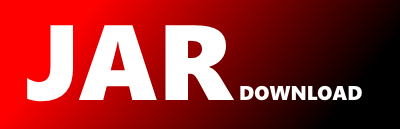
org.reactivecommons.async.commons.HandlerResolver Maven / Gradle / Ivy
package org.reactivecommons.async.commons;
import lombok.RequiredArgsConstructor;
import lombok.extern.java.Log;
import org.reactivecommons.async.api.handlers.registered.RegisteredCommandHandler;
import org.reactivecommons.async.api.handlers.registered.RegisteredEventListener;
import org.reactivecommons.async.api.handlers.registered.RegisteredQueryHandler;
import org.reactivecommons.async.commons.utils.matcher.KeyMatcher;
import org.reactivecommons.async.commons.utils.matcher.Matcher;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
@Log
@RequiredArgsConstructor
public class HandlerResolver {
private final Map> queryHandlers;
private final Map> eventListeners;
private final Map> eventsToBind;
private final Map> eventNotificationListeners;
private final Map> commandHandlers;
private final Matcher matcher = new KeyMatcher();
@SuppressWarnings("unchecked")
public RegisteredQueryHandler getQueryHandler(String path) {
return (RegisteredQueryHandler) queryHandlers
.computeIfAbsent(path, getMatchHandler(queryHandlers));
}
@SuppressWarnings("unchecked")
public RegisteredCommandHandler getCommandHandler(String path) {
return (RegisteredCommandHandler) commandHandlers
.computeIfAbsent(path, getMatchHandler(commandHandlers));
}
@SuppressWarnings("unchecked")
public RegisteredEventListener getEventListener(String path) {
if (eventListeners.containsKey(path)) {
return (RegisteredEventListener) eventListeners.get(path);
}
return (RegisteredEventListener) getMatchHandler(eventListeners).apply(path);
}
public Collection> getNotificationListeners() {
return eventNotificationListeners.values();
}
@SuppressWarnings("unchecked")
public RegisteredEventListener getNotificationListener(String path) {
return (RegisteredEventListener) eventNotificationListeners
.computeIfAbsent(path, getMatchHandler(eventNotificationListeners));
}
// Returns only the listenEvent not the handleDynamicEvents
public Collection> getEventListeners() {
return eventsToBind.values();
}
public List getEventNames() {
return List.copyOf(eventListeners.keySet());
}
public List getNotificationNames() {
return List.copyOf(eventNotificationListeners.keySet());
}
public void addEventListener(RegisteredEventListener, ?> listener) {
eventListeners.put(listener.getPath(), listener);
}
public void addQueryHandler(RegisteredQueryHandler, ?> handler) {
if (handler.getPath().contains("*") || handler.getPath().contains("#")) {
throw new RuntimeException("avoid * or # in dynamic handlers, make sure you have no conflicts with cached patterns");
}
queryHandlers.put(handler.getPath(), handler);
}
private Function getMatchHandler(Map handlers) {
return name -> {
String matched = matcher.match(handlers.keySet(), name);
return handlers.get(matched);
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy