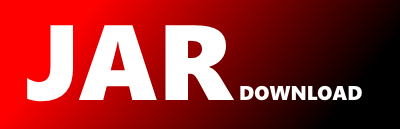
arez.annotations.Reference Maven / Gradle / Ivy
package arez.annotations;
import arez.Locator;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Target;
import javax.annotation.Nonnull;
/**
* A Reference annotation is placed on an abstract method that will resolve to a referenced object.
* The reference annotation is paired with a {@link ReferenceId} method that returns the id
* of the referenced object. The type
of the referenced object is the return type of the method
* annotated with @Reference
. The type
and id
are passed to the
* {@link Locator#findById(Class, Object)} method when the reference is resolved.
*
* * The reference can be resolved either eagerly (during a linking phase or when it is modified) or
* lazily (when accessed).
*
* The method must also conform to the following constraints:
*
* - Must not be annotated with any other arez annotation
* - Must have 0 parameters
* - Must return a value
* - Must be abstract
* - Must not throw exceptions
* - Must be accessible to the class annotated by the {@link ArezComponent} annotation.
*
*/
@Documented
@Target( ElementType.METHOD )
public @interface Reference
{
/**
* Return the name of the reference relative to the component. The value must conform
* to the requirements of a java identifier. If not specified, the name will be derived by assuming
* the naming convention "get[Name]" or failing that the name will be the method name.
*
* @return the name of the reference relative to the component.
*/
@Nonnull
String name() default "";
/**
* Return the strategy for resolving reference.
*
* @return the strategy for resolving reference.
*/
@Nonnull
LinkType load() default LinkType.EAGER;
/**
* Return the enum controlling whether there is an inverse for reference.
* {@link Feature#ENABLE} tells the annotation processor to expect an inverse and add code
* to maintain the inverse. {@link Feature#DISABLE} will generate no code to maintain
* inverse module. {@link Feature#AUTODETECT} will be treated as {@link Feature#ENABLE}
* if either the {@link #inverseName} or {@link #inverseMultiplicity} is specified.
*
* @return the enum controlling whether there is an inverse for reference
*/
@Nonnull
Feature inverse() default Feature.AUTODETECT;
/**
* Return the name of the inverse associated with the reference. The value must conform
* to the requirements of a java identifier. If not specified, the name will be derived
* by camelCasing the simple name of the class on which the {@link Reference} annotation
* is placed and then adding an s if {@link #inverseMultiplicity} is {@link Multiplicity#MANY}.
*
* @return the name of the reference relative to the component.
*/
@Nonnull
String inverseName() default "";
/**
* Define the expected multiplicity of the inverse associated with the reference.
*
* @return the expected multiplicity of the inverse associated with the reference.
*/
@Nonnull
Multiplicity inverseMultiplicity() default Multiplicity.MANY;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy