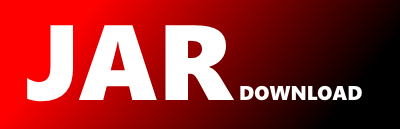
elemental2.core.JsMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elemental2-core Show documentation
Show all versions of elemental2-core Show documentation
Thin Java abstractions for the standard built-in objects for Javascript.
package elemental2.core;
import jsinterop.annotations.JsFunction;
import jsinterop.annotations.JsOverlay;
import jsinterop.annotations.JsPackage;
import jsinterop.annotations.JsType;
import jsinterop.base.Js;
@JsType(isNative = true, name = "Map", namespace = JsPackage.GLOBAL)
public class JsMap
implements JsIterable[]> {
@JsType(isNative = true, name = "?", namespace = JsPackage.GLOBAL)
public interface ConstructorIterableJsIterableTypeParameterArrayUnionType {
@JsOverlay
static JsMap.ConstructorIterableJsIterableTypeParameterArrayUnionType of(Object o) {
return Js.cast(o);
}
@JsOverlay
default KEY asKEY() {
return Js.cast(this);
}
@JsOverlay
default VALUE asVALUE() {
return Js.cast(this);
}
}
@JsType(isNative = true, name = "?", namespace = JsPackage.GLOBAL)
public interface ConstructorIterableUnionType {
@JsOverlay
static JsMap.ConstructorIterableUnionType of(Object o) {
return Js.cast(o);
}
@JsOverlay
default JsMap.ConstructorIterableJsIterableTypeParameterArrayUnionType[][]
asConstructorIterableArrayArrayUnionTypeArrayArray() {
return Js.cast(this);
}
@JsOverlay
default JsIterable[]>
asJsIterable() {
return Js.cast(this);
}
@JsOverlay
default boolean isConstructorIterableArrayArrayUnionTypeArrayArray() {
return (Object) this instanceof Object[];
}
}
@JsType(isNative = true, name = "?", namespace = JsPackage.GLOBAL)
public interface EntriesJsIteratorIterableTypeParameterArrayUnionType {
@JsOverlay
static JsMap.EntriesJsIteratorIterableTypeParameterArrayUnionType of(Object o) {
return Js.cast(o);
}
@JsOverlay
default KEY asKEY() {
return Js.cast(this);
}
@JsOverlay
default VALUE asVALUE() {
return Js.cast(this);
}
}
@JsFunction
public interface ForEachCallbackFn {
Object onInvoke(VALUE p0, KEY p1, JsMap extends KEY, ? extends VALUE> p2);
}
@JsType(isNative = true, name = "?", namespace = JsPackage.GLOBAL)
public interface JsIterableTypeParameterArrayUnionType {
@JsOverlay
static JsMap.JsIterableTypeParameterArrayUnionType of(Object o) {
return Js.cast(o);
}
@JsOverlay
default KEY asKEY() {
return Js.cast(this);
}
@JsOverlay
default VALUE asVALUE() {
return Js.cast(this);
}
}
public int size;
public JsMap() {}
public JsMap(
JsMap.ConstructorIterableJsIterableTypeParameterArrayUnionType[][] iterable) {}
public JsMap(JsMap.ConstructorIterableUnionType iterable) {}
public JsMap(
JsIterable[]>
iterable) {}
public native void clear();
public native boolean delete(KEY key);
public native JsIteratorIterable<
JsMap.EntriesJsIteratorIterableTypeParameterArrayUnionType[]>
entries();
public native Object forEach(
JsMap.ForEachCallbackFn super KEY, ? super VALUE> callback, Object thisArg);
public native Object forEach(JsMap.ForEachCallbackFn super KEY, ? super VALUE> callback);
public native VALUE get(KEY key);
public native boolean has(KEY key);
public native JsIteratorIterable keys();
public native JsMap set(KEY key, VALUE value);
public native JsIteratorIterable values();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy