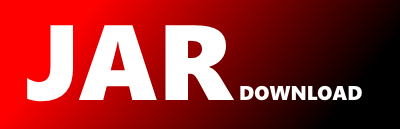
org.realityforge.replicant.client.EntitySubscriptionManagerImpl Maven / Gradle / Ivy
package org.realityforge.replicant.client;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.inject.Inject;
/**
* A class that records the subscriptions to graphs and entities.
*/
public class EntitySubscriptionManagerImpl
implements EntitySubscriptionManager
{
//Graph => InstanceID
private final HashMap> _instanceSubscriptions = new HashMap<>();
//Graph => Type
private final HashMap _typeSubscriptions = new HashMap<>();
// Entity map: Type => ID
private final HashMap, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy