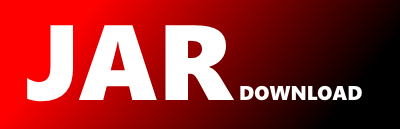
org.redisson.misc.PromiseDelegator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson-all Show documentation
Show all versions of redisson-all Show documentation
Easy Redis Java client and Real-Time Data Platform. Valkey compatible. Sync/Async/RxJava3/Reactive API. Client side caching. Over 50 Redis based Java objects and services: JCache API, Apache Tomcat, Hibernate, Spring, Set, Multimap, SortedSet, Map, List, Queue, Deque, Semaphore, Lock, AtomicLong, Map Reduce, Bloom filter, Scheduler, RPC
/**
* Copyright (c) 2013-2020 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.misc;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Executor;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
/**
*
* @author Nikita Koksharov
*
* @param type
*/
public class PromiseDelegator implements RPromise {
private final RPromise promise;
public PromiseDelegator(RPromise promise) {
super();
this.promise = promise;
}
public RPromise getInnerPromise() {
return promise;
}
public T join() {
return promise.join();
}
public boolean isSuccess() {
return promise.isSuccess();
}
public boolean trySuccess(T result) {
return promise.trySuccess(result);
}
public Throwable cause() {
return promise.cause();
}
public T getNow() {
return promise.getNow();
}
public boolean tryFailure(Throwable cause) {
return promise.tryFailure(cause);
}
public boolean await(long timeout, TimeUnit unit) throws InterruptedException {
return promise.await(timeout, unit);
}
public boolean setUncancellable() {
return promise.setUncancellable();
}
public boolean await(long timeoutMillis) throws InterruptedException {
return promise.await(timeoutMillis);
}
public RPromise await() throws InterruptedException {
return promise.await();
}
public boolean cancel(boolean mayInterruptIfRunning) {
return promise.cancel(mayInterruptIfRunning);
}
public RPromise awaitUninterruptibly() {
return promise.awaitUninterruptibly();
}
public RPromise sync() throws InterruptedException {
return promise.sync();
}
public RPromise syncUninterruptibly() {
return promise.syncUninterruptibly();
}
public boolean isCancelled() {
return promise.isCancelled();
}
public boolean isDone() {
return promise.isDone();
}
public T get() throws InterruptedException, ExecutionException {
return promise.get();
}
public boolean awaitUninterruptibly(long timeout, TimeUnit unit) {
return promise.awaitUninterruptibly(timeout, unit);
}
public T get(long timeout, TimeUnit unit) throws InterruptedException, ExecutionException, TimeoutException {
return promise.get(timeout, unit);
}
public CompletionStage thenApply(Function super T, ? extends U> fn) {
return promise.thenApply(fn);
}
public boolean awaitUninterruptibly(long timeoutMillis) {
return promise.awaitUninterruptibly(timeoutMillis);
}
public CompletionStage thenApplyAsync(Function super T, ? extends U> fn) {
return promise.thenApplyAsync(fn);
}
public CompletionStage thenApplyAsync(Function super T, ? extends U> fn, Executor executor) {
return promise.thenApplyAsync(fn, executor);
}
public CompletionStage thenAccept(Consumer super T> action) {
return promise.thenAccept(action);
}
public CompletionStage thenAcceptAsync(Consumer super T> action) {
return promise.thenAcceptAsync(action);
}
@Override
public boolean hasListeners() {
return promise.hasListeners();
}
public CompletionStage thenAcceptAsync(Consumer super T> action, Executor executor) {
return promise.thenAcceptAsync(action, executor);
}
public CompletionStage thenRun(Runnable action) {
return promise.thenRun(action);
}
public CompletionStage thenRunAsync(Runnable action) {
return promise.thenRunAsync(action);
}
public CompletionStage thenRunAsync(Runnable action, Executor executor) {
return promise.thenRunAsync(action, executor);
}
public CompletionStage thenCombine(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn) {
return promise.thenCombine(other, fn);
}
public CompletionStage thenCombineAsync(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn) {
return promise.thenCombineAsync(other, fn);
}
public CompletionStage thenCombineAsync(CompletionStage extends U> other,
BiFunction super T, ? super U, ? extends V> fn, Executor executor) {
return promise.thenCombineAsync(other, fn, executor);
}
public CompletionStage thenAcceptBoth(CompletionStage extends U> other,
BiConsumer super T, ? super U> action) {
return promise.thenAcceptBoth(other, action);
}
public CompletionStage thenAcceptBothAsync(CompletionStage extends U> other,
BiConsumer super T, ? super U> action) {
return promise.thenAcceptBothAsync(other, action);
}
public CompletionStage thenAcceptBothAsync(CompletionStage extends U> other,
BiConsumer super T, ? super U> action, Executor executor) {
return promise.thenAcceptBothAsync(other, action, executor);
}
public CompletionStage runAfterBoth(CompletionStage> other, Runnable action) {
return promise.runAfterBoth(other, action);
}
public CompletionStage runAfterBothAsync(CompletionStage> other, Runnable action) {
return promise.runAfterBothAsync(other, action);
}
public CompletionStage runAfterBothAsync(CompletionStage> other, Runnable action, Executor executor) {
return promise.runAfterBothAsync(other, action, executor);
}
public CompletionStage applyToEither(CompletionStage extends T> other, Function super T, U> fn) {
return promise.applyToEither(other, fn);
}
public CompletionStage applyToEitherAsync(CompletionStage extends T> other, Function super T, U> fn) {
return promise.applyToEitherAsync(other, fn);
}
public CompletionStage applyToEitherAsync(CompletionStage extends T> other, Function super T, U> fn,
Executor executor) {
return promise.applyToEitherAsync(other, fn, executor);
}
public CompletionStage acceptEither(CompletionStage extends T> other, Consumer super T> action) {
return promise.acceptEither(other, action);
}
public CompletionStage acceptEitherAsync(CompletionStage extends T> other, Consumer super T> action) {
return promise.acceptEitherAsync(other, action);
}
public CompletionStage acceptEitherAsync(CompletionStage extends T> other, Consumer super T> action,
Executor executor) {
return promise.acceptEitherAsync(other, action, executor);
}
public CompletionStage runAfterEither(CompletionStage> other, Runnable action) {
return promise.runAfterEither(other, action);
}
public CompletionStage runAfterEitherAsync(CompletionStage> other, Runnable action) {
return promise.runAfterEitherAsync(other, action);
}
public CompletionStage runAfterEitherAsync(CompletionStage> other, Runnable action, Executor executor) {
return promise.runAfterEitherAsync(other, action, executor);
}
public CompletionStage thenCompose(Function super T, ? extends CompletionStage> fn) {
return promise.thenCompose(fn);
}
public CompletionStage thenComposeAsync(Function super T, ? extends CompletionStage> fn) {
return promise.thenComposeAsync(fn);
}
public CompletionStage thenComposeAsync(Function super T, ? extends CompletionStage> fn,
Executor executor) {
return promise.thenComposeAsync(fn, executor);
}
public CompletionStage exceptionally(Function fn) {
return promise.exceptionally(fn);
}
public CompletionStage whenComplete(BiConsumer super T, ? super Throwable> action) {
return promise.whenComplete(action);
}
public CompletionStage whenCompleteAsync(BiConsumer super T, ? super Throwable> action) {
return promise.whenCompleteAsync(action);
}
public CompletionStage whenCompleteAsync(BiConsumer super T, ? super Throwable> action, Executor executor) {
return promise.whenCompleteAsync(action, executor);
}
public CompletionStage handle(BiFunction super T, Throwable, ? extends U> fn) {
return promise.handle(fn);
}
public CompletionStage handleAsync(BiFunction super T, Throwable, ? extends U> fn) {
return promise.handleAsync(fn);
}
public CompletionStage handleAsync(BiFunction super T, Throwable, ? extends U> fn, Executor executor) {
return promise.handleAsync(fn, executor);
}
public CompletableFuture toCompletableFuture() {
return promise.toCompletableFuture();
}
@Override
public void onComplete(BiConsumer super T, ? super Throwable> action) {
promise.onComplete(action);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy