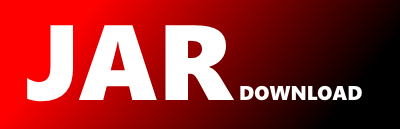
org.redisson.api.LocalCachedMapOptions Maven / Gradle / Ivy
Show all versions of redisson-all Show documentation
/**
* Copyright (c) 2013-2021 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import java.util.concurrent.TimeUnit;
import org.redisson.api.map.MapLoader;
import org.redisson.api.map.MapWriter;
/**
* Configuration for LocalCachedMap object.
*
* @author Nikita Koksharov
*
* @param key type
* @param value type
*/
public class LocalCachedMapOptions extends MapOptions {
/**
* Various strategies to avoid stale objects in local cache.
* Handle cases when map instance has been disconnected for a while.
*
*/
public enum ReconnectionStrategy {
/**
* No reconnect handling.
*/
NONE,
/**
* Clear local cache if map instance disconnected.
*/
CLEAR,
/**
* Store invalidated entry hash in invalidation log for 10 minutes.
* Cache keys for stored invalidated entry hashes will be removed
* if LocalCachedMap instance has been disconnected less than 10 minutes
* or whole local cache will be cleaned otherwise.
*/
LOAD
}
public enum SyncStrategy {
/**
* No synchronizations on map changes.
*/
NONE,
/**
* Invalidate local cache entry across all LocalCachedMap instances on map entry change. Broadcasts map entry hash (16 bytes) to all instances.
*/
INVALIDATE,
/**
* Update local cache entry across all LocalCachedMap instances on map entry change. Broadcasts full map entry state (Key and Value objects) to all instances.
*/
UPDATE
}
public enum EvictionPolicy {
/**
* Local cache without eviction.
*/
NONE,
/**
* Least Recently Used local cache.
*/
LRU,
/**
* Least Frequently Used local cache.
*/
LFU,
/**
* Local cache with Soft Reference used for values.
* All references will be collected by GC
*/
SOFT,
/**
* Local cache with Weak Reference used for values.
* All references will be collected by GC
*/
WEAK
};
public enum CacheProvider {
REDISSON,
CAFFEINE
}
public enum StoreMode {
/**
* Store data only in local cache.
*/
LOCALCACHE,
/**
* Store data only in both Redis and local cache.
*/
LOCALCACHE_REDIS
}
private ReconnectionStrategy reconnectionStrategy;
private SyncStrategy syncStrategy;
private EvictionPolicy evictionPolicy;
private int cacheSize;
private long timeToLiveInMillis;
private long maxIdleInMillis;
private CacheProvider cacheProvider;
private StoreMode storeMode;
private boolean storeCacheMiss;
protected LocalCachedMapOptions() {
}
protected LocalCachedMapOptions(LocalCachedMapOptions copy) {
this.reconnectionStrategy = copy.reconnectionStrategy;
this.syncStrategy = copy.syncStrategy;
this.evictionPolicy = copy.evictionPolicy;
this.cacheSize = copy.cacheSize;
this.timeToLiveInMillis = copy.timeToLiveInMillis;
this.maxIdleInMillis = copy.maxIdleInMillis;
this.cacheProvider = copy.cacheProvider;
this.storeMode = copy.storeMode;
this.storeCacheMiss = copy.storeCacheMiss;
}
/**
* Creates a new instance of LocalCachedMapOptions with default options.
*
* This is equivalent to:
*
* new LocalCachedMapOptions()
* .cacheSize(0).timeToLive(0).maxIdle(0)
* .evictionPolicy(EvictionPolicy.NONE)
* .reconnectionStrategy(ReconnectionStrategy.NONE)
* .cacheProvider(CacheProvider.REDISSON)
* .syncStrategy(SyncStrategy.INVALIDATE)
* .storeCacheMiss(false);
*
*
* @param key type
* @param value type
*
* @return LocalCachedMapOptions instance
*
*/
public static LocalCachedMapOptions defaults() {
return new LocalCachedMapOptions()
.cacheSize(0).timeToLive(0).maxIdle(0)
.evictionPolicy(EvictionPolicy.NONE)
.reconnectionStrategy(ReconnectionStrategy.NONE)
.cacheProvider(CacheProvider.REDISSON)
.storeMode(StoreMode.LOCALCACHE_REDIS)
.syncStrategy(SyncStrategy.INVALIDATE)
.storeCacheMiss(false);
}
public CacheProvider getCacheProvider() {
return cacheProvider;
}
public EvictionPolicy getEvictionPolicy() {
return evictionPolicy;
}
public int getCacheSize() {
return cacheSize;
}
public long getTimeToLiveInMillis() {
return timeToLiveInMillis;
}
public long getMaxIdleInMillis() {
return maxIdleInMillis;
}
/**
* Defines local cache size. If size is 0
then local cache is unbounded.
*
* @param cacheSize - size of cache
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions cacheSize(int cacheSize) {
this.cacheSize = cacheSize;
return this;
}
public ReconnectionStrategy getReconnectionStrategy() {
return reconnectionStrategy;
}
public SyncStrategy getSyncStrategy() {
return syncStrategy;
}
public LocalCachedMapOptions reconnectionStrategy(ReconnectionStrategy reconnectionStrategy) {
if (reconnectionStrategy == null) {
throw new NullPointerException("reconnectionStrategy can't be null");
}
this.reconnectionStrategy = reconnectionStrategy;
return this;
}
public LocalCachedMapOptions syncStrategy(SyncStrategy syncStrategy) {
if (syncStrategy == null) {
throw new NullPointerException("syncStrategy can't be null");
}
this.syncStrategy = syncStrategy;
return this;
}
/**
* Defines local cache eviction policy.
*
* @param evictionPolicy
* LRU
- uses local cache with LRU (least recently used) eviction policy.
*
LFU
- uses local cache with LFU (least frequently used) eviction policy.
*
SOFT
- uses local cache with soft references. The garbage collector will evict items from the local cache when the JVM is running out of memory.
*
WEAK
- uses local cache with weak references. The garbage collector will evict items from the local cache when it became weakly reachable.
*
NONE
- doesn't use eviction policy, but timeToLive and maxIdleTime params are still working.
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions evictionPolicy(EvictionPolicy evictionPolicy) {
if (evictionPolicy == null) {
throw new NullPointerException("evictionPolicy can't be null");
}
this.evictionPolicy = evictionPolicy;
return this;
}
/**
* Defines time to live in milliseconds of each map entry in local cache.
* If value equals to 0
then timeout is not applied
*
* @param timeToLiveInMillis - time to live in milliseconds
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions timeToLive(long timeToLiveInMillis) {
this.timeToLiveInMillis = timeToLiveInMillis;
return this;
}
/**
* Defines time to live of each map entry in local cache.
* If value equals to 0
then timeout is not applied
*
* @param timeToLive - time to live
* @param timeUnit - time unit
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions timeToLive(long timeToLive, TimeUnit timeUnit) {
return timeToLive(timeUnit.toMillis(timeToLive));
}
/**
* Defines max idle time in milliseconds of each map entry in local cache.
* If value equals to 0
then timeout is not applied
*
* @param maxIdleInMillis - time to live in milliseconds
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions maxIdle(long maxIdleInMillis) {
this.maxIdleInMillis = maxIdleInMillis;
return this;
}
/**
* Defines max idle time of each map entry in local cache.
* If value equals to 0
then timeout is not applied
*
* @param maxIdle - max idle time
* @param timeUnit - time unit
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions maxIdle(long maxIdle, TimeUnit timeUnit) {
return maxIdle(timeUnit.toMillis(maxIdle));
}
public StoreMode getStoreMode() {
return storeMode;
}
/**
* Defines store mode of cache data.
*
* @param storeMode
* LOCALCACHE
- store data in local cache only.
*
LOCALCACHE_REDIS
- store data in both Redis and local cache.
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions storeMode(StoreMode storeMode) {
this.storeMode = storeMode;
return this;
}
/**
* Defines Cache provider used as local cache store.
*
* @param cacheProvider
* REDISSON
- uses Redisson own implementation.
*
CAFFEINE
- uses Caffeine implementation.
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions cacheProvider(CacheProvider cacheProvider) {
this.cacheProvider = cacheProvider;
return this;
}
public boolean isStoreCacheMiss() {
return this.storeCacheMiss;
}
/**
* Defines whether to store a cache miss into the local cache.
*
* @param storeCacheMiss - whether to store a cache miss into the local cache
* @return LocalCachedMapOptions instance
*/
public LocalCachedMapOptions storeCacheMiss(boolean storeCacheMiss) {
this.storeCacheMiss = storeCacheMiss;
return this;
}
@Override
public LocalCachedMapOptions writeBehindBatchSize(int writeBehindBatchSize) {
return (LocalCachedMapOptions) super.writeBehindBatchSize(writeBehindBatchSize);
}
@Override
public LocalCachedMapOptions writeBehindDelay(int writeBehindDelay) {
return (LocalCachedMapOptions) super.writeBehindDelay(writeBehindDelay);
}
@Override
public LocalCachedMapOptions writer(MapWriter writer) {
return (LocalCachedMapOptions) super.writer(writer);
}
@Override
public LocalCachedMapOptions writeMode(org.redisson.api.MapOptions.WriteMode writeMode) {
return (LocalCachedMapOptions) super.writeMode(writeMode);
}
@Override
public LocalCachedMapOptions loader(MapLoader loader) {
return (LocalCachedMapOptions) super.loader(loader);
}
}