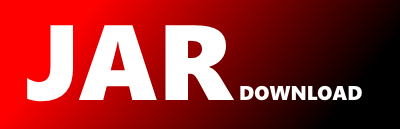
org.redisson.RedissonLexSortedSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson-all Show documentation
Show all versions of redisson-all Show documentation
Easy Redis Java client and Real-Time Data Platform. Valkey compatible. Sync/Async/RxJava3/Reactive API. Client side caching. Over 50 Redis based Java objects and services: JCache API, Apache Tomcat, Hibernate, Spring, Set, Multimap, SortedSet, Map, List, Queue, Deque, Semaphore, Lock, AtomicLong, Map Reduce, Bloom filter, Scheduler, RPC
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import java.util.SortedSet;
import org.redisson.api.RFuture;
import org.redisson.api.RLexSortedSet;
import org.redisson.api.RedissonClient;
import org.redisson.client.codec.StringCodec;
import org.redisson.client.protocol.RedisCommands;
import org.redisson.command.CommandAsyncExecutor;
import org.redisson.misc.CompletableFutureWrapper;
/**
* Sorted set contained values of String type
*
* @author Nikita Koksharov
*
*/
public class RedissonLexSortedSet extends RedissonScoredSortedSet implements RLexSortedSet {
public RedissonLexSortedSet(CommandAsyncExecutor commandExecutor, String name, RedissonClient redisson) {
super(StringCodec.INSTANCE, commandExecutor, name, redisson);
}
@Override
public int removeRange(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive) {
return get(removeRangeAsync(fromElement, fromInclusive, toElement, toInclusive));
}
@Override
public int removeRangeHead(String toElement, boolean toInclusive) {
return get(removeRangeHeadAsync(toElement, toInclusive));
}
@Override
public RFuture removeRangeHeadAsync(String toElement, boolean toInclusive) {
String toValue = value(toElement, toInclusive);
return commandExecutor.writeAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREMRANGEBYLEX, getRawName(), "-", toValue);
}
@Override
public int removeRangeTail(String fromElement, boolean fromInclusive) {
return get(removeRangeTailAsync(fromElement, fromInclusive));
}
@Override
public RFuture removeRangeTailAsync(String fromElement, boolean fromInclusive) {
String fromValue = value(fromElement, fromInclusive);
return commandExecutor.writeAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREMRANGEBYLEX, getRawName(), fromValue, "+");
}
@Override
public RFuture removeRangeAsync(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive) {
String fromValue = value(fromElement, fromInclusive);
String toValue = value(toElement, toInclusive);
return commandExecutor.writeAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREMRANGEBYLEX, getRawName(), fromValue, toValue);
}
@Override
public Collection range(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive) {
return get(rangeAsync(fromElement, fromInclusive, toElement, toInclusive));
}
@Override
public Collection rangeHead(String toElement, boolean toInclusive) {
return get(rangeHeadAsync(toElement, toInclusive));
}
@Override
public RFuture> rangeHeadAsync(String toElement, boolean toInclusive) {
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZRANGEBYLEX, getRawName(), "-", toValue);
}
@Override
public Collection rangeTail(String fromElement, boolean fromInclusive) {
return get(rangeTailAsync(fromElement, fromInclusive));
}
@Override
public RFuture> rangeTailAsync(String fromElement, boolean fromInclusive) {
String fromValue = value(fromElement, fromInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZRANGEBYLEX, getRawName(), fromValue, "+");
}
@Override
public RFuture> rangeAsync(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive) {
String fromValue = value(fromElement, fromInclusive);
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZRANGEBYLEX, getRawName(), fromValue, toValue);
}
@Override
public Collection range(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive,
int offset, int count) {
return get(rangeAsync(fromElement, fromInclusive, toElement, toInclusive, offset, count));
}
@Override
public Collection rangeHead(String toElement, boolean toInclusive, int offset, int count) {
return get(rangeHeadAsync(toElement, toInclusive, offset, count));
}
@Override
public RFuture> rangeHeadAsync(String toElement, boolean toInclusive, int offset, int count) {
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZRANGEBYLEX, getRawName(), "-", toValue, "LIMIT", offset, count);
}
@Override
public Collection rangeTail(String fromElement, boolean fromInclusive, int offset, int count) {
return get(rangeTailAsync(fromElement, fromInclusive, offset, count));
}
@Override
public RFuture> rangeTailAsync(String fromElement, boolean fromInclusive, int offset, int count) {
String fromValue = value(fromElement, fromInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZRANGEBYLEX, getRawName(), fromValue, "+", "LIMIT", offset, count);
}
@Override
public RFuture> rangeAsync(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive, int offset, int count) {
String fromValue = value(fromElement, fromInclusive);
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZRANGEBYLEX, getRawName(), fromValue, toValue, "LIMIT", offset, count);
}
@Override
public Collection rangeTailReversed(String fromElement, boolean fromInclusive) {
return get(rangeTailReversedAsync(fromElement, fromInclusive));
}
@Override
public Collection rangeHeadReversed(String toElement, boolean toInclusive) {
return get(rangeHeadReversedAsync(toElement, toInclusive));
}
@Override
public Collection rangeReversed(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive) {
return get(rangeReversedAsync(fromElement, fromInclusive, toElement, toInclusive));
}
@Override
public Collection rangeTailReversed(String fromElement, boolean fromInclusive, int offset, int count) {
return get(rangeTailReversedAsync(fromElement, fromInclusive, offset, count));
}
@Override
public Collection rangeHeadReversed(String toElement, boolean toInclusive, int offset, int count) {
return get(rangeHeadReversedAsync(toElement, toInclusive, offset, count));
}
@Override
public Collection rangeReversed(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive, int offset, int count) {
return get(rangeReversedAsync(fromElement, fromInclusive, toElement, toInclusive, offset, count));
}
@Override
public RFuture> rangeTailReversedAsync(String fromElement, boolean fromInclusive) {
String fromValue = value(fromElement, fromInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREVRANGEBYLEX, getRawName(), "+", fromValue);
}
@Override
public RFuture> rangeHeadReversedAsync(String toElement, boolean toInclusive) {
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREVRANGEBYLEX, getRawName(), toValue, "-");
}
@Override
public RFuture> rangeReversedAsync(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive) {
String fromValue = value(fromElement, fromInclusive);
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREVRANGEBYLEX, getRawName(), toValue, fromValue);
}
@Override
public RFuture> rangeTailReversedAsync(String fromElement, boolean fromInclusive, int offset,
int count) {
String fromValue = value(fromElement, fromInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREVRANGEBYLEX, getRawName(), "+", fromValue, "LIMIT", offset, count);
}
@Override
public RFuture> rangeHeadReversedAsync(String toElement, boolean toInclusive, int offset,
int count) {
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREVRANGEBYLEX, getRawName(), toValue, "-", "LIMIT", offset, count);
}
@Override
public RFuture> rangeReversedAsync(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive, int offset, int count) {
String fromValue = value(fromElement, fromInclusive);
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZREVRANGEBYLEX, getRawName(), toValue, fromValue, "LIMIT", offset, count);
}
@Override
public int countTail(String fromElement, boolean fromInclusive) {
return get(countTailAsync(fromElement, fromInclusive));
}
@Override
public RFuture countTailAsync(String fromElement, boolean fromInclusive) {
String fromValue = value(fromElement, fromInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZLEXCOUNT, getRawName(), fromValue, "+");
}
@Override
public int countHead(String toElement, boolean toInclusive) {
return get(countHeadAsync(toElement, toInclusive));
}
@Override
public RFuture countHeadAsync(String toElement, boolean toInclusive) {
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZLEXCOUNT, getRawName(), "-", toValue);
}
@Override
public int count(String fromElement, boolean fromInclusive, String toElement, boolean toInclusive) {
return get(countAsync(fromElement, fromInclusive, toElement, toInclusive));
}
@Override
public RFuture countAsync(String fromElement, boolean fromInclusive, String toElement,
boolean toInclusive) {
String fromValue = value(fromElement, fromInclusive);
String toValue = value(toElement, toInclusive);
return commandExecutor.readAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZLEXCOUNT, getRawName(), fromValue, toValue);
}
private String value(String fromElement, boolean fromInclusive) {
String fromValue = fromElement.toString();
if (fromInclusive) {
fromValue = "[" + fromValue;
} else {
fromValue = "(" + fromValue;
}
return fromValue;
}
@Override
public RFuture addAsync(String e) {
return commandExecutor.writeAsync(getRawName(), StringCodec.INSTANCE, RedisCommands.ZADD_BOOL_RAW, getRawName(), 0, e);
}
@Override
public RFuture addAllAsync(Collection extends String> c) {
if (c.isEmpty()) {
return new CompletableFutureWrapper<>(false);
}
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy