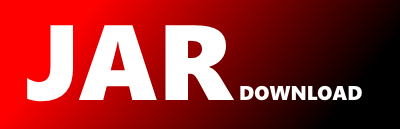
org.redisson.RedissonListMultimapCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson-all Show documentation
Show all versions of redisson-all Show documentation
Easy Redis Java client and Real-Time Data Platform. Valkey compatible. Sync/Async/RxJava3/Reactive API. Client side caching. Over 50 Redis based Java objects and services: JCache API, Apache Tomcat, Hibernate, Spring, Set, Multimap, SortedSet, Map, List, Queue, Deque, Semaphore, Lock, AtomicLong, Map Reduce, Bloom filter, Scheduler, RPC
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson;
import io.netty.buffer.ByteBuf;
import org.redisson.api.RFuture;
import org.redisson.api.RList;
import org.redisson.api.RListMultimapCache;
import org.redisson.client.codec.Codec;
import org.redisson.client.protocol.RedisCommands;
import org.redisson.command.CommandAsyncExecutor;
import org.redisson.eviction.EvictionScheduler;
import java.util.Arrays;
import java.util.Collection;
import java.util.concurrent.TimeUnit;
/**
* @author Nikita Koksharov
*
* @param key
* @param value
*/
public class RedissonListMultimapCache extends RedissonListMultimap implements RListMultimapCache {
private final RedissonMultimapCache baseCache;
public RedissonListMultimapCache(EvictionScheduler evictionScheduler, CommandAsyncExecutor connectionManager, String name) {
super(connectionManager, name);
baseCache = new RedissonMultimapCache<>(connectionManager, evictionScheduler, this, getTimeoutSetName(), prefix);
}
public RedissonListMultimapCache(EvictionScheduler evictionScheduler, Codec codec, CommandAsyncExecutor connectionManager, String name) {
super(codec, connectionManager, name);
baseCache = new RedissonMultimapCache<>(connectionManager, evictionScheduler, this, getTimeoutSetName(), prefix);
}
@Override
public RFuture containsKeyAsync(Object key) {
ByteBuf keyState = encodeMapKey(key);
String keyHash = hash(keyState);
String valuesName = getValuesName(keyHash);
return commandExecutor.evalReadAsync(getRawName(), codec, RedisCommands.EVAL_BOOLEAN,
"local value = redis.call('hget', KEYS[1], ARGV[2]); " +
"if value ~= false then " +
"local expireDate = 92233720368547758; " +
"local expireDateScore = redis.call('zscore', KEYS[2], ARGV[2]); "
+ "if expireDateScore ~= false then "
+ "expireDate = tonumber(expireDateScore) "
+ "end; "
+ "if expireDate <= tonumber(ARGV[1]) then "
+ "return 0;"
+ "end; "
+ "return redis.call('llen', ARGV[3]) > 0 and 1 or 0;" +
"end;" +
"return 0; ",
Arrays.asList(getRawName(), getTimeoutSetName()), System.currentTimeMillis(), keyState, valuesName);
}
String getTimeoutSetName() {
return suffixName(getRawName(), "redisson_list_multimap_ttl");
}
@Override
public RFuture containsValueAsync(Object value) {
ByteBuf valueState = encodeMapValue(value);
return commandExecutor.evalReadAsync(getRawName(), codec, RedisCommands.EVAL_BOOLEAN,
"local keys = redis.call('hgetall', KEYS[1]); " +
"for i, v in ipairs(keys) do " +
"if i % 2 == 0 then " +
"local expireDate = 92233720368547758; " +
"local expireDateScore = redis.call('zscore', KEYS[2], keys[i-1]); "
+ "if expireDateScore ~= false then "
+ "expireDate = tonumber(expireDateScore) "
+ "end; "
+ "if expireDate > tonumber(ARGV[2]) then " +
"local name = ARGV[3] .. v; " +
"local items = redis.call('lrange', name, 0, -1) " +
"for i=1,#items do " +
"if items[i] == ARGV[1] then " +
"return 1; " +
"end; " +
"end; " +
"end; " +
"end;" +
"end; " +
"return 0; ",
Arrays.asList(getRawName(), getTimeoutSetName()),
valueState, System.currentTimeMillis(), prefix);
}
@Override
public RFuture containsEntryAsync(Object key, Object value) {
ByteBuf keyState = encodeMapKey(key);
String keyHash = hash(keyState);
ByteBuf valueState = encodeMapValue(value);
String valuesName = getValuesName(keyHash);
return commandExecutor.evalReadAsync(getRawName(), codec, RedisCommands.EVAL_BOOLEAN,
"local expireDate = 92233720368547758; " +
"local expireDateScore = redis.call('zscore', KEYS[2], ARGV[2]); "
+ "if expireDateScore ~= false then "
+ "expireDate = tonumber(expireDateScore) "
+ "end; "
+ "if expireDate > tonumber(ARGV[1]) then " +
"local items = redis.call('lrange', KEYS[1], 0, -1); " +
"for i = 1, #items do " +
"if items[i] == ARGV[3] then " +
"return 1; " +
"end; " +
"end; " +
"end; " +
"return 0; ",
Arrays.asList(valuesName, getTimeoutSetName()),
System.currentTimeMillis(), keyState, valueState);
}
@Override
public RList get(K key) {
String keyHash = keyHash(key);
String valuesName = getValuesName(keyHash);
return new RedissonListMultimapValues<>(codec, commandExecutor, valuesName, getTimeoutSetName(), key);
}
@Override
public RFuture> getAllAsync(K key) {
ByteBuf keyState = encodeMapKey(key);
String keyHash = hash(keyState);
String valuesName = getValuesName(keyHash);
return commandExecutor.evalReadAsync(getRawName(), codec, RedisCommands.EVAL_LIST,
"local expireDate = 92233720368547758; " +
"local expireDateScore = redis.call('zscore', KEYS[2], ARGV[2]); "
+ "if expireDateScore ~= false then "
+ "expireDate = tonumber(expireDateScore) "
+ "end; "
+ "if expireDate > tonumber(ARGV[1]) then " +
"return redis.call('lrange', KEYS[1], 0, -1); " +
"end; " +
"return {}; ",
Arrays.asList(valuesName, getTimeoutSetName()), System.currentTimeMillis(), keyState);
}
@Override
public RFuture> removeAllAsync(Object key) {
ByteBuf keyState = encodeMapKey(key);
String keyHash = hash(keyState);
String valuesName = getValuesName(keyHash);
return commandExecutor.evalWriteAsync(getRawName(), codec, RedisCommands.EVAL_LIST,
"redis.call('hdel', KEYS[1], ARGV[1]); " +
"local members = redis.call('lrange', KEYS[2], 0, -1); " +
"redis.call('del', KEYS[2]); " +
"redis.call('zrem', KEYS[3], ARGV[1]); " +
"return members; ",
Arrays.asList(getRawName(), valuesName, getTimeoutSetName()), keyState);
}
@Override
public boolean expireKey(K key, long timeToLive, TimeUnit timeUnit) {
return get(expireKeyAsync(key, timeToLive, timeUnit));
}
@Override
public RFuture expireKeyAsync(K key, long timeToLive, TimeUnit timeUnit) {
return baseCache.expireKeyAsync(key, timeToLive, timeUnit);
}
@Override
public RFuture sizeInMemoryAsync() {
return baseCache.sizeInMemoryAsync();
}
@Override
public RFuture deleteAsync() {
return baseCache.deleteAsync();
}
@Override
public RFuture expireAsync(long timeToLive, TimeUnit timeUnit, String param, String... keys) {
return baseCache.expireAsync(timeToLive, timeUnit, param);
}
@Override
protected RFuture expireAtAsync(long timestamp, String param, String... keys) {
return baseCache.expireAtAsync(timestamp, param);
}
@Override
public RFuture clearExpireAsync() {
return baseCache.clearExpireAsync();
}
@Override
public void destroy() {
baseCache.destroy();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy