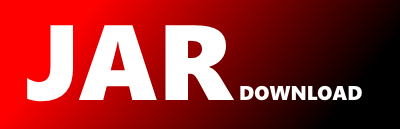
org.redisson.api.RJsonStoreRx Maven / Gradle / Ivy
Show all versions of redisson-all Show documentation
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Maybe;
import io.reactivex.rxjava3.core.Single;
import org.redisson.codec.JsonCodec;
import java.time.Duration;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* JSON Store which stores each entry as key and value. Both are POJO objects.
* Value is stored as JSON datatype in Redis.
*
* The implementation is available in Redisson PRO only.
*
* @author Nikita Koksharov
*
* @param the type of key
* @param the type of value
*
*/
public interface RJsonStoreRx extends RExpirableRx {
/**
* Gets value by specified key and JSONPath
*
* @param key entry key
* @param codec entry value codec
* @param paths JSON paths
* @return entry value
*
* @param the type of object
*/
Maybe get(K key, JsonCodec codec, String... paths);
/**
* Sets value by specified key and JSONPath only if previous value is empty.
*
* @param key entry key
* @param path JSON path
* @param value entry value
* @return {@code true} if successful, or {@code false} if
* value was already set
*/
Single setIfAbsent(K key, String path, Object value);
/**
* Sets value by specified key and JSONPath only if previous value is non-empty.
*
* @param key entry key
* @param path JSON path
* @param value object
* @return {@code true} if successful, or {@code false} if
* element wasn't set
*/
Single setIfExists(K key, String path, Object value);
/**
* Atomically sets the value to the given updated value
* by specified key and JSONPath, only if serialized state of
* the current value equals to serialized state of the expected value.
*
* @param key entry key
* @param path JSON path
* @param expect the expected value
* @param update the new value
* @return {@code true} if successful; or {@code false} if the actual value
* was not equal to the expected value.
*/
Single compareAndSet(K key, String path, Object expect, Object update);
/**
* Retrieves current value stored by specified key and JSONPath then
* replaces it with new value.
*
* @param key entry key
* @param codec entry value codec
* @param path JSON path
* @param newValue value to set
* @return previous value
*/
Maybe getAndSet(K key, JsonCodec codec, String path, Object newValue);
/**
* Stores value by specified key and JSONPath.
*
* @param key entry key
* @param path JSON path
* @param value value to set
*/
Completable set(K key, String path, Object value);
/**
* Returns size of string data by specified key and JSONPath
*
* @param key entry key
* @param path JSON path
* @return size of string
*/
Maybe stringSize(K key, String path);
/**
* Returns list of string data size by specified key and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @return list of string data sizes
*/
Single> stringSizeMulti(K key, String path);
/**
* Appends string data to element specified by specified key and JSONPath.
* Returns new size of string data.
*
* @param key entry key
* @param path JSON path
* @param value data
* @return size of string data
*/
Single stringAppend(K key, String path, Object value);
/**
* Appends string data to elements specified by specified key and JSONPath.
* Returns new size of string data.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param value data
* @return list of string data sizes
*/
Single> stringAppendMulti(K key, String path, Object value);
/**
* Appends values to array by specified key and JSONPath.
* Returns new size of array.
*
* @param key entry key
* @param path JSON path
* @param values values to append
* @return size of array
*/
Single arrayAppend(K key, String path, Object... values);
/**
* Appends values to arrays by specified key and JSONPath.
* Returns new size of arrays.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param values values to append
* @return list of arrays size
*/
Single> arrayAppendMulti(K key, String path, Object... values);
/**
* Returns index of object in array by specified key and JSONPath.
* -1 means object not found.
*
* @param key entry key
* @param path JSON path
* @param value value to search
* @return index in array
*/
Single arrayIndex(K key, String path, Object value);
/**
* Returns index of object in arrays by specified key and JSONPath.
* -1 means object not found.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param value value to search
* @return list of index in arrays
*/
Single> arrayIndexMulti(K key, String path, Object value);
/**
* Returns index of object in array by specified key and JSONPath
* in range between start
(inclusive) and end
(exclusive) indexes.
* -1 means object not found.
*
* @param key entry key
* @param path JSON path
* @param value value to search
* @param start start index, inclusive
* @param end end index, exclusive
* @return index in array
*/
Single arrayIndex(K key, String path, Object value, Long start, Long end);
/**
* Returns index of object in arrays by specified key and JSONPath
* in range between start
(inclusive) and end
(exclusive) indexes.
* -1 means object not found.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param value value to search
* @param start start index, inclusive
* @param end end index, exclusive
* @return list of index in arrays
*/
Single> arrayIndexMulti(K key, String path, Object value, Long start, Long end);
/**
* Inserts values into array by specified key and JSONPath.
* Values are inserted at defined index
.
*
* @param key entry key
* @param path JSON path
* @param index array index at which values are inserted
* @param values values to insert
* @return size of array
*/
Single arrayInsert(K key, String path, Long index, Object... values);
/**
* Inserts values into arrays by specified key and JSONPath.
* Values are inserted at defined index
.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param index array index at which values are inserted
* @param values values to insert
* @return list of arrays size
*/
Single> arrayInsertMulti(K key, String path, Long index, Object... values);
/**
* Returns size of array by specified key and JSONPath.
*
* @param key entry key
* @param path JSON path
* @return size of array
*/
Single arraySize(K key, String path);
/**
* Returns size of arrays by specified key and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @return list of arrays size
*/
Single> arraySizeMulti(K key, String path);
/**
* Polls last element of array by specified key and JSONPath.
*
* @param key entry key
* @param codec object codec
* @param path JSON path
* @return last element
*
* @param the type of object
*/
Maybe arrayPollLast(K key, JsonCodec codec, String path);
/**
* Polls last element of arrays by specified key and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param codec object codec
* @param path JSON path
* @return list of last elements
*
* @param the type of object
*/
Single> arrayPollLastMulti(K key, JsonCodec codec, String path);
/**
* Polls first element of array by specified key and JSONPath.
*
* @param key entry key
* @param codec object codec
* @param path JSON path
* @return first element
*
* @param the type of object
*/
Maybe arrayPollFirst(K key, JsonCodec codec, String path);
/**
* Polls first element of arrays by specified key and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param codec object codec
* @param path JSON path
* @return list of first elements
*
* @param the type of object
*/
Single> arrayPollFirstMulti(K key, JsonCodec codec, String path);
/**
* Pops element located at index of array by specified key and JSONPath.
*
* @param key entry key
* @param codec object codec
* @param path JSON path
* @param index array index
* @return element
*
* @param the type of object
*/
Maybe arrayPop(K key, JsonCodec codec, String path, Long index);
/**
* Pops elements located at index of arrays by specified key and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param codec object codec
* @param path JSON path
* @param index array index
* @return list of elements
*
* @param the type of object
*/
Single> arrayPopMulti(K key, JsonCodec codec, String path, Long index);
/**
* Trims array by specified key and JSONPath in range
* between start
(inclusive) and end
(inclusive) indexes.
*
* @param key entry key
* @param path JSON path
* @param start start index, inclusive
* @param end end index, inclusive
* @return length of array
*/
Single arrayTrim(K key, String path, Long start, Long end);
/**
* Trims arrays by specified key and JSONPath in range
* between start
(inclusive) and end
(inclusive) indexes.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param start start index, inclusive
* @param end end index, inclusive
* @return length of array
*/
Single> arrayTrimMulti(K key, String path, Long start, Long end);
/**
* Clears value by specified key
*
* @param key entry key
* @return {@code true} if successful, or {@code false} if
* entry doesn't exist
*/
Single clear(K key);
/**
* Clears json containers by specified keys.
*
* @param keys entry keys
* @return number of cleared containers
*/
Single clear(Set keys);
/**
* Clears json container by specified keys and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param path JSON path
* @param keys entry keys
* @return number of cleared containers
*/
Single clear(String path, Set keys);
/**
* Increments the current value specified by key and JSONPath.
*
* @param key entry key
* @param path JSON path
* @param delta increment value
* @return the updated value
*/
Maybe incrementAndGet(K key, String path, T delta);
/**
* Increments the current values specified by key and JSONPath.
* Compatible only with enhanced syntax starting with '$' character.
*
* @param key entry key
* @param path JSON path
* @param delta increment value
* @return list of updated value
*/
List incrementAndGetMulti(K key, String path, T delta);
/**
* Merges value into element by the specified key and JSONPath.
*
* @param key entry key
* @param path JSON path
* @param value value to merge
*/
Completable merge(K key, String path, Object value);
/**
* Returns keys amount in JSON container by specified key
*
* @param key entry key
* @return keys amount
*/
Maybe countKeys(K key);
/**
* Returns keys amount in JSON container specified by key and JSONPath
*
* @param key entry key
* @param path JSON path
* @return keys amount
*/
Maybe countKeys(K key, String path);
/**
* Returns list of keys amount in JSON containers specified by key and JSONPath
*
* @param key entry key
* @param path JSON path
* @return list of keys amount
*/
Single> countKeysMulti(K key, String path);
/**
* Returns list of keys in JSON container by specified key
*
* @return list of keys
*/
Single> getKeys(K key);
/**
* Returns list of keys in JSON container by specified key and JSONPath
*
* @param path JSON path
* @return list of keys
*/
Single> getKeys(K key, String path);
/**
* Returns list of keys in JSON containers by specified key and JSONPath
*
* @param path JSON path
* @return list of keys
*/
Single>> getKeysMulti(K key, String path);
/**
* Toggle Single value by specified key and JSONPath
*
* @param path JSON path
* @return new Single value
*/
Single toggle(K key, String path);
/**
* Toggle Single values by specified key and JSONPath
*
* @param path JSON path
* @return list of Single values
*/
Single> toggleMulti(K key, String path);
/**
* Returns type of value
*
* @return type of element
*/
Single getType(K key);
/**
* Returns type of element specified by key and JSONPath
*
* @param path JSON path
* @return type of element
*/
Single getType(K key, String path);
/**
* Deletes entry by specified key
*
* @param key entry key
* @return {@code true} if successful, or {@code false} if
* entry doesn't exist
*/
Single delete(K key);
/**
* Deletes entries by specified keys
*
* @param keys entry keys
* @return number of deleted elements
*/
Single delete(Set keys);
/**
* Deletes JSON elements specified by keys and JSONPath
*
* @param path JSON path
* @param keys entry keys
* @return number of deleted elements
*/
Single delete(String path, Set keys);
/**
* Returns size of entry in bytes specified by key.
*
* @param key entry key
* @return entry size
*/
Single sizeInMemory(K key);
/**
* Retrieves value by specified key.
*
* @param key entry key
* @return element
*/
Maybe get(K key);
/**
* Retrieves values by specified keys.
*
* @param keys entry keys
* @return map with entries where value mapped by key
*/
Single