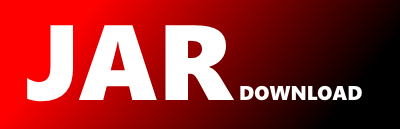
org.redisson.api.RedissonClient Maven / Gradle / Ivy
Show all versions of redisson-all Show documentation
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import org.redisson.api.options.*;
import org.redisson.api.redisnode.BaseRedisNodes;
import org.redisson.api.redisnode.RedisNodes;
import org.redisson.client.codec.Codec;
import org.redisson.codec.JsonCodec;
import org.redisson.config.Config;
import java.util.Collection;
import java.util.concurrent.TimeUnit;
/**
* Main Redisson interface for access
* to all redisson objects with sync/async interface.
*
* @see RedissonReactiveClient
* @see RedissonRxClient
*
* @author Nikita Koksharov
*
*/
public interface RedissonClient {
/**
* Returns time-series instance by name
*
* @param value type
* @param label type
* @param name name of instance
* @return RTimeSeries object
*/
RTimeSeries getTimeSeries(String name);
/**
* Returns time-series instance by name
* using provided codec
for values.
*
* @param value type
* @param label type
* @param name name of instance
* @param codec codec for values
* @return RTimeSeries object
*/
RTimeSeries getTimeSeries(String name, Codec codec);
/**
* Returns time-series instance with specified options
.
*
* @param value type
* @param label type
* @param options instance options
* @return RTimeSeries object
*/
RTimeSeries getTimeSeries(PlainOptions options);
/**
* Returns stream instance by name
*
* Requires Redis 5.0.0 and higher.
*
* @param type of key
* @param type of value
* @param name of stream
* @return RStream object
*/
RStream getStream(String name);
/**
* Returns stream instance by name
* using provided codec
for entries.
*
* Requires Redis 5.0.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of stream
* @param codec codec for entry
* @return RStream object
*/
RStream getStream(String name, Codec codec);
/**
* Returns time-series instance with specified options
.
*
* Requires Redis 5.0.0 and higher.
*
* @param type of key
* @param type of value
* @param options instance options
* @return RStream object
*/
RStream getStream(PlainOptions options);
/**
* Returns API for RediSearch module
*
* @return RSearch object
*/
RSearch getSearch();
/**
* Returns API for RediSearch module using defined codec for attribute values.
*
* @param codec codec for entry
* @return RSearch object
*/
RSearch getSearch(Codec codec);
/**
* Returns API for RediSearch module with specified options
.
*
* @param options instance options
* @return RSearch object
*/
RSearch getSearch(OptionalOptions options);
/**
* Returns rate limiter instance by name
*
* @param name of rate limiter
* @return RateLimiter object
*/
RRateLimiter getRateLimiter(String name);
/**
* Returns rate limiter instance with specified options
.
*
* @param options instance options
* @return RateLimiter object
*/
RRateLimiter getRateLimiter(CommonOptions options);
/**
* Returns binary stream holder instance by name
*
* @param name of binary stream
* @return BinaryStream object
*/
RBinaryStream getBinaryStream(String name);
/**
* Returns binary stream holder instance with specified options
.
*
* @param options instance options
* @return BinaryStream object
*/
RBinaryStream getBinaryStream(CommonOptions options);
/**
* Returns geospatial items holder instance by name
.
*
* @param type of value
* @param name name of object
* @return Geo object
*/
RGeo getGeo(String name);
/**
* Returns geospatial items holder instance by name
* using provided codec for geospatial members.
*
* @param type of value
* @param name name of object
* @param codec codec for value
* @return Geo object
*/
RGeo getGeo(String name, Codec codec);
/**
* Returns geospatial items holder instance with specified options
.
*
* @param type of value
* @param options instance options
* @return Geo object
*/
RGeo getGeo(PlainOptions options);
/**
* Returns set-based cache instance by name
.
* Supports value eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSet(String, Codec)}.
*
* @param type of value
* @param name name of object
* @return SetCache object
*/
RSetCache getSetCache(String name);
/**
* Returns set-based cache instance by name
.
* Supports value eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSet(String, Codec)}.
*
* @param type of value
* @param name name of object
* @param codec codec for values
* @return SetCache object
*/
RSetCache getSetCache(String name, Codec codec);
/**
* Returns set-based cache instance with specified options
.
* Supports value eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSet(PlainOptions)}.
*
* @param type of value
* @param options instance options
* @return SetCache object
*/
RSetCache getSetCache(PlainOptions options);
/**
* Returns map-based cache instance by name
* using provided codec
for both cache keys and values.
* Supports entry eviction with a given MaxIdleTime and TTL settings.
*
* If eviction is not required then it's better to use regular map {@link #getMap(String, Codec)}.
*
* @param type of key
* @param type of value
* @param name object name
* @param codec codec for keys and values
* @return MapCache object
*/
RMapCache getMapCache(String name, Codec codec);
/**
* Returns map-based cache instance by name
* using provided codec
for both cache keys and values.
* Supports entry eviction with a given MaxIdleTime and TTL settings.
*
* If eviction is not required then it's better to use regular map {@link #getMap(String, Codec)}.
*
* @param type of key
* @param type of value
* @param name object name
* @param codec codec for keys and values
* @param options map options
* @return MapCache object
*/
@Deprecated
RMapCache getMapCache(String name, Codec codec, MapCacheOptions options);
/**
* Returns map-based cache instance with specified options
.
* Supports entry eviction with a given MaxIdleTime and TTL settings.
*
* If eviction is not required then it's better to use regular map {@link #getMap(org.redisson.api.options.MapOptions)}.
*
* @param type of key
* @param type of value
* @param options instance options
* @return MapCache object
*/
RMapCache getMapCache(org.redisson.api.options.MapCacheOptions options);
/**
* Returns map-based cache instance by name.
* Supports entry eviction with a given MaxIdleTime and TTL settings.
*
* If eviction is not required then it's better to use regular map {@link #getMap(String)}.
*
* @param type of key
* @param type of value
* @param name name of object
* @return MapCache object
*/
RMapCache getMapCache(String name);
/**
* Returns map-based cache instance by name.
* Supports entry eviction with a given MaxIdleTime and TTL settings.
*
* If eviction is not required then it's better to use regular map {@link #getMap(String)}.
*
* @param type of key
* @param type of value
* @param name name of object
* @param options map options
* @return MapCache object
*/
@Deprecated
RMapCache getMapCache(String name, MapCacheOptions options);
/**
* Returns object holder instance by name.
*
* @param type of value
* @param name name of object
* @return Bucket object
*/
RBucket getBucket(String name);
/**
* Returns object holder instance by name
* using provided codec for object.
*
* @param type of value
* @param name name of object
* @param codec codec for values
* @return Bucket object
*/
RBucket getBucket(String name, Codec codec);
/**
* Returns object holder instance with specified options
.
*
* @param type of value
* @param options instance options
* @return Bucket object
*/
RBucket getBucket(PlainOptions options);
/**
* Returns interface for mass operations with Bucket objects.
*
* @return Buckets object
*/
RBuckets getBuckets();
/**
* Returns interface for mass operations with Bucket objects
* using provided codec for object.
*
* @param codec codec for bucket objects
* @return Buckets object
*/
RBuckets getBuckets(Codec codec);
/**
* Returns API for mass operations over Bucket objects with specified options
.
*
* @param options instance options
* @return Buckets object
*/
RBuckets getBuckets(OptionalOptions options);
/**
* Returns JSON data holder instance by name using provided codec.
*
* @param type of value
* @param name name of object
* @param codec codec for values
* @return JsonBucket object
*/
RJsonBucket getJsonBucket(String name, JsonCodec codec);
/**
* Returns JSON data holder instance with specified options
.
*
* @param type of value
* @param options instance options
* @return JsonBucket object
*/
RJsonBucket getJsonBucket(JsonBucketOptions options);
/**
* Returns API for mass operations over JsonBucket objects
* using provided codec for JSON object with default path.
*
* @param codec using provided codec for JSON object with default path.
* @return JsonBuckets
*/
RJsonBuckets getJsonBuckets(JsonCodec codec);
/**
* Returns HyperLogLog instance by name.
*
* @param type of value
* @param name name of object
* @return HyperLogLog object
*/
RHyperLogLog getHyperLogLog(String name);
/**
* Returns HyperLogLog instance by name
* using provided codec for hll objects.
*
* @param type of value
* @param name name of object
* @param codec codec for values
* @return HyperLogLog object
*/
RHyperLogLog getHyperLogLog(String name, Codec codec);
/**
* Returns HyperLogLog instance with specified options
.
*
* @param type of value
* @param options instance options
* @return HyperLogLog object
*/
RHyperLogLog getHyperLogLog(PlainOptions options);
/**
* Returns list instance by name.
*
* @param type of value
* @param name name of object
* @return List object
*/
RList getList(String name);
/**
* Returns list instance by name
* using provided codec for list objects.
*
* @param type of value
* @param name name of object
* @param codec codec for values
* @return List object
*/
RList getList(String name, Codec codec);
/**
* Returns list instance with specified options
.
*
* @param type of value
* @param options instance options
* @return List object
*/
RList getList(PlainOptions options);
/**
* Returns List based Multimap instance by name.
*
* @param type of key
* @param type of value
* @param name name of object
* @return ListMultimap object
*/
RListMultimap getListMultimap(String name);
/**
* Returns List based Multimap instance by name
* using provided codec for both map keys and values.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return ListMultimap object
*/
RListMultimap getListMultimap(String name, Codec codec);
/**
* Returns List based Multimap instance with specified options
.
*
* @param type of key
* @param type of value
* @param options instance options
* @return ListMultimap object
*/
RListMultimap getListMultimap(PlainOptions options);
/**
* Returns List based Multimap instance by name.
* Supports key-entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSetMultimap(String)}.
*
* @param type of key
* @param type of value
* @param name name of object
* @return ListMultimapCache object
*/
RListMultimapCache getListMultimapCache(String name);
/**
* Returns List based Multimap instance by name
* using provided codec for both map keys and values.
* Supports key-entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSetMultimap(String, Codec)}.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return ListMultimapCache object
*/
RListMultimapCache getListMultimapCache(String name, Codec codec);
/**
* Returns List based Multimap instance by name.
* Supports key-entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSetMultimap(String)}.
*
* @param type of key
* @param type of value
* @param options instance options
* @return ListMultimapCache object
*/
RListMultimapCache getListMultimapCache(PlainOptions options);
/**
* Returns List based Multimap instance by name.
* Supports key-entry eviction with a given TTL value.
* Stores insertion order and allows duplicates for values mapped to key.
*
* Uses Redis native commands for entry expiration and not a scheduled eviction task.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of object
* @return ListMultimapCache object
*/
RListMultimapCacheNative getListMultimapCacheNative(String name);
/**
* Returns List based Multimap instance by name
* using provided codec for both map keys and values.
* Supports key-entry eviction with a given TTL value.
* Stores insertion order and allows duplicates for values mapped to key.
*
* Uses Redis native commands for entry expiration and not a scheduled eviction task.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return ListMultimapCache object
*/
RListMultimapCacheNative getListMultimapCacheNative(String name, Codec codec);
/**
* Returns List based Multimap instance by name.
* Supports key-entry eviction with a given TTL value.
* Stores insertion order and allows duplicates for values mapped to key.
*
* Uses Redis native commands for entry expiration and not a scheduled eviction task.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param options instance options
* @return ListMultimapCache object
*/
RListMultimapCacheNative getListMultimapCacheNative(PlainOptions options);
/**
* Returns local cached map instance by name.
* Configured by parameters of options-object.
*
* @param type of key
* @param type of value
* @param name name of object
* @param options local map options
* @return LocalCachedMap object
*/
@Deprecated
RLocalCachedMap getLocalCachedMap(String name, LocalCachedMapOptions options);
/**
* Returns local cached map instance by name
* using provided codec. Configured by parameters of options-object.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @param options local map options
* @return LocalCachedMap object
*/
@Deprecated
RLocalCachedMap getLocalCachedMap(String name, Codec codec, LocalCachedMapOptions options);
/**
* Returns local cached map instance with specified options
.
*
* @param type of key
* @param type of value
* @param options instance options
* @return LocalCachedMap object
*/
RLocalCachedMap getLocalCachedMap(org.redisson.api.options.LocalCachedMapOptions options);
/**
* Returns map instance by name.
*
* @param type of key
* @param type of value
* @param name name of object
* @return Map object
*/
RMap getMap(String name);
/**
* Returns map instance by name.
*
* @param type of key
* @param type of value
* @param name name of object
* @param options map options
* @return Map object
*/
@Deprecated
RMap getMap(String name, MapOptions options);
/**
* Returns map instance by name
* using provided codec for both map keys and values.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return Map object
*/
RMap getMap(String name, Codec codec);
/**
* Returns map instance by name
* using provided codec for both map keys and values.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @param options map options
* @return Map object
*/
@Deprecated
RMap getMap(String name, Codec codec, MapOptions options);
/**
* Returns map instance by name.
*
* @param type of key
* @param type of value
* @param options instance options
* @return Map object
*/
RMap getMap(org.redisson.api.options.MapOptions options);
/**
* Returns map instance by name.
* Supports entry eviction with a given TTL.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of object
* @return Map object
*/
RMapCacheNative getMapCacheNative(String name);
/**
* Returns map instance by name
* using provided codec for both map keys and values.
* Supports entry eviction with a given TTL.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return Map object
*/
RMapCacheNative getMapCacheNative(String name, Codec codec);
/**
* Returns map instance.
* Supports entry eviction with a given TTL.
* Configured by the parameters of the options-object.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param options instance options
* @return Map object
*/
RMapCacheNative getMapCacheNative(org.redisson.api.options.MapOptions options);
/**
* Returns Set based Multimap instance by name.
*
* @param type of key
* @param type of value
* @param name name of object
* @return SetMultimap object
*/
RSetMultimap getSetMultimap(String name);
/**
* Returns Set based Multimap instance by name
* using provided codec for both map keys and values.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return SetMultimap object
*/
RSetMultimap getSetMultimap(String name, Codec codec);
/**
* Returns Set based Multimap instance with specified options
.
*
* @param type of key
* @param type of value
* @param options instance options
* @return SetMultimap object
*/
RSetMultimap getSetMultimap(PlainOptions options);
/**
* Returns Set based Multimap instance by name.
* Supports key-entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSetMultimap(String)}.
*
* @param type of key
* @param type of value
* @param name name of object
* @return SetMultimapCache object
*/
RSetMultimapCache getSetMultimapCache(String name);
/**
* Returns Set based Multimap instance by name
* using provided codec for both map keys and values.
* Supports key-entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSetMultimap(String, Codec)}.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return SetMultimapCache object
*/
RSetMultimapCache getSetMultimapCache(String name, Codec codec);
/**
* Returns Set based Multimap instance with specified options
.
* Supports key-entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSetMultimap(PlainOptions)}.
*
* @param type of key
* @param type of value
* @param options instance options
* @return SetMultimapCache object
*/
RSetMultimapCache getSetMultimapCache(PlainOptions options);
/**
* Returns Set based Multimap instance by name.
* Supports key-entry eviction with a given TTL value.
* Doesn't allow duplications for values mapped to key.
*
* Uses Redis native commands for entry expiration and not a scheduled eviction task.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of object
* @return SetMultimapCache object
*/
RSetMultimapCacheNative getSetMultimapCacheNative(String name);
/**
* Returns Set based Multimap instance by name
* using provided codec for both map keys and values.
* Supports key-entry eviction with a given TTL value.
* Doesn't allow duplications for values mapped to key.
*
* Uses Redis native commands for entry expiration and not a scheduled eviction task.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param name name of object
* @param codec codec for keys and values
* @return SetMultimapCache object
*/
RSetMultimapCacheNative getSetMultimapCacheNative(String name, Codec codec);
/**
* Returns Set based Multimap instance with specified options
.
* Supports key-entry eviction with a given TTL value.
* Doesn't allow duplications for values mapped to key.
*
* Uses Redis native commands for entry expiration and not a scheduled eviction task.
*
* Requires Redis 7.4.0 and higher.
*
* @param type of key
* @param type of value
* @param options instance options
* @return SetMultimapCache object
*/
RSetMultimapCacheNative getSetMultimapCacheNative(PlainOptions options);
/**
* Returns semaphore instance by name
*
* @param name name of object
* @return Semaphore object
*/
RSemaphore getSemaphore(String name);
/**
* Returns semaphore instance with specified options
.
*
* @param options instance options
* @return Semaphore object
*/
RSemaphore getSemaphore(CommonOptions options);
/**
* Returns semaphore instance by name.
* Supports lease time parameter for each acquired permit.
*
* @param name name of object
* @return PermitExpirableSemaphore object
*/
RPermitExpirableSemaphore getPermitExpirableSemaphore(String name);
/**
* Returns semaphore instance with specified options
.
* Supports lease time parameter for each acquired permit.
*
* @param options instance options
* @return PermitExpirableSemaphore object
*/
RPermitExpirableSemaphore getPermitExpirableSemaphore(CommonOptions options);
/**
* Returns Lock instance by name.
*
* Implements a non-fair locking so doesn't guarantees an acquire order by threads.
*
* To increase reliability during failover, all operations wait for propagation to all Redis slaves.
*
* @param name name of object
* @return Lock object
*/
RLock getLock(String name);
/**
* Returns Lock instance with specified options
.
*
* Implements a non-fair locking so doesn't guarantees an acquire order by threads.
*
* To increase reliability during failover, all operations wait for propagation to all Redis slaves.
*
* @param options instance options
* @return Lock object
*/
RLock getLock(CommonOptions options);
/**
* Returns Spin lock instance by name.
*
* Implements a non-fair locking so doesn't guarantees an acquire order by threads.
*
* Lock doesn't use a pub/sub mechanism
*
* @param name name of object
* @return Lock object
*/
RLock getSpinLock(String name);
/**
* Returns Spin lock instance by name with specified back off options.
*
* Implements a non-fair locking so doesn't guarantees an acquire order by threads.
*
* Lock doesn't use a pub/sub mechanism
*
* @param name name of object
* @return Lock object
*/
RLock getSpinLock(String name, LockOptions.BackOff backOff);
/**
* Returns Fenced Lock instance by name.
*
* Implements a non-fair locking so doesn't guarantee an acquire order by threads.
*
* @param name name of object
* @return Lock object
*/
RFencedLock getFencedLock(String name);
/**
* Returns Fenced Lock instance with specified options
..
*
* Implements a non-fair locking so doesn't guarantee an acquire order by threads.
*
* @param options instance options
* @return Lock object
*/
RFencedLock getFencedLock(CommonOptions options);
/**
* Returns MultiLock instance associated with specified locks
*
* @param locks collection of locks
* @return MultiLock object
*/
RLock getMultiLock(RLock... locks);
/**
* Returns RedissonFasterMultiLock instance associated with specified group
and values
*
* @param group the group of values
* @param values lock values
* @return BatchLock object
*/
RLock getMultiLock(String group, Collection