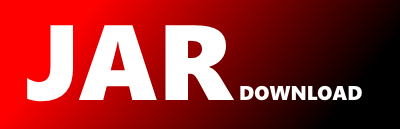
org.redisson.api.mapreduce.RCollectionMapReduce Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson-all Show documentation
Show all versions of redisson-all Show documentation
Easy Redis Java client and Real-Time Data Platform. Valkey compatible. Sync/Async/RxJava3/Reactive API. Client side caching. Over 50 Redis based Java objects and services: JCache API, Apache Tomcat, Hibernate, Spring, Set, Multimap, SortedSet, Map, List, Queue, Deque, Semaphore, Lock, AtomicLong, Map Reduce, Bloom filter, Scheduler, RPC
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api.mapreduce;
import java.util.concurrent.TimeUnit;
import org.redisson.api.RList;
import org.redisson.api.RScoredSortedSet;
import org.redisson.api.RSet;
import org.redisson.api.RSetCache;
import org.redisson.api.RSortedSet;
/**
*
* MapReduce allows to process large amount of data stored in
* {@link RSet}, {@link RList}, {@link RSetCache}, {@link RScoredSortedSet}, {@link RSortedSet} and others
* using Mapper, Reducer and/or Collator tasks launched across Redisson Nodes.
*
* Usage example:
*
*
* public class WordMapper implements RCollectionMapper<String, String, Integer> {
*
* public void map(String value, RCollector<String, Integer> collector) {
* String[] words = value.split("[^a-zA-Z]");
* for (String word : words) {
* collector.emit(word, 1);
* }
* }
*
* }
*
* public class WordReducer implements RReducer<String, Integer> {
*
* public Integer reduce(String reducedKey, Iterator<Integer> iter) {
* int sum = 0;
* while (iter.hasNext()) {
* Integer i = (Integer) iter.next();
* sum += i;
* }
* return sum;
* }
*
* }
*
* public class WordCollator implements RCollator<String, Integer, Integer> {
*
* public Integer collate(Map<String, Integer> resultMap) {
* int result = 0;
* for (Integer count : resultMap.values()) {
* result += count;
* }
* return result;
* }
*
* }
*
* RList<String> list = redisson.getList("myWords");
*
* Map<String, Integer> wordsCount = list.<String, Integer>mapReduce()
* .mapper(new WordMapper())
* .reducer(new WordReducer())
* .execute();
*
* Integer totalCount = list.<String, Integer>mapReduce()
* .mapper(new WordMapper())
* .reducer(new WordReducer())
* .execute(new WordCollator());
*
*
*
* @author Nikita Koksharov
*
* @param input value
* @param output key
* @param output value
*/
public interface RCollectionMapReduce extends RMapReduceExecutor {
/**
* Defines timeout for MapReduce process
*
* @param timeout for process
* @param unit of timeout
* @return self instance
*/
RCollectionMapReduce timeout(long timeout, TimeUnit unit);
/**
* Setup Mapper object
*
* @param mapper used during MapReduce
* @return self instance
*/
RCollectionMapReduce mapper(RCollectionMapper mapper);
/**
* Setup Reducer object
*
* @param reducer used during MapReduce
* @return self instance
*/
RCollectionMapReduce reducer(RReducer reducer);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy