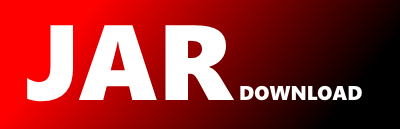
org.redisson.codec.ProtobufCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson-all Show documentation
Show all versions of redisson-all Show documentation
Easy Redis Java client and Real-Time Data Platform. Valkey compatible. Sync/Async/RxJava3/Reactive API. Client side caching. Over 50 Redis based Java objects and services: JCache API, Apache Tomcat, Hibernate, Spring, Set, Multimap, SortedSet, Map, List, Queue, Deque, Semaphore, Lock, AtomicLong, Map Reduce, Bloom filter, Scheduler, RPC
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.codec;
import com.fasterxml.jackson.databind.cfg.SerializerFactoryConfig;
import com.fasterxml.jackson.databind.ser.BasicSerializerFactory;
import com.google.protobuf.MessageLite;
import io.netty.buffer.ByteBuf;
import io.netty.buffer.ByteBufAllocator;
import io.netty.buffer.ByteBufInputStream;
import io.netty.buffer.ByteBufOutputStream;
import io.protostuff.LinkedBuffer;
import io.protostuff.ProtostuffIOUtil;
import io.protostuff.Schema;
import io.protostuff.runtime.RuntimeSchema;
import org.redisson.client.codec.BaseCodec;
import org.redisson.client.codec.Codec;
import org.redisson.client.handler.State;
import org.redisson.client.protocol.Decoder;
import org.redisson.client.protocol.Encoder;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Set;
public class ProtobufCodec extends BaseCodec {
private final Class> mapKeyClass;
private final Class> mapValueClass;
private final Class> valueClass;
//classes in blacklist will not be serialized using protobuf ,but instead will use blacklistCodec
private final Set protobufBlacklist;
//default value is JsonJacksonCodec
private final Codec blacklistCodec;
public ProtobufCodec(Class> mapKeyClass, Class> mapValueClass) {
this(mapKeyClass, mapValueClass, null, null);
}
/**
* @param blacklistCodec classes in protobufBlacklist will use this codec
*/
public ProtobufCodec(Class> mapKeyClass, Class> mapValueClass, Codec blacklistCodec) {
this(mapKeyClass, mapValueClass, null, blacklistCodec);
}
public ProtobufCodec(Class> valueClass) {
this(null, null, valueClass, null);
}
/**
* @param blacklistCodec classes in protobufBlacklist will use this codec
*/
public ProtobufCodec(Class> valueClass, Codec blacklistCodec) {
this(null, null, valueClass, blacklistCodec);
}
private ProtobufCodec(Class> mapKeyClass, Class> mapValueClass, Class> valueClass, Codec blacklistCodec) {
this.mapKeyClass = mapKeyClass;
this.mapValueClass = mapValueClass;
this.valueClass = valueClass;
if (blacklistCodec == null) {
this.blacklistCodec = new JsonJacksonCodec();
} else {
if (blacklistCodec instanceof ProtobufCodec) {
//will loop infinitely when encode or decode
throw new IllegalArgumentException("BlacklistCodec can not be ProtobufCodec");
}
this.blacklistCodec = blacklistCodec;
}
protobufBlacklist = new HashSet<>();
protobufBlacklist.addAll(BasicSerializerFactoryConcreteGetter.getConcreteKeySet());
protobufBlacklist.add(ArrayList.class.getName());
protobufBlacklist.add(HashSet.class.getName());
protobufBlacklist.add(HashMap.class.getName());
}
public void addBlacklist(Class> clazz) {
protobufBlacklist.add(clazz.getName());
}
public void removeBlacklist(Class> clazz) {
protobufBlacklist.remove(clazz.getName());
}
@Override
public Decoder
© 2015 - 2024 Weber Informatics LLC | Privacy Policy