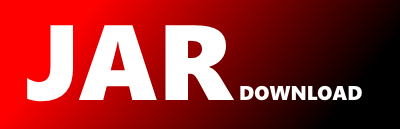
org.redisson.spring.cache.CacheConfig Maven / Gradle / Ivy
Show all versions of redisson-all Show documentation
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.spring.cache;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.Reader;
import java.net.URL;
import java.util.Map;
/**
* Cache config object used for Spring cache configuration.
*
* @author Nikita Koksharov
*
*/
public class CacheConfig {
private long ttl;
private long maxIdleTime;
private int maxSize;
/**
* Creates config object with
* ttl = 0
and maxIdleTime = 0
.
*
*/
public CacheConfig() {
}
/**
* Creates config object.
*
* @param ttl - time to live for key\value entry in milliseconds.
* If 0
then time to live doesn't affect entry expiration.
* @param maxIdleTime - max idle time for key\value entry in milliseconds.
*
* if maxIdleTime
and ttl
params are equal to 0
* then entry stores infinitely.
*/
public CacheConfig(long ttl, long maxIdleTime) {
super();
this.ttl = ttl;
this.maxIdleTime = maxIdleTime;
}
public long getTTL() {
return ttl;
}
/**
* Set time to live for key\value entry in milliseconds.
*
* @param ttl - time to live for key\value entry in milliseconds.
* If 0
then time to live doesn't affect entry expiration.
*/
public void setTTL(long ttl) {
this.ttl = ttl;
}
public int getMaxSize() {
return maxSize;
}
/**
* Set max size of map. Superfluous elements are evicted using LRU algorithm.
*
* @param maxSize - max size
* If 0
the cache is unbounded (default).
*/
public void setMaxSize(int maxSize) {
this.maxSize = maxSize;
}
public long getMaxIdleTime() {
return maxIdleTime;
}
/**
* Set max idle time for key\value entry in milliseconds.
*
* @param maxIdleTime - max idle time for key\value entry in milliseconds.
* If 0
then max idle time doesn't affect entry expiration.
*/
public void setMaxIdleTime(long maxIdleTime) {
this.maxIdleTime = maxIdleTime;
}
/**
* Read config objects stored in JSON format from String
*
* @param content of config
* @return config
* @throws IOException error
*/
public static Map fromJSON(String content) throws IOException {
return new CacheConfigSupport().fromJSON(content);
}
/**
* Read config objects stored in JSON format from InputStream
*
* @param inputStream of config
* @return config
* @throws IOException error
*/
public static Map fromJSON(InputStream inputStream) throws IOException {
return new CacheConfigSupport().fromJSON(inputStream);
}
/**
* Read config objects stored in JSON format from File
*
* @param file of config
* @return config
* @throws IOException error
*/
public static Map fromJSON(File file) throws IOException {
return new CacheConfigSupport().fromJSON(file);
}
/**
* Read config objects stored in JSON format from URL
*
* @param url of config
* @return config
* @throws IOException error
*/
public static Map fromJSON(URL url) throws IOException {
return new CacheConfigSupport().fromJSON(url);
}
/**
* Read config objects stored in JSON format from Reader
*
* @param reader of config
* @return config
* @throws IOException error
*/
public static Map fromJSON(Reader reader) throws IOException {
return new CacheConfigSupport().fromJSON(reader);
}
/**
* Convert current configuration to JSON format
*
* @param config object
* @return json string
* @throws IOException error
*/
public static String toJSON(Map config) throws IOException {
return new CacheConfigSupport().toJSON(config);
}
/**
* Read config objects stored in YAML format from String
*
* @param content of config
* @return config
* @throws IOException error
*/
public static Map fromYAML(String content) throws IOException {
return new CacheConfigSupport().fromYAML(content);
}
/**
* Read config objects stored in YAML format from InputStream
*
* @param inputStream of config
* @return config
* @throws IOException error
*/
public static Map fromYAML(InputStream inputStream) throws IOException {
return new CacheConfigSupport().fromYAML(inputStream);
}
/**
* Read config objects stored in YAML format from File
*
* @param file of config
* @return config
* @throws IOException error
*/
public static Map fromYAML(File file) throws IOException {
return new CacheConfigSupport().fromYAML(file);
}
/**
* Read config objects stored in YAML format from URL
*
* @param url of config
* @return config
* @throws IOException error
*/
public static Map fromYAML(URL url) throws IOException {
return new CacheConfigSupport().fromYAML(url);
}
/**
* Read config objects stored in YAML format from Reader
*
* @param reader of config
* @return config
* @throws IOException error
*/
public static Map fromYAML(Reader reader) throws IOException {
return new CacheConfigSupport().fromYAML(reader);
}
/**
* Convert current configuration to YAML format
*
* @param config map
* @return yaml string
* @throws IOException error
*/
public static String toYAML(Map config) throws IOException {
return new CacheConfigSupport().toYAML(config);
}
}