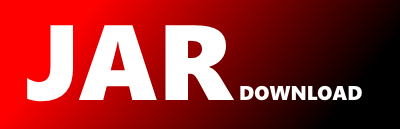
org.redisson.spring.cache.RedissonSpringCacheManager Maven / Gradle / Ivy
Show all versions of redisson-all Show documentation
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.spring.cache;
import java.io.IOException;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.ConcurrentHashMap;
import org.redisson.api.RMap;
import org.redisson.api.RMapCache;
import org.redisson.api.RedissonClient;
import org.redisson.client.codec.Codec;
import org.springframework.beans.factory.BeanDefinitionStoreException;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.cache.Cache;
import org.springframework.cache.CacheManager;
import org.springframework.cache.transaction.TransactionAwareCacheDecorator;
import org.springframework.context.ResourceLoaderAware;
import org.springframework.core.io.Resource;
import org.springframework.core.io.ResourceLoader;
/**
* A {@link org.springframework.cache.CacheManager} implementation
* backed by Redisson instance.
*
* @author Nikita Koksharov
*
*/
@SuppressWarnings("unchecked")
public class RedissonSpringCacheManager implements CacheManager, ResourceLoaderAware, InitializingBean {
ResourceLoader resourceLoader;
private boolean dynamic = true;
private boolean allowNullValues = true;
private boolean transactionAware = false;
Codec codec;
RedissonClient redisson;
Map configMap = new ConcurrentHashMap();
ConcurrentMap instanceMap = new ConcurrentHashMap();
String configLocation;
/**
* Creates CacheManager supplied by Redisson instance
*
* @param redisson object
*/
public RedissonSpringCacheManager(RedissonClient redisson) {
this(redisson, (String) null, null);
}
/**
* Creates CacheManager supplied by Redisson instance and
* Cache config mapped by Cache name
*
* @param redisson object
* @param config object
*/
public RedissonSpringCacheManager(RedissonClient redisson, Map config) {
this(redisson, config, null);
}
/**
* Creates CacheManager supplied by Redisson instance, Codec instance
* and Cache config mapped by Cache name.
*
* Each Cache instance share one Codec instance.
*
* @param redisson object
* @param config object
* @param codec object
*/
public RedissonSpringCacheManager(RedissonClient redisson, Map config, Codec codec) {
this.redisson = redisson;
this.configMap = (Map) config;
this.codec = codec;
}
/**
* Creates CacheManager supplied by Redisson instance
* and Cache config mapped by Cache name.
*
* Loads the config file from the class path, interpreting plain paths as class path resource names
* that include the package path (e.g. "mypackage/myresource.txt").
*
* @param redisson object
* @param configLocation path
*/
public RedissonSpringCacheManager(RedissonClient redisson, String configLocation) {
this(redisson, configLocation, null);
}
/**
* Creates CacheManager supplied by Redisson instance, Codec instance
* and Config location path.
*
* Each Cache instance share one Codec instance.
*
* Loads the config file from the class path, interpreting plain paths as class path resource names
* that include the package path (e.g. "mypackage/myresource.txt").
*
* @param redisson object
* @param configLocation path
* @param codec object
*/
public RedissonSpringCacheManager(RedissonClient redisson, String configLocation, Codec codec) {
this.redisson = redisson;
this.configLocation = configLocation;
this.codec = codec;
}
/**
* Defines possibility of storing {@code null} values.
*
* Default is true
*
* @param allowNullValues stores if true
*/
public void setAllowNullValues(boolean allowNullValues) {
this.allowNullValues = allowNullValues;
}
/**
* Defines if cache aware of Spring-managed transactions.
* If {@code true} put/evict operations are executed only for successful transaction in after-commit phase.
*
* Default is false
*
* @param transactionAware cache is transaction aware if true
*/
public void setTransactionAware(boolean transactionAware) {
this.transactionAware = transactionAware;
}
/**
* Defines 'fixed' cache names.
* A new cache instance will not be created in dynamic for non-defined names.
*
* `null` parameter setups dynamic mode
*
* @param names of caches
*/
public void setCacheNames(Collection names) {
if (names != null) {
for (String name : names) {
getCache(name);
}
dynamic = false;
} else {
dynamic = true;
}
}
/**
* Set cache config location
*
* @param configLocation object
*/
public void setConfigLocation(String configLocation) {
this.configLocation = configLocation;
}
/**
* Set cache config mapped by cache name
*
* @param config object
*/
public void setConfig(Map config) {
this.configMap = (Map) config;
}
/**
* Set Redisson instance
*
* @param redisson instance
*/
public void setRedisson(RedissonClient redisson) {
this.redisson = redisson;
}
/**
* Set Codec instance shared between all Cache instances
*
* @param codec object
*/
public void setCodec(Codec codec) {
this.codec = codec;
}
protected CacheConfig createDefaultConfig() {
return new CacheConfig();
}
@Override
public Cache getCache(String name) {
Cache cache = instanceMap.get(name);
if (cache != null) {
return cache;
}
if (!dynamic) {
return cache;
}
CacheConfig config = configMap.get(name);
if (config == null) {
config = createDefaultConfig();
configMap.put(name, config);
}
if (config.getMaxIdleTime() == 0 && config.getTTL() == 0 && config.getMaxSize() == 0) {
return createMap(name, config);
}
return createMapCache(name, config);
}
private Cache createMap(String name, CacheConfig config) {
RMap