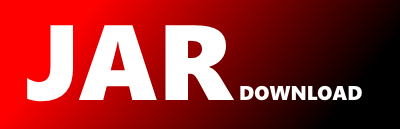
io.reactivex.rxjava3.internal.schedulers.InstantPeriodicTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson-all Show documentation
Show all versions of redisson-all Show documentation
Easy Redis Java client and Real-Time Data Platform. Valkey compatible. Sync/Async/RxJava3/Reactive API. Client side caching. Over 50 Redis based Java objects and services: JCache API, Apache Tomcat, Hibernate, Spring, Set, Multimap, SortedSet, Map, List, Queue, Deque, Semaphore, Lock, AtomicLong, Map Reduce, Bloom filter, Scheduler, RPC
The newest version!
/*
* Copyright (c) 2016-present, RxJava Contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See
* the License for the specific language governing permissions and limitations under the License.
*/
package io.reactivex.rxjava3.internal.schedulers;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicReference;
import io.reactivex.rxjava3.disposables.Disposable;
import io.reactivex.rxjava3.internal.functions.Functions;
import io.reactivex.rxjava3.plugins.RxJavaPlugins;
/**
* Wrapper for a regular task that gets immediately rescheduled when the task completed.
*/
final class InstantPeriodicTask implements Callable, Disposable {
final Runnable task;
final AtomicReference> rest;
final AtomicReference> first;
final ExecutorService executor;
Thread runner;
static final FutureTask CANCELLED = new FutureTask<>(Functions.EMPTY_RUNNABLE, null);
InstantPeriodicTask(Runnable task, ExecutorService executor) {
super();
this.task = task;
this.first = new AtomicReference<>();
this.rest = new AtomicReference<>();
this.executor = executor;
}
@Override
public Void call() {
runner = Thread.currentThread();
try {
task.run();
runner = null;
setRest(executor.submit(this));
} catch (Throwable ex) {
// Exceptions.throwIfFatal(ex); nowhere to go
runner = null;
RxJavaPlugins.onError(ex);
throw ex;
}
return null;
}
@Override
public void dispose() {
Future> current = first.getAndSet(CANCELLED);
if (current != null && current != CANCELLED) {
current.cancel(runner != Thread.currentThread());
}
current = rest.getAndSet(CANCELLED);
if (current != null && current != CANCELLED) {
current.cancel(runner != Thread.currentThread());
}
}
@Override
public boolean isDisposed() {
return first.get() == CANCELLED;
}
void setFirst(Future> f) {
for (;;) {
Future> current = first.get();
if (current == CANCELLED) {
f.cancel(runner != Thread.currentThread());
return;
}
if (first.compareAndSet(current, f)) {
return;
}
}
}
void setRest(Future> f) {
for (;;) {
Future> current = rest.get();
if (current == CANCELLED) {
f.cancel(runner != Thread.currentThread());
return;
}
if (rest.compareAndSet(current, f)) {
return;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy