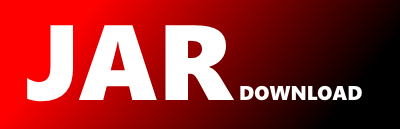
com.lambdaworks.redis.pubsub.PubSubOutput Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
// Copyright (C) 2011 - Will Glozer. All rights reserved.
package com.lambdaworks.redis.pubsub;
import com.lambdaworks.redis.codec.RedisCodec;
import com.lambdaworks.redis.protocol.CommandOutput;
import java.nio.ByteBuffer;
/**
* One element of the redis pub/sub stream. May be a message or notification
* of subscription details.
*
* @param Value type.
*
* @author Will Glozer
*/
public class PubSubOutput extends CommandOutput {
enum Type { message, pmessage, psubscribe, punsubscribe, subscribe, unsubscribe }
private Type type;
private String channel;
private String pattern;
private long count;
public PubSubOutput(RedisCodec codec) {
super(codec, null);
}
public Type type() {
return type;
}
public String channel() {
return channel;
}
public String pattern() {
return pattern;
}
public long count() {
return count;
}
@Override
@SuppressWarnings("fallthrough")
public void set(ByteBuffer bytes) {
if (type == null) {
type = Type.valueOf(decodeAscii(bytes));
return;
}
switch (type) {
case pmessage:
if (pattern == null) {
pattern = decodeAscii(bytes);
break;
}
case message:
if (channel == null) {
channel = decodeAscii(bytes);
break;
}
if (channel.startsWith("__keyspace@")
|| channel.startsWith("__keyevent@")) {
output = (V)decodeAscii(bytes);
} else {
output = codec.decodeValue(bytes);
}
break;
case psubscribe:
case punsubscribe:
pattern = decodeAscii(bytes);
break;
case subscribe:
case unsubscribe:
channel = decodeAscii(bytes);
break;
}
}
@Override
public void set(long integer) {
count = integer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy