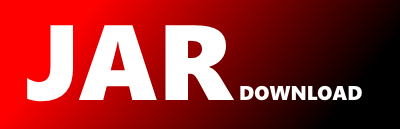
org.redisson.RedissonRx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2019 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson;
import org.redisson.api.BatchOptions;
import org.redisson.api.ClusterNode;
import org.redisson.api.MapOptions;
import org.redisson.api.Node;
import org.redisson.api.NodesGroup;
import org.redisson.api.RAtomicDoubleRx;
import org.redisson.api.RAtomicLongRx;
import org.redisson.api.RBatchRx;
import org.redisson.api.RBitSetRx;
import org.redisson.api.RBlockingDequeRx;
import org.redisson.api.RBlockingQueueRx;
import org.redisson.api.RBucketRx;
import org.redisson.api.RDequeRx;
import org.redisson.api.RGeoRx;
import org.redisson.api.RHyperLogLogRx;
import org.redisson.api.RKeysRx;
import org.redisson.api.RLexSortedSetRx;
import org.redisson.api.RListMultimapRx;
import org.redisson.api.RListRx;
import org.redisson.api.RLockRx;
import org.redisson.api.RMapCacheRx;
import org.redisson.api.RMapRx;
import org.redisson.api.RPatternTopicRx;
import org.redisson.api.RPermitExpirableSemaphoreRx;
import org.redisson.api.RQueueRx;
import org.redisson.api.RRateLimiterRx;
import org.redisson.api.RReadWriteLockRx;
import org.redisson.api.RScoredSortedSetRx;
import org.redisson.api.RScriptRx;
import org.redisson.api.RSemaphoreRx;
import org.redisson.api.RSetCache;
import org.redisson.api.RSetCacheRx;
import org.redisson.api.RSetMultimapRx;
import org.redisson.api.RSetRx;
import org.redisson.api.RStreamRx;
import org.redisson.api.RTopic;
import org.redisson.api.RTopicRx;
import org.redisson.api.RTransactionRx;
import org.redisson.api.RedissonRxClient;
import org.redisson.api.TransactionOptions;
import org.redisson.client.codec.Codec;
import org.redisson.config.Config;
import org.redisson.config.ConfigSupport;
import org.redisson.connection.ConnectionManager;
import org.redisson.eviction.EvictionScheduler;
import org.redisson.pubsub.SemaphorePubSub;
import org.redisson.rx.CommandRxExecutor;
import org.redisson.rx.CommandRxService;
import org.redisson.rx.RedissonBatchRx;
import org.redisson.rx.RedissonBlockingDequeRx;
import org.redisson.rx.RedissonBlockingQueueRx;
import org.redisson.rx.RedissonKeysRx;
import org.redisson.rx.RedissonLexSortedSetRx;
import org.redisson.rx.RedissonListMultimapRx;
import org.redisson.rx.RedissonListRx;
import org.redisson.rx.RedissonMapCacheRx;
import org.redisson.rx.RedissonMapRx;
import org.redisson.rx.RedissonReadWriteLockRx;
import org.redisson.rx.RedissonScoredSortedSetRx;
import org.redisson.rx.RedissonSetCacheRx;
import org.redisson.rx.RedissonSetMultimapRx;
import org.redisson.rx.RedissonSetRx;
import org.redisson.rx.RedissonTopicRx;
import org.redisson.rx.RedissonTransactionRx;
import org.redisson.rx.RxProxyBuilder;
/**
* Main infrastructure class allows to get access
* to all Redisson objects on top of Redis server.
*
* @author Nikita Koksharov
*
*/
public class RedissonRx implements RedissonRxClient {
protected final EvictionScheduler evictionScheduler;
protected final CommandRxExecutor commandExecutor;
protected final ConnectionManager connectionManager;
protected final Config config;
protected final SemaphorePubSub semaphorePubSub = new SemaphorePubSub();
protected RedissonRx(Config config) {
this.config = config;
Config configCopy = new Config(config);
connectionManager = ConfigSupport.createConnectionManager(configCopy);
commandExecutor = new CommandRxService(connectionManager);
evictionScheduler = new EvictionScheduler(commandExecutor);
}
@Override
public RStreamRx getStream(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonStream(commandExecutor, name), RStreamRx.class);
}
@Override
public RStreamRx getStream(String name, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonStream(codec, commandExecutor, name), RStreamRx.class);
}
@Override
public RGeoRx getGeo(String name) {
RedissonScoredSortedSet set = new RedissonScoredSortedSet(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, new RedissonGeo(commandExecutor, name, null),
new RedissonScoredSortedSetRx(set), RGeoRx.class);
}
@Override
public RGeoRx getGeo(String name, Codec codec) {
RedissonScoredSortedSet set = new RedissonScoredSortedSet(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, new RedissonGeo(codec, commandExecutor, name, null),
new RedissonScoredSortedSetRx(set), RGeoRx.class);
}
@Override
public RLockRx getFairLock(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonFairLock(commandExecutor, name), RLockRx.class);
}
@Override
public RRateLimiterRx getRateLimiter(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonRateLimiter(commandExecutor, name), RRateLimiterRx.class);
}
@Override
public RSemaphoreRx getSemaphore(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonSemaphore(commandExecutor, name, semaphorePubSub), RSemaphoreRx.class);
}
@Override
public RPermitExpirableSemaphoreRx getPermitExpirableSemaphore(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonPermitExpirableSemaphore(commandExecutor, name, semaphorePubSub), RPermitExpirableSemaphoreRx.class);
}
@Override
public RReadWriteLockRx getReadWriteLock(String name) {
return new RedissonReadWriteLockRx(commandExecutor, name);
}
@Override
public RLockRx getLock(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonLock(commandExecutor, name), RLockRx.class);
}
@Override
public RMapCacheRx getMapCache(String name, Codec codec) {
RedissonMapCache map = new RedissonMapCache(codec, evictionScheduler, commandExecutor, name, null, null);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapCacheRx(map), RMapCacheRx.class);
}
@Override
public RMapCacheRx getMapCache(String name) {
RedissonMapCache map = new RedissonMapCache(evictionScheduler, commandExecutor, name, null, null);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapCacheRx(map), RMapCacheRx.class);
}
@Override
public RBucketRx getBucket(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonBucket(commandExecutor, name), RBucketRx.class);
}
@Override
public RBucketRx getBucket(String name, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonBucket(codec, commandExecutor, name), RBucketRx.class);
}
@Override
public RHyperLogLogRx getHyperLogLog(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonHyperLogLog(commandExecutor, name), RHyperLogLogRx.class);
}
@Override
public RHyperLogLogRx getHyperLogLog(String name, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonHyperLogLog(codec, commandExecutor, name), RHyperLogLogRx.class);
}
@Override
public RListRx getList(String name) {
RedissonList list = new RedissonList(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, list,
new RedissonListRx(list), RListRx.class);
}
@Override
public RListRx getList(String name, Codec codec) {
RedissonList list = new RedissonList(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, list,
new RedissonListRx(list), RListRx.class);
}
@Override
public RListMultimapRx getListMultimap(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonListMultimap(commandExecutor, name),
new RedissonListMultimapRx(commandExecutor, name), RListMultimapRx.class);
}
@Override
public RListMultimapRx getListMultimap(String name, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonListMultimap(codec, commandExecutor, name),
new RedissonListMultimapRx(codec, commandExecutor, name), RListMultimapRx.class);
}
@Override
public RSetMultimapRx getSetMultimap(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonSetMultimap(commandExecutor, name),
new RedissonSetMultimapRx(commandExecutor, name, this), RSetMultimapRx.class);
}
@Override
public RSetMultimapRx getSetMultimap(String name, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonSetMultimap(codec, commandExecutor, name),
new RedissonSetMultimapRx(codec, commandExecutor, name, this), RSetMultimapRx.class);
}
@Override
public RMapRx getMap(String name) {
RedissonMap map = new RedissonMap(commandExecutor, name, null, null);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapRx(map, this), RMapRx.class);
}
@Override
public RMapRx getMap(String name, Codec codec) {
RedissonMap map = new RedissonMap(codec, commandExecutor, name, null, null);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapRx(map, this), RMapRx.class);
}
@Override
public RSetRx getSet(String name) {
RedissonSet set = new RedissonSet(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonSetRx(set, this), RSetRx.class);
}
@Override
public RSetRx getSet(String name, Codec codec) {
RedissonSet set = new RedissonSet(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonSetRx(set, this), RSetRx.class);
}
@Override
public RScoredSortedSetRx getScoredSortedSet(String name) {
RedissonScoredSortedSet set = new RedissonScoredSortedSet(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonScoredSortedSetRx(set), RScoredSortedSetRx.class);
}
@Override
public RScoredSortedSetRx getScoredSortedSet(String name, Codec codec) {
RedissonScoredSortedSet set = new RedissonScoredSortedSet(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonScoredSortedSetRx(set), RScoredSortedSetRx.class);
}
@Override
public RLexSortedSetRx getLexSortedSet(String name) {
RedissonLexSortedSet set = new RedissonLexSortedSet(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonLexSortedSetRx(set), RLexSortedSetRx.class);
}
@Override
public RTopicRx getTopic(String name) {
RTopic topic = new RedissonTopic(commandExecutor, name);
return RxProxyBuilder.create(commandExecutor, topic, new RedissonTopicRx(topic), RTopicRx.class);
}
@Override
public RTopicRx getTopic(String name, Codec codec) {
RTopic topic = new RedissonTopic(codec, commandExecutor, name);
return RxProxyBuilder.create(commandExecutor, topic, new RedissonTopicRx(topic), RTopicRx.class);
}
@Override
public RPatternTopicRx getPatternTopic(String pattern) {
return RxProxyBuilder.create(commandExecutor, new RedissonPatternTopic(commandExecutor, pattern), RPatternTopicRx.class);
}
@Override
public RPatternTopicRx getPatternTopic(String pattern, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonPatternTopic(codec, commandExecutor, pattern), RPatternTopicRx.class);
}
@Override
public RQueueRx getQueue(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonQueue(commandExecutor, name, null),
new RedissonListRx(new RedissonList(commandExecutor, name, null)), RQueueRx.class);
}
@Override
public RQueueRx getQueue(String name, Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonQueue(codec, commandExecutor, name, null),
new RedissonListRx(new RedissonList(codec,commandExecutor, name, null)), RQueueRx.class);
}
@Override
public RBlockingQueueRx getBlockingQueue(String name) {
RedissonBlockingQueue queue = new RedissonBlockingQueue(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, queue,
new RedissonBlockingQueueRx(queue), RBlockingQueueRx.class);
}
@Override
public RBlockingQueueRx getBlockingQueue(String name, Codec codec) {
RedissonBlockingQueue queue = new RedissonBlockingQueue(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, queue,
new RedissonBlockingQueueRx(queue), RBlockingQueueRx.class);
}
@Override
public RDequeRx getDeque(String name) {
RedissonDeque queue = new RedissonDeque(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, queue,
new RedissonListRx(queue), RDequeRx.class);
}
@Override
public RDequeRx getDeque(String name, Codec codec) {
RedissonDeque queue = new RedissonDeque(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, queue,
new RedissonListRx(queue), RDequeRx.class);
}
@Override
public RSetCacheRx getSetCache(String name) {
RSetCache set = new RedissonSetCache(evictionScheduler, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonSetCacheRx(set, this), RSetCacheRx.class);
}
@Override
public RSetCacheRx getSetCache(String name, Codec codec) {
RSetCache set = new RedissonSetCache(codec, evictionScheduler, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, set,
new RedissonSetCacheRx(set, this), RSetCacheRx.class);
}
@Override
public RAtomicLongRx getAtomicLong(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonAtomicLong(commandExecutor, name), RAtomicLongRx.class);
}
@Override
public RAtomicDoubleRx getAtomicDouble(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonAtomicDouble(commandExecutor, name), RAtomicDoubleRx.class);
}
@Override
public RBitSetRx getBitSet(String name) {
return RxProxyBuilder.create(commandExecutor, new RedissonBitSet(commandExecutor, name), RBitSetRx.class);
}
@Override
public RScriptRx getScript() {
return RxProxyBuilder.create(commandExecutor, new RedissonScript(commandExecutor), RScriptRx.class);
}
@Override
public RScriptRx getScript(Codec codec) {
return RxProxyBuilder.create(commandExecutor, new RedissonScript(commandExecutor, codec), RScriptRx.class);
}
@Override
public RBatchRx createBatch(BatchOptions options) {
RedissonBatchRx batch = new RedissonBatchRx(evictionScheduler, connectionManager, options);
if (config.isReferenceEnabled()) {
batch.enableRedissonReferenceSupport(this);
}
return batch;
}
@Override
public RKeysRx getKeys() {
return RxProxyBuilder.create(commandExecutor, new RedissonKeys(commandExecutor), new RedissonKeysRx(commandExecutor), RKeysRx.class);
}
@Override
public Config getConfig() {
return config;
}
@Override
public NodesGroup getNodesGroup() {
return new RedisNodes(connectionManager);
}
@Override
public NodesGroup getClusterNodesGroup() {
if (!connectionManager.isClusterMode()) {
throw new IllegalStateException("Redisson not in cluster mode!");
}
return new RedisNodes(connectionManager);
}
@Override
public void shutdown() {
connectionManager.shutdown();
}
@Override
public boolean isShutdown() {
return connectionManager.isShutdown();
}
@Override
public boolean isShuttingDown() {
return connectionManager.isShuttingDown();
}
protected void enableRedissonReferenceSupport() {
this.commandExecutor.enableRedissonReferenceSupport(this);
}
@Override
public RMapCacheRx getMapCache(String name, Codec codec, MapOptions options) {
RedissonMapCache map = new RedissonMapCache(codec, evictionScheduler, commandExecutor, name, null, options);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapCacheRx(map), RMapCacheRx.class);
}
@Override
public RMapCacheRx getMapCache(String name, MapOptions options) {
RedissonMapCache map = new RedissonMapCache(evictionScheduler, commandExecutor, name, null, options);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapCacheRx(map), RMapCacheRx.class);
}
@Override
public RMapRx getMap(String name, MapOptions options) {
RedissonMap map = new RedissonMap(commandExecutor, name, null, options);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapRx(map, this), RMapRx.class);
}
@Override
public RMapRx getMap(String name, Codec codec, MapOptions options) {
RedissonMap map = new RedissonMap(codec, commandExecutor, name, null, options);
return RxProxyBuilder.create(commandExecutor, map,
new RedissonMapRx(map, this), RMapRx.class);
}
@Override
public RTransactionRx createTransaction(TransactionOptions options) {
return new RedissonTransactionRx(commandExecutor, options);
}
@Override
public RBlockingDequeRx getBlockingDeque(String name) {
RedissonBlockingDeque deque = new RedissonBlockingDeque(commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, deque,
new RedissonBlockingDequeRx(deque), RBlockingDequeRx.class);
}
@Override
public RBlockingDequeRx getBlockingDeque(String name, Codec codec) {
RedissonBlockingDeque deque = new RedissonBlockingDeque(codec, commandExecutor, name, null);
return RxProxyBuilder.create(commandExecutor, deque,
new RedissonBlockingDequeRx(deque), RBlockingDequeRx.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy