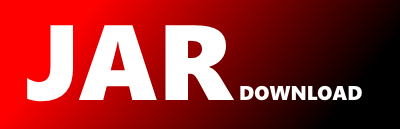
org.redisson.core.RMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright 2014 Nikita Koksharov, Nickolay Borbit
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.core;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentMap;
/**
* Distributed and concurrent implementation of {@link java.util.concurrent.ConcurrentMap}
* and {@link java.util.Map}
*
* This map doesn't allow to store null
as key or value.
*
* @author Nikita Koksharov
*
* @param key
* @param value
*/
public interface RMap extends ConcurrentMap, RExpirable, RMapAsync {
/**
* Atomically adds the given delta
to the current value
* by mapped key
.
*
* Works only for numeric values!
*
* @param key
* @param delta the value to add
* @return the updated value
*/
V addAndGet(K key, Number delta);
/**
* Gets a map slice contains the mappings with defined keys
* by one operation. This operation NOT traverses all map entries
* like any other filter*
method, so works faster.
*
* The returned map is NOT backed by the original map.
*
* @param keys map keys
* @return
*/
Map getAll(Set keys);
/**
* Returns a map slice containing the mappings in whose Map.Entry<K, V> entries
* satisfy a predicate. This operation traverses all map entries with small memory footprint.
*
* The returned map is NOT backed by the original map.
*
* @param predicate
* @return
*/
@Deprecated
Map filterEntries(Predicate> predicate);
/**
* Returns a map slice containing the mappings in whose values
* satisfy a predicate. Traverses all map entries with small memory footprint.
*
* The returned map is NOT backed by the original map.
*
* @param predicate
* @return
*/
@Deprecated
Map filterValues(Predicate predicate);
/**
* Returns a map slice containing the mappings in whose keys
* satisfy a predicate. Traverses all map entries with small memory footprint.
*
* The returned map is NOT backed by the original map.
*
* @param predicate
* @return
*/
@Deprecated
Map filterKeys(Predicate predicate);
/**
* Removes keys
from map by one operation
*
* Works faster than RMap.remove
but not returning
* the value associated with key
*
* @param keys
* @return the number of keys that were removed from the hash, not including specified but non existing keys
*/
long fastRemove(K ... keys);
/**
* Associates the specified value
with the specified key
.
*
* Works faster than RMap.put
but not returning
* the previous value associated with key
*
* @param key
* @param value
* @return true
if key is a new key in the hash and value was set.
* false
if key already exists in the hash and the value was updated.
*/
boolean fastPut(K key, V value);
boolean fastPutIfAbsent(K key, V value);
/**
* Read all keys at once
*
* @return
*/
Set readAllKeySet();
/**
* Read all values at once
*
* @return
*/
Collection readAllValues();
/**
* Read all map entries at once
*
* @return
*/
Set> readAllEntrySet();
/**
* Use {@link #entrySet().iterator()}
*
*/
@Deprecated
Iterator> entryIterator();
/**
* Use {@link #keySet().iterator()}
*
*/
@Deprecated
Iterator keyIterator();
/**
* Use {@link #values().iterator()}
*
*/
@Deprecated
Iterator valueIterator();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy