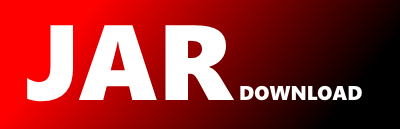
org.redisson.api.RBatchReactive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright 2014 Nikita Koksharov, Nickolay Borbit
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import java.util.List;
import org.reactivestreams.Publisher;
import org.redisson.client.codec.Codec;
/**
* Interface for using pipeline feature.
*
* All method invocations on objects
* from this interface are batched to separate queue and could be executed later
* with execute()
method.
*
*
* @author Nikita Koksharov
*
*/
public interface RBatchReactive {
/**
* Returns set-based cache instance by name
.
* Uses map (value_hash, value) under the hood for minimal memory consumption.
* Supports value eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSet(String, Codec)}.
*
* @param name
* @param codec
* @return
*/
RSetCacheReactive getSetCache(String name);
/**
* Returns set-based cache instance by name
* using provided codec
for values.
* Uses map (value_hash, value) under the hood for minimal memory consumption.
* Supports value eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getSet(String, Codec)}.
*
* @param name
* @param codec
* @return
*/
RSetCacheReactive getSetCache(String name, Codec codec);
/**
* Returns map-based cache instance by name
* using provided codec
for both cache keys and values.
* Supports entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getMap(String, Codec)}.
*
* @param name
* @param codec
* @return
*/
RMapCacheReactive getMapCache(String name, Codec codec);
/**
* Returns map-based cache instance by name
.
* Supports entry eviction with a given TTL value.
*
* If eviction is not required then it's better to use regular map {@link #getMap(String)}.
*
* @param name
* @return
*/
RMapCacheReactive getMapCache(String name);
/**
* Returns object holder by name
*
* @param name of object
* @return
*/
RBucketReactive getBucket(String name);
RBucketReactive getBucket(String name, Codec codec);
/**
* Returns HyperLogLog object
*
* @param name of object
* @return
*/
RHyperLogLogReactive getHyperLogLog(String name);
RHyperLogLogReactive getHyperLogLog(String name, Codec codec);
/**
* Returns list instance by name.
*
* @param name of list
* @return
*/
RListReactive getList(String name);
RListReactive getList(String name, Codec codec);
/**
* Returns map instance by name.
*
* @param name of map
* @return
*/
RMapReactive getMap(String name);
RMapReactive getMap(String name, Codec codec);
/**
* Returns set instance by name.
*
* @param name of set
* @return
*/
RSetReactive getSet(String name);
RSetReactive getSet(String name, Codec codec);
/**
* Returns topic instance by name.
*
* @param name of topic
* @return
*/
RTopicReactive getTopic(String name);
RTopicReactive getTopic(String name, Codec codec);
/**
* Returns queue instance by name.
*
* @param name of queue
* @return
*/
RQueueReactive getQueue(String name);
RQueueReactive getQueue(String name, Codec codec);
/**
* Returns blocking queue instance by name.
*
* @param name of queue
* @return
*/
RBlockingQueueReactive getBlockingQueue(String name);
RBlockingQueueReactive getBlockingQueue(String name, Codec codec);
/**
* Returns deque instance by name.
*
* @param name of deque
* @return
*/
RDequeReactive getDequeReactive(String name);
RDequeReactive getDequeReactive(String name, Codec codec);
/**
* Returns "atomic long" instance by name.
*
* @param name of the "atomic long"
* @return
*/
RAtomicLongReactive getAtomicLongReactive(String name);
/**
* Returns Redis Sorted Set instance by name
*
* @param name
* @return
*/
RScoredSortedSetReactive getScoredSortedSet(String name);
RScoredSortedSetReactive getScoredSortedSet(String name, Codec codec);
/**
* Returns String based Redis Sorted Set instance by name
* All elements are inserted with the same score during addition,
* in order to force lexicographical ordering
*
* @param name
* @return
*/
RLexSortedSetReactive getLexSortedSet(String name);
/**
* Returns bitSet instance by name.
*
* @param name of bitSet
* @return
*/
RBitSetReactive getBitSet(String name);
/**
* Returns script operations object
*
* @return
*/
RScriptReactive getScript();
/**
* Returns keys operations.
* Each of Redis/Redisson object associated with own key
*
* @return
*/
RKeysReactive getKeys();
/**
* Executes all operations accumulated during Reactive methods invocations Reactivehronously.
*
* In cluster configurations operations grouped by slot ids
* so may be executed on different servers. Thus command execution order could be changed
*
* @return List with result object for each command
*/
Publisher> execute();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy