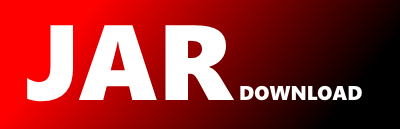
org.redisson.api.RSetReactive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2020 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.util.Set;
/**
* Reactive interface for Redis based implementation of {@link java.util.Set}
*
* @author Nikita Koksharov
*
* @param type of value
*/
public interface RSetReactive extends RCollectionReactive, RSortableReactive> {
/**
* Returns RPermitExpirableSemaphore
instance associated with value
*
* @param value - set value
* @return RPermitExpirableSemaphore object
*/
RPermitExpirableSemaphoreReactive getPermitExpirableSemaphore(V value);
/**
* Returns RSemaphore
instance associated with value
*
* @param value - set value
* @return RSemaphore object
*/
RSemaphoreReactive getSemaphore(V value);
/**
* Returns RLock
instance associated with value
*
* @param value - set value
* @return RLock object
*/
RLockReactive getFairLock(V value);
/**
* Returns RReadWriteLock
instance associated with value
*
* @param value - set value
* @return RReadWriteLock object
*/
RReadWriteLockReactive getReadWriteLock(V value);
/**
* Returns lock instance associated with value
*
* @param value - set value
* @return RLock object
*/
RLockReactive getLock(V value);
/**
* Returns elements iterator fetches elements in a batch.
* Batch size is defined by count
param.
*
* @param count - size of elements batch
* @return iterator
*/
Flux iterator(int count);
/**
* Returns elements iterator fetches elements in a batch.
* Batch size is defined by count
param.
* If pattern is not null then only elements match this pattern are loaded.
*
* @param pattern - search pattern
* @param count - size of elements batch
* @return iterator
*/
Flux iterator(String pattern, int count);
/**
* Returns elements iterator.
* If pattern
is not null then only elements match this pattern are loaded.
*
* @param pattern - search pattern
* @return iterator
*/
Flux iterator(String pattern);
/**
* Removes and returns random elements limited by amount
*
* @param amount of random elements
* @return random elements
*/
Mono> removeRandom(int amount);
/**
* Removes and returns random element
*
* @return random element
*/
Mono removeRandom();
/**
* Returns random element
*
* @return random element
*/
Mono random();
/**
* Returns random elements from set limited by count
*
* @param count - values amount to return
* @return random elements
*/
Mono> random(int count);
/**
* Move a member from this set to the given destination set in async mode.
*
* @param destination the destination set
* @param member the member to move
* @return true if the element is moved, false if the element is not a
* member of this set or no operation was performed
*/
Mono move(String destination, V member);
/**
* Read all elements at once
*
* @return values
*/
Mono> readAll();
/**
* Union sets specified by name and write to current set.
* If current set already exists, it is overwritten.
*
* @param names - name of sets
* @return size of union
*/
Mono union(String... names);
/**
* Union sets specified by name with current set.
* Without current set state change.
*
* @param names - name of sets
* @return size of union
*/
Mono> readUnion(String... names);
/**
* Diff sets specified by name and write to current set.
* If current set already exists, it is overwritten.
*
* @param names - name of sets
* @return size of diff
*/
Mono diff(String... names);
/**
* Diff sets specified by name with current set.
* Without current set state change.
*
* @param names - name of sets
* @return values
*/
Mono> readDiff(String... names);
/**
* Intersection sets specified by name and write to current set.
* If current set already exists, it is overwritten.
*
* @param names - name of sets
* @return size of intersection
*/
Mono intersection(String... names);
/**
* Intersection sets specified by name with current set.
* Without current set state change.
*
* @param names - name of sets
* @return values
*/
Mono> readIntersection(String... names);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy