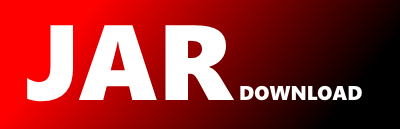
org.redisson.api.mapreduce.RMapReduce Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api.mapreduce;
import java.util.concurrent.TimeUnit;
/**
*
* MapReduce allows to process large amount of data stored in Redis map
* using Mapper, Reducer and/or Collator tasks launched across Redisson Nodes.
*
* Usage example:
*
*
* public class WordMapper implements RMapper<String, String, String, Integer> {
*
* public void map(String key, String value, RCollector<String, Integer> collector) {
* String[] words = value.split("[^a-zA-Z]");
* for (String word : words) {
* collector.emit(word, 1);
* }
* }
*
* }
*
* public class WordReducer implements RReducer<String, Integer> {
*
* public Integer reduce(String reducedKey, Iterator<Integer> iter) {
* int sum = 0;
* while (iter.hasNext()) {
* Integer i = (Integer) iter.next();
* sum += i;
* }
* return sum;
* }
*
* }
*
* public class WordCollator implements RCollator<String, Integer, Integer> {
*
* public Integer collate(Map<String, Integer> resultMap) {
* int result = 0;
* for (Integer count : resultMap.values()) {
* result += count;
* }
* return result;
* }
*
* }
*
* RMap<String, String> map = redisson.getMap("myWords");
*
* Map<String, Integer> wordsCount = map.<String, Integer>mapReduce()
* .mapper(new WordMapper())
* .reducer(new WordReducer())
* .execute();
*
* Integer totalCount = map.<String, Integer>mapReduce()
* .mapper(new WordMapper())
* .reducer(new WordReducer())
* .execute(new WordCollator());
*
*
*
* @author Nikita Koksharov
*
* @param input key
* @param input value
* @param output key
* @param output value
*/
public interface RMapReduce extends RMapReduceExecutor {
/**
* Defines timeout for MapReduce process.
* 0
means infinity timeout.
*
* @param timeout for process
* @param unit of timeout
* @return self instance
*/
RMapReduce timeout(long timeout, TimeUnit unit);
/**
* Setup Mapper object
*
* @param mapper used during MapReduce
* @return self instance
*/
RMapReduce mapper(RMapper mapper);
/**
* Setup Reducer object
*
* @param reducer used during MapReduce
* @return self instance
*/
RMapReduce reducer(RReducer reducer);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy