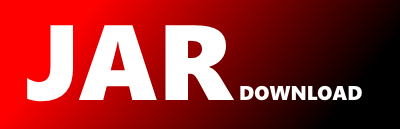
org.redisson.rx.RedissonTransactionRx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.rx;
import org.redisson.api.RBucketRx;
import org.redisson.api.RMap;
import org.redisson.api.RMapCache;
import org.redisson.api.RMapCacheRx;
import org.redisson.api.RMapRx;
import org.redisson.api.RSet;
import org.redisson.api.RSetCache;
import org.redisson.api.RSetCacheRx;
import org.redisson.api.RSetRx;
import org.redisson.api.RTransaction;
import org.redisson.api.RTransactionRx;
import org.redisson.api.TransactionOptions;
import org.redisson.client.codec.Codec;
import org.redisson.reactive.RedissonSetReactive;
import org.redisson.transaction.RedissonTransaction;
import io.reactivex.rxjava3.core.Completable;
/**
*
* @author Nikita Koksharov
*
*/
public class RedissonTransactionRx implements RTransactionRx {
private final RTransaction transaction;
private final CommandRxExecutor executorService;
public RedissonTransactionRx(CommandRxExecutor executorService, TransactionOptions options) {
this.transaction = new RedissonTransaction(executorService, options);
this.executorService = executorService;
}
@Override
public RBucketRx getBucket(String name) {
return RxProxyBuilder.create(executorService, transaction.getBucket(name), RBucketRx.class);
}
@Override
public RBucketRx getBucket(String name, Codec codec) {
return RxProxyBuilder.create(executorService, transaction.getBucket(name, codec), RBucketRx.class);
}
@Override
public RMapRx getMap(String name) {
RMap map = transaction.getMap(name);
return RxProxyBuilder.create(executorService, map,
new RedissonMapRx(map, null), RMapRx.class);
}
@Override
public RMapRx getMap(String name, Codec codec) {
RMap map = transaction.getMap(name, codec);
return RxProxyBuilder.create(executorService, map,
new RedissonMapRx(map, null), RMapRx.class);
}
@Override
public RMapCacheRx getMapCache(String name, Codec codec) {
RMapCache map = transaction.getMapCache(name, codec);
return RxProxyBuilder.create(executorService, map,
new RedissonMapCacheRx(map, executorService), RMapCacheRx.class);
}
@Override
public RMapCacheRx getMapCache(String name) {
RMapCache map = transaction.getMapCache(name);
return RxProxyBuilder.create(executorService, map,
new RedissonMapCacheRx(map, executorService), RMapCacheRx.class);
}
@Override
public RSetRx getSet(String name) {
RSet set = transaction.getSet(name);
return RxProxyBuilder.create(executorService, set,
new RedissonSetReactive(set, null), RSetRx.class);
}
@Override
public RSetRx getSet(String name, Codec codec) {
RSet set = transaction.getSet(name, codec);
return RxProxyBuilder.create(executorService, set,
new RedissonSetRx(set, null), RSetRx.class);
}
@Override
public RSetCacheRx getSetCache(String name) {
RSetCache set = transaction.getSetCache(name);
return RxProxyBuilder.create(executorService, set,
new RedissonSetCacheRx(set, null), RSetCacheRx.class);
}
@Override
public RSetCacheRx getSetCache(String name, Codec codec) {
RSetCache set = transaction.getSetCache(name, codec);
return RxProxyBuilder.create(executorService, set,
new RedissonSetCacheRx(set, null), RSetCacheRx.class);
}
@Override
public Completable commit() {
return executorService.flowable(() -> transaction.commitAsync()).ignoreElements();
}
@Override
public Completable rollback() {
return executorService.flowable(() -> transaction.rollbackAsync()).ignoreElements();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy