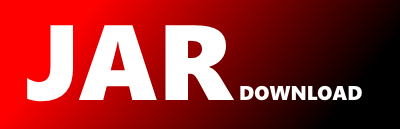
org.redisson.api.RDequeReactive Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import org.redisson.api.queue.DequeMoveArgs;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* Reactive interface for Deque object
*
* @author Nikita Koksharov
*
* @param the type of elements held in this collection
*/
public interface RDequeReactive extends RQueueReactive {
/**
* Adds element at the head of existing deque.
*
* @param elements - elements to add
* @return length of the list
*/
Mono addFirstIfExists(V... elements);
/**
* Adds element at the tail of existing deque.
*
* @param elements - elements to add
* @return length of the list
*/
Mono addLastIfExists(V... elements);
/**
* Adds elements at the head of deque.
*
* @param elements - elements to add
* @return length of the deque
*/
Mono addFirst(V... elements);
/**
* Adds elements at the tail of deque.
*
* @param elements - elements to add
* @return length of the deque
*/
Mono addLast(V... elements);
Flux descendingIterator();
/**
* Removes last occurrence of element o
*
* @param o - element
* @return true
if object has been removed otherwise false
*/
Mono removeLastOccurrence(Object o);
/**
* Retrieves and removes the last element of deque.
* Returns null
if there are no elements in deque.
*
* @return element
*/
Mono removeLast();
/**
* Retrieves and removes the first element of deque.
* Returns null
if there are no elements in deque.
*
* @return element
*/
Mono removeFirst();
/**
* Removes first occurrence of element o
*
* @param o - element to remove
* @return true
if object has been removed otherwise false
*/
Mono removeFirstOccurrence(Object o);
/**
* Adds element at the head of this deque.
*
* @param e - element to add
* @return void
*/
Mono push(V e);
/**
* Retrieves and removes element at the head of this deque.
* Returns null
if there are no elements in deque.
*
* @return element
*/
Mono pop();
/**
* Retrieves and removes element at the tail of this deque.
* Returns null
if there are no elements in deque.
*
* @return element
*/
Mono pollLast();
/**
* Retrieves and removes element at the head of this deque.
* Returns null
if there are no elements in deque.
*
* @return element
*/
Mono pollFirst();
/**
* Retrieves and removes the tail elements of this queue.
* Elements amount limited by limit
param.
*
* @return list of tail elements
*/
Flux pollLast(int limit);
/**
* Retrieves and removes the head elements of this queue.
* Elements amount limited by limit
param.
*
* @return list of head elements
*/
Flux pollFirst(int limit);
/**
* Returns element at the tail of this deque
* or null
if there are no elements in deque.
*
* @return element
*/
Mono peekLast();
/**
* Returns element at the head of this deque
* or null
if there are no elements in deque.
*
* @return element
*/
Mono peekFirst();
/**
* Adds element at the tail of this deque.
*
* @param e - element to add
* @return true
if element was added to this deque otherwise false
*/
Mono offerLast(V e);
/**
* Returns element at the tail of this deque
* or null
if there are no elements in deque.
*
* @return element
*/
Mono getLast();
/**
* Adds element at the tail of this deque.
*
* @param e - element to add
* @return void
*/
Mono addLast(V e);
/**
* Adds element at the head of this deque.
*
* @param e - element to add
* @return void
*/
Mono addFirst(V e);
/**
* Adds element at the head of this deque.
*
* @param e - element to add
* @return true
if element was added to this deque otherwise false
*/
Mono offerFirst(V e);
/**
* Move element from this deque to the given destination deque.
* Returns moved element.
*
* Usage examples:
*
* V element = deque.move(DequeMoveArgs.pollLast()
* .addFirstTo("deque2"));
*
*
* V elements = deque.move(DequeMoveArgs.pollFirst()
* .addLastTo("deque2"));
*
*
* Requires Redis 6.2.0 and higher.
*
* @param args - arguments object
* @return moved element
*/
Mono move(DequeMoveArgs args);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy