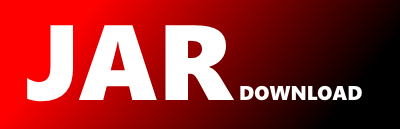
org.redisson.api.RAtomicDoubleAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
/**
* Distributed implementation to the AtomicDouble
*
* @author Nikita Koksharov
*
*/
public interface RAtomicDoubleAsync extends RExpirableAsync {
/**
* Atomically sets the value to the given updated value
* only if the current value {@code ==} the expected value.
*
* @param expect the expected value
* @param update the new value
* @return true if successful; or false if the actual value
* was not equal to the expected value.
*/
RFuture compareAndSetAsync(double expect, double update);
/**
* Atomically adds the given value to the current value.
*
* @param delta the value to add
* @return the updated value
*/
RFuture addAndGetAsync(double delta);
/**
* Atomically decrements the current value by one.
*
* @return the updated value
*/
RFuture decrementAndGetAsync();
/**
* Returns current value.
*
* @return current value
*/
RFuture getAsync();
/**
* Returns and deletes object
*
* @return the current value
*/
RFuture getAndDeleteAsync();
/**
* Atomically adds the given value to the current value.
*
* @param delta the value to add
* @return the updated value
*/
RFuture getAndAddAsync(double delta);
/**
* Atomically sets the given value and returns the old value.
*
* @param newValue the new value
* @return the old value
*/
RFuture getAndSetAsync(double newValue);
/**
* Atomically increments the current value by one.
*
* @return the updated value
*/
RFuture incrementAndGetAsync();
/**
* Atomically increments the current value by one.
*
* @return the old value
*/
RFuture getAndIncrementAsync();
/**
* Atomically decrements by one the current value.
*
* @return the previous value
*/
RFuture getAndDecrementAsync();
/**
* Atomically sets the given value.
*
* @param newValue the new value
* @return void
*/
RFuture setAsync(double newValue);
/**
* Adds object event listener
*
* @see org.redisson.api.listener.IncrByListener
* @see org.redisson.api.ExpiredObjectListener
* @see org.redisson.api.DeletedObjectListener
*
* @param listener - object event listener
* @return listener id
*/
RFuture addListenerAsync(ObjectListener listener);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy