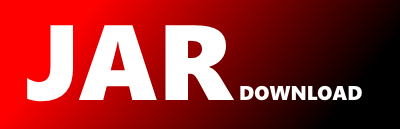
org.redisson.api.RBitSetRx Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of redisson Show documentation
Show all versions of redisson Show documentation
Redis Java client with features of In-Memory Data Grid
/**
* Copyright (c) 2013-2024 Nikita Koksharov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.redisson.api;
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Single;
import java.util.BitSet;
/**
* RxJava2 interface for BitSet object
*
* @author Nikita Koksharov
*
*/
public interface RBitSetRx extends RExpirableRx {
/**
* Returns signed number at specified
* offset
and size
*
* @param size - size of signed number up to 64 bits
* @param offset - offset of signed number
* @return signed number
*/
Single getSigned(int size, long offset);
/**
* Returns previous value of signed number and replaces it
* with defined value
at specified offset
*
* @param size - size of signed number up to 64 bits
* @param offset - offset of signed number
* @param value - value of signed number
* @return previous value of signed number
*/
Single setSigned(int size, long offset, long value);
/**
* Increments current signed value by
* defined increment
value and size
* at specified offset
* and returns result.
*
* @param size - size of signed number up to 64 bits
* @param offset - offset of signed number
* @param increment - increment value
* @return result value
*/
Single incrementAndGetSigned(int size, long offset, long increment);
/**
* Returns unsigned number at specified
* offset
and size
*
* @param size - size of unsigned number up to 64 bits
* @param offset - offset of unsigned number
* @return unsigned number
*/
Single getUnsigned(int size, long offset);
/**
* Returns previous value of unsigned number and replaces it
* with defined value
at specified offset
*
* @param size - size of unsigned number up to 64 bits
* @param offset - offset of unsigned number
* @param value - value of unsigned number
* @return previous value of unsigned number
*/
Single setUnsigned(int size, long offset, long value);
/**
* Increments current unsigned value by
* defined increment
value and size
* at specified offset
* and returns result.
*
* @param size - size of unsigned number up to 64 bits
* @param offset - offset of unsigned number
* @param increment - increment value
* @return result value
*/
Single incrementAndGetUnsigned(int size, long offset, long increment);
/**
* Returns byte number at specified offset
*
* @param offset - offset of number
* @return number
*/
Single getByte(long offset);
/**
* Returns previous value of byte number and replaces it
* with defined value
at specified offset
*
* @param offset - offset of number
* @param value - value of number
* @return previous value of number
*/
Single setByte(long offset, byte value);
/**
* Increments current byte value on defined increment
value at specified offset
* and returns result.
*
* @param offset - offset of number
* @param increment - increment value
* @return result value
*/
Single incrementAndGetByte(long offset, byte increment);
/**
* Returns short number at specified offset
*
* @param offset - offset of number
* @return number
*/
Single getShort(long offset);
/**
* Returns previous value of short number and replaces it
* with defined value
at specified offset
*
* @param offset - offset of number
* @param value - value of number
* @return previous value of number
*/
Single setShort(long offset, short value);
/**
* Increments current short value on defined increment
value at specified offset
* and returns result.
*
* @param offset - offset of number
* @param increment - increment value
* @return result value
*/
Single incrementAndGetShort(long offset, short increment);
/**
* Returns integer number at specified offset
*
* @param offset - offset of number
* @return number
*/
Single getInteger(long offset);
/**
* Returns previous value of integer number and replaces it
* with defined value
at specified offset
*
* @param offset - offset of number
* @param value - value of number
* @return previous value of number
*/
Single setInteger(long offset, int value);
/**
* Increments current integer value on defined increment
value at specified offset
* and returns result.
*
* @param offset - offset of number
* @param increment - increment value
* @return result value
*/
Single incrementAndGetInteger(long offset, int increment);
/**
* Returns long number at specified offset
*
* @param offset - offset of number
* @return number
*/
Single getLong(long offset);
/**
* Returns previous value of long number and replaces it
* with defined value
at specified offset
*
* @param offset - offset of number
* @param value - value of number
* @return previous value of number
*/
Single setLong(long offset, long value);
/**
* Increments current long value on defined increment
value at specified offset
* and returns result.
*
* @param offset - offset of number
* @param increment - increment value
* @return result value
*/
Single incrementAndGetLong(long offset, long increment);
Single toByteArray();
/**
* Returns "logical size" = index of highest set bit plus one.
* Returns zero if there are no any set bit.
*
* @return "logical size" = index of highest set bit plus one
*/
Single length();
/**
* Set all bits to value
from fromIndex
(inclusive) to toIndex
(exclusive)
*
* @param fromIndex inclusive
* @param toIndex exclusive
* @param value true = 1, false = 0
* @return void
*
*/
Completable set(long fromIndex, long toIndex, boolean value);
/**
* Set all bits to zero from fromIndex
(inclusive) to toIndex
(exclusive)
*
* @param fromIndex inclusive
* @param toIndex exclusive
* @return void
*
*/
Completable clear(long fromIndex, long toIndex);
/**
* Copy bits state of source BitSet object to this object
*
* @param bs - BitSet source
* @return void
*/
Completable set(BitSet bs);
/**
* Executes NOT operation over all bits
*
* @return void
*/
Completable not();
/**
* Set all bits to one from fromIndex
(inclusive) to toIndex
(exclusive)
*
* @param fromIndex inclusive
* @param toIndex exclusive
* @return void
*/
Completable set(long fromIndex, long toIndex);
/**
* Returns number of set bits.
*
* @return number of set bits.
*/
Single size();
/**
* Returns true
if bit set to one and false
overwise.
*
* @param bitIndex - index of bit
* @return true
if bit set to one and false
overwise.
*/
Single get(long bitIndex);
/**
* Set bit to one at specified bitIndex
*
* @param bitIndex - index of bit
* @return true
- if previous value was true,
* false
- if previous value was false
*/
Single set(long bitIndex);
/**
* Set bit to value
at specified bitIndex
*
* @param bitIndex - index of bit
* @param value true = 1, false = 0
* @return true
- if previous value was true,
* false
- if previous value was false
*/
Single set(long bitIndex, boolean value);
/**
* Returns the number of bits set to one.
*
* @return number of bits
*/
Single cardinality();
/**
* Set bit to zero at specified bitIndex
*
* @param bitIndex - index of bit
* @return true
- if previous value was true,
* false
- if previous value was false
*/
Single clear(long bitIndex);
/**
* Set all bits to zero
*
* @return void
*/
Completable clear();
/**
* Executes OR operation over this object and specified bitsets.
* Stores result into this object.
*
* @param bitSetNames - name of stored bitsets
* @return void
*/
Completable or(String... bitSetNames);
/**
* Executes AND operation over this object and specified bitsets.
* Stores result into this object.
*
* @param bitSetNames - name of stored bitsets
* @return void
*/
Completable and(String... bitSetNames);
/**
* Executes XOR operation over this object and specified bitsets.
* Stores result into this object.
*
* @param bitSetNames - name of stored bitsets
* @return void
*/
Completable xor(String... bitSetNames);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy